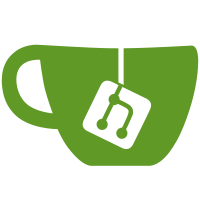
Added default parameter to GetCombinedSlaveValue method and revised calls to method to utilize default parameter Added code to convert xF8 to degree symbol Revised CheckNoteCautionTab to handle when step format data calls for separate boxes for notes and cautions Added BoxLeftAdj property to support Braidwood boxed items
1666 lines
51 KiB
C#
1666 lines
51 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using System.ComponentModel;
|
|
using System.Drawing;
|
|
using DescriptiveEnum;
|
|
using System.Xml;
|
|
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
[Serializable]
|
|
[TypeConverter(typeof(ExpandableObjectConverter))]
|
|
public class DocVersionConfig : ConfigDynamicTypeDescriptor, INotifyPropertyChanged
|
|
{
|
|
#region DynamicTypeDescriptor
|
|
internal override bool IsReadOnly
|
|
{
|
|
get { return _DocVersion == null; }
|
|
}
|
|
#endregion
|
|
#region XML
|
|
private XMLProperties _Xp;
|
|
private XMLProperties Xp
|
|
{
|
|
get { return _Xp; }
|
|
}
|
|
#endregion
|
|
#region Constructors
|
|
//PROPGRID: Hide ParentLookup
|
|
[Browsable(false)]
|
|
public bool ParentLookup
|
|
{
|
|
get { return _Xp.ParentLookup; }
|
|
set { _Xp.ParentLookup = value; }
|
|
}
|
|
//private bool _AncestorLookup;
|
|
////PROPGRID: Hide AncestorLookup
|
|
//[Browsable(false)]
|
|
//public bool AncestorLookup
|
|
//{
|
|
// get { return _AncestorLookup; }
|
|
// set { _AncestorLookup = value; }
|
|
//}
|
|
private DocVersion _DocVersion;
|
|
public DocVersionConfig(DocVersion docVersion)
|
|
{
|
|
_DocVersion = docVersion;
|
|
string xml = docVersion.Config;
|
|
if (xml == string.Empty) xml = "<Config/>";
|
|
_Xp = new XMLProperties(xml);
|
|
if (docVersion.MyDocVersionInfo.MyFolder != null) _Xp.LookInAncestor += new XMLPropertiesEvent(Xp_LookInAncestorFolder);
|
|
}
|
|
private string Xp_LookInAncestorFolder(object sender, XMLPropertiesArgs args)
|
|
{
|
|
if (args.AncestorLookup || ParentLookup)
|
|
{
|
|
for (FolderInfo folder = _DocVersion != null ? _DocVersion.MyDocVersionInfo.MyFolder : _DocVersionInfo.MyFolder; folder != null; folder = folder.MyParent)
|
|
{
|
|
string retval = folder.FolderConfig.GetValue(args.Group, args.Item);
|
|
if (retval != string.Empty) return retval;
|
|
}
|
|
}
|
|
return string.Empty;
|
|
}
|
|
//private string Xp_LookInAncestorFolderInfo(object sender, XMLPropertiesArgs args)
|
|
//{
|
|
// if (args.AncestorLookup || ParentLookup)
|
|
// {
|
|
// for (FolderInfo folder = _DocVersionInfo.MyFolder; folder != null; folder = folder.MyParent)
|
|
// {
|
|
// string retval = folder.FolderConfig.GetValue(args.Group, args.Item);
|
|
// if (retval != string.Empty) return retval;
|
|
// }
|
|
// }
|
|
// return string.Empty;
|
|
//}
|
|
private DocVersionInfo _DocVersionInfo;
|
|
public DocVersionConfig(DocVersionInfo docVersionInfo)
|
|
{
|
|
_DocVersionInfo = docVersionInfo;
|
|
string xml = docVersionInfo.Config;
|
|
if (xml == string.Empty) xml = "<Config/>";
|
|
_Xp = new XMLProperties(xml);
|
|
if (docVersionInfo.MyFolder != null) _Xp.LookInAncestor += new XMLPropertiesEvent(Xp_LookInAncestorFolder);
|
|
}
|
|
public DocVersionConfig(string xml)
|
|
{
|
|
_Xp = new XMLProperties(xml);
|
|
}
|
|
public DocVersionConfig()
|
|
{
|
|
string xml = "<Config/>";
|
|
_Xp = new XMLProperties(xml);
|
|
}
|
|
internal string GetValue(string group, string item)
|
|
{
|
|
return _Xp[group, item];
|
|
}
|
|
#endregion
|
|
#region Local Properties
|
|
[Category("General")]
|
|
[DisplayName("Name")]
|
|
[Description("Name")]
|
|
public string Name
|
|
{
|
|
get { return (_DocVersion != null ? _DocVersion.Name : _DocVersionInfo.Name); }
|
|
set { if (_DocVersion != null) _DocVersion.Name = value; }
|
|
}
|
|
[Category("General")]
|
|
[DisplayName("Procedure Set Revision")]
|
|
[Description("Westinghouse Owners Group Revision")]
|
|
public string ProcedureSetRev
|
|
{
|
|
get
|
|
{
|
|
return _Xp["ProcedureSet", "Revision"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["ProcedureSet", "Revision"] = value;
|
|
OnPropertyChanged("ProcedureSet_Revision");
|
|
}
|
|
}
|
|
[Category("General")]
|
|
//PROPGRID: Hide Title
|
|
[Browsable(false)]
|
|
[DisplayName("Title")]
|
|
[Description("Title")]
|
|
public string Title
|
|
{
|
|
get { return (_DocVersion != null ? _DocVersion.Title : _DocVersionInfo.Title); }
|
|
set { if (_DocVersion != null) _DocVersion.Title = value; }
|
|
}
|
|
[Category("Format Settings")]
|
|
[DisplayName("Format")]
|
|
[Description("Format")]
|
|
[TypeConverter(typeof(FormatList))]
|
|
public string FormatSelection
|
|
{
|
|
get
|
|
{
|
|
if (_DocVersion != null && _DocVersion.MyFormat != null) return _DocVersion.MyFormat.FullName;
|
|
if (_DocVersionInfo != null && _DocVersionInfo.MyFormat != null) return _DocVersionInfo.MyFormat.FullName;
|
|
return null;
|
|
}
|
|
set
|
|
{
|
|
if (_DocVersion != null)
|
|
{
|
|
_DocVersion.MyFormat = FormatList.ToFormat(value); // Can only be set if _DocVersion is set
|
|
//_DocVersion.ActiveFormat = null;
|
|
}
|
|
}
|
|
}
|
|
[Category("Format Settings")]
|
|
[DisplayName("Default Format")]
|
|
[Description("Default Format")]
|
|
[TypeConverter(typeof(FormatList))]
|
|
public string DefaultFormatSelection
|
|
{
|
|
get
|
|
{
|
|
if (_DocVersion != null && _DocVersion.MyDocVersionInfo.MyFolder != null && _DocVersion.MyDocVersionInfo.MyFolder.ActiveFormat != null) return _DocVersion.MyDocVersionInfo.MyFolder.ActiveFormat.FullName;
|
|
if (_DocVersionInfo != null && _DocVersionInfo.MyFolder != null && _DocVersionInfo.MyFolder.ActiveFormat != null) return _DocVersionInfo.MyFolder.ActiveFormat.FullName;
|
|
return null;
|
|
}
|
|
}
|
|
public DocVersion MyDocVersion
|
|
{ get { return _DocVersion; } }
|
|
private bool _SaveChangesToDocVersionConfig = true;
|
|
|
|
public bool SaveChangesToDocVersionConfig
