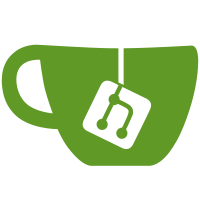
Fixed Find and Replace logic to keep it from getting into an infinite loop Used new CSLA code to hanndle deletion of procedures with external transitions New Enhanced Document properties
3056 lines
112 KiB
C#
3056 lines
112 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.ComponentModel;
|
|
using System.Data;
|
|
using System.Drawing;
|
|
using System.Text;
|
|
using System.Text.RegularExpressions;
|
|
using System.Windows.Forms;
|
|
using System.Runtime.InteropServices; // For DragHelper
|
|
using System.Reflection;
|
|
using Csla;
|
|
using Csla.Validation;
|
|
using VEPROMS.CSLA.Library;
|
|
using System.IO;
|
|
using Volian.Base.Library;
|
|
using DevComponents.DotNetBar;
|
|
|
|
namespace Volian.Controls.Library
|
|
{
|
|
#region DelegatesAndEventArgs
|
|
public delegate void vlnTreeViewEvent(object sender, vlnTreeEventArgs args);
|
|
public delegate void vlnTreeViewTimeEvent(object sender, vlnTreeTimeEventArgs args);
|
|
public delegate void vlnTreeViewStatusEvent(object sender, vlnTreeStatusEventArgs args);
|
|
public delegate ItemInfo vlnTreeViewClipboardStatusEvent(object sender, vlnTreeEventArgs args);
|
|
public delegate void vlnTreeViewItemInfoEvent(object sender, vlnTreeItemInfoEventArgs args);
|
|
public delegate bool vlnTreeViewBoolEvent(object sender, vlnTreeEventArgs args);
|
|
public delegate bool vlnTreeViewItemInfoDeleteEvent(object sender, vlnTreeItemInfoEventArgs args);
|
|
public delegate bool vlnTreeViewItemInfoInsertEvent(object sender, vlnTreeItemInfoInsertEventArgs args);
|
|
public delegate bool vlnTreeViewItemInfoPasteEvent(object sender, vlnTreeItemInfoPasteEventArgs args);
|
|
public delegate TreeNode vlnTreeViewTreeNodeEvent(object sender, vlnTreeEventArgs args);
|
|
public delegate DialogResult vlnTreeViewPropertyEvent(object sender, vlnTreePropertyEventArgs args);
|
|
public delegate DialogResult vlnTreeViewPSIEvent(object sender, vlnTreeEventArgs args);
|
|
public delegate DialogResult vlnTreeViewSIEvent(object sender, vlnTreeEventArgs args);
|
|
public delegate void vlnTreeViewSectionInfoEvent(object sender, vlnTreeSectionInfoEventArgs args);
|
|
public delegate void WordSectionDeletedEvent(object sender, WordSectionEventArgs args);
|
|
public delegate void vlnTreeViewPdfEvent(object sender, vlnTreeViewPdfArgs args);
|
|
public delegate string vlnTreeViewGetChangeIdEvent(object sender, vlnTreeItemInfoEventArgs args);
|
|
public partial class vlnTreeSectionInfoEventArgs
|
|
{
|
|
private bool _IsDeleting = false;
|
|
public bool IsDeleting
|
|
{
|
|
get { return _IsDeleting; }
|
|
set { _IsDeleting = value; }
|
|
}
|
|
private SectionInfo _MySectionInfo;
|
|
public SectionInfo MySectionInfo
|
|
{
|
|
get { return _MySectionInfo; }
|
|
set { _MySectionInfo = value; }
|
|
}
|
|
public vlnTreeSectionInfoEventArgs(SectionInfo mySectionInfo)
|
|
{
|
|
_MySectionInfo = mySectionInfo;
|
|
}
|
|
public vlnTreeSectionInfoEventArgs(SectionInfo mySectionInfo,bool isDeleting)
|
|
{
|
|
_MySectionInfo = mySectionInfo;
|
|
_IsDeleting = isDeleting;
|
|
}
|
|
}
|
|
public partial class vlnTreeViewPdfArgs
|
|
{
|
|
private string _MyFilename;
|
|
public string MyFilename
|
|
{
|
|
get { return _MyFilename; }
|
|
set { _MyFilename = value; }
|
|
}
|
|
private byte[] _MyBuffer;
|
|
public byte[] MyBuffer
|
|
{
|
|
get { return _MyBuffer; }
|
|
set { _MyBuffer = value; }
|
|
}
|
|
private string _MyWatermark;
|
|
public string MyWatermark
|
|
{
|
|
get { return _MyWatermark; }
|
|
set { _MyWatermark = value; }
|
|
}
|
|
public vlnTreeViewPdfArgs(string filename, byte[] buffer, string watermark)
|
|
{
|
|
_MyFilename = filename;
|
|
_MyBuffer = buffer;
|
|
_MyWatermark = watermark;
|
|
}
|
|
}
|
|
public partial class vlnTreeTimeEventArgs
|
|
{
|
|
private TimeSpan _myTimeSpan;
|
|
public TimeSpan MyTimeSpan
|
|
{
|
|
get { return _myTimeSpan; }
|
|
set { _myTimeSpan = value; }
|
|
}
|
|
private string _MyMessage;
|
|
public string MyMessage
|
|
{
|
|
get { return _MyMessage; }
|
|
set { _MyMessage = value; }
|
|
}
|
|
public vlnTreeTimeEventArgs(DateTime dtStart, string message)
|
|
{
|
|
MyTimeSpan = TimeSpan.FromTicks(DateTime.Now.Ticks - dtStart.Ticks);
|
|
MyMessage = message;
|
|
}
|
|
}
|
|
public partial class vlnTreeStatusEventArgs
|
|
{
|
|
private bool _MyStatus;
|
|
public bool MyStatus
|
|
{
|
|
get { return _MyStatus; }
|
|
set { _MyStatus = value; }
|
|
}
|
|
private string _MyMessage;
|
|
public string MyMessage
|
|
{
|
|
get { return _MyMessage; }
|
|
set { _MyMessage = value; }
|
|
}
|
|
|
|
public vlnTreeStatusEventArgs(bool status, string message)
|
|
{
|
|
MyStatus = status;
|
|
MyMessage = message;
|
|
}
|
|
}
|
|
public partial class vlnTreeEventArgs
|
|
{
|
|
#region Business Methods
|
|
private TreeNode _Node;
|
|
public TreeNode Node
|
|
{
|
|
get { return _Node; }
|
|
set { _Node = value; }
|
|
}
|
|
private TreeNode _Destination=null;
|
|
public TreeNode Destination
|
|
{
|
|
get { return _Destination; }
|
|
set { _Destination = value; }
|
|
}
|
|
private int _Index;
|
|
public int Index
|
|
{
|
|
get { return _Index; }
|
|
set { _Index = value; }
|
|
}
|
|
//jcb multiunit
|
|
private string _Unit;
|
|
public string Unit
|
|
{
|
|
get { return _Unit; }
|
|
set { _Unit = value; }
|
|
}
|
|
private int _UnitIndex = -1;
|
|
public int UnitIndex
|
|
{
|
|
get { return _UnitIndex; }
|
|
set { _UnitIndex = value; }
|
|
}
|
|
//end jcb multiunit
|
|
#endregion
|
|
#region Factory Methods
|
|
private vlnTreeEventArgs() { ;}
|
|
public vlnTreeEventArgs(TreeNode node)
|
|
{
|
|
_Node = node;
|
|
}
|
|
public vlnTreeEventArgs(TreeNode node, TreeNode destination, int index)
|
|
{
|
|
_Node = node;
|
|
_Destination = destination;
|
|
_Index = index;
|
|
}
|
|
//jcb multiunit
|
|
public vlnTreeEventArgs(TreeNode node, TreeNode destination, int index, string unit, int unitIndex)
|
|
{
|
|
_Node = node;
|
|
_Destination = destination;
|
|
_Index = index;
|
|
_Unit = unit;
|
|
_UnitIndex = unitIndex;
|
|
}
|
|
//end jcb multiunit
|
|
#endregion
|
|
public override string ToString()
|
|
{
|
|
return string.Format("Node={0},Destination={1},Index={2},Unit={3},UnitIndex={4}",NodePath(this.Node),this.Destination, this.Index,this.Unit, this.UnitIndex);
|
|
}
|
|
|
|
private string NodePath(TreeNode node)
|
|
{
|
|
string retval = "";
|
|
if (node.Parent != null)
|
|
retval = NodePath(node.Parent) + ":";
|
|
retval += node.Text;
|
|
return retval;
|
|
}
|
|
}
|
|
public partial class vlnTreeItemInfoEventArgs
|
|
{
|
|
#region Business Methods
|
|
private ItemInfo _MyItemInfo;
|
|
public ItemInfo MyItemInfo
|
|
{
|
|
get { return _MyItemInfo; }
|
|
set { _MyItemInfo = value; }
|
|
}
|
|
#endregion
|
|
#region Factory Methods
|
|
private vlnTreeItemInfoEventArgs() { ;}
|
|
public vlnTreeItemInfoEventArgs(ItemInfo myItemInfo)
|
|
{
|
|
_MyItemInfo = myItemInfo;
