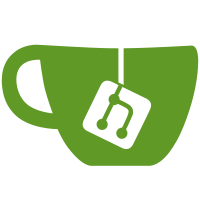
Added code to display message box when no procedures are found to be processed and terminates the processing. Utilized ChildrenProcessed property when processing procedures Added format changes to correct remaining Braidwood issues Added code to resolve unit information in section transition Modified page break logic to resolve issue with Braidwood deviation documents Added code to resolve page number when section is a word document section Added check to verify location was not already in list prior to adding it to list Reduced number of instances of Volian flexgrid during printing
353 lines
13 KiB
C#
353 lines
13 KiB
C#
// ========================================================================
|
|
// Copyright 2006 - Volian Enterprises, Inc. All rights reserved.
|
|
// Volian Enterprises - Proprietary Information - DO NOT COPY OR DISTRIBUTE
|
|
// ------------------------------------------------------------------------
|
|
// $Workfile: $ $Revision: $
|
|
// $Author: $ $Date: $
|
|
//
|
|
// $History: $
|
|
// ========================================================================
|
|
using System;
|
|
using System.Drawing;
|
|
using System.Collections;
|
|
using System.ComponentModel;
|
|
using System.Windows.Forms;
|
|
using System.Data;
|
|
using System.Data.OleDb;
|
|
using System.Collections.Specialized;
|
|
using System.Collections.Generic;
|
|
using System.Xml;
|
|
using System.IO;
|
|
using System.Text;
|
|
using VEPROMS.CSLA.Library;
|
|
using vlnObjectLibrary;
|
|
using vlnServerLibrary;
|
|
using Org.Mentalis.Files;
|
|
using Config;
|
|
using Utils;
|
|
|
|
namespace DataLoader
|
|
{
|
|
public partial class Loader
|
|
{
|
|
private List<Folder> vlnDataPathFolders() // was vlnDataPath
|
|
{
|
|
List<Folder> dpf = new List<Folder>();
|
|
// Get path to config file
|
|
string sCfg = Environment.GetEnvironmentVariable("veconfig");
|
|
IniReader cfg = new IniReader(sCfg);
|
|
// Get DataPath
|
|
string sDP = cfg.ReadString("menu", "DataPath");
|
|
// Split DataPath into directories
|
|
|
|
// Get the current list of folders so that if it already exists, just get the 'Folder', otherwise
|
|
// make it. It was done this way because there was no way to get the Folder given a name/Title.
|
|
FolderInfoList fil = FolderInfoList.Get();
|
|
|
|
foreach (string s1 in sDP.Split(";".ToCharArray()))
|
|
{
|
|
if (s1.Length > 0)
|
|
{
|
|
string[] s2 = s1.Split(",".ToCharArray());
|
|
Folder fld = GetFolderFromName(s2[1], s2[0], fil);
|
|
if (fld == null)
|
|
fld = Folder.MakeFolder(sysFolder, dbConn, s2[1], s2[0], FolderName(s2[0]), null, null, DateTime.Now, "Migration");
|
|
dpf.Add(fld);
|
|
}
|
|
}
|
|
return dpf;
|
|
}
|
|
private Folder GetFolderFromName(string name, string title, FolderInfoList fil)
|
|
{
|
|
Folder fld = null;
|
|
foreach (FolderInfo fi in fil)
|
|
{
|
|
string fi_Title = fi.Title.TrimEnd('\\');
|
|
//if (fi.Name.ToUpper() == name.ToUpper() && fi.Title.ToUpper() == title.ToUpper())
|
|
if (fi.Name.ToUpper() == name.ToUpper() && fi_Title.ToUpper() == title.ToUpper())
|
|
{
|
|
fld = Folder.Get(fi.FolderID);
|
|
return fld;
|
|
}
|
|
}
|
|
return null;
|
|
}
|
|
private string FolderName(string Path)
|
|
{
|
|
int ind = Path.LastIndexOf("\\");
|
|
if (ind == Path.Length-1 || ind < 0) return Path;
|
|
return Path.Substring(ind + 1);
|
|
}
|
|
private DocVersion GetDocVersionFromName(Folder fld, string f)
|
|
{
|
|
DocVersion dv = null;
|
|
DocVersionInfoList dvil = DocVersionInfoList.GetByFolderID(fld.FolderID);
|
|
foreach (DocVersionInfo dvi in dvil)
|
|
{
|
|
if (dvi.Name.ToUpper() == f.ToUpper())
|
|
{
|
|
dv = DocVersion.Get(dvi.VersionID);
|
|
return dv;
|
|
}
|
|
}
|
|
return null;
|
|
}
|
|
private object cslaObject(vlnObject vb, Connection dbConn, Object parfld, TreeNode tn)
|
|
{
|
|
switch (vb.Type)
|
|
{
|
|
case "plant":
|
|
case "set":
|
|
// if folder exists... get the Folder object, otherwise create it.
