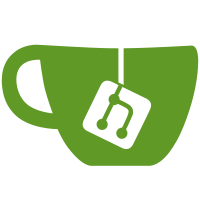
Skip commented lines in INI file. Remove inline comments before processing INI file. Use more explicit comparisons to determine type of command. Replace spaces with {sp}.
1165 lines
42 KiB
C#
1165 lines
42 KiB
C#
using System;
|
|
using System.Windows.Forms;
|
|
using System.IO;
|
|
using System.Text;
|
|
using System.Text.RegularExpressions;
|
|
using System.Collections;
|
|
using System.Runtime.Serialization.Formatters.Binary; // read in struct
|
|
using System.Xml.Xsl;
|
|
using System.Xml;
|
|
using System.Xml.Serialization;
|
|
using System.ComponentModel;
|
|
|
|
#region Structs
|
|
// top of the page style list.
|
|
[Serializable]
|
|
public struct PageStyles
|
|
{
|
|
public string Name;
|
|
public PageStyle [] PgStyles;
|
|
}
|
|
[Serializable]
|
|
public struct PageStyle
|
|
{
|
|
public string Name;
|
|
public short Index;
|
|
public PSItem [] Items;
|
|
}
|
|
[Serializable]
|
|
public struct PSItem
|
|
{
|
|
public float Row;
|
|
public float Col;
|
|
//public E_PageStructMod Justify;
|
|
public string Justify;
|
|
public VE_Font Style;
|
|
public string Token;
|
|
}
|
|
//// top of the doc style list.
|
|
[Serializable]
|
|
public struct DocStyles
|
|
{
|
|
public string Name;
|
|
public DocStyle [] DcStyles;
|
|
}
|
|
[Serializable]
|
|
public struct DocStyle
|
|
{
|
|
public string Name;
|
|
public short Index;
|
|
public short pagestyle; // Index into page style array
|
|
public VE_Font dstyle; // 'Printer driver styles' for the entire document
|
|
public float TopMargin; // First 'document text' row on printed page/ renamed to TopMargin
|
|
public float FooterLen; // Number of lines required for footer
|
|
public float LeftMargin; // Size of left margin
|
|
public float PageLength;
|
|
public float PageWidth;
|
|
public short numberingsequence; // 0 - no page number
|
|
// 1 - included with steps
|
|
// 2 - within sections ie. document styles
|
|
// 3 - within each accessory document
|
|
public bool IsStepSection; // using oldtonew, determine whether is step
|
|
// section or word doc section (new to 32-bit)
|
|
public bool UseCheckOffs; // This was originally in fmt. Load fmt to get
|
|
public int oldtonew; // Bits for converting from old to new
|
|
// document style
|
|
public bool CancelSectTitle; // Was format flags CancelSecTitlesOnS1 & CancelSecTitlesOnS2
|
|
public bool SpecialStepsFoldout; // Was format flag SpecialStepsFoldout - but put on E2 (was in 16bit code)
|
|
public bool UndSpecialStepsFoldout; // Was format flag UndSpecialStepsFoldout - but put here on E2 only.
|
|
public short ContTopHLS; // Flag for including High Level step
|
|
// as part of top continue message.
|
|
public float CTMargin; // Margin for top message
|
|
public float CBMargin; // Margin for bottom message
|
|
public short CBLoc; // 0 - place continue string at end of text
|
|
// 1 - between end of text & bottom of page
|
|
// 2 - place at bottom of page
|
|
//
|
|
public VE_Font ContStyle; // Style of continue messages
|
|
public short EndFlag; // Does end statement exist for this type
|
|
public VE_Font EndStyle; // Style of end message at end of page
|
|
public string ContTop; // Message at top of page
|
|
public string ContBottom; // Message at bottom of page
|
|
public string EndString; // String for end of document
|
|
public string FinalMsg; // Message for the final page of th text
|
|
public VE_DocStyle DocStructStyle; // Describe How / When structure is used
|
|
}
|
|
#endregion
|
|
#region ENums
|
|
[Flags]
|
|
public enum E_PageStructMod : uint
|
|
{
|
|
PSCenter = 0, // Page style, center field
|
|
PSLeft = 1, // Page style, left justify
|
|
PSRight = 2, // Page style, right justify
|
|
// *** PS modifiers: ***
|
|
PSBottom = 4, // Page style, always use bottom half line
|
|
PSTop = 8, // Page style, always use top half line
|
|
PSTrue = 16, // page style, don't adjust per CPI* (not needed after 4.0)
|
|
PSNotFirst = 32, // page style, don't put this token on the first page of section
|
|
PSOnlyFirst = 64, // page style, only put this token on the first page of section
|
|
PSRelRow = 128, // place in RelPageList
|
|
PSNoHalfLine= 256, // DontDoHalflines for his paglist row item
|
|
PSNotLast = 0x200, // 512 - use this token on all but the last page of this section
|
|
PSRtfOnly = 0x400, // Only use this token when the driver is rtf
|
|
PSRtfNot = 0x800, // Do NOT use token when driver is rtf
|
|
PSGDIOnly = 0X1000, // Only use this token when the driver is GDI
|
|
PSGDINot = 0x2000, // Do NOT use token when driver is GDI
|
|
PSAdjBngRow = 0x4000 // If the pagelist item exceeds the row it's printed on,
|
|
// then adjust the starting row for the procedure body
|
|
};
|
|
// Possible settings for DocStructStyle (DSS_)
|
|
// These styles describe How and When the defined Doc Style is used
|
|
[Flags] public enum E_DocStructStyle:uint
|
|
{
|
|
UseOnAllPages = 0, // Default
|
|
UseOnFirstPage = 1, // Use only on the first page
|
|
UseOnAllButFirstPage = 2, // Use on all but the first page
|
|
UseOnLastPage = 4, // NO LOGIC exists for this selection. Use only on the last page
|
|
UseSectionFoldout = 8, // Attach section foldouts (only active if using SectionLevelFoldouts_ON
|
|
DontCountFoldoutPgs = 16, // Used with the USESECTIONFOLDOUT flag. Keeps foldout pages from
|
|
// being included in the section total page count.
|
|
TableOfContents = 32,
|
|
DontCountInTabOfCont = 64,
|
|
Placekeeper = 128,
|
|
Align1StLevSubWHLS = 0x00000100, // guess?