|
|
}
|
|
#endregion
|
|
}
|
|
public enum E_InsertType {Before, After, Child};
|
|
public partial class vlnTreeItemInfoInsertEventArgs
|
|
{
|
|
#region Business Methods
|
|
private ItemInfo _MyItemInfo;
|
|
public ItemInfo MyItemInfo
|
|
{
|
|
get { return _MyItemInfo; }
|
|
set { _MyItemInfo = value; }
|
|
}
|
|
private E_InsertType _InsertType;
|
|
public E_InsertType InsertType
|
|
{
|
|
get { return _InsertType; }
|
|
set { _InsertType = value; }
|
|
}
|
|
private int _Type;
|
|
public int Type
|
|
{
|
|
get { return _Type; }
|
|
set { _Type = value; }
|
|
}
|
|
private E_FromType _FromType;
|
|
public E_FromType FromType
|
|
{
|
|
get { return _FromType; }
|
|
set { _FromType = value; }
|
|
}
|
|
private string _StepText;
|
|
public string StepText
|
|
{
|
|
get { return _StepText; }
|
|
set { _StepText = value; }
|
|
}
|
|
#endregion
|
|
#region Factory Methods
|
|
private vlnTreeItemInfoInsertEventArgs() { ;}
|
|
public vlnTreeItemInfoInsertEventArgs(ItemInfo myItemInfo, E_InsertType insertType, string stepText)
|
|
{
|
|
_MyItemInfo = myItemInfo;
|
|
_InsertType = insertType;
|
|
_StepText = stepText;
|
|
}
|
|
public vlnTreeItemInfoInsertEventArgs(ItemInfo myItemInfo, E_InsertType insertType, string stepText, int type, E_FromType fromType)
|
|
{
|
|
_MyItemInfo = myItemInfo;
|
|
_InsertType = insertType;
|
|
_StepText = stepText;
|
|
_Type = type;
|
|
_FromType = fromType;
|
|
}
|
|
#endregion
|
|
}
|
|
#region PasteEventArgs
|
|
public partial class vlnTreeItemInfoPasteEventArgs
|
|
{
|
|
#region Business Methods
|
|
private ItemInfo _MyItemInfo;
|
|
public ItemInfo MyItemInfo
|
|
{
|
|
get { return _MyItemInfo; }
|
|
set { _MyItemInfo = value; }
|
|
}
|
|
private int _CopyStartID;
|
|
public int CopyStartID
|
|
{
|
|
get { return _CopyStartID; }
|
|
set { _CopyStartID = value; }
|
|
}
|
|
private ItemInfo.EAddpingPart _PasteType;
|
|
public ItemInfo.EAddpingPart PasteType
|
|
{
|
|
get { return _PasteType; }
|
|
set { _PasteType = value; }
|
|
}
|
|
private int? _Type;
|
|
public int? Type
|
|
{
|
|
get { return _Type; }
|
|
set { _Type = value; }
|
|
}
|
|
#endregion
|
|
#region Factory Methods
|
|
private vlnTreeItemInfoPasteEventArgs() { ;}
|
|
public vlnTreeItemInfoPasteEventArgs(ItemInfo myItemInfo, int copyStartId, ItemInfo.EAddpingPart pasteType, int? type)
|
|
{
|
|
_MyItemInfo = myItemInfo;
|
|
_CopyStartID = copyStartId;
|
|
_PasteType = pasteType;
|
|
_Type = type;
|
|
}
|
|
|
|
#endregion
|
|
}
|
|
#endregion
|
|
public partial class vlnTreePropertyEventArgs : EventArgs
|
|
{
|
|
private string _Title;
|
|
public string Title
|
|
{
|
|
get { return _Title; }
|
|
set { _Title = value; }
|
|
}
|
|
private object _ConfigObject;
|
|
public object ConfigObject
|
|
{
|
|
get { return _ConfigObject; }
|
|
set { _ConfigObject = value; }
|
|
}
|
|
private FolderConfig _FolderConfig;
|
|
public FolderConfig FolderConfig
|
|
{
|
|
get { return _FolderConfig; }
|
|
set { _ConfigObject = _FolderConfig = value; }
|
|
}
|
|
private DocVersionConfig _DocVersionConfig;
|
|
public DocVersionConfig DocVersionConfig
|
|
{
|
|
get { return _DocVersionConfig; }
|
|
set { _ConfigObject = _DocVersionConfig = value; }
|
|
}
|
|
private ProcedureConfig _ProcedureConfig;
|
|
public ProcedureConfig ProcedureConfig
|
|
{
|
|
get { return _ProcedureConfig; }
|
|
set { _ConfigObject = _ProcedureConfig = value; }
|
|
}
|
|
private SectionConfig _SectionConfig;
|
|
public SectionConfig SectionConfig
|
|
{
|
|
get { return _SectionConfig; }
|
|
set { _ConfigObject = _SectionConfig = value; }
|
|
}
|
|
private vlnTreePropertyEventArgs() { ;}
|
|
public vlnTreePropertyEventArgs(string title, FolderConfig folderConfig)
|
|
{
|
|
_Title = title;
|
|
FolderConfig = folderConfig;
|
|
}
|
|
public vlnTreePropertyEventArgs(string title, DocVersionConfig docVersionConfig)
|
|
{
|
|
_Title = title;
|
|
DocVersionConfig = docVersionConfig;
|
|
}
|
|
public vlnTreePropertyEventArgs(string title, ProcedureConfig procedureConfig)
|
|
{
|
|
_Title = title;
|
|
ProcedureConfig = procedureConfig;
|
|
}
|
|
public vlnTreePropertyEventArgs(string title, SectionConfig sectionConfig)
|
|
{
|
|
_Title = title;
|
|
DocStyleListConverter.MySection = sectionConfig.MySection;
|
|
SectionConfig = sectionConfig;
|
|
}
|
|
}
|
|
#endregion
|
|
public partial class vlnTreeView : TreeView
|
|
{
|
|
private SessionInfo _MySessionInfo;
|
|
public SessionInfo MySessionInfo
|
|
{
|
|
get { return _MySessionInfo; }
|
|
set { _MySessionInfo = value; }
|
|
}
|
|
#region Local Vars
|
|
private static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
|
|
FolderInfo _LastFolderInfo = null;
|
|
ProcedureInfo _LastProcedureInfo = null;
|
|
DocVersionInfo _LastDocVersionInfo = null;
|
|
SectionInfo _LastSectionInfo = null;
|
|
VETreeNode _LastTreeNode = null;
|
|
StepInfo _LastStepInfo = null;
|
|
ItemInfo _LastItemInfo = null;
|
|
#endregion
|
|
#region Events
|
|
public event vlnTreeViewGetChangeIdEvent GetChangeId;
|
|
private string OnGetChangeId(object sender, vlnTreeItemInfoEventArgs args)
|
|
{
|
|
if (GetChangeId != null) return GetChangeId(sender, args);
|
|
return null;
|
|
}
|
|
public event vlnTreeViewPdfEvent ViewPDF;
|
|
private void OnViewPDF(object sender, vlnTreeViewPdfArgs args)
|
|
{
|
|
if (ViewPDF != null) ViewPDF(sender, args);
|
|
}
|
|
public event vlnTreeViewItemInfoDeleteEvent DeleteItemInfo;
|
|
private bool OnDeleteItemInfo(object sender, vlnTreeItemInfoEventArgs args)
|
|
{
|
|
if (DeleteItemInfo != null) return DeleteItemInfo(sender, args);
|
|
return false;
|
|
}
|
|
public event vlnTreeViewItemInfoInsertEvent InsertItemInfo;
|
|
private bool OnInsertItemInfo(object sender, vlnTreeItemInfoInsertEventArgs args)
|
|
{
|
|
if (InsertItemInfo != null) return InsertItemInfo(sender, args);
|
|
return false;
|
|
}
|
|
public event vlnTreeViewItemInfoPasteEvent PasteItemInfo;
|
|
private bool OnPasteItemInfo(object sender, vlnTreeItemInfoPasteEventArgs args)
|
|
{
|
|
if (PasteItemInfo != null) return PasteItemInfo(sender, args);
|
|
return false;
|
|
}
|
|
public event vlnTreeViewEvent NodeMove;
|
|
private void OnNodeMove(object sender, vlnTreeEventArgs args)
|
|
{
|
|
if (NodeMove != null) NodeMove(sender, args);
|
|
}
|
|
public event vlnTreeViewClipboardStatusEvent ClipboardStatus;
|
|
private ItemInfo OnClipboardStatus(object sender, vlnTreeEventArgs args)
|
|
{
|
|
ItemInfo rv = null;
|
|
if (ClipboardStatus != null) rv = ClipboardStatus(sender, args);
|
|
return rv;
|
|
}
|
|
public event vlnTreeViewEvent NodeCopy;
|
|
private void OnNodeCopy(object sender, vlnTreeEventArgs args)
|
|
{
|
|
if (NodeCopy != null) NodeCopy(sender, args);
|
|
}
|
|
public event vlnTreeViewPropertyEvent NodeOpenProperty;
|
|
private DialogResult OnNodeOpenProperty(object sender, vlnTreePropertyEventArgs args)
|
|
{
|
|