|
|
FolderInfoList fil = FolderInfoList.Get();
|
|
Folder fld = GetFolderFromName(vb.Title, vb.Path, fil);
|
|
if (fld==null) fld = Folder.MakeFolder((Folder)parfld, dbConn, vb.Title, vb.Path, FolderName(vb.Path), null, null, DateTime.Now, "Migration");
|
|
tn.Tag = fld;
|
|
return (object) fld;
|
|
case "version":
|
|
// see if version exists for the input folder, parfld.
|
|
DocVersion v = GetDocVersionFromName((Folder)parfld, vb.Title);
|
|
if (v == null)
|
|
{
|
|
ConfigFile cfg = new ConfigFile();
|
|
|
|
XmlDocument d = cfg.IniToXml(vb.Path + "\\proc.ini");
|
|
AddSlaveUnits(d, vb.Path);
|
|
if (frmMain.ProcessFailed)
|
|
return null;
|
|
//DocVersionConfig fld_cfg = new DocVersionConfig(d == null ? "" : d.InnerXml);
|
|
FolderConfig fld_cfg = (d==null)?null:new FolderConfig(d.InnerXml);
|
|
// translate curset.dat into xml & add to d (somehow).
|
|
string csfile = string.Format("{0}\\curset.dat",vb.Path);
|
|
string defPlantFmt = null;
|
|
if (File.Exists(csfile))
|
|
{
|
|
CurSet cs = new CurSet(csfile);
|
|
try
|
|
{
|
|
if (fld_cfg == null) fld_cfg = new FolderConfig();
|
|
fld_cfg = cs.Convert(fld_cfg);
|
|
defPlantFmt = cs.GetDefFmt();
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
log.ErrorFormat("error in convert curset.dat, ex = {0}", ex.Message);
|
|
frmMain.AddError(ex, "error in convert curset.dat");
|
|
}
|
|
}
|
|
// *** RHM 20120613 - Fix
|
|
// *** This was naming the DocVersion to the folder name
|
|
//string titlepath = vb.Path + "\\" + "Title";
|
|
//FileInfo fi = new FileInfo(titlepath);
|
|
//string thetitle = vb.Title;
|
|
//if (File.Exists(titlepath))
|
|
//{
|
|
// StreamReader myReader = new StreamReader(titlepath);
|
|
// thetitle = myReader.ReadLine();
|
|
// myReader.Close();
|
|
//}
|
|
Folder tmpfld = (Folder)parfld;
|
|
FormatInfo format = null;
|
|
if (defPlantFmt != null && defPlantFmt != "")
|
|
{
|
|
format = GetFormat(defPlantFmt);
|
|
}
|
|
else
|
|
format = FormatInfo.Get(1);
|
|
|
|
using(Format fmt = format.Get())
|
|
v = DocVersion.MakeDocVersion(tmpfld, (int)DocVersionType(vb.Path.ToLower()), vb.Title, vb.Path.ToLower(), null, fmt, fld_cfg == null ? null : fld_cfg.ToString(), DateTime.Now, "Migration");
|
|
}
|
|
tn.Tag = v;
|
|
MigrateDocVersion(v, false);
|
|
return (object)v;
|
|
}
|
|
return 0;
|
|
}
|
|
|
|
private void AddSlaveUnits(XmlDocument d, string path)
|
|
{
|
|
string masterDir = GetMasterDir(d);
|
|
if (masterDir == null)
|
|
{
|
|
//not a master slave procedure set
|
|
return;
|
|
}
|
|
DirectoryInfo master = new DirectoryInfo(path);
|
|
if (master.Name.ToUpper() != masterDir.ToUpper()) return;
|
|
List<string> names = GetUnitNames(d);
|
|
if (names == null)
|
|
{
|
|
frmMain.ProcessFailed = true;
|
|
MessageBox.Show("Could not find Unit names in slave proc.ini files");
|
|
frmMain.MyError = "Could not find Unit names in slave proc.ini files";
|
|
return;
|
|
}
|
|
DirectoryInfo[] slaves = master.Parent.GetDirectories(masterDir.ToUpper().Replace(".PRC",".SL*"));
|
|
XmlDocument units = new XmlDocument();
|
|
units.LoadXml("<Slaves/>");
|
|
int idx = 0;
|
|