|
|
{
|
|
get { return _SaveChangesToDocVersionConfig; }
|
|
set { _SaveChangesToDocVersionConfig = value; }
|
|
}
|
|
#endregion
|
|
#region ToString
|
|
public override string ToString()
|
|
{
|
|
string s = _Xp.ToString();
|
|
if (s == "<Config/>" || s == "<Config></Config>") return string.Empty;
|
|
return s;
|
|
}
|
|
#endregion
|
|
//<Config><RODefaults Setpoint="SP1" Graphics="IG1" ROPATH="g:\ops\vehlp\ro" /><PrintSettings ChangeBar="3" ChangeBarLoc="1" ChangeBarText="3" numcopies="1" Watermark="1" userformat=" " disableduplex="False" /><format plant="OHLP" /></Config>
|
|
|
|
#region RODefaults // From proc.ini
|
|
[Category("Referenced Objects")]
|
|
[DisplayName("Default RO Prefix")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Setpoint Prefix")]
|
|
public string RODefaults_setpointprefix
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["RODefaults", "Setpoint"];// get the saved value
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("RODefaults", "Setpoint"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
s = "SP1";// default to volian default
|
|
|
|
return s;
|
|
}
|
|
set
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("RODefaults", "Setpoint"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = "SP1";
|
|
|
|
if (parval.Equals(value))
|
|
_Xp["RODefaults", "Setpoint"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["RODefaults", "Setpoint"] = value; // save selected value
|
|
|
|
OnPropertyChanged("RODefaults_setpointprefix");
|
|
}
|
|
}
|
|
[Category("Referenced Objects")]
|
|
[DisplayName("Default Graphics Prefix")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Graphics Prefix")]
|
|
public string RODefaults_graphicsprefix
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["RODefaults", "Graphics"];// get the saved value
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("RODefaults", "Graphics"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
s = "IG1";// default to volian default
|
|
|
|
return s;
|
|
}
|
|
set
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("RODefaults", "Graphics"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = "IG1";
|
|
|
|
if (parval.Equals(value))
|
|
_Xp["RODefaults", "Graphics"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["RODefaults", "Graphics"] = value; // save selected value
|
|
|
|
OnPropertyChanged("RODefaults_graphicsprefix");
|
|
}
|
|
}
|
|
#endregion
|
|
#region PrintSettingsCategory // From curset.dat
|
|
[Category("Print Settings")]
|
|
//PROPGRID: Hide Printer
|
|
[Browsable(false)]
|
|
[DisplayName("Number of Copies")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Number of Copies")]
|
|
public int Print_NumCopies
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "numcopies"];
|
|
if (s == string.Empty) return 1;
|
|
return int.Parse(_Xp["PrintSettings", "numcopies"]);
|
|
}
|
|
set
|
|
{
|
|
_Xp["PrintSettings", "numcopies"] = value.ToString();
|
|
OnPropertyChanged("Print_NumCopies");
|
|
}
|
|
}
|
|
[Category("Print Settings")]
|
|
//PROPGRID: Hide Printer
|
|
[Browsable(false)]
|
|
[DisplayName("Printer")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Printer")]
|
|
public string Print_Printer
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "Printer"];
|
|
return s;
|
|
}
|
|
set
|
|
{
|
|
_Xp["PrintSettings", "Printer"] = value.ToString();
|
|
OnPropertyChanged("Print_Printer");
|
|
}
|
|
}
|
|
//[TypeConverter(typeof(EnumDescConverter))]
|
|
//public enum PrintWatermark : int
|
|
//{
|
|
// None = 0, Reference, Draft, Master, Sample,
|
|
// [Description("Information Only")]
|
|
// InformationOnly
|
|
//}
|
|
|
|
[Category("Print Settings")]
|
|
[DisplayName("Watermark")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Watermark")]
|
|
public PrintWatermark Print_Watermark
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "Watermark"];
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("PrintSettings", "Watermark"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
return PrintWatermark.Draft;// default to volian default
|
|
|
|
return (PrintWatermark)int.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("PrintSettings", "Watermark"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = ((int)(PrintWatermark.Draft)).ToString();
|
|
|
|
if (parval.Equals(((int)value).ToString()))
|
|
_Xp["PrintSettings", "Watermark"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["PrintSettings", "Watermark"] = ((int)value).ToString(); // save selected value
|
|
|
|
OnPropertyChanged("Print_Watermark");
|
|
}
|
|
}
|
|
[Category("Print Settings")]
|
|
[DisplayName("Disable Automatic Duplexing")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Disable Duplex Printing")]
|
|
public bool Print_DisableDuplex
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "disableduplex"];
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("PrintSettings", "disableduplex"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
s = "false";// default to volian default
|
|
|
|
return bool.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("PrintSettings", "disableduplex"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = "false";
|
|
|
|
if (parval.Equals(value.ToString()))
|
|
_Xp["PrintSettings", "disableduplex"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["PrintSettings", "disableduplex"] = value.ToString(); // save selected value
|
|
|
|
OnPropertyChanged("Print_DisableDuplex");
|
|
}
|
|
}
|
|
//// Change Bar Use from 16-bit code:
|
|
//// No Default
|
|
//// Without Change Bars
|
|
//// With Default Change Bars
|
|
//// With User Specified Change Bars
|
|
//[TypeConverter(typeof(EnumDescConverter))]
|
|
//public enum PrintChangeBar : int
|
|
//{
|
|
// [Description("No Default, Select Before Printing")]
|
|
// SelectBeforePrinting = 0,
|
|