if (NodeOpenProperty != null) return NodeOpenProperty(sender, args);
|
|
return DialogResult.Cancel;
|
|
}
|
|
public event vlnTreeViewPSIEvent NodePSI;
|
|
private DialogResult OnNodePSI(object sender, vlnTreeEventArgs args)
|
|
{
|
|
if (NodePSI != null) return NodePSI(sender, args);
|
|
return DialogResult.Cancel;
|
|
}
|
|
public event vlnTreeViewSIEvent NodeSI;
|
|
private DialogResult OnNodeSI(object sender, vlnTreeEventArgs args)
|
|
{
|
|
if (NodeSI != null) return NodeSI(sender, args);
|
|
return DialogResult.Cancel;
|
|
}
|
|
public event vlnTreeViewEvent NodeSelect;
|
|
private void OnNodeSelect(object sender, vlnTreeEventArgs args)
|
|
{
|
|
if (NodeSelect != null) NodeSelect(sender, args);
|
|
}
|
|
public event vlnTreeViewEvent CreateContinuousActionSummary;
|
|
private void OnCreateContinuousActionSummary(object sender, vlnTreeEventArgs args)
|
|
{
|
|
if (CreateContinuousActionSummary != null) CreateContinuousActionSummary(sender, args);
|
|
}
|
|
public event vlnTreeViewEvent PrintProcedure;
|
|
private void OnPrintProcedure(object sender, vlnTreeEventArgs args)
|
|
{
|
|
if (PrintProcedure != null) PrintProcedure(sender, args);
|
|
}
|
|
public event vlnTreeViewEvent PrintAllProcedures;
|
|
private void OnPrintAllProcedures(object sender, vlnTreeEventArgs args)
|
|
{
|
|
if (PrintAllProcedures != null) PrintAllProcedures(sender, args);
|
|
}
|
|
public event vlnTreeViewEvent ApproveProcedure;
|
|
private void OnApproveProcedure(object sender, vlnTreeEventArgs args)
|
|
{
|
|
if (ApproveProcedure != null) ApproveProcedure(sender, args);
|
|
}
|
|
public event vlnTreeViewEvent ApproveAllProcedures;
|
|
private void OnApproveAllProcedures(object sender, vlnTreeEventArgs args)
|
|
{
|
|
if (ApproveAllProcedures != null) ApproveAllProcedures(sender, args);
|
|
}
|
|
public event vlnTreeViewEvent ApproveSomeProcedures;
|
|
private void OnApproveSomeProcedures(object sender, vlnTreeEventArgs args)
|
|
{
|
|
if (ApproveSomeProcedures != null) ApproveSomeProcedures(sender, args);
|
|
}
|
|
public event vlnTreeViewEvent ReportAllProceduresInconsistencies;
|
|
private void OnReportAllProceduresInconsistencies(object sender, vlnTreeEventArgs args)
|
|
{
|
|
if (ReportAllProceduresInconsistencies != null) ReportAllProceduresInconsistencies(sender, args);
|
|
}
|
|
public event vlnTreeViewEvent RefreshCheckedOutProcedures;
|
|
private void OnRefreshCheckedOutProcedures(object sender, vlnTreeEventArgs args)
|
|
{
|
|
if (RefreshCheckedOutProcedures != null) RefreshCheckedOutProcedures(sender, args);
|
|
}
|
|
public event vlnTreeViewEvent ProcedureCheckedOutTo;
|
|
private void OnProcedureCheckedOutTo(object sender, vlnTreeEventArgs args)
|
|
{
|
|
if (ProcedureCheckedOutTo != null) ProcedureCheckedOutTo(sender, args);
|
|
}
|
|
public event vlnTreeViewEvent ExportImportProcedureSets;
|
|
private void OnExportImportProcedureSets(object sender, vlnTreeEventArgs args)
|
|
{
|
|
if (ExportImportProcedureSets != null) ExportImportProcedureSets(sender, args);
|
|
}
|
|
public event vlnTreeViewEvent PrintTransitionReport;
|
|
private void OnPrintTransitionReport(object sender, vlnTreeEventArgs args)
|
|
{
|
|
if (PrintTransitionReport != null) PrintTransitionReport(sender, args);
|
|
}
|
|
public event vlnTreeViewEvent NodeNew;
|
|
private void OnNodeNew(object sender, vlnTreeEventArgs args)
|
|
{
|
|
if (NodeNew != null) NodeNew(sender, args);
|
|
}
|
|
/// <summary>
|
|
/// Raised after a new step is added.
|
|
/// </summary>
|
|
public event vlnTreeViewEvent NodeInsert;
|
|
private void OnNodeInsert(object sender, vlnTreeEventArgs args)
|
|
{
|
|
if (NodeInsert != null) NodeInsert(sender, args);
|
|
}
|
|
public event vlnTreeViewEvent NodeSelectionChange;
|
|
private void OnNodeSelectionChange(object sender, vlnTreeEventArgs args)
|
|
{
|
|
if (NodeSelectionChange != null) NodeSelectionChange(sender, args);
|
|
}
|
|
public event vlnTreeViewSectionInfoEvent SectionShouldClose;
|
|
private void OnSectionShouldClose(object sender, vlnTreeSectionInfoEventArgs args)
|
|
{
|
|
if (SectionShouldClose != null) SectionShouldClose(sender, args);
|
|
}
|
|
public event WordSectionDeletedEvent WordSectionDeleted;
|
|
internal void OnWordSectionDeleted(object sender, WordSectionEventArgs args)
|
|
{
|
|
WordSectionDeleted(sender, args);
|
|
}
|
|
public event vlnTreeViewItemInfoEvent OpenItem;
|
|
private void OnOpenItem(object sender, vlnTreeItemInfoEventArgs args)
|
|
{
|
|
if (OpenItem != null) OpenItem(sender, args);
|
|
}
|
|
#endregion
|
|
#region Constructors
|
|
public vlnTreeView()
|
|
{
|
|
InitializeComponent();
|
|
this.AllowDrop = true;
|
|
DragHelper.InitCommonControls();
|
|
this.ItemDrag += new ItemDragEventHandler(tv_ItemDrag);
|
|
this.DragDrop += new DragEventHandler(tv_DragDrop);
|
|
this.DragEnter += new DragEventHandler(tv_DragEnter);
|
|
this.DragLeave += new EventHandler(tv_DragLeave);
|
|
this.DragOver += new DragEventHandler(tv_DragOver);
|
|
this.MouseDown += new MouseEventHandler(tv_MouseDown);
|
|
this.KeyPress += new KeyPressEventHandler(tv_KeyPress);
|
|
base.AfterSelect += new TreeViewEventHandler(tv_AfterSelect);
|
|
}
|
|
#endregion
|
|
#region MenuSupport
|
|
private void tv_AfterSelect(object sender, TreeViewEventArgs e)
|
|
{
|
|
if (!_AdjustingTree)
|
|
OnNodeSelectionChange(sender, new vlnTreeEventArgs(e.Node));
|
|
}
|
|
// use to determine which menu items have been selected for those tree nodes
|
|
|
|
// that allow more than one type of operation associated with their selection.
|
|
public enum MenuSelections : int
|
|
{
|
|
Folder = 1, FolderBefore = 2, FolderAfter = 3, DocVersion = 4, Procedure = 5, ProcedureBefore = 6, ProcedureAfter = 7, Section = 8, SectionBefore = 9, SectionAfter = 10, Step = 11, StepBefore = 12, StepAfter = 13, StepReplace = 14
|
|
}
|
|
void tv_MouseDown(object sender, MouseEventArgs e)
|
|
{
|
|
if (e.Button == MouseButtons.Right)
|
|
{
|
|
OwnerInfoList oil = null;
|
|
OwnerInfo oi = null;
|
|
VETreeNode tn = this.GetNodeAt(new Point(e.X, e.Y)) as VETreeNode;
|
|
if (tn != null)
|
|
{
|
|
this.SelectedNode = tn as TreeNode;
|
|
Application.DoEvents();
|
|
// Display Menu
|
|
ToolStripMenuItem mi = new ToolStripMenuItem();
|
|
ContextMenu cm = new ContextMenu();
|
|
UserInfo ui = UserInfo.GetByUserID(VlnSettings.UserID);
|
|
if (ui == null)
|
|
{
|
|
MessageBox.Show("Security has not been defined for PROMS. All functionality has been defaulted to the lowest level for all users until security is defined.", "no security defined", MessageBoxButtons.OK, MessageBoxIcon.Warning);
|
|
return;
|
|
}
|
|
this.Cursor = Cursors.WaitCursor;
|
|
#region Menu_New
|
|
if (tn.VEObject as FolderInfo != null)
|
|
{
|
|
// For Folders, if no children, can add either docversion or folder. If children are
|
|
// folders then can only add another folder, and if children are docversions can only
|
|
// add docversion.