|
|
DoubleBoxHLS = 0x00000200,
|
|
UseAltCntrline = 0x00000400,
|
|
DontNumberInTOC = 0x00000800, // Don't include page number for this section in the Table of Contents
|
|
UseSpecialFigFrame = 0x00001000, // for use with stp55 and 55a in bge
|
|
HLSUseLetters = 0x00002000, // docstyles with this bit set in the DocStructStyle will
|
|
// default to using letters for HLSteps
|
|
NoSectionInStepContinue = 0x00004000, // don't include the section number in the step continue
|
|
BottomSectionContinue = 0x00008000, // print the continue message if the section continues
|
|
AlwaysDotStep = 0x00010000, // put the period after step number in the continue message
|
|
XBlankW1stLevSub = 0x00020000, // insert an extra blank line before a 1st level substep
|
|
AffectedPages = 0x00040000,
|
|
DSS_TreatAsTrueSectNum = 0x00080000, // in conjunction with tietabtolevel, takes section number
|
|
// from the last space and appends a period
|
|
SampleWatermark = 0x00100000, // Will force "SAMPLE" to be printed across the page
|
|
// on all pages in this section
|
|
DSS_PageBreakHLS = 0x00200000, // Page Breaks on all high level steps
|
|
DSS_NoChkIfContTypeHigh = 0x00400000, // Will suppress the checkoff if type is HIGH and the
|
|
// step is continued on the next page
|
|
DSS_WidthOvrdStdHLS = 0x00800000, // Width Override for Standard HLStep in this section
|
|
DSS_AddDotZeroStdHLS = 0x01000000, // Append .0 to the Standard HLStep for this section
|
|
DSS_SectionCompress = 0x02000000, // Compress all the steps of this section (i.e. use 7 LPI)
|
|
DSS_PrintSectOnFirst = 0x04000000, // Prints section title/number <SECTIONLEVELTITLE> only on the first
|
|
// page of an attachment section, assuming numberingsequence is not 1
|
|
DSS_UnNumLikeRoman = 0x08000000, // the substeps underneath unnumbered HLSteps will have same tabs as romans
|
|
DSS_DontChangeRomanLevel = 0x10000000, // Dont alter the the substep level for roman-numeral-tabbed hlsteps
|
|
DSS_SkipTwoStepLevels = 0x20000000, // Skip two step levels for this doc style
|
|
DSS_SkipOneStepLevel = 0x40000000, // Skip one step level for this doc style
|
|
DSS_SimpleTopSectionContinue = 0x80000000, // Use the Top continue message as the section continue */
|
|
};
|
|
public enum E_DocStyleUse:uint
|
|
{
|
|
UseOnAllPages=0,UseOnFirstPage=1,UseOnAllButFirstPage=2,UseOnLastPage=4
|
|
};
|
|
[Flags]
|
|
public enum E_DocStyle:uint
|
|
{
|
|
None=0,UseSectionFoldout=8,DontCountFoldoutPgs=16,TableOfContents=32,
|
|
DontCountInTabOfCont=64,Placekeeper=128,Align1StLevSubWHLS=0x00000100,
|
|
DoubleBoxHLS=0x00000200,UseAltCntrline=0x00000400,DontNumberInTOC=0x00000800,
|
|
UseSpecialFigFrame=0x00001000,HLSUseLetters=0x00002000,NoSectionInStepContinue=0x00004000,
|
|
BottomSectionContinue=0x00008000,AlwaysDotStep=0x00010000,XBlankW1stLevSub=0x00020000,
|
|
AffectedPages=0x00040000,DSS_TreatAsTrueSectNum=0x00080000,SampleWatermark=0x00100000,
|
|
DSS_PageBreakHLS=0x00200000,DSS_NoChkIfContTypeHigh=0x00400000,DSS_WidthOvrdStdHLS=0x00800000,
|
|
DSS_AddDotZeroStdHLS=0x01000000,DSS_SectionCompress=0x02000000,DSS_PrintSectOnFirst=0x04000000,
|
|
DSS_UnNumLikeRoman=0x08000000,DSS_DontChangeRomanLevel=0x10000000,
|
|
DSS_SkipTwoStepLevels=0x20000000,DSS_SkipOneStepLevel=0x40000000,DSS_SimpleTopSectionContinue=0x80000000
|
|
};
|
|
//[Flags]
|
|
//public enum E_Style:uint
|
|
//{
|
|
// ELITE=0,PICA=1,SANSERIF=2,EXPANDED=4,PICA12=5,COMPRESSEDHVLPT18=8,HVLPT25=9,SPECIALCHARS=10,PT14=11,SANSERIF14=12,SANSERIF17=13,
|
|
// HVLPT12=14,NARRATOR=15,MEDUPUNIVERS=16,LGUPMED16=17,PROPT10=18,LG1275HP4SI=19,
|
|
// HVLPT10=20,HVLPT8=21,HVLPT14=22,SANSERIF25=23,EYECHART=24,TIMES11=25,SANSCOND=26,
|
|
// UNDERLINE=64,BOLD=128,LANDSCAPE=256,ITALICS=512,BOXED=1024,BOXELEMENT=0x00000800,
|
|
// TBCENTERED=4096,RTCHECKOFF=8192,LTCHECKOFF=16384,BIGSCRIPT=32768,HLTEXTHL=65536,
|
|
// RTCHECKOFFWITHASTERISK=131072,DB_UNDERLINE=262144,COLDOTS=524288,
|
|
// MMBOLD=1048576,RIGHTJUSTIFY=2097152,SUBSCRIPT=4194304,SUPERSCRIPT=8388608,
|
|
// PAGELISTITEM=16777216,PRINTONTOPOFLINE=33554432,HORZCENTER=67108864,
|
|
// CIRCLESTRING2=0x08000000,ALIGNWITHUP1=0x10000000,ALIGNWSECNUM=0x20000000,
|
|
// MATCHCOLUMNMODE=0x40000000,KEEPRNOSUBSTYLE=0x80000000
|
|
//};
|
|
public enum E_FontName:uint
|
|
{
|
|
Elite=0,Pica=1,LnPrn=2,Condense=3,SanSerif=4,Pica12=5,Proportional=6,
|
|
Propt12=7,HvlPt18=8,HvlPt25=9,SpecialChars=10,Pt14=11,SanSerif14=12,SanSerif17=13,
|
|
HvlPt12=14,Narrator=15,MedUpUnivers=16,LgUpMed16=17,Propt10=18,Lg1275Hp4Si=19,
|
|
HvlPt10=20,HvlPt8=21,HvlPt14=22,SanSerif25=23,EyeChart=24,Times11=25,SansCond=26
|
|
};
|
|
//[Flags]
|
|
//public enum E_FontStyle:uint
|
|
//{
|
|
// NONE=0,UNDERLINE=64,BOLD=128,LANDSCAPE=256,ITALICS=512,BOXED=1024,BOXELEMENT=0x00000800,
|
|
// TBCENTERED=4096,RTCHECKOFF=8192,LTCHECKOFF=16384,BIGSCRIPT=32768,HLTEXTHL=65536,
|
|
// RTCHECKOFFWITHASTERISK=131072,DB_UNDERLINE=262144,COLDOTS=524288,
|
|
// MMBOLD=1048576,RIGHTJUSTIFY=2097152,SUBSCRIPT=4194304,SUPERSCRIPT=8388608,
|
|
// PAGELISTITEM=16777216,PRINTONTOPOFLINE=33554432,HORZCENTER=67108864,
|
|
// CIRCLESTRING2=0x08000000,ALIGNWITHUP1=0x10000000,ALIGNWSECNUM=0x20000000,
|
|
// MATCHCOLUMNMODE=0x40000000,KEEPRNOSUBSTYLE=0x80000000
|
|
//};
|
|
[Flags] public enum E_FontStyle:uint
|
|
{
|
|
None=0,Underline=64,Bold=128,Italics=512,BigScript=32768,
|
|
DbUnderline=262144,MmBold=1048576,Subscript=4194304,Superscript=8388608
|
|
};
|
|
public enum E_Justify : uint
|
|
{
|
|
None=0, Center=1, Left=2, Right=3
|
|
};
|
|
public enum E_TabCheckOff : uint
|
|
{
|
|
None=0, Left=1, Right=2
|
|
};
|
|
[Flags] public enum E_Style:uint
|
|
{
|
|
None=0,Landscape=256,Boxed=1024,BoxElement=0x00000800,TbCentered=4096,RtCheckoff=8192,
|
|
LtCheckOff=16384,HlTextHl=65536,RtCheckoffWithAsterisk=131072,ColDots=524288,
|
|
RightJustify=2097152,PageListItem=16777216,PrintOnTopOfLine=33554432,
|
|
HorzCenter=67108864,CircleString2=0x08000000,AlighWithUp1=0x10000000,
