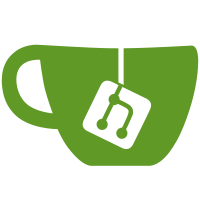
Eliminate unused variables Use settings for Format Load Output status of Formats being loaded Save File Last Write UTC as Document.DTS added History_OriginalFileName to DocumentConfig Use settings rather than local variables Only use the first 12 characters of a ROID for DROUsage Lookup
243 lines
8.4 KiB
C#
243 lines
8.4 KiB
C#
// ========================================================================
|
|
// Copyright 2006 - Volian Enterprises, Inc. All rights reserved.
|
|
// Volian Enterprises - Proprietary Information - DO NOT COPY OR DISTRIBUTE
|
|
// ------------------------------------------------------------------------
|
|
// $Workfile: $ $Revision: $
|
|
// $Author: $ $Date: $
|
|
//
|
|
// $History: $
|
|
// ========================================================================
|
|
using System;
|
|
using System.Drawing;
|
|
using System.Collections;
|
|
using System.ComponentModel;
|
|
using System.Windows.Forms;
|
|
using System.Data;
|
|
using System.Data.OleDb;
|
|
using System.Collections.Specialized;
|
|
using System.Collections.Generic;
|
|
using System.Xml;
|
|
using System.Xml.XPath;
|
|
using System.IO;
|
|
using System.Text;
|
|
using VEPROMS.CSLA.Library;
|
|
|
|
namespace DataLoader
|
|
{
|
|
public partial class Loader
|
|
{
|
|
//public string _FmtAllPath;
|
|
//public string _GenmacAllPath;
|
|
//private FormatInfoList _AllFormats;
|
|
|
|
//public FormatInfoList AllFormats
|
|
//{
|
|
// get
|
|
// {
|
|
// if (_AllFormats == null)
|
|
// _AllFormats = FormatInfoList.Get();
|
|
// return _AllFormats;
|
|
// }
|
|
//}
|
|
//private Dictionary<string, int> _LookupFormats;
|
|
//public Dictionary<string, int> LookupFormats
|
|
//{
|
|
// get
|
|
// {
|
|
// if (_LookupFormats == null)
|
|
// {
|
|
// _LookupFormats = new Dictionary<string, int>();
|
|
// foreach (FormatInfo myFormat in AllFormats)
|
|
// {
|
|
// _LookupFormats.Add(myFormat.Name, myFormat.FormatID);
|
|
// }
|
|
// }
|
|
// return _LookupFormats;
|
|
// }
|
|
//}
|
|
//private Format AddFormatToDB(Format parent, string format, bool issub, DateTime Dts, string Userid)
|
|
//{
|
|
// string fmtdata = null;
|
|
// string genmacdata = null;
|
|
// XmlDocument xd = null;
|
|
|
|
// //string path = "c:\\development\\fmtall\\" + format + "all.xml";
|
|
// string path = _FmtAllPath + "\\" + format + "all.xml";
|
|
// if (File.Exists(path))
|
|
// {
|
|
// try
|
|
// {
|
|
// StreamReader srf = new StreamReader(path);
|
|
// xd = new XmlDocument();
|
|
// xd.Load(srf);
|
|
// //xd.Load(path);
|
|
// fmtdata = xd.OuterXml;
|
|
// }
|
|
// catch (Exception ex)
|
|
// {
|
|
// frmMain.AddError(ex, "AddFormatToDB('{0}','{1}')", parent.Name, path);
|
|
// return null;
|
|
// }
|
|
// }
|
|
|
|
// // Do we need genmac - only if non-subformat
|
|
// if (!issub)
|
|
// {
|
|
// //path = "c:\\development\\genmacall\\" + format + ".svg";
|
|
// path = _GenmacAllPath + "\\" + format + ".svg";
|
|
// if (File.Exists(path))
|
|
// {
|
|
// try
|
|
// {
|
|
// StreamReader sr = new StreamReader(path);
|
|
// XmlDocument xdg = new XmlDocument();
|
|
// xdg.Load(sr);
|
|
// //xdg.Load(path);
|
|
// genmacdata = xdg.OuterXml;
|
|
// }
|
|
// catch (Exception ex)
|
|
// {
|
|
// frmMain.AddError(ex, "AddFormatToDB('{0}','{1}')", parent.Name, path);
|
|
// return null;
|
|
// }
|
|
// }
|
|
// }
|
|
// // Get the name & then create the record.
|
|
// if (Userid == null || Userid == "") Userid = "Migration";
|
|
|
|
// string nmattr = "Default";
|
|
|
|
// // use xpath to get name.
|
|
// if (parent != null)
|
|
// {
|
|
// XmlNode nmnode = xd.SelectSingleNode("//FormatData");
|
|
// if (nmnode is XmlElement)
|
|
// {
|
|
// XmlElement xm = (XmlElement)nmnode;
|
|
// nmattr = xm.GetAttribute("Name");
|
|
// }
|
|
// }
|
|
// // use the format name if base or non sub. If it's a sub, remove the "_".