|
|
FolderInfo fi = tn.VEObject as FolderInfo;
|
|
bool DoSpecificInfo = fi.ActiveFormat.PlantFormat.FormatData.SpecificInfo;
|
|
if (ui.IsAdministrator() || ui.IsSetAdministrator(fi))// && fi.MyParent == null) //VEPROMS level
|
|
{
|
|
if(fi.HasWorkingDraft)
|
|
cm.MenuItems.Add("Export Procedure Set", new EventHandler(mi_Click));
|
|
else
|
|
cm.MenuItems.Add("Import Procedure Set", new EventHandler(mi_Click));
|
|
if (DoSpecificInfo)
|
|
cm.MenuItems.Add("Folder Specific Information", new EventHandler(mi_Click));
|
|
}
|
|
if (ui.IsAdministrator() || ui.IsSetAdministrator(fi))
|
|
{
|
|
if (fi.MyParent != null) // don't allow insert before/after if at top node
|
|
{
|
|
if (!ui.IsAdministrator() && DoSpecificInfo) cm.MenuItems.Add("Folder Specific Information", new EventHandler(mi_Click));
|
|
cm.MenuItems.Add("Insert Folder Before", new EventHandler(mi_Click));
|
|
cm.MenuItems.Add("Insert Folder After", new EventHandler(mi_Click));
|
|
}
|
|
if (fi.FolderDocVersionCount == 0) cm.MenuItems.Add("New Folder", new EventHandler(mi_Click));
|
|
if (fi.ChildFolderCount == 0 && !fi.HasWorkingDraft) cm.MenuItems.Add("Create Working Draft", new EventHandler(mi_Click));
|
|
}
|
|
if (fi.HasWorkingDraft)
|
|
cm.MenuItems.Add("Print Transition Report", new EventHandler(mi_Click));
|
|
}
|
|
else if (tn.VEObject as DocVersionInfo != null) // DocVersions can only contain procs
|
|
{
|
|
DocVersionInfo dvi = tn.VEObject as DocVersionInfo;
|
|
if (ui.IsAdministrator() || ui.IsSetAdministrator(dvi))
|
|
{
|
|
cm.MenuItems.Add("Import Procedure", mi_Click);
|
|
}
|
|
if (ui.IsAdministrator() || ui.IsSetAdministrator(dvi) || ui.IsWriter(dvi))
|
|
{
|
|
OwnerInfoList.Reset();
|
|
oil = OwnerInfoList.GetByVersionID(dvi.VersionID);
|
|
if (dvi.ActiveFormat.PlantFormat.FormatData.SpecificInfo)
|
|
cm.MenuItems.Add("Working Draft Specific Information", new EventHandler(mi_Click));
|
|
cm.MenuItems.Add("Refresh Checked Out Procedures", new EventHandler(mi_Click));
|
|
cm.MenuItems.Add("New Procedure", new EventHandler(mi_Click));
|
|
if (dvi.MultiUnitCount > 1)
|
|
{
|
|
MenuItem mip = new MenuItem("Print All Procedures for");
|
|
MenuItem mia = new MenuItem("Approve All Procedures for");
|
|
MenuItem mis = new MenuItem("Approve Some Procedures for");
|
|
int k = 0;
|
|
foreach (string s in dvi.UnitNames)
|
|
{
|
|
k++;
|
|
MenuItem mp = mip.MenuItems.Add(s, new EventHandler(miMultiUnit_Click));
|
|
mp.Tag = k;
|
|
MenuItem ma = mia.MenuItems.Add(s, new EventHandler(miMultiUnit_Click));
|
|
ma.Tag = k;
|
|
MenuItem ms = mis.MenuItems.Add(s, new EventHandler(miMultiUnit_Click));
|
|
ms.Tag = k;
|
|
}
|
|
//MenuItem mmp = mip.MenuItems.Add("All Units", new EventHandler(miMultiUnit_Click));
|
|
//mmp.Tag = 0;
|
|
//MenuItem mma = mia.MenuItems.Add("All Units", new EventHandler(miMultiUnit_Click));
|
|
//mma.Tag = 0;
|
|
//MenuItem mms = mis.MenuItems.Add("All Units", new EventHandler(miMultiUnit_Click));
|
|
//mms.Tag = 0;
|
|
cm.MenuItems.Add(mip);
|
|
cm.MenuItems.Add(mia);
|
|
cm.MenuItems.Add(mis);
|
|
}
|
|
else
|
|
{
|
|
cm.MenuItems.Add("Print All Procedures", new EventHandler(mi_Click));
|
|
cm.MenuItems.Add("Approve All Procedures", new EventHandler(mi_Click));
|
|
cm.MenuItems.Add("Approve Some Procedures", new EventHandler(mi_Click));
|
|
}
|
|
cm.MenuItems.Add("Report All Procedures Inconsistencies", new EventHandler(mi_Click));
|
|
}
|
|
else
|
|
{
|
|
OwnerInfoList.Reset();
|
|
oil = OwnerInfoList.GetByVersionID(dvi.VersionID);
|
|
cm.MenuItems.Add("Refresh Checked Out Procedures", new EventHandler(mi_Click));
|
|
if (dvi.MultiUnitCount > 1)
|
|
{
|
|
MenuItem mip = new MenuItem("Print All Procedures for");
|
|
int k = 0;
|
|
foreach (string s in dvi.UnitNames)
|
|
{
|
|
k++;
|
|
MenuItem mp = mip.MenuItems.Add(s, new EventHandler(miMultiUnit_Click));
|
|
mp.Tag = k;
|
|
}
|
|
//MenuItem mmp = mip.MenuItems.Add("All Units", new EventHandler(miMultiUnit_Click));
|
|
//mmp.Tag = 0;
|
|
//MenuItem mma = mia.MenuItems.Add("All Units", new EventHandler(miMultiUnit_Click));
|
|
//mma.Tag = 0;
|
|
//MenuItem mms = mis.MenuItems.Add("All Units", new EventHandler(miMultiUnit_Click));
|
|
//mms.Tag = 0;
|
|
cm.MenuItems.Add(mip);
|
|
}
|
|
else
|
|
{
|
|
cm.MenuItems.Add("Print All Procedures", new EventHandler(mi_Click));
|
|
}
|
|
}
|
|
//if (ui.IsAdministrator() || ui.IsSetAdministrator(dvi))
|
|
//{
|
|
// cm.MenuItems.Add("Check Out Procedure Set", new EventHandler(mi_Click));
|
|
// cm.MenuItems.Add("Check In Procedure Set", new EventHandler(mi_Click));
|
|
//}
|
|
if (ui.IsAdministrator() || ui.IsSetAdministrator(dvi) || ui.IsROEditor(dvi))
|
|
{
|
|
cm.MenuItems.Add("Run RO Editor", new EventHandler(mi_Click));
|
|
}
|
|
if (ui.IsAdministrator() || ui.IsSetAdministrator(dvi))
|
|
{
|
|
MenuItem urv = cm.MenuItems.Add("Update RO Values", new EventHandler(mi_Click));
|
|
urv.Enabled = dvi.NewerRoFst;
|
|
}
|
|
}
|
|
else if (tn.VEObject as ProcedureInfo != null) // Procs can only contain sections
|
|
{
|
|
ProcedureInfo pri = tn.VEObject as ProcedureInfo;
|
|
oi = OwnerInfo.GetByItemID(pri.ItemID, CheckOutType.Procedure);
|
|
if (ui.IsAdministrator() || ui.IsSetAdministrator(pri.MyDocVersion))
|
|
{
|
|
cm.MenuItems.Add("Export Procedure", mi_Click);
|
|
}
|
|
if (ui.IsAdministrator() || ui.IsSetAdministrator(pri.MyDocVersion) || ui.IsWriter(pri.MyDocVersion))
|
|
{
|
|
if (oi != null && oi.SessionID != MySessionInfo.SessionID)
|
|
cm.MenuItems.Add(string.Format("Procedure Checked Out to {0}", oi.SessionUserID), new EventHandler(mi_Click));
|
|
if (pri.ActiveFormat.PlantFormat.FormatData.ProcData.PSI.Caption != null) cm.MenuItems.Add("Procedure Specific Information", new EventHandler(mi_Click));
|
|
cm.MenuItems.Add("Insert Procedure Before", new EventHandler(mi_Click));
|
|
cm.MenuItems.Add("Insert Procedure After", new EventHandler(mi_Click));
|
|
|
|
// if this format has change ids that were added from edit, allow removal of
|
|
// change ids for this procedure:
|
|
//FolderInfo fi = FolderInfo.Get(1);
|
|
//FormatInfo frmI = FormatInfo.Get(fi.FormatID ?? 1);
|
|
//if (frmI.PlantFormat.FormatData.ProcData.ChangeBarData.ChgBarMessageFromEdit)