// [Description("Without Change Bars")]
|
|
// Without,
|
|
// [Description("With Default Change Bars")]
|
|
// WithDefault,
|
|
// [Description("Use Custom Change Bars")]
|
|
// WithUserSpecified
|
|
//}
|
|
|
|
[Category("Format Settings")]
|
|
[DisplayName("Change Bars")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Change Bar Use")]
|
|
public PrintChangeBar Print_ChangeBar
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "ChangeBar"];
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("PrintSettings", "ChangeBar"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
return PrintChangeBar.SelectBeforePrinting;// default to volian default
|
|
|
|
return (PrintChangeBar)int.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
if (_SaveChangesToDocVersionConfig)
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("PrintSettings", "ChangeBar"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = ((int)(PrintChangeBar.SelectBeforePrinting)).ToString();
|
|
|
|
if (parval.Equals(((int)value).ToString()))
|
|
_Xp["PrintSettings", "ChangeBar"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["PrintSettings", "ChangeBar"] = ((int)value).ToString(); // save selected value
|
|
|
|
OnPropertyChanged("Print_ChangeBar");
|
|
}
|
|
}
|
|
}
|
|
//// User Specified Change Bar Location from16-bit code:
|
|
//// With Text
|
|
//// Outside Box
|
|
//// AER on LEFT, RNO on Right
|
|
//// To the Left of Text
|
|
//[TypeConverter(typeof(EnumDescConverter))]
|
|
//public enum PrintChangeBarLoc : int
|
|
//{
|
|
// [Description("With Text")]
|
|
// WithText = 0,
|
|
// [Description("Outside Box")]
|
|
// OutsideBox,
|
|
// [Description("AER on Left RNO on Right")]
|
|
// AERleftRNOright,
|
|
// [Description("To the Left of the Text")]
|
|
// LeftOfText
|
|
//}
|
|
[Category("Format Settings")]
|
|
[DisplayName("Change Bar Position")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("User Specified Change Bar Location")]
|
|
public PrintChangeBarLoc Print_ChangeBarLoc
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "ChangeBarLoc"];
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("PrintSettings", "ChangeBarLoc"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
return PrintChangeBarLoc.WithText;// default to volian default
|
|
|
|
return (PrintChangeBarLoc)int.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
if (_SaveChangesToDocVersionConfig)
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("PrintSettings", "ChangeBarLoc"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = ((int)(PrintChangeBarLoc.WithText)).ToString();
|
|
|
|
if (parval.Equals(((int)value).ToString()))
|
|
_Xp["PrintSettings", "ChangeBarLoc"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["PrintSettings", "ChangeBarLoc"] = ((int)value).ToString(); // save selected value
|
|
|
|
OnPropertyChanged("Print_ChangeBarLoc");
|
|
}
|
|
}
|
|
}
|
|
|
|
//// Change Bar Text from16-bit code:
|
|
//// Date and Change ID
|
|
//// Revision Number
|
|
//// Change ID
|
|
//// No Change Bar Message
|
|
//// User Defined Message
|
|
//[TypeConverter(typeof(EnumDescConverter))]
|
|
//public enum PrintChangeBarText : int
|
|
//{
|
|
// [Description("Date and Change ID")]
|
|
// DateChgID = 0,
|
|
// [Description("Revision Number")]
|
|
// RevNum,
|
|
// [Description("Change ID")]
|
|
// ChgID,
|
|
// [Description("No Change Bar Text")]
|
|
// None,
|
|
// [Description("Custom Change Bar Text")]
|
|
// UserDef
|
|
//}
|
|
[Category("Format Settings")]
|
|
[DisplayName("Change Bar Text Type")]
|
|
[Description("Change Bar Text")]
|
|
public PrintChangeBarText Print_ChangeBarText
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "ChangeBarText"];
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("PrintSettings", "ChangeBarText"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
return PrintChangeBarText.DateChgID;// default to volian default
|
|
|
|
return (PrintChangeBarText)int.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
if (_SaveChangesToDocVersionConfig)
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("PrintSettings", "ChangeBarText"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = ((int)(PrintChangeBarText.DateChgID)).ToString();
|
|
|
|
if (parval.Equals(((int)value).ToString()))
|
|
_Xp["PrintSettings", "ChangeBarText"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["PrintSettings", "ChangeBarText"] = ((int)value).ToString(); // save selected value
|
|
|
|
OnPropertyChanged("Print_ChangeBarText");
|
|
}
|
|
}
|
|
}
|
|
[Category("Format Settings")]
|
|
[DisplayName("Custom Change Bar Message Line One")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("User Change Bar Message1")]
|
|
public string Print_UserCBMess1
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "usercbmess1"];// get the saved value
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("PrintSettings", "usercbmess1"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
return "";// default to volian default
|
|
|
|
return s;
|
|
}
|
|
set
|
|
{
|
|
if (_SaveChangesToDocVersionConfig)
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("PrintSettings", "usercbmess1"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = "";
|
|
|
|
if (parval.Equals(value))
|
|
_Xp["PrintSettings", "usercbmess1"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["PrintSettings", "usercbmess1"] = value; // save selected value
|
|
|
|
OnPropertyChanged("Print_UserCBMess1");
|
|
}
|
|
}
|
|
}
|
|
[Category("Format Settings")]
|
|
[DisplayName("Custom Change Bar Message Line Two")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("User Change Bar Message2")]
|
|
public string Print_UserCBMess2
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "usercbmess2"];// get the saved value