|
|
AlignWSecNum=0x20000000,MatchColumnMode=0x40000000,KeepRNOSubStyle=0x80000000
|
|
};
|
|
|
|
public enum E_OldToNewSteps : uint
|
|
{
|
|
S0 = 1, S1 = 2, S2 = 4, E0 = 1048576, E1 = 2097152, E2 = 4194304, X0 = 67108864, X1 = 134217728, X2 = 268435456
|
|
};
|
|
#endregion
|
|
#region TypeConverters
|
|
[Serializable,TypeConverter(typeof(VE_DocStyleConverter))]
|
|
public class VE_DocStyle
|
|
{
|
|
uint i;
|
|
public VE_DocStyle(string s)
|
|
{
|
|
|
|
}
|
|
public VE_DocStyle(uint i)
|
|
{
|
|
this.i=i;
|
|
}
|
|
public VE_DocStyle()
|
|
{
|
|
this.i=0;
|
|
}
|
|
public E_DocStyleUse DocStyleUse
|
|
{
|
|
get{return (E_DocStyleUse)(i & 0x7);}
|
|
set{i = ((uint)value) | (i & 0xFFFFFFF8);}
|
|
}
|
|
public string DocStyle
|
|
{
|
|
get{return ((E_DocStyle)(i & 0xFFFFFFF8)).ToString();}
|
|
set{i = ((uint)Enum.Parse(typeof (E_DocStyle),value)) | (i & 0x7);}
|
|
}
|
|
}
|
|
internal class VE_DocStyleConverter:ExpandableObjectConverter
|
|
{
|
|
public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType)
|
|
{
|
|
if(sourceType == typeof(string))
|
|
{
|
|
return false;
|
|
}
|
|
return base.CanConvertFrom(context,sourceType);
|
|
}
|
|
public override object ConvertFrom(ITypeDescriptorContext context, System.Globalization.CultureInfo culture, object value)
|
|
{
|
|
return base.ConvertFrom (context, culture, value);
|
|
}
|
|
public override object ConvertTo(ITypeDescriptorContext context, System.Globalization.CultureInfo culture, object value, Type destinationType)
|
|
{
|
|
if(destinationType==typeof(string))
|
|
{
|
|
VE_DocStyle v = (VE_DocStyle)value;
|
|
return string.Format((v.DocStyle=="None"?"{0}":"{0}, {1}"),v.DocStyleUse,v.DocStyle);
|
|
}
|
|
return base.ConvertTo (context, culture, value, destinationType);
|
|
}
|
|
}
|
|
|
|
[Serializable,TypeConverter(typeof(VE_FontConverter))]
|
|
public class VE_Font
|
|
{
|
|
uint i;
|
|
private bool _PartialInheritance = false;
|
|
|
|
//public bool PartialInheritance
|
|
//{
|
|
// get { return _PartialInheritance; }
|
|
// set { _PartialInheritance = value; }
|
|
//}
|
|
public bool CanInherit()
|
|
{
|
|
return !_PartialInheritance;
|
|
}
|
|
public VE_Font(string s)
|
|
{
|
|
|
|
}
|
|
public VE_Font(uint i)
|
|
{
|
|
this.i=i;
|
|
}
|
|
public VE_Font()
|
|
{
|
|
this.i=0;
|
|
}
|
|
public VE_Font(XmlNode fxml)
|
|
{
|
|
XmlNode tmp = fxml.SelectSingleNode("Family");
|
|
if (tmp!=null)FontFamily = tmp.InnerText;
|
|
tmp = fxml.SelectSingleNode("Size");
|
|
if (tmp != null) FontSize = tmp.InnerText;
|
|
tmp = fxml.SelectSingleNode("Style");
|
|
if (tmp != null) FontStyle = tmp.InnerText;
|
|
}
|
|
private string _FontFamily = null;
|
|
private bool _FontFamilySet = false;
|
|
|
|
public string FontFamily
|
|
{
|
|
get
|
|
{
|
|
if ((!_FontFamilySet)&&(_FontFamily == null)) _FontFamily = CvtFont.CvtFont.ConvertToFamily(((E_FontName)(i & 0x3f)).ToString());
|
|
_FontFamilySet = true;
|
|
return _FontFamily;
|
|
}
|
|
set { CheckPartial(_FontFamily,value); _FontFamily = value; }
|
|
}
|
|
private void CheckPartial(string member, string value)
|
|
{
|
|
if (member != null && value == null)
|
|
_PartialInheritance = true;
|
|
}
|
|
private string _FontSize = null;
|
|
private bool _FontSizeSet = false;
|
|
public string FontSize
|
|
{
|
|
get
|
|
{
|
|
if ((!_FontSizeSet)&&(_FontSize == null)) _FontSize = CvtFont.CvtFont.ConvertToSize(((E_FontName)(i & 0x3f)).ToString()).ToString();
|
|
_FontSizeSet = true;
|
|
return _FontSize;
|
|
}
|
|
set { CheckPartial(_FontSize, value); _FontSize = value; }
|
|
//set { i = ((uint)value) | (i & 0xFFFFFFC0); }
|
|
}
|
|
//public E_FontName FontName
|
|
//{
|
|
// get{return (E_FontName)(i & 0x3F);}
|
|
// set{i = ((uint)value) | (i & 0xFFFFFFC0);}
|
|
//}
|
|
private string _FontStyle = null;
|
|
private bool _FontStyleSet = false;
|
|
public string FontStyle
|
|
{
|
|
get{
|
|
if ((!_FontStyleSet) && (_FontStyle == null)) _FontStyle = ((E_FontStyle)(i & 0xD482C0)).ToString();
|
|
//if ((!_FontStyleSet) && (_FontStyle == null)) _FontStyle = ((E_FontStyle)(i & 0xFFFFFFC0)).ToString();
|
|
_FontStyleSet = true;
|
|
return _FontStyle;
|
|
}
|
|
// return (E_FontStyle)(i & 0xFFFFFFC0);}
|
|
set { CheckPartial(_FontStyle, value); _FontStyle = value; }
|
|
//set{i = ((uint)value) | (i & 0x3F);}
|
|
}
|
|
private string _CPI;
|
|
private bool _CPISet = false;
|
|
public string CPI
|
|
{
|
|
get
|
|
{
|
|
if (!_CPISet)
|
|
{
|
|
_CPI = CvtFont.CvtFont.GetCPIs(i).ToString();
|
|
}
|
|
_CPISet = true;
|
|
return _CPI;
|
|
}
|
|
set { CheckPartial(_CPI, value); _CPI = value; }
|
|
}
|
|
private string _FontCheckOff = null;
|
|
private bool _FontCheckOffSet = false;
|
|
public string FontCheckOff
|
|
{
|
|
get
|
|
{
|
|
// 16-bit code had check offs in font style integer flag. 32-bit is converting this to its own
|
|
// xml attribute not part of the font. This code is used for the format -> xml conversion
|
|
// the 32-bit code should NOT have this as part of the font. RTCHECKOFF in 16-bit code = 8192
|
|
// and LTCHECKOFF in 16-bit code = 16384
|
|
if ((!_FontCheckOffSet) && (_FontCheckOff == null))
|
|
{
|
|
if ((i & 8192)>0) _FontCheckOff = E_TabCheckOff.Right.ToString();
|
|
else if ((i & 16384) > 0) _FontCheckOff = E_TabCheckOff.Left.ToString();
|
|
else _FontCheckOff = E_TabCheckOff.None.ToString();
|
|
}
|
|
_FontCheckOffSet = true;
|
|
return _FontCheckOff;
|
|
}
|
|
set { CheckPartial(_FontCheckOff, value); _FontCheckOff = value; }
|
|
}
|
|
private string _FontJustify = null;
|
|
private bool _FontJustifySet = false;
|
|
public string FontJustify
|
|
{
|
|
get
|
|
{
|
|
// 16-bit code had justify in font style integer flag. 32-bit is converting this to its own
|
|
// xml attribute not part of the font. This code is used for the format -> xml conversion
|
|
// the 32-bit code should NOT have this as part of the font. TBCENTERED in 16-bit code = 4096
|
|
// and RIGHTJUSTIFY in 16-bit code = 2097152. Note 16-bit did not have a left justify.