|
|
// cm.MenuItems.Add("Remove Change Ids", new EventHandler(mi_Click));
|
|
cm.MenuItems.Add("New Section", new EventHandler(mi_Click));
|
|
if (pri.MyDocVersion.MultiUnitCount > 1)
|
|
{
|
|
MenuItem micas = new MenuItem("Create Continuous Action Summary");
|
|
MenuItem mip = new MenuItem("Print");
|
|
MenuItem mia = new MenuItem("Approve");
|
|
int k = 0;
|
|
foreach (string s in pri.MyDocVersion.UnitNames)
|
|
{
|
|
k++;
|
|
MenuItem mp = mip.MenuItems.Add(s, new EventHandler(miMultiUnit_Click));
|
|
mp.Tag = k;
|
|
MenuItem ma = mia.MenuItems.Add(s, new EventHandler(miMultiUnit_Click));
|
|
ma.Tag = k;
|
|
MenuItem mc = micas.MenuItems.Add(s, new EventHandler(miMultiUnit_Click));
|
|
mc.Tag = k;
|
|
}
|
|
cm.MenuItems.Add(micas);
|
|
cm.MenuItems.Add(mip);
|
|
cm.MenuItems.Add(mia);
|
|
AddApprovedRevisionsMultiUnit(cm.MenuItems, pri);
|
|
}
|
|
else
|
|
{
|
|
cm.MenuItems.Add("Create Continuous Action Summary", new EventHandler(mi_Click));
|
|
cm.MenuItems.Add("Print", new EventHandler(mi_Click));
|
|
cm.MenuItems.Add("Approve", new EventHandler(mi_Click));
|
|
AddApprovedRevisions(cm.MenuItems, pri);
|
|
}
|
|
}
|
|
else
|
|
{
|
|
if (oi != null && oi.SessionID != MySessionInfo.SessionID)
|
|
cm.MenuItems.Add(string.Format("Procedure Checked Out to {0}", oi.SessionUserID), new EventHandler(mi_Click));
|
|
if (pri.MyDocVersion.MultiUnitCount > 1)
|
|
{
|
|
MenuItem mip = new MenuItem("Print");
|
|
int k = 0;
|
|
foreach (string s in pri.MyDocVersion.UnitNames)
|
|
{
|
|
k++;
|
|
MenuItem mp = mip.MenuItems.Add(s, new EventHandler(miMultiUnit_Click));
|
|
mp.Tag = k;
|
|
}
|
|
cm.MenuItems.Add(mip);
|
|
AddApprovedRevisionsMultiUnit(cm.MenuItems, pri);
|
|
}
|
|
else
|
|
{
|
|
cm.MenuItems.Add("Print", new EventHandler(mi_Click));
|
|
AddApprovedRevisions(cm.MenuItems, pri);
|
|
}
|
|
}
|
|
cm.MenuItems.Add("Print Transition Report", new EventHandler(mi_Click));
|
|
}
|
|
else if (tn.VEObject as SectionInfo != null)
|
|
{
|
|
// A step Section can contain other steps or can contain subsections (either step section
|
|
// or word doc section). Also note that there can be a mix.
|
|
// A word doc section can contain another subsection (either step section or word doc section),
|
|
// but cannot contain steps.
|
|
SectionInfo si = tn.VEObject as SectionInfo;
|
|
if (ui.IsAdministrator() || ui.IsSetAdministrator(si.MyProcedure.MyDocVersion) || ui.IsWriter(si.MyProcedure.MyDocVersion))
|
|
{
|
|
if (si.HasWordContent)
|
|
{
|
|
oi = OwnerInfo.GetByItemID(si.MyContent.MyEntry.DocID, CheckOutType.Document);
|
|
}
|
|
si.MyConfig = null;
|
|
// Do not need step versus Word doc options, user enters this in property page during
|
|
// insert process.
|
|
if (oi != null && oi.SessionID != MySessionInfo.SessionID)
|
|
cm.MenuItems.Add(string.Format("Document Checked Out to {0}", oi.SessionUserID), new EventHandler(mi_Click));
|
|
cm.MenuItems.Add("Insert Section Before", new EventHandler(mi_Click));
|
|
cm.MenuItems.Add("Insert Section After", new EventHandler(mi_Click));
|
|
if (!si.IsAutoTOCSection)
|
|
{
|
|
bool meta = si.ActiveFormat.PlantFormat.FormatData.SectData.UseMetaSections;
|
|
if (meta) cm.MenuItems.Add("New Subsection", new EventHandler(mi_Click));
|
|
// if this section has subsections, then be sure that the 'editable' data config
|
|
// is set to allow new step creation.
|
|
if (si.IsStepSection)
|
|
{
|
|
SectionConfig sc = si.MyConfig as SectionConfig;
|
|
if (si.Sections == null || si.Sections.Count == 0 || (meta && sc != null && si.Sections != null && si.Sections.Count > 0 && sc.SubSection_Edit == "Y"))
|
|
cm.MenuItems.Add("New Step", new EventHandler(mi_Click));
|
|
}
|
|
}
|
|
}
|
|
}
|
|
else if (tn.VEObject as StepInfo != null)
|
|
{
|
|
// check the format for allowable inserts, and also,
|
|
ItemInfo i = tn.VEObject as ItemInfo;
|
|
if (ui.IsAdministrator() || ui.IsSetAdministrator(i.MyProcedure.MyDocVersion) || ui.IsWriter(i.MyProcedure.MyDocVersion))
|
|
{
|
|
//oi = OwnerInfo.GetByItemID(i.MyProcedure.ItemID);
|
|
if (AddToInsertMenu(i, 0)) cm.MenuItems.Add("Insert Step Before", new EventHandler(mi_Click));
|
|
if (AddToInsertMenu(i, 1)) cm.MenuItems.Add("Insert Step After", new EventHandler(mi_Click));
|
|
}
|
|
}
|
|
#endregion
|
|
#region Menu_Open
|
|
if (!tn.IsExpanded && tn.VEObject as SectionInfo != null)
|
|
{
|
|
SectionInfo si = tn.VEObject as SectionInfo;
|
|
if (si.IsStepSection) cm.MenuItems.Add("Open", new EventHandler(mi_Click));
|
|
}
|
|
else if (!tn.IsExpanded && tn.VEObject as StepInfo != null)
|
|
{
|
|
StepInfo stpi = tn.VEObject as StepInfo;
|
|
if (stpi.HasChildren) cm.MenuItems.Add("Open", new EventHandler(mi_Click));
|
|
}
|
|
else if (!tn.IsExpanded)
|
|
cm.MenuItems.Add("Open", new EventHandler(mi_Click));
|
|
else
|
|
cm.MenuItems.Add("Collapse Procedures", new EventHandler(mi_Click));
|
|
#endregion
|
|
#region Menu_CutCopy
|
|
// For initial release, copy is not available for folders or docversions
|
|
if (tn.VEObject as ItemInfo != null)
|
|
{
|
|
ItemInfo i = tn.VEObject as ItemInfo;
|
|
if (ui.IsAdministrator() || ui.IsSetAdministrator(i.MyProcedure.MyDocVersion) || ui.IsWriter(i.MyProcedure.MyDocVersion))
|
|
cm.MenuItems.Add("Copy", new EventHandler(mi_Click));
|
|
}
|
|
#endregion
|
|
bool ok = false;
|
|
if (tn.VEObject is FolderInfo && (ui.IsAdministrator() || ui.IsSetAdministrator(tn.VEObject as FolderInfo)))
|
|
ok = true;
|
|
else if (tn.VEObject is DocVersionInfo && (ui.IsAdministrator() || ui.IsSetAdministrator(tn.VEObject as DocVersionInfo)))
|
|
ok = true;
|
|
else if (tn.VEObject is ItemInfo && (ui.IsAdministrator() || ui.IsSetAdministrator((tn.VEObject as ItemInfo).MyProcedure.MyDocVersion) || ui.IsWriter((tn.VEObject as ItemInfo).MyProcedure.MyDocVersion)))
|
|
ok = true;
|
|
if(ok)
|
|
Menu_Paste(tn, cm);
|
|
#region Menu_Delete
|
|
if (ok)
|
|
{
|
|
// Add delete to the menu unless at the very 'top' node, on a grouping (partinfo)
|
|
// node (RNOs, Steps, Cautions, Notes) or Folder/DocVersion that contains any items.
|
|
PartInfo pi = tn.VEObject as PartInfo;
|
|
if (pi == null && tn.Parent != null) // it's not a part and it's not the top....