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("PrintSettings", "usercbmess2"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
return "";// default to volian default
|
|
|
|
return s;
|
|
}
|
|
set
|
|
{
|
|
if (_SaveChangesToDocVersionConfig)
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("PrintSettings", "usercbmess2"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = "";
|
|
|
|
if (parval.Equals(value))
|
|
_Xp["PrintSettings", "usercbmess2"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["PrintSettings", "usercbmess2"] = value; // save selected value
|
|
|
|
OnPropertyChanged("Print_UserCBMess2");
|
|
}
|
|
}
|
|
}
|
|
[Category("Print Settings")]
|
|
//PROPGRID: Hide User Format
|
|
[Browsable(false)]
|
|
[DisplayName("User Format")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("User Format")]
|
|
public string Print_UserFormat
|
|
{
|
|
get
|
|
{
|
|
return _Xp["PrintSettings", "userformat"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["PrintSettings", "userformat"] = value;
|
|
OnPropertyChanged("Print_UserFormat");
|
|
}
|
|
}
|
|
//public enum PrintPagination : int
|
|
//{
|
|
// Free = 0, Fixed, Auto
|
|
//}
|
|
[Category("Print Settings")]
|
|
[DisplayName("Pagination")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Pagination")]
|
|
public PrintPagination Print_Pagination
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "Pagination"];
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("PrintSettings", "Pagination"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
return PrintPagination.Auto;// default to volian default
|
|
|
|
return (PrintPagination)int.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("PrintSettings", "Pagination"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
parval = ((int)(PrintPagination.Auto)).ToString();
|
|
|
|
if (parval.Equals(((int)value).ToString()))
|
|
_Xp["PrintSettings", "Pagination"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["PrintSettings", "Pagination"] = ((int)value).ToString(); // save selected value
|
|
|
|
OnPropertyChanged("Print_Pagination");
|
|
}
|
|
}
|
|
[Category("Print Settings")]
|
|
[DisplayName("PDFLocation")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("PDF Location")]
|
|
public string Print_PDFLocation
|
|
{
|
|
get
|
|
{
|
|
return _Xp["PrintSettings", "PDFLocation"];
|
|
}
|
|
set
|
|
{
|
|
if (_SaveChangesToDocVersionConfig)
|
|
{
|
|
_Xp["PrintSettings", "PDFLocation"] = value; // save selected value
|
|
OnPropertyChanged("Print_PDFLocation");
|
|
}
|
|
}
|
|
}
|
|
[Category("Print Settings")]
|
|
[DisplayName("AlwaysOverwritePDF")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Always Overwrite PDF File")]
|
|
public bool Print_AlwaysOverwritePDF
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "AlwaysOverwritePDF"];
|
|
|
|
// If there is no value, then default to true
|
|
if (s == string.Empty)
|
|
s = "true"; // default
|
|
|
|
return bool.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
if (_SaveChangesToDocVersionConfig)
|
|
{
|
|
string parval = _Xp.ParentValue("PrintSettings", "AlwaysOverwritePDF");
|
|
|
|
_Xp["PrintSettings", "AlwaysOverwritePDF"] = value.ToString();
|
|
|
|
OnPropertyChanged("Print_AlwaysOverwritePDF");
|
|
}
|
|
}
|
|
}
|
|
[Category("Print Settings")]
|
|
[DisplayName("AlwaysViewPDFAfterCreate")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Always View PDF File After Create")]
|
|
public bool Print_AlwaysViewPDFAfterCreate
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "AlwaysViewPDFAfterCreate"];
|
|
|
|
// If there is no value, then default to true
|
|
if (s == string.Empty)
|
|
s = "true"; // default
|
|
|
|
return bool.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
if (_SaveChangesToDocVersionConfig)
|
|
{
|
|
string parval = _Xp.ParentValue("PrintSettings", "AlwaysViewPDFAfterCreate");
|
|
|
|
_Xp["PrintSettings", "AlwaysViewPDFAfterCreate"] = value.ToString();
|
|
|
|
OnPropertyChanged("Print_AlwaysViewPDFAfterCreate");
|
|
}
|
|
}
|
|
}
|
|
[Category("Print Settings")]
|
|
[DisplayName("AddBlankPagesWhenUsingDuplexFoldouts")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Add Blank Pages in Procedures that Print Duplex Foldouts")]
|
|
public bool Print_AddBlankPagesWhenUsingDuplexFoldouts
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "AddBlankPagesWhenUsingDuplexFoldouts"];
|
|
|
|
// If there is no value, then default to true
|
|
if (s == string.Empty)
|
|
s = "false"; // default
|
|
|
|
return bool.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
if (_SaveChangesToDocVersionConfig)
|
|
{
|
|
string parval = _Xp.ParentValue("PrintSettings", "AddBlankPagesWhenUsingDuplexFoldouts");
|
|
|
|
_Xp["PrintSettings", "AddBlankPagesWhenUsingDuplexFoldouts"] = value.ToString();
|
|
|
|
OnPropertyChanged("Print_AddBlankPagesWhenUsingDuplexFoldouts");
|
|
}
|
|
}
|
|
}
|
|
#endregion
|
|
#region Unit // From PROC.INI
|
|
//MultiUnitCount
|
|
[Category("Unit")]
|
|
[DisplayName("Unit Count")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Number of Units")]
|
|
public int Unit_Count
|
|
{
|
|
get
|
|
{
|
|
return _Xp.XmlContents.SelectNodes("//Slave").Count;
|
|
}
|
|
}
|
|
private int _SelectedSlave = 0;
|
|
//[Browsable(false)]
|
|
public int SelectedSlave
|
|
{
|
|
get { return _SelectedSlave; }
|
|
set { _SelectedSlave = value; }//Volian.Base.Library.vlnStackTrace.ShowStackLocal("SelectedSlave = {0}", _SelectedSlave.ToString()); }
|
|
}
|
|
[Category("Unit")]
|
|
[DisplayName("Unit Number")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Assigned Unit Number")]
|
|
public string Unit_Number
|
|
{
|
|
get
|
|
{
|
|
string s = GetCombinedSlaveValue("Number") ?? _Xp["Unit", "Number"];// get the saved value
|
|
if (SelectedSlave > 0)
|
|
s = _Xp["Slave[@index='" + SelectedSlave.ToString() + "']", "Number"];
|
|
return s;
|
|
}
|
|
set
|
|
{
|
|