foreach (DirectoryInfo slave in slaves)
|
|
{
|
|
FileInfo[] inis = slave.GetFiles("proc.ini");
|
|
if (inis.Length > 0)
|
|
{
|
|
FileInfo ini = inis[0];
|
|
ConfigFile cfg = new ConfigFile();
|
|
XmlDocument xSlave = cfg.IniToXml(ini.FullName);
|
|
xSlave.LoadXml(xSlave.OuterXml.Replace("<Unit", "<Slave"));
|
|
string slaveDir = GetMasterDir(xSlave);
|
|
if (slaveDir == null)
|
|
{
|
|
frmMain.ProcessFailed = true;
|
|
MessageBox.Show("Could not find MasterDir in slave proc.ini files");
|
|
frmMain.MyError = "Could not find MasterDir in slave proc.ini files";
|
|
return;
|
|
}
|
|
if (slaveDir.ToUpper() == masterDir.ToUpper())
|
|
{
|
|
XmlNode unit = xSlave.SelectSingleNode("Config/Slave");
|
|
XmlAttribute oldindex = xSlave.CreateAttribute("oldindex");
|
|
oldindex.InnerText = slave.Extension.ToUpper().Replace(".SL", "");
|
|
unit.Attributes.SetNamedItem(oldindex);
|
|
XmlAttribute index = xSlave.CreateAttribute("index");
|
|
idx++;
|
|
index.InnerText = idx.ToString();
|
|
unit.Attributes.SetNamedItem(index);
|
|
if (names.Count == slaves.Length)
|
|
oldindex.InnerText = index.InnerText;
|
|
if (xSlave.SelectSingleNode("Config/ProcedureSet") != null)
|
|
{
|
|
XmlAttribute setName = xSlave.CreateAttribute("SetName");
|
|
if(xSlave.SelectSingleNode("Config/ProcedureSet/@Name") != null)
|
|
setName.InnerText = xSlave.SelectSingleNode("Config/ProcedureSet/@Name").InnerText;
|
|
unit.Attributes.SetNamedItem(setName);
|
|
XmlAttribute setID = xSlave.CreateAttribute("SetID");
|
|
if(xSlave.SelectSingleNode("Config/ProcedureSet/@ID") != null)
|
|
setID.InnerText = xSlave.SelectSingleNode("Config/ProcedureSet/@ID").InnerText;
|
|
unit.Attributes.SetNamedItem(setID);
|
|
}
|
|
units.DocumentElement.AppendChild(units.ImportNode(unit, true));
|
|
}
|
|
}
|
|
}
|
|
d.DocumentElement.AppendChild(d.ImportNode(units.DocumentElement, true));
|
|
//d.DocumentElement.RemoveChild(d.SelectSingleNode("Config/Unit"));
|
|
Console.WriteLine(d.OuterXml);
|
|
}
|
|
private static XmlNode GetXMLNode(XmlDocument d, string nodeName)
|
|
{
|
|
return GetXMLNode(d.DocumentElement, nodeName);
|
|
}
|
|
private static XmlNode GetXMLNode(XmlNode nd, string nodeName)
|
|
{
|
|
XmlNodeList xl = nd.SelectNodes(string.Format("//{0}", nodeName));
|
|
if (xl.Count == 0 && System.Text.RegularExpressions.Regex.IsMatch(nodeName, "^[A-Za-z0-9]+$"))
|
|
xl = nd.SelectNodes(
|
|
string.Format("//*[translate(local-name(), 'ABCDEFGHIJKLMNOPQRSTUVWXYZ','abcdefghijklmnopqrstuvwxyz')='{0}']", nodeName.ToLower()));
|
|
switch (xl.Count)
|
|
{
|
|
case 0: // No nodes found
|
|
return null;
|
|
case 1: // Found the node
|
|
XmlNode xn = xl[0];
|
|
if (xn.GetType() == typeof(XmlElement)) return xn;
|
|
throw new Exception(string.Format("Retrieved {0} while looking for XmlElement //{1}", xn.GetType().ToString(), nodeName));
|
|
default: // Found more than 1 node
|
|
throw new Exception(string.Format("Found more than one node //{0}", nodeName));
|
|
}
|
|
}
|
|
private static XmlAttribute GetXMLAttribute(XmlNode xx, string item)
|
|
{
|
|
//XmlNodeList xl = xx.SelectNodes(string.Format("@{0}", item));