|
|
{
|
|
FolderInfo fi = tn.VEObject as FolderInfo;
|
|
if (fi == null || tn.Nodes.Count == 0) // it's not a folder or it has no children
|
|
{
|
|
DocVersionInfo di = tn.VEObject as DocVersionInfo;
|
|
if (di == null || tn.Nodes.Count == 0) // it's not a docversion or it has no children
|
|
cm.MenuItems.Add("Delete", new EventHandler(mi_Click));
|
|
}
|
|
}
|
|
}
|
|
#endregion
|
|
#region Menu_Properties
|
|
// Add Properties to the menu unless at the very 'top' node or on a grouping (partinfo)
|
|
// node (RNOs, Steps, Cautions, Notes) or at the step level.
|
|
ok = tn.VEObject as FolderInfo != null ? ui.IsAdministrator() : tn.VEObject as DocVersionInfo != null ? (ui.IsAdministrator() || ui.IsSetAdministrator(tn.VEObject as DocVersionInfo)) : (ui.IsAdministrator() || ((tn.VEObject as ItemInfo) != null && (ui.IsSetAdministrator((tn.VEObject as ItemInfo).MyProcedure.MyDocVersion) || ui.IsWriter((tn.VEObject as ItemInfo).MyProcedure.MyDocVersion))));
|
|
PartInfo pia = tn.VEObject as PartInfo;
|
|
ItemInfo ii = tn.VEObject as ItemInfo;
|
|
if (ok)
|
|
if (pia == null && tn.VEObject as StepInfo == null) cm.MenuItems.Add("Properties...", new EventHandler(mi_Click));
|
|
#endregion
|
|
if (oil != null && oil.Count > 0 && tn.VEObject as DocVersionInfo != null)
|
|
{
|
|
/*
|
|
--docversion level
|
|
Approve All Procedures
|
|
Report All Procedures Inconsistencies
|
|
*/
|
|
bool greyOut = false;
|
|
foreach (OwnerInfo own in oil)
|
|
{
|
|
if (own.SessionID != MySessionInfo.SessionID)
|
|
{
|
|
greyOut = true;
|
|
break;
|
|
}
|
|
}
|
|
if (greyOut)
|
|
{
|
|
foreach (MenuItem itm in cm.MenuItems)
|
|
{
|
|
if (itm.Text == "Approve All Procedures" || itm.Text == "Report All Procedures Inconsistencies")
|
|
itm.Enabled = false;
|
|
}
|
|
}
|
|
}
|
|
if (oi != null && oi.SessionID != MySessionInfo.SessionID)
|
|
{
|
|
/*
|
|
--procedure level
|
|
Procedure Specific Information
|
|
New Section
|
|
Approve
|
|
Open
|
|
Copy
|
|
Delete
|
|
Properties...
|
|
|
|
--section level
|
|
all of them
|
|
|
|
--step level
|
|
all of them
|
|
*/
|
|
if (tn.VEObject as ProcedureInfo != null)
|
|
{
|
|
foreach (MenuItem itm in cm.MenuItems)
|
|
{
|
|
if(itm.Text == "Procedure Specific Information" || itm.Text == "New Section" || itm.Text == "Approve" || itm.Text == "Open" ||
|
|
itm.Text == "Copy" || itm.Text == "Delete" || itm.Text == "Properties..." || itm.Text == "Replace Existing Procedure")
|
|
itm.Enabled = false;
|
|
}
|
|
}
|
|
if (tn.VEObject as SectionInfo != null || tn.VEObject as StepInfo != null)
|
|
{
|
|
foreach (MenuItem itm in cm.MenuItems)
|
|
{
|
|
if(!itm.Text.StartsWith("Document Checked Out"))
|
|
itm.Enabled = false;
|
|
}
|
|
}
|
|
}
|
|
this.Cursor = Cursors.Default;
|
|
cm.Show(this, new Point(e.X, e.Y));
|
|
}
|
|
}
|
|
}
|
|
|
|
private void AddApprovedRevisionsMultiUnit(Menu.MenuItemCollection menuItemCollection, ProcedureInfo pri)
|
|
{
|
|
RevisionInfoList ril = RevisionInfoList.GetByItemID(pri.ItemID);
|
|
if (ril.Count == 0) return;
|
|
MenuItem mi = menuItemCollection.Add("Versions");
|
|
int k = 0;
|
|
int lastApprovedRevisionID = 0;
|
|
foreach (string s in pri.MyDocVersion.UnitNames)
|
|
{
|
|
k++;
|
|
MenuItem mv = mi.MenuItems.Add(s);
|
|
mv.Tag = k;
|
|
ril = RevisionInfoList.GetByItemIDandUnitID(pri.ItemID, k);
|
|
foreach (RevisionInfo ri in ril)
|
|
{
|
|
MenuItem mir = mv.MenuItems.Add(ri.ToString());
|
|
mir.Tag = ri;
|
|
if (ri.LatestVersion.MyStage.IsApproved > 0 && (ri.RevisionID > lastApprovedRevisionID))
|
|
lastApprovedRevisionID = ri.RevisionID;
|
|
if (ri.LatestVersion.PDF != null)
|
|
{
|
|
MenuItem mirp = mir.MenuItems.Add("View Procedure");
|
|
mirp.Tag = ri;
|
|
mirp.Click += new EventHandler(MultiUnitApprovedRevision_Click);
|
|
}
|
|
if (ri.LatestVersion.SummaryPDF != null)
|
|
{
|
|
MenuItem mirs = mir.MenuItems.Add("View Summary of Changes");
|
|
mirs.Tag = ri;
|
|
mirs.Click += new EventHandler(MultiUnitSummaryOfChanges_Click);
|
|
}
|
|
//end added jcb 20111031
|
|
mv.Tag = lastApprovedRevisionID;
|
|
}
|
|
}
|
|
}
|
|
public void AddNewNode(IVEDrillDownReadOnly o)
|
|
{
|
|
VETreeNode tn = new VETreeNode(o);
|
|
SelectedNode.Nodes.Add(tn);
|
|
}
|
|
private void AddApprovedRevisions(Menu.MenuItemCollection menuItemCollection, ProcedureInfo pri)
|
|
{
|
|
RevisionInfoList ril = null;
|
|
try
|
|
{
|
|
ril = RevisionInfoList.GetByItemID(pri.ItemID);
|
|
}
|
|
catch
|
|
{
|
|
return;
|
|
}
|
|
if (ril.Count == 0) return;
|
|
MenuItem mi = menuItemCollection.Add("Versions");
|
|
int lastApprovedRevisionID = 0;
|
|
foreach (RevisionInfo ri in ril)
|
|
{
|
|
MenuItem mir = mi.MenuItems.Add(ri.ToString());
|
|
mir.Tag = ri;
|
|
bool addImportMI = false;
|
|
if (ri.LatestVersion.MyStage.IsApproved > 0 && (ri.RevisionID > lastApprovedRevisionID))
|
|
{
|
|
lastApprovedRevisionID = ri.RevisionID;
|
|
addImportMI = true;
|
|
}
|
|
//mir.Click += new EventHandler(ApprovedRevision_Click);
|
|
//added jcb 20111031
|
|
if (ri.LatestVersion.PDF != null)
|
|
{
|
|
MenuItem mirp = mir.MenuItems.Add("View Procedure");
|
|
mirp.Tag = ri;
|
|
mirp.Click += new EventHandler(ApprovedRevision_Click);
|
|
}
|
|
if (ri.LatestVersion.SummaryPDF != null)
|
|
{
|
|
MenuItem mirs = mir.MenuItems.Add("View Summary of Changes");
|
|
mirs.Tag = ri;
|
|
mirs.Click += new EventHandler(SummaryOfChanges_Click);
|
|
}
|
|
if (addImportMI && ri.LatestVersion.ApprovedXML != null)
|
|
{
|
|
MenuItem miri = mir.MenuItems.Add("Import Procedure");
|
|
miri.Tag = ri;
|
|
miri.Click += new EventHandler(ImportProcedure_Click);
|
|
}
|
|
//end added jcb 20111031
|
|
mi.Tag = lastApprovedRevisionID;
|
|
}
|
|
}
|
|
|
|
void ImportProcedure_Click(object sender, EventArgs e)
|
|
{
|
|
RevisionInfo ri = (sender as MenuItem).Tag as RevisionInfo;
|
|
System.Xml.XmlDocument xd = new System.Xml.XmlDocument();
|
|
xd.LoadXml(ri.LatestVersion.ApprovedXML);
|
|
MessageBox.Show(xd.SelectSingleNode("procedure/content/@number").InnerText);
|
|
}
|
|
|
|
void ApprovedRevision_Click(object sender, EventArgs e)
|
|
{
|
|
bool superceded = false;
|
|
MenuItem mi = sender as MenuItem;
