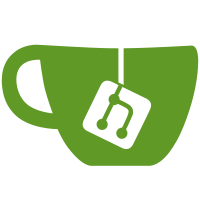
Added code to retrieve command-line parameters. Added property CalledFromCSLA to retrieve the CSLA object and method used by DBTracking. Added ProfileTimer object to track CPU using by methods
220 lines
5.7 KiB
C#
220 lines
5.7 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
|
|
namespace Volian.Base.Library
|
|
{
|
|
public class VlnTimer
|
|
{
|
|
private DateTime _LastTime = DateTime.Now;
|
|
public DateTime LastTime
|
|
{
|
|
get { return _LastTime; }
|
|
set { _LastTime = value; }
|
|
}
|
|
private string _LastProcess = "Initialize";
|
|
public string LastProcess
|
|
{
|
|
get { return _LastProcess; }
|
|
set { _LastProcess = value; }
|
|
}
|
|
public string ActiveProcess
|
|
{
|
|
get { return _LastProcess; }
|
|
set
|
|
{
|
|
DateTime tNow = DateTime.Now;
|
|
TimeSpan ts = tNow - LastTime;
|
|
LastTime = tNow;
|
|
if (!ElapsedTimes.ContainsKey(LastProcess))
|
|
ElapsedTimes.Add(LastProcess, ts);
|
|
else
|
|
ElapsedTimes[LastProcess] += ts;
|
|
LastProcess = value;
|
|
}
|
|
}
|
|
Dictionary<string, TimeSpan> _ElapsedTimes = new Dictionary<string, TimeSpan>();
|
|
public Dictionary<string, TimeSpan> ElapsedTimes
|
|
{
|
|
get { return _ElapsedTimes; }
|
|
set { _ElapsedTimes = value; }
|
|
}
|
|
public void ShowElapsedTimes()
|
|
{
|
|
ActiveProcess = "fini";
|
|
Console.WriteLine("'Process'\t'Elapsed'");
|
|
foreach (string proc in ElapsedTimes.Keys)
|
|
Console.WriteLine("'{0}'\t{1}", proc, ElapsedTimes[proc].TotalSeconds);
|
|
}
|
|
public void ShowElapsedTimes(string title)
|
|
{
|
|
Console.WriteLine(title);
|
|
ShowElapsedTimes();
|
|
}
|
|
}
|
|
public static class ProfileTimer
|
|
{
|
|
private static Stack<string> _MyStack = new Stack<string>();
|
|
|
|
public static Stack<string> MyStack
|
|
{
|
|
get { return _MyStack; }
|
|
set { _MyStack = value; }
|
|
}
|
|
private static DateTime _StartTime = DateTime.Now;
|
|
public static DateTime StartTime
|
|
{
|
|
get { return _StartTime; }
|
|
set { _StartTime = value; }
|
|
}
|
|
delegate int DoPushTrack(string start);
|
|
private static DoPushTrack myDoPush = new DoPushTrack(IgnorePush);
|
|
public static int Push(string start)
|
|
{
|
|
return DoPush(start);
|
|
}
|
|
private static int DoPush(string start)
|
|
{
|
|
MyStack.Push(Start);
|
|
Start = start;
|
|
return MyStack.Count;
|
|
}
|
|
private static int IgnorePush(string start)
|
|
{
|
|
Start = start;
|
|
return 1;
|
|
}
|
|
delegate int DoPopTrack(int depth);
|
|
private static DoPopTrack myDoPop = new DoPopTrack(IgnorePop);
|
|
public static int Pop(int depth)
|
|
{
|
|
return DoPop(depth);
|
|
}
|
|
private static int DoPop(int depth)
|
|
{
|
|
if (MyStack.Count != depth)
|
|
Console.WriteLine("Profile Stack Issues {0}", Start);
|
|
Start = MyStack.Pop();
|
|
return MyStack.Count;
|
|
}
|
|
private static int IgnorePop(int depth)
|
|
{
|
|
return 0;
|
|
}
|
|
public static int Depth
|
|
{
|
|
get
|
|
{
|
|
return MyStack.Count;
|
|
}
|
|
}
|
|
private static Dictionary<string, long> _TimerTable = new Dictionary<string, long>();
|
|
public static Dictionary<string, long> TimerTable
|
|
{
|
|
get { return ProfileTimer._TimerTable; }