|
|
if ((!_FontJustifySet) && (_FontJustify == null))
|
|
{
|
|
if ((i & 4096) > 0) _FontJustify = E_Justify.Center.ToString();
|
|
else if ((i & 2097152) > 0) _FontJustify = E_Justify.Right.ToString();
|
|
else _FontJustify = E_Justify.None.ToString();
|
|
}
|
|
_FontJustifySet = true;
|
|
return _FontJustify;
|
|
}
|
|
set { CheckPartial(_FontJustify, value); _FontJustify = value; }
|
|
}
|
|
public bool IsBoxed
|
|
{
|
|
get
|
|
{
|
|
return ((i & (int)E_Style.Boxed) > 0);
|
|
}
|
|
}
|
|
//CIRCLESTRING2=0x08000000
|
|
public bool HasCircleString2()
|
|
{
|
|
if ((i & 0x08000000)>0) return true;
|
|
else return false;
|
|
}
|
|
public VE_Font Copy()
|
|
{
|
|
VE_Font c = new VE_Font(i);
|
|
return c;
|
|
}
|
|
}
|
|
internal class VE_FontConverter:ExpandableObjectConverter
|
|
{
|
|
public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType)
|
|
{
|
|
if(sourceType == typeof(string))
|
|
{
|
|
return false;
|
|
}
|
|
return base.CanConvertFrom(context,sourceType);
|
|
}
|
|
public override object ConvertFrom(ITypeDescriptorContext context, System.Globalization.CultureInfo culture, object value)
|
|
{
|
|
return base.ConvertFrom (context, culture, value);
|
|
}
|
|
public override object ConvertTo(ITypeDescriptorContext context, System.Globalization.CultureInfo culture, object value, Type destinationType)
|
|
{
|
|
if(destinationType==typeof(string))
|
|
{
|
|
VE_Font v = (VE_Font)value;
|
|
return string.Format((v.FontStyle == "None" ? "Family={0} Size={1}" : "{0}, {1}, {2}"), v.FontFamily, v.FontSize, v.FontStyle);
|
|
}
|
|
return base.ConvertTo (context, culture, value, destinationType);
|
|
}
|
|
}
|
|
#endregion
|
|
|
|
|
|
///////////////////////////
|
|
namespace fmtxml
|
|
{
|
|
public class FmtToXml
|
|
{
|
|
private BinaryReader brFmt;
|
|
private string fmtName;
|
|
private VE_Font DefBaseFont;
|
|
private VE_Font DefPlantFont;
|
|
private string MyPath;
|
|
public FmtToXml(string nm, string path)
|
|
{
|
|
MyPath = path;
|
|
fmtName = nm;
|
|
//if (fmtName.ToUpper() != "OHLP" && fmtName.ToUpper() != "BASE" && fmtName.ToUpper() != "WCN2") return;
|
|
try
|
|
{
|
|
// get the default base & plant files to use when figuring out inheritance.
|
|
if (GetPlantBaseDefaultFonts())
|
|
{
|
|
|
|
// will have entire name, such as aep.x01 - use number to differentiate it.
|
|
int indx = nm.IndexOf('.');
|
|
if (indx > 0)
|
|
{
|
|
LoadPageFile(nm);
|
|
LoadDocFile(nm);
|
|
}
|
|
else
|
|
{
|
|
LoadPageFile(nm + ".pag");
|
|
LoadDocFile(nm + ".doc");
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
MessageBox.Show("Format name = " + nm, ex.Message);
|
|
}
|
|
}
|
|
private float RowToPoints(int i)
|
|
{
|
|
return (i * 12); // row_in_points = input * 72/6, row = input * 12
|
|
}
|
|
private float ColToPoints(float f, uint style)
|
|
{
|
|
return 72 * (f / CvtFont.CvtFont.GetCPIs(style));
|
|
}
|
|
private string GetAsciiStringUntilNull(BinaryReader br)
|
|
{
|
|
StringBuilder inpstr = new StringBuilder();
|
|
byte input = 0;
|
|
int cnt = 0;
|
|
bool stillread = true;
|
|
do
|
|
{
|
|
try
|
|
{
|
|
input = br.ReadByte();
|
|
if (input==0) stillread=false;
|
|
inpstr.Append(Convert.ToChar(input));
|
|
}
|
|
catch (System.IO.EndOfStreamException)
|
|
{
|
|
stillread=false;
|
|
}
|
|
cnt++;
|
|
}while (stillread);
|
|
|
|
string retstr = inpstr.ToString();
|
|
if (retstr.Substring(retstr.Length-1,1)=="\0") return retstr.Substring(0,retstr.Length-1);
|
|
return retstr;
|
|
}
|
|
private string GetAsciiString(BinaryReader br, int len)
|
|
{
|
|
StringBuilder inpstr = new StringBuilder();
|
|
byte input = 0;
|
|
int cnt = 0;
|
|
do
|
|
{
|
|
try
|
|
{
|
|
input = br.ReadByte();
|
|
inpstr.Append(Convert.ToChar(input));
|
|
}
|
|
catch (System.IO.EndOfStreamException)
|
|
{
|
|
input = 0;
|
|
while (cnt < len)
|
|
{
|
|
inpstr.Append(Convert.ToChar(input));
|
|
cnt++;
|
|
}
|
|
}
|
|
cnt++;
|
|
}while (cnt < len);
|
|
|
|
string retstr = inpstr.ToString();
|
|
if (retstr.Substring(retstr.Length-1,1)=="\0") return retstr.Substring(0,retstr.Length-1);
|
|
return retstr;
|
|
}
|
|
private VE_Font LoadVE_Font()
|
|
{
|
|
VE_Font retval=new VE_Font(brFmt.ReadUInt32());
|
|
return retval;
|
|
}
|
|
private VE_DocStyle LoadVE_DocStyle()
|
|
{
|
|
VE_DocStyle retval=new VE_DocStyle(brFmt.ReadUInt32());
|
|
return retval;
|
|
}
|
|
public void LoadPageFile(string fname)
|
|
{
|
|
ArrayList stringoffsets = new ArrayList();
|
|
short strings = 0;
|
|
PageStyles pgstyles = new PageStyles();
|
|
|
|
// read in title.
|
|
string fnm = MyPath + @"\" + fname;
|
|
brFmt = new BinaryReader(File.Open(fnm,System.IO.FileMode.Open,System.IO.FileAccess.ReadWrite,FileShare.ReadWrite));
|
|
pgstyles.Name = GetAsciiString(brFmt);
|
|
|
|
// read in struct name/def name/page file name
|
|
//for (int i=0;i<3;i++)
|
|
//{
|
|
// len = brFmt.ReadInt16();
|
|
// str = GetAsciiString(brFmt,len);
|
|
//}
|
|
string structName = GetAsciiString(brFmt);
|
|
string includeName = GetAsciiString(brFmt);
|
|
string formatName = GetAsciiString(brFmt);
|
|
// Get the number of page styles, and create the array for them.