|
|
// string fname = issub ? format.Replace("_", "") : format;
|
|
// Format rec = null;
|
|
// try
|
|
// {
|
|
// if (!LookupFormats.ContainsKey(fname))
|
|
// {
|
|
// rec = Format.MakeFormat(parent, fname, nmattr, fmtdata, genmacdata, Dts, Userid);
|
|
// }
|
|
// else
|
|
// {
|
|
// rec = Format.Get(LookupFormats[fname]);
|
|
// rec.Data = fmtdata;
|
|
// rec.GenMac = genmacdata;
|
|
// rec = rec.Save();
|
|
// }
|
|
// }
|
|
// catch (Exception ex)
|
|
// {
|
|
// frmMain.AddError(ex, "AddFormatToDB-make format('{0}','{1}')", parent.Name, path);
|
|
// }
|
|
// return rec;
|
|
//}
|
|
//public void LoadAllFormats()
|
|
//{
|
|
// Format basefmt = null;
|
|
// Format parent = null;
|
|
|
|
|
|
// // Load base format.
|
|
// basefmt = AddFormatToDB(null, "base", false, DateTime.Now, "Migration");
|
|
// if (basefmt == null) return;
|
|
// // now loop through all files... If there is an _ in the name, it's a subformat
|
|
// // (for example, AEP_00.xml) skip it in main loop, it's handled for each format.
|
|
// DirectoryInfo di = new DirectoryInfo(_FmtAllPath); //(@"c:\development\fmtall");
|
|
// FileInfo[] fis = di.GetFiles("*.xml");
|
|
|
|
// foreach (FileInfo fi in fis)
|
|
// {
|
|
// //if (fi.Name.ToUpper() == "WCN2ALL.XML"|| fi.Name.ToUpper() == "OHLPALL.XML")
|
|
// //{
|
|
// bool issub = (fi.Name.IndexOf("_") > 0) ? true : false;
|
|
// if (!issub && fi.Name.ToLower() != "baseall.xml")
|
|
// {
|
|
// string fmtname = fi.Name.Substring(0, fi.Name.Length - 7);
|
|
// // remove the all.xml part of the filename.
|
|
// try
|
|
// {
|
|
// parent = AddFormatToDB(basefmt, fmtname, issub, DateTime.Now, "Migration");
|
|
// if (parent != null)
|
|
// {
|
|
// // now see if there are any subformats associated with this, if so
|
|
// // add them here...
|
|
// DirectoryInfo sdi = new DirectoryInfo(_FmtAllPath); //("c:\\development\\fmtall");
|
|
// FileInfo[] sfis = di.GetFiles(fmtname + "_*.xml");
|
|
// foreach (FileInfo sfi in sfis)
|
|
// {
|
|
// string nm = sfi.Name.Substring(0, sfi.Name.Length - 7);
|
|
// //Console.WriteLine("Processing {0}", sfi.Name);
|
|
// frmMain.Status = string.Format("Processing Format {0}", sfi.Name);
|
|
// try
|
|
// {
|
|
// AddFormatToDB(parent, nm, true, DateTime.Now, "Migration");
|
|
// }
|
|
// catch (Exception ex)
|
|
// {
|
|
// frmMain.AddError(ex, "LoadAllFormats() '{0}'", sfi.Name);
|
|
// //Console.WriteLine("{0} - {1}", ex.GetType().Name, ex.Message);
|
|
// }
|
|
// }
|
|
// }
|
|
// }
|
|
// catch (Exception ex)
|
|
// {
|
|
// frmMain.AddError(ex, "LoadAllFormats() '{0}'", fi.Name);
|
|
// //Console.WriteLine("{0} - {1}", ex.GetType().Name, ex.Message);
|
|
// }
|
|
// }
|
|
// //}
|
|
// }
|
|
//}
|
|
public FormatInfo GetFormat(string format)
|
|
{
|
|
Format parent = null;
|
|
Format rec = null;
|
|
|
|
// get base
|
|
Format baseparent = Format.Get(1);
|
|
|
|
// if there is no format - what should be done? maybe nothing.
|
|
if (format == null || format == "") return null;
|
|
|
|
try
|
|
{
|
|
if (format.IndexOf(' ') > -1) // will have spaces if it's a user format
|
|
{
|
|
string part1 = format.Substring(0, format.IndexOf(' '));
|
|
string part2 = format.Substring(format.LastIndexOf(' ') + 1, 2);
|
|
format = part1 + part2;
|
|
|
|
// get the parent format's id (for example, AEP for AEP00).
|
|
parent = Format.GetByParentID_Name(baseparent.FormatID, part1);
|
|
}
|
|
else
|
|
parent = baseparent;
|
|
|
|
// see if the format has been added, if not go get it.
|
|
rec = Format.GetByParentID_Name(parent.FormatID, format);
|
|
// JSJ - 02/04/10 this fixes cases where a subformat is no longer available,
|
|
// default to the parent format
|
|
if (rec == null)
|
|
rec = parent;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
log.ErrorFormat("Error getting xml version of format {0}", ex.Message);
|
|
frmMain.AddError(ex, "GetFormat('{0}')", format);
|
|
}
|
|
FormatInfo recInfo = FormatInfo.Get(rec.FormatID);
|
|
return recInfo;
|
|
}
|
|
|
|
}
|
|
} |