|
|
XmlNodeList xl = xx.SelectNodes(
|
|
string.Format("@*[translate(local-name(), 'ABCDEFGHIJKLMNOPQRSTUVWXYZ','abcdefghijklmnopqrstuvwxyz')='{0}']", item.ToLower()));
|
|
switch (xl.Count)
|
|
{
|
|
case 0: // No nodes found
|
|
return null;
|
|
case 1: // Found the node
|
|
XmlNode xn = xl[0];
|
|
if (xn.GetType() == typeof(XmlAttribute)) return (XmlAttribute)xn;
|
|
throw new Exception(string.Format("Retrieved {0} while looking for XmlAttribute @{1}", xn.GetType().ToString(), item));
|
|
default: // Found more than 1 node
|
|
throw new Exception(string.Format("Found more than one node @{0}", item));
|
|
}
|
|
}
|
|
private List<string> GetUnitNames(XmlDocument d)
|
|
{
|
|
XmlNode zapp = GetXMLNode(d, "Unit");// d.SelectSingleNode("//Unit");
|
|
if (zapp == null) return null;
|
|
XmlNode zmstr = GetXMLAttribute(zapp, "Name");// zapp.Attributes.GetNamedItem("Name");
|
|
if (zmstr == null) return null;
|
|
string[] names = zmstr.InnerText.Split(",".ToCharArray());
|
|
return new List<string>(names);
|
|
}
|
|
|
|
private static string GetMasterDir(XmlDocument d)
|
|
{
|
|
XmlNode zapp = GetXMLNode(d, "Applicability");// d.SelectSingleNode("//Applicability");
|
|
if (zapp == null) return null;
|
|
XmlNode zmstr = GetXMLAttribute(zapp, "MasterDir");// zapp.Attributes.GetNamedItem("MasterDir");
|
|
if (zmstr == null) return null;
|
|
return zmstr.InnerText;
|
|
}
|
|
private string _OnlyThisFolder;
|
|
public string OnlyThisFolder
|
|
{
|
|
get
|
|
{
|
|
if (_OnlyThisFolder == null)
|
|
{
|
|
if (frmMain.MySettings.OnlyThisSet)
|
|
{
|
|
DirectoryInfo dir = new DirectoryInfo(frmMain.MySettings.ProcedureSetPath);
|
|
_OnlyThisFolder = dir.Parent.FullName.ToUpper();
|
|
}
|
|
else if ((frmMain.MySettings.ProcessOnlyInLocation ?? "") != "")
|
|
_OnlyThisFolder = frmMain.MySettings.ProcessOnlyInLocation.ToUpper();
|
|
else
|
|
_OnlyThisFolder = "";
|
|
}
|
|
return _OnlyThisFolder;
|
|
}
|
|
}
|
|
private bool IsInSelectedPlantData(Object parent)
|
|
{
|
|
if (OnlyThisFolder == "")
|
|
return true;
|
|
Folder fld = parent as Folder;
|
|
if (fld == null)
|
|
return true;
|
|
if (fld.MyParent.MyParent != null)
|
|
return true;
|
|
if (fld.Title.ToUpper() == OnlyThisFolder)
|
|
return true;
|
|
return false;
|
|
}
|
|
private void MigrateChildren(vlnObject vb, vlnServer vs, Connection dbConn, Object parent, TreeNode tn)
|
|
{
|
|
if (vb.Type != "version")
|
|
{
|
|
vb.LoadChildren(vs.GetChildren(vb.ToString()));
|
|
List<vlnObject> lv = vb.Children;
|
|
if (vb.Children.Count == 0)
|
|
frmMain.AddError("No children found for {0}", vb.Path);
|
|
foreach (vlnObject vbc in lv)
|
|
{
|
|
if (vbc.Path.ToUpper().StartsWith(OnlyThisFolder) || OnlyThisFolder.StartsWith(vbc.Path.ToUpper()))
|
|
{
|
|
TreeNode tnc = tn.Nodes.Add(vbc.Title);
|
|
object idc = cslaObject(vbc, dbConn, parent, tnc);
|
|
if (frmMain.ProcessFailed)
|
|
return;
|
|
frmMain.Status = "Loading " + vbc.Title;
|
|
MigrateChildren(vbc, vs, dbConn, idc, tnc);
|
|
frmMain.ChildrenProcessed++;
|
|
}
|
|
}
|
|
}
|
|
frmMain.Status = " ";
|
|
}
|
|
}
|
|
} |