|
|
short numpgstyles = brFmt.ReadInt16();
|
|
|
|
PageStyle [] pgs = new PageStyle[numpgstyles];
|
|
pgstyles.PgStyles = pgs;
|
|
|
|
short offptr = brFmt.ReadInt16();
|
|
short vnum = brFmt.ReadInt16();
|
|
if (vnum!=0)
|
|
{
|
|
strings = vnum;
|
|
for(int i=0;i<strings;i++)
|
|
{
|
|
vnum=brFmt.ReadInt16();
|
|
stringoffsets.Add(vnum);
|
|
}
|
|
}
|
|
bool didLineDraw = false;
|
|
for (short i=0;i<numpgstyles;i++)
|
|
{
|
|
PageStyle pg = new PageStyle();
|
|
pg.Index=i;
|
|
pg.Name = GetAsciiString(brFmt);
|
|
|
|
PSItem [] psitms = new PSItem[1];
|
|
PSItem [] Fpsitms = null;
|
|
bool isfirst=true;
|
|
int cnt = 0;
|
|
|
|
while ((vnum = brFmt.ReadInt16()) != 0)
|
|
{
|
|
long fseek = brFmt.BaseStream.Position;
|
|
PSItem pi = new PSItem();
|
|
int tmp = brFmt.ReadInt32(); // not sure what's stored here?
|
|
// convert the row & column to points, i.e. a point = 1/72 inch
|
|
// the row is set based on 6 rows per inch
|
|
// the column is 12 char/inch
|
|
pi.Row = RowToPoints(brFmt.ReadInt16());
|
|
int lcol = brFmt.ReadInt16();
|
|
uint tmpjust = brFmt.ReadUInt16();
|
|
E_PageStructMod etmpjust = (E_PageStructMod)tmpjust;
|
|
pi.Justify = ((E_PageStructMod)tmpjust).ToString();
|
|
float adj = 0.5F;
|
|
if ((etmpjust & E_PageStructMod.PSTrue) == 0) adj = -0.5F;
|
|
if ((etmpjust & E_PageStructMod.PSLeft) == E_PageStructMod.PSLeft) adj = 0;
|
|
if ((etmpjust & E_PageStructMod.PSRight) == E_PageStructMod.PSRight) adj *= 2;
|
|
uint tmpstyle = brFmt.ReadUInt32();
|
|
pi.Style = new VE_Font(tmpstyle);
|
|
string tkn = DoReplaceTokens(GetAsciiStringUntilNull(brFmt));
|
|
// see if any initial line draw chars are in token (WCN2 and others). If so,
|
|
// remove the line draw & its following space. Also adjust the column.
|
|
int coladj = 0; // see if any initial draw chars are included and if so remove.
|
|
pi.Token = tkn==null||tkn==""?tkn:RemoveInitialLineDraw(tkn, ref coladj);
|
|
pi.Col = ColToPoints(lcol + coladj + adj, tmpstyle);
|
|
if (pi.Token != null)
|
|
{
|
|
// replace the '<' with '{' & '>' with '}'
|
|
pi.Token = pi.Token.Replace('<', '{');
|
|
pi.Token = pi.Token.Replace('>', '}');
|
|
|
|
cnt++;
|
|
if (!isfirst)
|
|
{
|
|
Fpsitms = new PSItem[cnt];
|
|
psitms.CopyTo(Fpsitms, 0);
|
|
Fpsitms[cnt - 1] = pi;
|
|
psitms = Fpsitms;
|
|
|
|
}
|
|
else
|
|
psitms[0] = pi;
|
|
isfirst = false;
|
|
}
|
|
else
|
|
{
|
|
if (!didLineDraw)
|
|
{
|
|
didLineDraw = true;
|
|
pi.Token = "{BOX9}";
|
|
cnt++;
|
|
if (!isfirst)
|
|
{
|
|
Fpsitms = new PSItem[cnt];
|
|
psitms.CopyTo(Fpsitms, 0);
|
|
Fpsitms[cnt - 1] = pi;
|
|
psitms = Fpsitms;
|
|
|
|
}
|
|
else
|
|
psitms[0] = pi;
|
|
isfirst = false;
|
|
}
|
|
}
|
|
brFmt.BaseStream.Position = fseek+vnum;
|
|
}
|
|
pg.Items = psitms;
|
|
pgs[i] = pg;
|
|
}
|
|
brFmt.Close();
|
|
DoPSFontInherit(ref pgstyles);
|
|
|
|
// First serialize files based on the above - then use xsl to transform
|
|
// into prefered xml.
|
|
if (!Directory.Exists("fmt_xml")) Directory.CreateDirectory("fmt_xml");
|
|
if (!Directory.Exists("tmp_fmt_xml")) Directory.CreateDirectory("tmp_fmt_xml");
|
|
XmlSerializer serializer = new XmlSerializer(typeof(PageStyles));
|
|
string lfmtname = fmtName;
|
|
// for subformats, make the name FMT_00 where FMT is format name & 00 is number
|
|
// from original name. This will allow the data load to know it's a sub.
|
|
int indx = fmtName.IndexOf('.');
|
|
if (indx>0)
|
|
lfmtname = fmtName.Substring(0,fmtName.Length-4) + "_" + fmtName.Substring(fmtName.Length-2,2);
|
|
string destfile = "tmp_fmt_xml\\" + lfmtname + "p.xml";
|
|
if (File.Exists(destfile)) File.Delete(destfile);
|
|
TextWriter writer = new StreamWriter(destfile);
|
|
try
|
|
{
|
|
serializer.Serialize(writer, pgstyles);
|
|
writer.Close();
|
|
XslCompiledTransform xsl = FmtFileToXml.getTransform(Application.StartupPath + "\\TranslatePag.XSL");
|
|
string sResults = "fmt_xml\\" + lfmtname + "p.xml";
|
|
xsl.Transform(destfile, sResults); // Perform Transform
|
|
if (lfmtname == "GEN")
|
|
File.Copy(sResults, "fmt_xml\\BASEp.xml"); // Create BASE from GEN format
|
|
}
|
|
catch (Exception e)
|
|
{
|
|
Console.WriteLine(e.Message);
|
|
writer.Close();
|
|
}
|
|
}
|
|
|
|
private string RemoveInitialLineDraw(string tkn, ref int coladj)
|
|
{
|
|
// if first two characters are line draw and space, remove them and adjust column.
|
|
if (tkn[0] == '\xB3' && tkn[1] == ' ')
|
|
{
|
|
coladj += 2;
|
|
tkn = tkn.Substring(2, tkn.Length - 2);
|
|
//trim any end line char too.
|
|
tkn = tkn.Replace('\xB3',' ');
|
|
tkn = tkn.TrimEnd(" ".ToCharArray());
|
|
}
|
|
return tkn;
|
|
}
|
|
|
|
private string GetAsciiString(BinaryReader brFmt)
|
|
{
|
|
short len = brFmt.ReadInt16();
|
|
return GetAsciiString(brFmt, len);
|
|
}
|
|
public void LoadDocFile(string fname)
|
|
{
|
|
ArrayList stringoffsets = new ArrayList();
|
|
short strings = 0;
|
|
DocStyles dcstyles = new DocStyles();
|
|
|
|
// read in title.