if (SelectedSlave > 0)
|
|
_Xp["Slave[@index='" + SelectedSlave.ToString() + "']", "Number"] = value; // save selected value
|
|
else
|
|
_Xp["Unit", "Number"] = value;
|
|
OnPropertyChanged("Unit_Number");
|
|
}
|
|
}
|
|
[Browsable(false)]
|
|
public string Old_Index
|
|
{
|
|
get
|
|
{
|
|
string s = "";
|
|
if (SelectedSlave > 0)
|
|
s = _Xp["Slave[@index='" + SelectedSlave.ToString() + "']", "oldindex"];
|
|
return s;
|
|
}
|
|
}
|
|
|
|
[Category("Unit")]
|
|
[DisplayName("Other Unit Number")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Assigned Other Unit Number")]
|
|
public string Other_Unit_Number
|
|
{
|
|
get
|
|
{
|
|
string s = GetCombinedSlaveValue("OtherNumber") ?? _Xp["Unit", "OtherNumber"];// get the saved value
|
|
if (SelectedSlave > 0)
|
|
s = _Xp["Slave[@index='" + SelectedSlave.ToString() + "']", "OtherNumber"];
|
|
return s;
|
|
}
|
|
set
|
|
{
|
|
if (SelectedSlave > 0)
|
|
_Xp["Slave[@index='" + SelectedSlave.ToString() + "']", "OtherNumber"] = value; // save selected value
|
|
else
|
|
_Xp["Unit", "OtherNumber"] = value;
|
|
OnPropertyChanged("Other_Unit_Number");
|
|
}
|
|
}
|
|
[Category("Unit")]
|
|
[DisplayName("Unit Name")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Assigned Unit Name")]
|
|
public string Unit_Name
|
|
{
|
|
get
|
|
{
|
|
string s = GetCombinedSlaveValue("Name") ?? _Xp["Unit", "Name"];// get the saved value
|
|
if (SelectedSlave > 0)
|
|
s = _Xp["Slave[@index='" + SelectedSlave.ToString() + "']", "Name"];
|
|
return s;
|
|
}
|
|
set
|
|
{
|
|
if (SelectedSlave > 0)
|
|
_Xp["Slave[@index='" + SelectedSlave.ToString() + "']", "Name"] = value; // save selected value
|
|
else
|
|
_Xp["Unit", "Name"] = value;
|
|
OnPropertyChanged("Unit_Name");
|
|
}
|
|
}
|
|
[Category("Unit")]
|
|
[DisplayName("Other Unit Name")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Assigned Other Unit Name")]
|
|
public string Other_Unit_Name
|
|
{
|
|
get
|
|
{
|
|
string s = GetCombinedSlaveValue("OtherName") ?? _Xp["Unit", "OtherName"];// get the saved value
|
|
if (SelectedSlave > 0)
|
|
s = _Xp["Slave[@index='" + SelectedSlave.ToString() + "']", "OtherName"];
|
|
return s;
|
|
}
|
|
set
|
|
{
|
|
if (SelectedSlave > 0)
|
|
_Xp["Slave[@index='" + SelectedSlave.ToString() + "']", "OtherName"] = value; // save selected value
|
|
else
|
|
_Xp["Unit", "OtherName"] = value;
|
|
OnPropertyChanged("Other_Unit_Name");
|
|
}
|
|
}
|
|
[Category("Unit")]
|
|
[DisplayName("Unit Text")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Assigned Unit Text")]
|
|
public string Unit_Text
|
|
{
|
|
get
|
|
{
|
|
string s = GetCombinedSlaveValue("Text") ?? _Xp["Unit", "Text"];// get the saved value
|
|
if (SelectedSlave > 0)
|
|
s = _Xp["Slave[@index='" + SelectedSlave.ToString() + "']", "Text"];
|
|
return s;
|
|
}
|
|
set
|
|
{
|
|
if (SelectedSlave > 0)
|
|
_Xp["Slave[@index='" + SelectedSlave.ToString() + "']", "Text"] = value; // save selected value
|
|
else
|
|
_Xp["Unit", "Text"] = value;
|
|
OnPropertyChanged("Unit_Text");
|
|
}
|
|
}
|
|
[Category("Unit")]
|
|
[DisplayName("Other Unit Text")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Assigned Other Unit Text")]
|
|
public string Other_Unit_Text
|
|
{
|
|
get
|
|
{
|
|
string s = GetCombinedSlaveValue("OtherText") ?? _Xp["Unit", "OtherText"];// get the saved value
|
|
if (SelectedSlave > 0)
|
|
s = _Xp["Slave[@index='" + SelectedSlave.ToString() + "']", "OtherText"];
|
|
return s;
|
|
}
|
|
set
|
|
{
|
|
if (SelectedSlave > 0)
|
|
_Xp["Slave[@index='" + SelectedSlave.ToString() + "']", "OtherText"] = value; // save selected value
|
|
else
|
|
_Xp["Unit", "OtherText"] = value;
|
|
OnPropertyChanged("Other_Unit_Text");
|
|
}
|
|
}
|
|
[Category("Unit")]
|
|
[DisplayName("Unit ID")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Assigned Unit ID")]
|
|
public string Unit_ID
|
|
{
|
|
get
|
|
{
|
|
string s = GetCombinedSlaveValue("ID") ?? _Xp["Unit", "ID"];// get the saved value
|
|
if (SelectedSlave > 0)
|
|
s = _Xp["Slave[@index='" + SelectedSlave.ToString() + "']", "ID"];
|
|
return s;
|
|
}
|
|
set
|
|
{
|
|
if (SelectedSlave > 0)
|
|
_Xp["Slave[@index='" + SelectedSlave.ToString() + "']", "ID"] = value; // save selected value
|
|
else
|
|
_Xp["Unit", "ID"] = value;
|
|
OnPropertyChanged("Unit_ID");
|
|
}
|
|
}
|
|
[Category("Unit")]
|
|
[DisplayName("Other Unit ID")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Assigned Other Unit ID")]
|
|
public string Other_Unit_ID
|
|
{
|
|
get
|
|
{
|
|
string s = GetCombinedSlaveValue("OtherID") ?? _Xp["Unit", "OtherID"];// get the saved value
|
|
if (SelectedSlave > 0)
|
|
s = _Xp["Slave[@index='" + SelectedSlave.ToString() + "']", "OtherID"];
|
|
return s;
|
|
}
|
|
set
|
|
{
|
|
if (SelectedSlave > 0)
|
|
_Xp["Slave[@index='" + SelectedSlave.ToString() + "']", "OtherID"] = value; // save selected value
|
|
else
|
|
_Xp["Unit", "OtherID"] = value;
|
|
OnPropertyChanged("Other_Unit_ID");
|
|
}
|
|
}
|
|
[Category("Unit")]
|
|
[DisplayName("Unit Specific Procedure Number")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Assigned Unit Specific Procedure Number")]
|
|
public string Unit_ProcedureNumber
|
|
{
|
|
get
|
|
{
|
|
string s = GetCombinedSlaveValue("ProcedureNumber", null) ?? _Xp["Unit", "ProcedureNumber"];// get the saved value
|
|
if (SelectedSlave > 0)
|
|
s = _Xp["Slave[@index='" + SelectedSlave.ToString() + "']", "ProcedureNumber"];
|
|
return s;
|
|
}
|
|
set
|
|
{
|
|
if (SelectedSlave > 0)
|
|
_Xp["Slave[@index='" + SelectedSlave.ToString() + "']", "ProcedureNumber"] = value; // save selected value
|
|
else
|
|
_Xp["Unit", "ProcedureNumber"] = value;
|
|
OnPropertyChanged("Unit_ProcedureNumber");
|
|
}
|
|
}
|
|
[Category("Unit")]
|
|
[DisplayName("Unit Specific Procedure Set Name")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Assigned Unit Specific Procedure Set Name")]