|
|
if (mi == null) return;
|
|
RevisionInfo ri = mi.Tag as RevisionInfo;
|
|
{
|
|
MenuItem mip = mi.Parent as MenuItem;
|
|
if (ri.RevisionID < int.Parse(mip.Parent.Tag.ToString()))
|
|
superceded = true;
|
|
}
|
|
vlnTreeViewPdfArgs args = new vlnTreeViewPdfArgs(Volian.Base.Library.TmpFile.CreateFileName(ProcedureInfo.Get(ri.ItemID).PDFNumber), ri.LatestVersion.PDF, superceded ? "Superceded" : "");
|
|
OnViewPDF(sender, args);
|
|
// System.Diagnostics.Process pp = System.Diagnostics.Process.Start(GetDocPdf(ri, superceded));
|
|
}
|
|
void MultiUnitApprovedRevision_Click(object sender, EventArgs e)
|
|
{
|
|
bool superceded = false;
|
|
MenuItem mi = sender as MenuItem;
|
|
if (mi == null) return;
|
|
RevisionInfo ri = mi.Tag as RevisionInfo;
|
|
{
|
|
MenuItem mip = mi.Parent as MenuItem;
|
|
if (ri.RevisionID < int.Parse(mip.Parent.Tag.ToString()))
|
|
superceded = true;
|
|
}
|
|
ItemInfo ii = ItemInfo.Get(ri.ItemID);
|
|
ii.MyDocVersion.DocVersionConfig.SelectedSlave = ri.MyConfig.Applicability_Index;
|
|
vlnTreeViewPdfArgs args = new vlnTreeViewPdfArgs(Volian.Base.Library.TmpFile.CreateFileName(ProcedureInfo.Get(ri.ItemID).PDFNumber), ri.LatestVersion.PDF, superceded ? "Superceded" : "");
|
|
OnViewPDF(sender, args);
|
|
// System.Diagnostics.Process pp = System.Diagnostics.Process.Start(GetDocPdf(ri, superceded));
|
|
}
|
|
void SummaryOfChanges_Click(object sender, EventArgs e)
|
|
{
|
|
MenuItem mi = sender as MenuItem;
|
|
if (mi == null) return;
|
|
RevisionInfo ri = mi.Tag as RevisionInfo;
|
|
vlnTreeViewPdfArgs args = new vlnTreeViewPdfArgs(Volian.Base.Library.TmpFile.CreateFileName(ProcedureInfo.Get(ri.ItemID).PDFNumber + " Summary of Changes"), ri.LatestVersion.SummaryPDF, "");
|
|
OnViewPDF(sender, args);
|
|
// System.Diagnostics.Process pps = System.Diagnostics.Process.Start(GetDocSummaryPdf(ri));
|
|
}
|
|
void MultiUnitSummaryOfChanges_Click(object sender, EventArgs e)
|
|
{
|
|
MenuItem mi = sender as MenuItem;
|
|
if (mi == null) return;
|
|
RevisionInfo ri = mi.Tag as RevisionInfo;
|
|
ItemInfo ii = ItemInfo.Get(ri.ItemID);
|
|
ii.MyDocVersion.DocVersionConfig.SelectedSlave = ri.MyConfig.Applicability_Index;
|
|
vlnTreeViewPdfArgs args = new vlnTreeViewPdfArgs(Volian.Base.Library.TmpFile.CreateFileName(ProcedureInfo.Get(ri.ItemID).PDFNumber + " Summary of Changes"), ri.LatestVersion.SummaryPDF, "");
|
|
OnViewPDF(sender, args);
|
|
// System.Diagnostics.Process pps = System.Diagnostics.Process.Start(GetDocSummaryPdf(ri));
|
|
}
|
|
//private string GetDocPdf(RevisionInfo ri, bool superceded)
|
|
//{
|
|
// string fileName = Volian.Base.Library.TmpFile.CreateFileName(ItemInfo.Get(ri.ItemID).DisplayNumber);
|
|
// FileInfo fi = new FileInfo(VlnSettings.TemporaryFolder + @"\" + fileName);
|
|
// FileStream fs = fi.Create();
|
|
// byte[] myPdf = ri.LatestVersion.PDF;
|
|
// if (myPdf != null) fs.Write(myPdf, 0, myPdf.Length);
|
|
// fs.Close();
|
|
// if (superceded)
|
|
// AddSupercededWatermark(VlnSettings.TemporaryFolder + @"\" + fileName);
|
|
// return VlnSettings.TemporaryFolder + @"\" + fileName;
|
|
//}
|
|
|
|
//private void AddSupercededWatermark(string p)
|
|
//{
|
|
// MessageBox.Show("superceded");
|
|
//}
|
|
//private string GetDocSummaryPdf(RevisionInfo ri)
|
|
//{
|
|
// string fileName = Volian.Base.Library.TmpFile.CreateFileName(ItemInfo.Get(ri.ItemID).DisplayNumber + " Summary of Changes");
|
|
// FileInfo fi = new FileInfo(VlnSettings.TemporaryFolder + @"\" + fileName);
|
|
// FileStream fs = fi.Create();
|
|
// byte[] myPdf = ri.LatestVersion.SummaryPDF;
|
|
// if (myPdf != null) fs.Write(myPdf, 0, myPdf.Length);
|
|
// fs.Close();
|
|
// return VlnSettings.TemporaryFolder + @"\" + fileName;
|
|
//}
|
|
|
|
|
|
private bool AddToInsertMenu(ItemInfo ii, int ba) // 0 = before, 1 = after
|
|
{
|
|
// set up insert buttons based on format
|
|
bool retB = true;
|
|
E_AccStep? actable = 0;
|
|
StepData sd = ii.FormatStepData;
|
|
actable = sd.StepEditData.AcTable;
|
|
if (actable == null) actable = 0;
|
|
|
|
return (ba == 0) ? !ii.IsRNOPart && (actable & E_AccStep.AddingPrev) > 0 :
|
|
!ii.IsRNOPart && (actable & E_AccStep.AddingNext) > 0;
|
|
}
|
|
private void Menu_Paste(VETreeNode tn, ContextMenu cm)
|
|
{
|
|
#region MenuPaste
|
|
// Find what's in paste buffer & determine whether the paste can occur for the selected node.
|
|
ItemInfo iiClipboard = OnClipboardStatus(this, new vlnTreeEventArgs(tn));
|
|
if (iiClipboard != null)
|
|
{
|
|
// can it be pasted at current node.
|
|
if (tn.VEObject as DocVersionInfo != null) // paste item must be a proc
|
|
{
|
|
if (iiClipboard.IsProcedure) cm.MenuItems.Add("Paste Procedure", new EventHandler(mi_Click));
|
|
}
|
|
else
|
|
{
|
|
ItemInfo iiPasteHere = tn.VEObject as ItemInfo;
|
|
if (iiPasteHere == null) return;
|
|
bool okToReplace = iiClipboard.ItemID != iiPasteHere.ItemID;
|
|
if (iiPasteHere != null)
|
|
{
|
|
SectionInfo si = (tn.VEObject as SectionInfo != null) ? tn.VEObject as SectionInfo : null;
|
|
if (iiPasteHere.IsProcedure && iiClipboard.IsProcedure) // procedure can be pasted before/replace/after
|
|
{
|
|
cm.MenuItems.Add("Paste Procedure Before", new EventHandler(mi_Click));
|
|
if (okToReplace) cm.MenuItems.Add("Replace Existing Procedure", new EventHandler(mi_Click));
|
|
cm.MenuItems.Add("Paste Procedure After", new EventHandler(mi_Click));
|
|
}
|
|
else if (iiPasteHere.IsProcedure && iiClipboard.IsSection) // procedure must have sections only
|
|
cm.MenuItems.Add("Paste Section", new EventHandler(mi_Click));
|
|
else if (iiPasteHere.IsSection && iiClipboard.IsSection)
|
|
{
|
|
cm.MenuItems.Add("Paste Section Before", new EventHandler(mi_Click));
|
|
if (okToReplace) cm.MenuItems.Add("Replace Existing Section", new EventHandler(mi_Click));
|
|
cm.MenuItems.Add("Paste Section After", new EventHandler(mi_Click));
|
|
if (si.ActiveFormat.PlantFormat.FormatData.SectData.UseMetaSections && iiPasteHere.IsStepSection && iiClipboard.IsStepSection)
|
|
{
|
|
// only paste a subsection if the paste here is an empty section or has
|
|
// subsections.