|
|
string fnm = MyPath + @"\" + fname;
|
|
// construct format file name from this path.\
|
|
string fmtFileName = fname;
|
|
if (fname.Contains(".Y"))
|
|
{
|
|
// subformat - replace .Y with .X
|
|
fmtFileName = MyPath + @"\" + fname.Replace(".Y", ".X");
|
|
}
|
|
else
|
|
{
|
|
fmtFileName = MyPath + @"\" + fname.Substring(0, fname.IndexOf(".")) + ".FMT";
|
|
}
|
|
if (fname == "CPL.Y01") Console.WriteLine(fname);
|
|
brFmt = new BinaryReader(File.Open(fnm,System.IO.FileMode.Open,System.IO.FileAccess.ReadWrite,FileShare.ReadWrite));
|
|
dcstyles.Name = GetAsciiString(brFmt);
|
|
|
|
// read in struct name/def name/doc file name
|
|
string structName = GetAsciiString(brFmt);
|
|
string includeName = GetAsciiString(brFmt);
|
|
string formatName = GetAsciiString(brFmt);
|
|
DocStyle[] dcs = null;
|
|
if (structName != "DOCSTYL.STR")
|
|
{
|
|
Console.WriteLine("Other Structure {0}", structName);
|
|
if (structName == "BKPGDOC.STR")
|
|
{
|
|
for(int i=0;i<4;i++) brFmt.ReadInt16(); // Skip 4 integers
|
|
dcs = new DocStyle[4];
|
|
string baseName = GetAsciiString(brFmt);
|
|
dcs[0].Index = 0;
|
|
dcs[1].Index = 1;
|
|
dcs[2].Index = 2;
|
|
dcs[3].Index = 3;
|
|
dcs[0].Name = baseName + "- portrait @ 6 lpi";
|
|
dcs[1].Name = baseName + "- portrait @ 4 lpi";
|
|
dcs[2].Name = baseName + "- landscape @ 6 lpi";
|
|
dcs[3].Name = baseName + "- landscape @ 4 lpi";
|
|
brFmt.ReadInt16();
|
|
dcs[0].dstyle = LoadVE_Font();
|
|
dcs[1].dstyle = dcs[0].dstyle.Copy();
|
|
dcs[2].dstyle = dcs[0].dstyle.Copy();
|
|
dcs[3].dstyle = dcs[0].dstyle.Copy();
|
|
dcs[0].TopMargin = dcs[1].TopMargin = dcs[2].TopMargin = dcs[3].TopMargin = brFmt.ReadInt16();
|
|
dcs[0].LeftMargin = dcs[1].LeftMargin = dcs[2].LeftMargin = dcs[3].LeftMargin = brFmt.ReadInt16();
|
|
dcs[0].PageLength = brFmt.ReadInt16();
|
|
dcs[1].PageLength = brFmt.ReadInt16();
|
|
dcs[2].PageLength = brFmt.ReadInt16();
|
|
dcs[3].PageLength = brFmt.ReadInt16();
|
|
}
|
|
}
|
|
else
|
|
{
|
|
|
|
// Get the number of page styles, and create the array for them.
|
|
short numdcstyles = brFmt.ReadInt16();
|
|
dcs = new DocStyle[numdcstyles];
|
|
|
|
short offptr = brFmt.ReadInt16(); // not sure what these bytes contain?
|
|
short vnum = brFmt.ReadInt16();
|
|
if (vnum != 0)
|
|
{
|
|
strings = vnum;
|
|
for (int i = 0; i < strings; i++)
|
|
{
|
|
vnum = brFmt.ReadInt16();
|
|
stringoffsets.Add(vnum);
|
|
}
|
|
}
|
|
|
|
for (short i = 0; i < numdcstyles; i++)
|
|
{
|
|
DocStyle dc = new DocStyle();
|
|
dc.Index = i;
|
|
dc.Name = GetAsciiString(brFmt);
|
|
vnum = brFmt.ReadInt16(); // not sure what this is!
|
|
dc.pagestyle = brFmt.ReadInt16();
|
|
uint tmpstyle = brFmt.ReadUInt32();
|
|
dc.dstyle = new VE_Font(tmpstyle);
|
|
dc.TopMargin = RowToPoints(brFmt.ReadInt16());
|
|
dc.FooterLen = RowToPoints(brFmt.ReadInt16());
|
|
dc.LeftMargin = ColToPoints(brFmt.ReadInt16(), tmpstyle);
|
|
dc.PageLength = RowToPoints(brFmt.ReadInt16());
|
|
dc.PageWidth = ColToPoints(brFmt.ReadInt16(), tmpstyle);
|
|
dc.numberingsequence = brFmt.ReadInt16();
|
|
dc.oldtonew = brFmt.ReadInt32();
|
|
SetupDocStyleFromFmtFlags(ref dc, fmtFileName);
|
|
dc.IsStepSection = ConvertToSectType(dc.oldtonew);
|
|
dc.ContTopHLS = brFmt.ReadInt16();
|
|
dc.CTMargin = RowToPoints(brFmt.ReadInt16());
|
|
dc.CBMargin = RowToPoints(brFmt.ReadInt16());
|
|
dc.CBLoc = brFmt.ReadInt16();
|
|
dc.ContStyle = LoadVE_Font();
|
|
dc.EndFlag = brFmt.ReadInt16();
|
|
dc.EndStyle = LoadVE_Font();
|
|
dc.UseCheckOffs = UseCheckOffsIn(fname.Substring(0, fname.Length - 4), dc.oldtonew);
|
|
// use the string offsets to read in the strings.
|
|
int[] offst = new int[strings];
|
|
for (int j = 0; j < strings; j++)
|
|
{
|
|
offst[j] = brFmt.ReadInt32();
|
|
}
|
|
dc.DocStructStyle = LoadVE_DocStyle();
|
|
if (offst[0] != 0) dc.ContTop = DoReplaceTokens(GetAsciiStringUntilNull(brFmt));
|
|
else dc.ContTop = null;
|
|
if (offst[1] != 0) dc.ContBottom = DoReplaceTokens(GetAsciiStringUntilNull(brFmt));
|
|
else dc.ContBottom = null;
|
|
if (offst[2] != 0) dc.EndString = DoReplaceTokens(GetAsciiStringUntilNull(brFmt));
|
|
else dc.EndString = null;
|
|
if (dc.EndString == "")
|
|
{
|
|
dc.EndString = null;
|
|
dc.EndFlag = 0;
|
|
}
|
|
if (offst[3] != 0) dc.FinalMsg = DoReplaceTokens(GetAsciiStringUntilNull(brFmt));
|
|
else dc.FinalMsg = null;
|
|
if (dc.ContTop == null && dc.ContBottom == null) dc.ContStyle = null;
|
|
if (dc.EndString == null) dc.EndStyle = null;
|
|
|
|
dcs[i] = dc;
|
|
vnum = brFmt.ReadInt16();
|
|
}
|
|
}
|
|
dcstyles.DcStyles = dcs;
|
|
brFmt.Close();
|
|
|
|
// Inherit fonts.
|
|
DoDSFontInherit(ref dcstyles);
|
|
|
|
// First serialize files based on the above - then use xsl to transform
|
|
// into prefered xml.
|
|
if (!Directory.Exists("fmt_xml")) Directory.CreateDirectory("fmt_xml");
|
|
if (!Directory.Exists("tmp_fmt_xml")) Directory.CreateDirectory("tmp_fmt_xml");
|
|
XmlSerializer serializer = new XmlSerializer(typeof(DocStyles));
|
|
string lfmtname = fmtName;
|
|
int indx = fmtName.IndexOf('.');
|
|
// for subformats, make the name FMT_00 where FMT is format name & 00 is number
|
|
// from original name. This will allow the data load to know it's a sub.