|
|
public string Unit_ProcedureSetName
|
|
{
|
|
get
|
|
{
|
|
string s = GetCombinedSlaveValue("SetName", null) ?? _Xp["ProcedureSet", "Name"];// get the saved value
|
|
if (SelectedSlave > 0)
|
|
s = _Xp["Slave[@index='" + SelectedSlave.ToString() + "']", "SetName"];
|
|
return s;
|
|
}
|
|
set
|
|
{
|
|
if (SelectedSlave > 0)
|
|
_Xp["Slave[@index='" + SelectedSlave.ToString() + "']", "SetName"] = value; // save selected value
|
|
else
|
|
_Xp["ProcedureSet", "Name"] = value;
|
|
OnPropertyChanged("Unit_ProcedureSetName");
|
|
}
|
|
}
|
|
[Category("Unit")]
|
|
[DisplayName("Unit Specific Procedure Set ID")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Assigned Unit Specific Procedure Set ID")]
|
|
public string Unit_ProcedureSetID
|
|
{
|
|
get
|
|
{
|
|
string s = GetCombinedSlaveValue("SetID", null) ?? _Xp["ProcedureSet", "ID"];// get the saved value
|
|
if (SelectedSlave > 0)
|
|
s = _Xp["Slave[@index='" + SelectedSlave.ToString() + "']", "SetID"];
|
|
return s;
|
|
}
|
|
set
|
|
{
|
|
if (SelectedSlave > 0)
|
|
_Xp["Slave[@index='" + SelectedSlave.ToString() + "']", "SetID"] = value; // save selected value
|
|
else
|
|
_Xp["ProcedureSet", "ID"] = value;
|
|
OnPropertyChanged("Unit_ProcedureSetID");
|
|
}
|
|
}
|
|
#endregion
|
|
#region DELETE_ME
|
|
/*
|
|
#region ColorCategory // From veproms.ini
|
|
// Note that not all possibilities from 16-bit will be added here, until
|
|
// it is determined how these will be used
|
|
[Category("Color")]
|
|
[DisplayName("ro")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("ro")]
|
|
public string Color_ro
|
|
{
|
|
get
|
|
{
|
|
return _Xp["Color", "ro"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["Color", "ro"] = value;
|
|
OnPropertyChanged("Color_ro");
|
|
}
|
|
}
|
|
[Category("Color")]
|
|
[DisplayName("editbackground")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("editbackground")]
|
|
public string Color_editbackground
|
|
{
|
|
get
|
|
{
|
|
return _Xp["Color", "editbackground"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["Color", "editbackground"] = value;
|
|
OnPropertyChanged("Color_editbackground");
|
|
}
|
|
}
|
|
[Category("Color")]
|
|
[DisplayName("black")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("black")]
|
|
public string Color_black
|
|
{
|
|
get
|
|
{
|
|
return _Xp["Color", "black"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["Color", "black"] = value;
|
|
OnPropertyChanged("Color_black");
|
|
}
|
|
}
|
|
[Category("Color")]
|
|
[DisplayName("blue")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("blue")]
|
|
public string Color_blue
|
|
{
|
|
get
|
|
{
|
|
return _Xp["Color", "blue"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["Color", "blue"] = value;
|
|
OnPropertyChanged("Color_blue");
|
|
}
|
|
}
|
|
#endregion // From veproms.ini
|
|
#region SystemPrintCategory // From veproms.ini
|
|
[Category("System Print")]
|
|
[DisplayName("Underline Width")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Underline Width")]
|
|
public int SysPrint_UWidth
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["SystemPrint", "UnderlineWidth"];
|
|
if (s == string.Empty) return 10;
|
|
return int.Parse(_Xp["SystemPrint", "UnderlineWidth"]);
|
|
}
|
|
set
|
|
{
|
|
_Xp["SystemPrint", "UnderlineWidth"] = value.ToString();
|
|
OnPropertyChanged("SysPrint_UWidth");
|
|
}
|
|
}
|
|
[Category("System Print")]
|
|
[DisplayName("Vertical Offset")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Vertical Offset")]
|
|
public int SysPrint_VOffset
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["SystemPrint", "VerticalOffset"];
|
|
if (s == string.Empty) return 0;
|
|
return int.Parse(_Xp["SystemPrint", "VerticalOffset"]);
|
|
}
|
|
set
|
|
{
|
|
_Xp["SystemPrint", "VerticalOffset"] = value.ToString();
|
|
OnPropertyChanged("SysPrint_VOffset");
|
|
}
|
|
}
|
|
[Category("System Print")]
|
|
[DisplayName("Stroke Width")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Stroke Width")]
|
|
public int SysPrint_StrokeWidth
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["SystemPrint", "StrokeWidth"];
|
|
if (s == string.Empty) return 0;
|
|
return int.Parse(_Xp["SystemPrint", "StrokeWidth"]);
|
|
}
|
|
set
|
|
{
|
|
_Xp["SystemPrint", "StrokeWidth"] = value.ToString();
|
|
OnPropertyChanged("SysPrint_StrokeWidth");
|
|
}
|
|
}
|
|
[Category("System Print")]
|
|
[DisplayName("Stroke Width Bold")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Stroke Width Bold")]
|
|
public int SysPrint_StrokeWidthBold
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["SystemPrint", "StrokeWidthBold"];
|
|
if (s == string.Empty) return 0;
|
|
return int.Parse(_Xp["SystemPrint", "StrokeWidthBold"]);
|
|
}
|
|
set
|
|
{
|
|
_Xp["SystemPrint", "StrokeWidthBold"] = value.ToString();
|
|
OnPropertyChanged("SysPrint_StrokeWidthBold");
|
|
}
|
|
}
|
|
#endregion
|
|
#region StartupCategory // From veproms.ini
|
|
[Category("Startup")]
|
|
[DisplayName("Startup MessageBox Title")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Startup MessageBox Title")]
|
|
public string Startup_MsgTitle
|
|
{
|
|
get
|
|
{
|
|
return _Xp["Startup", "MessageBoxTitle"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["Startup", "MessageBoxTitle"] = value;
|
|
OnPropertyChanged("Startup_MsgTitle");
|
|
}
|
|
}
|
|
[Category("Startup")]
|
|
[DisplayName("Startup Message File")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Startup Message File")]
|
|
public string Startup_MsgFile
|
|
{
|
|
get
|
|
{
|
|
return _Xp["Startup", "MessageFile"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["Startup", "MessageFile"] = value;
|
|
OnPropertyChanged("Startup_MsgFile");
|
|
}
|
|
}
|
|
#endregion
|
|
#region ProcedureListTabStopsCategory // From veproms.ini
|
|