|
|
set { ProfileTimer._TimerTable = value; }
|
|
}
|
|
delegate void DoTrack(string module);
|
|
private static DoTrack myDoTrack = new DoTrack(IgnoreModule);
|
|
public static void TurnOnTracking(string filename)
|
|
{
|
|
DebugProfile.Open(VlnSettings.TemporaryFolder + "\\" + filename);
|
|
myDoTrack = new DoTrack(TrackModule);
|
|
myDoPush = new DoPushTrack(DoPush);
|
|
myDoPop = new DoPopTrack(DoPop);
|
|
}
|
|
public static void TurnOffTracking()
|
|
{
|
|
myDoTrack = new DoTrack(IgnoreModule);
|
|
myDoPush = new DoPushTrack(IgnorePush);
|
|
myDoPop = new DoPopTrack(IgnorePop);
|
|
}
|
|
private static string _Start = "Start";
|
|
public static string Start
|
|
{
|
|
get { return _Start; }
|
|
set
|
|
{
|
|
myDoTrack(value);
|
|
}
|
|
}
|
|
private static void TrackModule(string module)
|
|
{
|
|
DateTime dtNext = DateTime.Now;
|
|
//Console.WriteLine("{0},'{1}'", TimeSpan.FromTicks(dtNext.Ticks - LastTime.Ticks).TotalSeconds, Description);
|
|
AddTimerInfo(_Start, dtNext.Ticks - LastTime.Ticks);
|
|
_Start = module;
|
|
_LastTime = dtNext;
|
|
}
|
|
private static void IgnoreModule(string module)
|
|
{
|
|
_Start = module;
|
|
}
|
|
private static void AddTimerInfo(string description, long ticks)
|
|
{
|
|
if (TimerTable.ContainsKey(description))
|
|
TimerTable[description] += ticks;
|
|
else
|
|
TimerTable.Add(description, ticks);
|
|
}
|
|
public static void ShowTimerTable()
|
|
{
|
|
if (DebugProfile.IsOpen)
|
|
{
|
|
if (MyStack.Count > 0)
|
|
{
|
|
DebugProfile.WriteLine("{0} Items on Stack", MyStack.Count);
|
|
Dictionary<string, int> stackCount = new Dictionary<string, int>();
|
|
foreach (string loc in MyStack)
|
|
{
|
|
if (!stackCount.ContainsKey(loc))
|
|
stackCount.Add(loc, 1);
|
|
else
|
|
stackCount[loc]++;
|
|
}
|
|
foreach (string stkey in stackCount.Keys)
|
|
DebugProfile.WriteLine("\"{0}\"\t{1}", stkey, stackCount[stkey]);
|
|
DebugProfile.WriteLine("-----------------------------------");
|
|
}
|
|
long total = 0;
|
|
foreach (long ticks in TimerTable.Values)
|
|
total += ticks;
|
|
SortedDictionary<long, List<string>> sList = new SortedDictionary<long, List<string>>();
|
|
foreach (string str in TimerTable.Keys)
|
|
{
|
|
long key = -TimerTable[str];
|
|
if (!sList.ContainsKey(key))
|
|
sList.Add(key, new List<string>());
|
|
sList[key].Add(str);
|
|
}
|
|
long limit = (95 * total) / 100;
|
|
foreach (long lkey in sList.Keys)
|
|
{
|
|
foreach (string str1 in sList[lkey])
|
|
{
|
|
TimeSpan secs = TimeSpan.FromTicks(-lkey);
|
|
if (limit > 0)
|
|
DebugProfile.WriteLine("\"{0}\"\t{1}\t{2}", str1, secs.TotalSeconds, (100 * secs.Ticks) / total);
|
|
limit += lkey;
|
|
}
|
|
}
|
|
DebugProfile.WriteLine("\"Total\"\t{0}", TimeSpan.FromTicks(total).TotalSeconds);
|
|
DebugProfile.Show();
|
|
}
|
|
}
|
|
public static void Reset()
|
|
{
|
|
_TimerTable = new Dictionary<string, long>();
|
|
_Start = "Start";
|
|
_LastTime = DateTime.Now;
|
|
_MyStack = new Stack<string>();
|
|
}
|
|
private static DateTime _LastTime = DateTime.Now;
|
|
public static DateTime LastTime
|
|
{
|
|
get { return _LastTime; }
|
|
set { _LastTime = value; }
|
|
}
|
|
}
|
|
}
|