|
|
if (indx > 0)
|
|
lfmtname = fmtName.Substring(0, fmtName.Length - 4) + "_" + fmtName.Substring(fmtName.Length - 2, 2);
|
|
string destfile = "tmp_fmt_xml\\" + lfmtname + "d.xml";
|
|
if (File.Exists(destfile)) File.Delete(destfile);
|
|
TextWriter writer = new StreamWriter(destfile);
|
|
try
|
|
{
|
|
serializer.Serialize(writer, dcstyles);
|
|
writer.Close();
|
|
XslCompiledTransform xsl = FmtFileToXml.getTransform(Application.StartupPath + "\\TranslateDoc.XSL");
|
|
string sResults = "fmt_xml\\" + lfmtname + "d.xml";
|
|
xsl.Transform(destfile, sResults); // Perform Transform
|
|
xsl = FmtFileToXml.getTransform(Application.StartupPath + "\\removeFmtUseCO.XSL");
|
|
//xsl.Load(Application.StartupPath + "\\removeempty.XSL");
|
|
string sFormatResults = "fmt_xml\\" + lfmtname + "f.xml";
|
|
string sResults2 = "fmt_xml\\" + lfmtname + "xf.xml";
|
|
xsl.Transform(sFormatResults, sResults2);
|
|
File.Delete(sFormatResults);
|
|
File.Move(sResults2, sFormatResults);
|
|
if (lfmtname == "GEN")
|
|
File.Copy(sResults, "fmt_xml\\BASEd.xml"); // Create BASE from GEN format
|
|
}
|
|
catch (Exception e)
|
|
{
|
|
Console.WriteLine(e.Message);
|
|
writer.Close();
|
|
}
|
|
}
|
|
|
|
private bool UseCheckOffsIn(string p, int p_2)
|
|
{
|
|
try
|
|
{
|
|
XmlDocument xdoc = new XmlDocument();
|
|
string path = "fmt_xml\\" + p + "f.xml";
|
|
if (!File.Exists(path)) return false;
|
|
xdoc.Load("fmt_xml\\" + p + "f.xml");
|
|
XmlNode useCO = xdoc.SelectSingleNode("FormatData/ProcData/CheckOffData/@UseCheckOffsIn");
|
|
if (useCO == null) return false;
|
|
int iDoc = System.Convert.ToInt32(useCO.Value);
|
|
if ((p_2 & iDoc)>0) return true;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
Console.WriteLine("here");
|
|
}
|
|
return false;
|
|
}
|
|
private void SetupDocStyleFromFmtFlags(ref DocStyle dc, string fmtFileName)
|
|
{
|
|
int oldToNew = dc.oldtonew;
|
|
BinaryReader brFmt = new BinaryReader(File.Open(fmtFileName, System.IO.FileMode.Open, System.IO.FileAccess.ReadWrite, FileShare.ReadWrite));
|
|
int[] tmpshort3 = new int[3];
|
|
ArrayList stringoffsets = new ArrayList();
|
|
byte tmpbyte;
|
|
Int16 tmpshort;
|
|
string tmpstr;
|
|
try
|
|
{
|
|
tmpshort = brFmt.ReadInt16();
|
|
tmpstr = fmtxml.FmtFileToXml.GetStringUntilNull(brFmt);
|
|
for (int i = 0; i < 3; i++)
|
|
{
|
|
tmpshort = brFmt.ReadInt16();
|
|
tmpstr = fmtxml.FmtFileToXml.GetStringUntilNull(brFmt);
|
|
}
|
|
|
|
short vnum = brFmt.ReadInt16(); // number of ints, etc.
|
|
long posatints = brFmt.BaseStream.Position;
|
|
long tstloc = vnum + posatints;
|
|
tmpbyte = brFmt.ReadByte(); // FirstChar
|
|
Int16[] xtraflag = new Int16[19];
|
|
for (int i = 0; i < 19; i++)
|
|
{
|
|
xtraflag[i] = brFmt.ReadInt16();
|
|
}
|
|
dc.CancelSectTitle = CheckSectType(oldToNew, xtraflag);
|
|
dc.SpecialStepsFoldout = GetSpecialStepsFoldout(oldToNew, xtraflag);
|
|
dc.UndSpecialStepsFoldout = GetUndSpecialStepsFoldout(oldToNew, xtraflag);
|
|
}
|
|
catch (Exception exception)
|
|
{
|
|
Console.WriteLine("Didn't process docstyle");
|
|
}
|
|
}
|
|
|
|
private bool GetUndSpecialStepsFoldout(int oldToNew, Int16[] flg)
|
|
{
|
|
if (((oldToNew & (int)E_OldToNewSteps.E2) != 0) && ((flg[10] & fmtxml.FmtFileToXml.UNDSPECIALSTEPSFOLDOUT) > 0)) return true;
|
|
return false;
|
|
}
|
|
|
|
private bool GetSpecialStepsFoldout(int oldToNew, Int16[] flg)
|
|
{
|
|
if (((oldToNew & (int)E_OldToNewSteps.E2) != 0) && ((flg[7] & fmtxml.FmtFileToXml.SPECIALSTEPSFOLDOUT) > 0)) return true;
|
|
return false;
|
|
}
|
|
private bool CheckSectType(int oldToNew, Int16[] flg)
|
|
{
|
|
if (((oldToNew & (int)E_OldToNewSteps.S2) != 0) && ((flg[6] & fmtxml.FmtFileToXml.CANCELSECTITLESONS2) > 0)) return true;
|
|
if (((oldToNew & (int)E_OldToNewSteps.S1) != 0) && ((flg[7] & fmtxml.FmtFileToXml.CANCELSECTITLESONS1) > 0)) return true;
|
|
if (((oldToNew & (int)E_OldToNewSteps.E0) != 0) && ((flg[11] & fmtxml.FmtFileToXml.CANCELSECTITLESONE0) > 0)) return true;
|
|
if (((oldToNew & (int)E_OldToNewSteps.E2) != 0) && ((flg[11] & fmtxml.FmtFileToXml.CANCELSECTITLESONE2) > 0)) return true;
|
|
return false;
|
|
}
|
|
|
|
private bool ConvertToSectType(int p)
|
|
{
|
|
if (((p & (int)E_OldToNewSteps.S0) != 0) || ((p & (int)E_OldToNewSteps.S1) != 0) || ((p & (int)E_OldToNewSteps.S2) != 0) ||
|
|
((p & (int)E_OldToNewSteps.E0) != 0) || ((p & (int)E_OldToNewSteps.E1) != 0) || ((p & (int)E_OldToNewSteps.E2) != 0) ||
|
|
((p & (int)E_OldToNewSteps.X0) != 0) || ((p & (int)E_OldToNewSteps.X1) != 0) || ((p & (int)E_OldToNewSteps.X2) != 0))
|
|
return true;
|
|
return false;
|
|
}
|
|
private string DoReplaceTokens(string p)
|
|
{
|
|
if (p == null || p == "") return p;
|
|
string wkstr = p;
|
|
// use regular expressions to do the following...
|
|
|
|
// first see if entire string is line draw type chars, if so, just don't include it.
|
|
if (AllDrawChars(wkstr)) return null;
|
|
try
|
|
{
|
|
// replace newline with {par}
|
|
wkstr = wkstr.Replace("\n", "{par}");
|
|
|
|
// special change bar token (various cpl formats)
|
|
wkstr = wkstr.Replace("\x08\x08\x08\x08\x08\x05", "{CHG_BAR with Backspace Token}");
|
|
|
|
// special change bar token (various gpc formats)
|
|
wkstr = wkstr.Replace("\x05", "{CHG_BAR Token}");
|
|
|
|
// backspage - just flag for positioning if needed.