[Category("ProcedureListTabStops")]
|
|
[DisplayName("Procedure Number Tab")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Procedure Number Tab")]
|
|
public int ProcListTab_Number
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["ProcedureListTabStops", "ProcedureNumberTab"];
|
|
if (s == string.Empty) return 75;
|
|
return int.Parse(_Xp["ProcedureListTabStops", "ProcedureNumberTab"]);
|
|
}
|
|
set
|
|
{
|
|
_Xp["ProcedureListTabStops", "ProcedureNumberTab"] = value.ToString();
|
|
OnPropertyChanged("ProcListTab_Number");
|
|
}
|
|
}
|
|
[Category("ProcedureListTabStops")]
|
|
[DisplayName("Procedure Title Tab")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Procedure Title Tab")]
|
|
public int ProcListTab_Title
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["ProcedureListTabStops", "ProcedureTitleTab"];
|
|
if (s == string.Empty) return 175;
|
|
return int.Parse(_Xp["ProcedureListTabStops", "ProcedureTitleTab"]);
|
|
}
|
|
set
|
|
{
|
|
_Xp["ProcedureListTabStops", "ProcedureTitleTab"] = value.ToString();
|
|
OnPropertyChanged("ProcListTab_Title");
|
|
}
|
|
}
|
|
#endregion
|
|
#region FormatCategory
|
|
public enum FormatColumns : int
|
|
{
|
|
Default=0,OneColumn,TwoColumn,ThreeColumn,FourColumns
|
|
}
|
|
[Category("Format")]
|
|
[DisplayName("Column Layout")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Column Layout")]
|
|
public FormatColumns Format_Columns
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["format", "columns"];
|
|
if (s == string.Empty) return (FormatColumns)0;
|
|
return (FormatColumns)int.Parse(_Xp["format", "columns"]);
|
|
//return Enum.Parse(typeof(FormatColumns),_Xp["format", "columns"]);
|
|
}
|
|
set
|
|
{
|
|
if (value == 0) _Xp["format", "columns"] = string.Empty;
|
|
else _Xp["format", "columns"] = ((int)value).ToString();
|
|
OnPropertyChanged("Format_Columns");
|
|
}
|
|
}
|
|
[Category("Format")]
|
|
[DisplayName("Plant Format Name")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Default Plant Format")]
|
|
public string Format_Plant
|
|
{
|
|
get
|
|
{ return _Xp["format", "plant"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["format", "plant"] = value;
|
|
OnPropertyChanged("Format_Plant");
|
|
}
|
|
}
|
|
#endregion
|
|
#region DefaultsCategory // from proc.ini
|
|
[Category("Defaults")]
|
|
[DisplayName("Default Setpoint Prefix")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Default Setpoint Prefix")]
|
|
public string Default_SPPrefix
|
|
{
|
|
get { return _Xp["default", "spprefix"]; }
|
|
set
|
|
{
|
|
_Xp["default", "spprefix"] = value;
|
|
OnPropertyChanged("Default_SPPrefix");
|
|
}
|
|
}
|
|
[Category("Defaults")]
|
|
[DisplayName("Default Image Prefix")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Default Image Prefix")]
|
|
public string Default_IMPrefix
|
|
{
|
|
get { return _Xp["default", "imprefix"]; }
|
|
set
|
|
{
|
|
_Xp["default", "imprefix"] = value;
|
|
OnPropertyChanged("RO_IMPrefix");
|
|
}
|
|
}
|
|
#endregion
|
|
#region PrintSettingsCategory // From curset.dat
|
|
[Category("Print Settings")]
|
|
[DisplayName("Number of Copies")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Number of Copies")]
|
|
public int Print_NumCopies
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "numcopies"];
|
|
if (s == string.Empty) return 1;
|
|
return int.Parse(_Xp["PrintSettings", "numcopies"]);
|
|
}
|
|
set
|
|
{
|
|
_Xp["PrintSettings", "numcopies"] = value.ToString();
|
|
OnPropertyChanged("Print_NumCopies");
|
|
}
|
|
}
|
|
public enum PrintPagination : int
|
|
{
|
|
Free = 0, Fixed, Auto
|
|
}
|
|
[Category("Print Settings")]
|
|
[DisplayName("Pagination")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Pagination")]
|
|
public PrintPagination Print_Pagination
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "Pagination"];
|
|
if (s == string.Empty) return PrintPagination.Auto;
|
|
return (PrintPagination)int.Parse(_Xp["PrintSettings", "Pagination"]);
|
|
}
|
|
set
|
|
{
|
|
if (value == PrintPagination.Auto) _Xp["PrintSettings", "Pagination"] = string.Empty;
|
|
else _Xp["PrintSettings", "Pagination"] = ((int)value).ToString();
|
|
OnPropertyChanged("Print_Pagination");
|
|
}
|
|
}
|
|
public enum PrintWatermark : int
|
|
{
|
|
None = 0, Reference, Draft, Master, Sample, InformationOnly
|
|
}
|
|
[Category("Print Settings")]
|
|
[DisplayName("Watermark")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Watermark")]
|
|
public PrintWatermark Print_Watermark
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "Watermark"];
|
|
if (s == string.Empty) return PrintWatermark.Draft;
|
|
return (PrintWatermark)int.Parse(_Xp["PrintSettings", "Watermark"]);
|
|
}
|
|
set
|
|
{
|
|
if (value == PrintWatermark.Draft) _Xp["PrintSettings", "Watermark"] = string.Empty;
|
|
else _Xp["PrintSettings", "Watermark"] = ((int)value).ToString();
|
|
OnPropertyChanged("Print_Watermark");
|
|
}
|
|
}
|
|
[Category("Print Settings")]
|
|
[DisplayName("Disable Duplex Printing")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Disable Duplex Printing")]
|
|
public bool Print_DisableDuplex
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "disableduplex"];
|
|
if (s == string.Empty) return false;
|
|
return bool.Parse(_Xp["PrintSettings", "disableduplex"]);
|
|
}
|
|
set
|
|
{
|
|
_Xp["PrintSettings", "disableduplex"] = value.ToString();
|
|
OnPropertyChanged("Print_DisableDuplex");
|
|
}
|
|
}
|
|
// Change Bar Use from 16-bit code:
|
|
// No Default
|
|
// Without Change Bars
|
|
// With Default Change Bars
|
|
// With User Specified Change Bars
|
|
public enum PrintChangeBar : int
|
|
{
|
|
NoDefault=0, Without, WithDefault, WithUserSpecified
|
|
}
|
|
[Category("Print Settings")]
|
|
[DisplayName("Change Bar")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Change Bar Use")]
|
|
public PrintChangeBar Print_ChangeBar
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "ChangeBar"];
|
|
if (s == string.Empty) return PrintChangeBar.NoDefault;
|
|
return (PrintChangeBar)int.Parse(_Xp["PrintSettings", "ChangeBar"]);
|
|
}
|
|
set
|
|
{
|
|
if (value == PrintChangeBar.NoDefault) _Xp["PrintSettings", "ChangeBar"] = string.Empty;
|
|
else _Xp["PrintSettings", "ChangeBar"] = ((int)value).ToString();
|
|
OnPropertyChanged("Print_ChangeBar");
|
|
}
|
|
}
|
|
// User Specified Change Bar Location from16-bit code:
|
|
// With Text
|
|
// Outside Box
|
|
// AER on LEFT, RNO on Right
|
|
// To the Left of Text
|
|
public enum PrintChangeBarLoc : int
|
|
{
|
|
WithText = 0, OutsideBox, AERleftRNOright, LeftOfText
|
|
}
|
|
[Category("Print Settings")]
|
|
[DisplayName("Change Bar Location")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("User Specified Change Bar Location")]
|
|
public PrintChangeBarLoc Print_ChangeBarLoc
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "ChangeBarLoc"];
|
|
if (s == string.Empty) return PrintChangeBarLoc.WithText;
|
|
return (PrintChangeBarLoc)int.Parse(_Xp["PrintSettings", "ChangeBarLoc"]);
|
|
}
|
|
set
|
|
{
|
|
if (value == PrintChangeBarLoc.WithText) _Xp["PrintSettings", "ChangeBarLoc"] = string.Empty;
|
|
else _Xp["PrintSettings", "ChangeBarLoc"] = ((int)value).ToString();
|
|
OnPropertyChanged("Print_ChangeBarLoc");
|
|
}
|
|
}
|
|
|
|
// Change Bar Text from16-bit code:
|
|
// Date and Change ID
|
|
// Revision Number
|
|
// Change ID
|
|
// No Change Bar Message
|
|
// User Defined Message
|
|
public enum PrintChangeBarText : int
|
|
{
|
|
DateChgID = 0, RevNum, ChgID, None, UserDef
|
|
}
|
|
[Category("Print Settings")]
|
|
[DisplayName("Change Bar Text")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Change Bar Text")]
|
|
public PrintChangeBarText Print_ChangeBarText
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["PrintSettings", "ChangeBarText"];
|
|
if (s == string.Empty) return PrintChangeBarText.DateChgID;
|
|
return (PrintChangeBarText)int.Parse(_Xp["PrintSettings", "ChangeBarText"]);
|
|
}
|
|
set
|
|
{
|
|
if (value == PrintChangeBarText.DateChgID) _Xp["PrintSettings", "ChangeBarText"] = string.Empty;
|
|
else _Xp["PrintSettings", "ChangeBarText"] = ((int)value).ToString();
|
|
OnPropertyChanged("Print_ChangeBarText");
|
|
}
|
|
}
|
|
|
|
[Category("Print Settings")]
|
|
[DisplayName("User Format")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("User Format")]
|
|
public string Print_UserFormat
|
|
{
|
|
get
|
|
{
|
|
return _Xp["PrintSettings", "userformat"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["PrintSettings", "userformat"] = value;
|
|
OnPropertyChanged("Print_UserFormat");
|
|
}
|
|
}
|
|
|
|
[Category("Print Settings")]
|
|
[DisplayName("User Change Bar Message1")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("User Change Bar Message1")]
|
|
public string Print_UserCBMess1
|
|
{
|
|
get
|
|
{
|
|
return _Xp["PrintSettings", "usercbmess1"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["PrintSettings", "usercbmess1"] = value;
|
|
OnPropertyChanged("Print_UserCBMess1");
|
|
}
|
|
}
|
|
|
|
[Category("Print Settings")]
|
|
[DisplayName("User Change Bar Message2")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("User Change Bar Message2")]
|
|
public string Print_UserCBMess2
|
|
{
|
|
get
|
|
{
|
|
return _Xp["PrintSettings", "usercbmess2"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["PrintSettings", "usercbmess2"] = value;
|
|
OnPropertyChanged("Print_UserCBMess2");
|
|
}
|
|
}
|
|
#endregion
|
|
[Category("Defaults")]
|
|
[DisplayName("Default BackColor")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Default Background Color")]
|
|
public Color Default_BkColor
|
|
{
|
|
get
|
|
{
|
|
//return _Xp["default", "BkColor"];
|
|
string sColor = _Xp["default", "BkColor"];
|
|
if (sColor == string.Empty) sColor = "White";
|
|
if (sColor[0] == '[')
|
|
{
|
|
string[] parts = sColor.Substring(1, sColor.Length - 2).Split(",".ToCharArray());
|
|
return Color.FromArgb(Int32.Parse(parts[0]), Int32.Parse(parts[1]), Int32.Parse(parts[2]));
|
|
}
|
|
else return Color.FromName(sColor);
|
|
}
|
|
set
|
|
{
|
|
if (value.IsNamedColor) _Xp["default", "BkColor"] = value.Name;
|
|
else
|
|
{
|
|
_Xp["default", "BkColor"] = string.Format("[{0},{1},{2}]", value.R, value.G, value.B);
|
|
}
|
|
OnPropertyChanged("Default_BkColor");
|
|
|
|
}
|
|
}
|
|
}
|
|
}
|
|
*/
|
|
#endregion
|
|
public void AddSlave(string xml)
|
|
{
|
|
XmlDocument xd = new XmlDocument();
|
|
xd.LoadXml(xml);
|
|
XmlNode pn = _Xp.XmlContents.SelectSingleNode("//Slaves");
|
|
XmlNode nn = _Xp.XmlContents.ImportNode(xd.DocumentElement, true);
|
|
pn.AppendChild(nn);
|
|
}
|
|
public void RemoveSlave(int index)
|
|
{
|
|
XmlNode dd = _Xp.XmlContents.SelectSingleNode("//Slave[@index='" + index.ToString() + "']");
|
|
dd.ParentNode.RemoveChild(dd);
|
|
}
|
|
public int MaxSlaveIndex
|
|
{
|
|
get
|
|
{
|
|
int k = 0;
|
|
XmlNodeList nl = _Xp.XmlContents.SelectNodes("//Slave/@index");
|
|
foreach (XmlNode n in nl)
|
|
k = Math.Max(k, int.Parse(n.InnerText));
|
|
return k;
|
|
}
|
|
}
|
|
//added by jcb to fix master issue byron/braidwood
|
|
private string GetCombinedSlaveValue(string item, string defaultValue)
|
|
{
|
|
if (MaxSlaveIndex > 0)
|
|
{
|
|
string s = "";
|
|
string sep = "";
|
|
XmlNodeList nl = _Xp.XmlContents.SelectNodes("//Slave");
|
|
foreach (XmlNode nd in nl)
|
|
{
|
|
string ss = nd.Attributes.GetNamedItem("index").InnerText;
|
|
s += sep + _Xp["Slave[@index='" + ss + "']", item];
|
|
sep = ",";
|
|
}
|
|
return s;
|
|
}
|
|
return defaultValue;
|
|
}
|
|
private string GetCombinedSlaveValue(string item)
|
|
{
|
|
return GetCombinedSlaveValue(item, "0");
|
|
}
|
|
//end added by jcb to fix master issue byron/braidwood
|
|
}
|
|
} |