|
|
wkstr = wkstr.Replace("\x08", "{Backspace}");
|
|
|
|
//sub on/off
|
|
wkstr = wkstr.Replace("\x19\x19", "\x19"); // replace double sub on with single
|
|
wkstr = wkstr.Replace("~~", "\x19");
|
|
wkstr = Regex.Replace(wkstr, @"\x19([^\x19\x18 ]*?)(?:[\x19\x18]|(?= )|\Z)(.*?)", @"\sub$1\nosupersub$2");
|
|
|
|
//super on/off
|
|
wkstr = Regex.Replace(wkstr, @"\x18([^\x19\x18 ]*?)(?:[\x19\x18]|(?= )|\Z)(.*?)", @"\super$1\nosupersub$2");
|
|
|
|
//bold on/off
|
|
wkstr = Regex.Replace(wkstr, @"\x01([^\x01 ]*?)(?:[\x01]|(?= )|\Z)(.*?)", @"\b$1\b0$2");
|
|
wkstr = Regex.Replace(wkstr, @"\x13([^\x13\xd6 ]*?)(?:[\x13\xd6]|(?= )|\Z)(.*?)", @"\b$1\b0$2");
|
|
|
|
//underline on/off
|
|
//wkstr = Regex.Replace(wkstr, @"\x16([^\x16 ]*?)(?:[\x16]|(?= )|\Z)(.*?)", @"\ul$1\ul0$2");
|
|
wkstr = Regex.Replace(wkstr, @"\x16([^\x16 ]*?)(?:[\x16]|(?= )|\Z)(.*?)", @"\ul$1\ulnone$2");
|
|
|
|
//narrator
|
|
wkstr = Regex.Replace(wkstr, @"\x86([^\x86 ]*?)(?:[\x86]|(?= )|\Z)(.*?)", @"\f{Narrator}$1\f0$2");
|
|
|
|
// remove hanging indent, vertical tab
|
|
wkstr = Regex.Replace(wkstr, @"\x02", @"\NonSupported Hanging Indent (Hex x02)");
|
|
wkstr = Regex.Replace(wkstr, @"\x0B", @"\NonSupported Vertical Tab (Hex x0b");
|
|
|
|
// remove some unknown chars for ano & fpl - these plants are not supported
|
|
// as of conversion date.
|
|
wkstr = Regex.Replace(wkstr, @"\x95", @"\NonSupported Unknown (Hex x95)"); //ano2vlv
|
|
wkstr = Regex.Replace(wkstr, @"\x1bf1", @"\NonSupported Unknown (hex 1b f1)"); //fpl
|
|
wkstr = Regex.Replace(wkstr, @"\x1bf0", @"\NonSupported Unknown (hex 1b f0)"); //fpl
|
|
wkstr = Regex.Replace(wkstr, @"\x1bM1", @"\NonSupported Unknown (hex 1b M1)"); //fpl
|
|
wkstr = Regex.Replace(wkstr, @"\x1bM0", @"\NonSupported Unknown (hex 1b M0)"); //fpl
|
|
|
|
// ATA page??
|
|
wkstr = Regex.Replace(wkstr, @"\x1b", @"{NonSupported Hex 1b}");
|
|
// replace \xff with \xA0, i.e. hardspace
|
|
wkstr = Regex.Replace(wkstr, @"\xFF", @"\xA0");
|
|
|
|
// replace x10 & x11 in various ano tabs - not this needs fixed if ANO comes
|
|
// back into the program
|
|
wkstr = wkstr.Replace("\x10", "");
|
|
wkstr = wkstr.Replace("\x11", "");
|
|
|
|
// replace x15 with {RO} for now.
|
|
wkstr = wkstr.Replace("\x15", "{RO}");
|
|
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
Console.WriteLine("error replacing token (regex) = {0}", ex.Message);
|
|
}
|
|
|
|
return wkstr;
|
|
}
|
|
|
|
private bool AllDrawChars(string wkstr)
|
|
{
|
|
foreach (char c in wkstr)
|
|
{
|
|
if (c < '\xB3' || c > '\xDF') return false;
|
|
}
|
|
return true;
|
|
}
|
|
private void CompareFonts(VE_Font parFont, ref VE_Font subFont)
|
|
{
|
|
if (parFont != null && subFont != null)
|
|
{
|
|
if (parFont.FontFamily == subFont.FontFamily) subFont.FontFamily = null;
|
|
if (parFont.FontSize == subFont.FontSize) subFont.FontSize = null;
|
|
if (parFont.FontStyle == subFont.FontStyle) subFont.FontStyle = null;
|
|
}
|
|
}
|
|
private void DoPSFontInherit(ref PageStyles pagstyles)
|
|
{
|
|
for (int i=0; i<pagstyles.PgStyles.Length; i++)
|
|
{
|
|
for (int j = 0; j < pagstyles.PgStyles[i].Items.Length; j++)
|
|
{
|
|
CompareFonts(DefPlantFont, ref pagstyles.PgStyles[i].Items[j].Style);
|
|
CompareFonts(DefBaseFont, ref pagstyles.PgStyles[i].Items[j].Style);
|
|
}
|
|
}
|
|
}
|
|
private void DoDSFontInherit(ref DocStyles docstyles)
|
|
{
|
|
// loop through all of the document styles. For each, check font inheritance
|
|
// for End Message Style, Continue Message Style & main document style.
|
|
for (int i=0; i<docstyles.DcStyles.Length; i++)
|
|
{
|
|
CompareFonts(docstyles.DcStyles[i].dstyle, ref docstyles.DcStyles[i].EndStyle);
|
|
CompareFonts(DefPlantFont, ref docstyles.DcStyles[i].EndStyle);
|
|
CompareFonts(DefBaseFont, ref docstyles.DcStyles[i].EndStyle);
|
|
CompareFonts(docstyles.DcStyles[i].dstyle, ref docstyles.DcStyles[i].ContStyle);
|
|
CompareFonts(DefPlantFont, ref docstyles.DcStyles[i].ContStyle);
|
|
CompareFonts(DefBaseFont, ref docstyles.DcStyles[i].ContStyle);
|
|
CompareFonts(DefPlantFont, ref docstyles.DcStyles[i].dstyle);
|
|
CompareFonts(DefBaseFont, ref docstyles.DcStyles[i].dstyle);
|
|
}
|
|
}
|
|
private bool GetPlantBaseDefaultFonts()
|
|
{
|
|
// read the xml files for the base & plant to get the default fonts and
|
|
// make a VE_font for each.
|
|
try
|
|
{
|
|
XmlDocument xdoc = new XmlDocument();
|
|
xdoc.Load("fmt_xml\\basef.xml");
|
|
XmlNode xfont = xdoc.SelectSingleNode("FormatData/Font");
|
|
DefBaseFont = new VE_Font(xfont);
|
|
|
|
string lfmtName = fmtName;
|
|
// for subformats, make the name FMT_00 where FMT is format name & 00 is number
|
|
// from original name. This will allow the data load to know it's a sub.
|
|
int indx = fmtName.IndexOf('.');
|
|
if (indx > 0)
|
|
lfmtName = fmtName.Substring(0, fmtName.Length - 4) + "_" + fmtName.Substring(fmtName.Length - 2, 2);
|
|
string fName = "fmt_xml\\" + lfmtName + "f.xml";
|
|
if (!File.Exists(fName)) return false;
|
|
xdoc.Load(fName);
|
|
xfont = xdoc.SelectSingleNode("FormatData/Font");
|
|
if (xfont!=null)DefPlantFont = new VE_Font(xfont);
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
Console.WriteLine(ex.Message);
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
|
|
}
|
|
}
|