|
|
if (iiPasteHere.Sections == null || iiPasteHere.Sections.Count > 0)
|
|
cm.MenuItems.Add("Paste Subsection", new EventHandler(mi_Click));
|
|
}
|
|
}
|
|
else if (iiPasteHere.IsStepSection && iiClipboard.IsStep)
|
|
cm.MenuItems.Add("Paste Step", new EventHandler(mi_Click));
|
|
else if (iiPasteHere.IsStep && iiClipboard.IsStep)
|
|
{
|
|
if (AddToInsertMenu(iiPasteHere, 0)) cm.MenuItems.Add("Paste Step Before", new EventHandler(mi_Click));
|
|
if (okToReplace) cm.MenuItems.Add("Replace Existing Step", new EventHandler(mi_Click));
|
|
if (AddToInsertMenu(iiPasteHere, 1)) cm.MenuItems.Add("Paste Step After", new EventHandler(mi_Click));
|
|
}
|
|
}
|
|
}
|
|
}
|
|
#endregion menupaste
|
|
}
|
|
//jcb multiunit
|
|
void miMultiUnit_Click(object sender, EventArgs e)
|
|
{
|
|
MenuItem mi = sender as MenuItem;
|
|
MenuItem mip = mi.Parent as MenuItem;
|
|
switch (mip.Text)
|
|
{
|
|
case "Print":
|
|
OnPrintProcedure(this, new vlnTreeEventArgs(SelectedNode as VETreeNode, null, 0, mi.Text, (int)mi.Tag));
|
|
break;
|
|
case "Print All Procedures for":
|
|
OnPrintAllProcedures(this, new vlnTreeEventArgs(SelectedNode as VETreeNode, null, 0,mi.Text,(int)mi.Tag));
|
|
break;
|
|
case "Approve":
|
|
OnApproveProcedure(this, new vlnTreeEventArgs(SelectedNode as VETreeNode, null, 0, mi.Text, (int)mi.Tag));
|
|
break;
|
|
case "Approve All Procedures for":
|
|
OnApproveAllProcedures(this, new vlnTreeEventArgs(SelectedNode as VETreeNode, null, 0, mi.Text, (int)mi.Tag));
|
|
break;
|
|
case "Approve Some Procedures for":
|
|
OnApproveSomeProcedures(this, new vlnTreeEventArgs(SelectedNode as VETreeNode, null, 0, mi.Text, (int)mi.Tag));
|
|
break;
|
|
case "Create Continuous Action Summary":
|
|
OnCreateContinuousActionSummary(this, new vlnTreeEventArgs(SelectedNode as VETreeNode, null,0,mi.Text,(int)mi.Tag));
|
|
break;
|
|
default:
|
|
MessageBox.Show(string.Format("Unrecognized Menu Item '{0}'", mip.Text));
|
|
break;
|
|
}
|
|
}
|
|
//end jcb multiunit
|
|
void mi_Click(object sender, EventArgs e)
|
|
{
|
|
MenuItem mi = sender as MenuItem;
|
|
if (mi == null)
|
|
return;
|
|
if (mi.Text == "Working Draft Specific Information" || mi.Text == "Folder Specific Information")
|
|
{
|
|
VETreeNode tn = SelectedNode as VETreeNode;
|
|
OnNodeSI(this, new vlnTreeEventArgs(tn, null, 0));
|
|
return;
|
|
}
|
|
if (mi.Text == "Collapse Procedures")
|
|
{
|
|
CollapseProcedures();
|
|
return;
|
|
}
|
|
if (mi.Text == "Print Transition Report")
|
|
{
|
|
OnPrintTransitionReport(this, new vlnTreeEventArgs(SelectedNode as VETreeNode));
|
|
return;
|
|
}
|
|
if (mi.Text == "Export Procedure Set" || mi.Text == "Export Procedure")
|
|
{
|
|
OnExportImportProcedureSets(this, new vlnTreeEventArgs(SelectedNode as VETreeNode, null, 0));
|
|
return;
|
|
}
|
|
if (mi.Text == "Import Procedure Set" || mi.Text == "Import Procedure")
|
|
{
|
|
OnExportImportProcedureSets(this, new vlnTreeEventArgs(SelectedNode as VETreeNode, null, 1));
|
|
return;
|
|
}
|
|
if (mi.Text.StartsWith("Procedure Checked Out to") || mi.Text.StartsWith("Document Checked Out to"))
|
|
{
|
|
OnProcedureCheckedOutTo(this, new vlnTreeEventArgs(SelectedNode as VETreeNode, null, 0));
|
|
return;
|
|
}
|
|
switch (mi.Text)
|
|
{
|
|
case "Open":
|
|
OpenNode();
|
|
break;
|
|
case "Insert Folder Before":
|
|
tv_NodeNew(MenuSelections.FolderBefore);
|
|
break;
|
|
case "Insert Folder After":
|
|
tv_NodeNew(MenuSelections.FolderAfter);
|
|
break;
|
|
case "New Folder":
|
|
SelectedNode.Expand();
|
|
tv_NodeNew(MenuSelections.Folder);
|
|
break;
|
|
case "Create Working Draft":
|
|
SelectedNode.Expand();
|
|
tv_NodeNew(MenuSelections.DocVersion);
|
|
break;
|
|
case "New Procedure":
|
|
SelectedNode.Expand();
|
|
tv_NodeNew(MenuSelections.Procedure);
|
|
break;
|
|
case "Insert Procedure Before":
|
|
tv_NodeNew(MenuSelections.ProcedureBefore);
|
|
break;
|
|
case "Insert Procedure After":
|
|
tv_NodeNew(MenuSelections.ProcedureAfter);
|
|
break;
|
|
case "Remove Change Ids":
|
|
tv_RemoveChgIds();
|
|
break;
|
|
case "New Section":
|
|
case "New Subsection":
|
|
SelectedNode.Expand();
|
|
tv_NodeNew(MenuSelections.Section);
|
|
break;
|
|
case "Insert Section Before":
|
|
tv_NodeNew(MenuSelections.SectionBefore);
|
|
break;
|
|
case "Insert Section After":
|
|
tv_NodeNew(MenuSelections.SectionAfter);
|
|
break;
|
|
case "Insert Step Before":
|
|
tv_NodeNew(MenuSelections.StepBefore);
|
|
break;
|
|
case "Insert Step After":
|
|
tv_NodeNew(MenuSelections.StepAfter);
|
|
break;
|
|
case "New Step":
|
|
SelectedNode.Expand();
|
|
tv_NodeNew(MenuSelections.Step);
|
|
break;
|
|
case "Copy"://Copy the selected node
|
|
tv_NodeCopy();
|
|
break;
|
|
// lots of paste options:
|
|
case "Paste Procedure":
|
|
case "Paste Procedure Before":
|
|
case "Replace Existing Procedure":
|
|
case "Paste Procedure After":
|
|
case "Paste Section":
|
|
case "Paste Section Before":
|
|
case "Replace Existing Section":
|
|
case "Paste Section After":
|
|
case "Paste Step":
|
|
case "Paste Step Before":
|
|
case "Replace Existing Step":
|
|
case "Paste Step After":
|
|
case "Paste Subsection":
|
|
tv_NodePaste(mi.Text);
|
|
break;
|
|
case "Delete":
|
|
if (tv_NodeDelete())
|
|
{
|
|
TreeNode myParent = SelectedNode.Parent;
|
|
SelectedNode.Remove();
|
|
SelectedNode = myParent;
|
|
OnNodeSelect(this, new vlnTreeEventArgs(SelectedNode));
|
|
}
|
|
break;
|
|
case "Properties..."://Show the properties for the selected node
|
|
SetLastValues((VETreeNode)SelectedNode);
|
|
SetupNodeProperties();
|
|
break;
|
|
case "Procedure Specific Information":
|
|
VETreeNode tn = SelectedNode as VETreeNode;
|
|
ProcedureInfo pi = tn.VEObject as ProcedureInfo;
|
|
if (pi != null)
|
|
{
|
|
using (Procedure proc = pi.Get())
|
|
{
|
|
OnNodePSI(this, new vlnTreeEventArgs(tn, null, 0));
|
|
}
|
|
}
|
|
break;
|
|
case "Print":
|
|
OnPrintProcedure(this, new vlnTreeEventArgs(SelectedNode as VETreeNode, null, 0));
|
|
break;
|
|
case "Print All Procedures":
|
|
OnPrintAllProcedures(this, new vlnTreeEventArgs(SelectedNode as VETreeNode, null, 0));
|
|
break;
|
|
case "Approve":
|
|
OnApproveProcedure(this, new vlnTreeEventArgs(SelectedNode as VETreeNode, null, 0));
|
|
break;
|
|
case "Approve All Procedures":
|
|
OnApproveAllProcedures(this, new vlnTreeEventArgs(SelectedNode as VETreeNode, null, 0));
|
|
break;
|
|
case "Approve Some Procedures":
|
|
OnApproveSomeProcedures(this, new vlnTreeEventArgs(SelectedNode as VETreeNode, null, 0));
|
|
break;
|
|
case "Report All Procedures Inconsistencies":
|
|
OnReportAllProceduresInconsistencies(this, new vlnTreeEventArgs(SelectedNode as VETreeNode, null, 0));
|
|
break;
|
|
case "Refresh Checked Out Procedures":
|
|
OnRefreshCheckedOutProcedures(this, new vlnTreeEventArgs(SelectedNode as VETreeNode, null, 0));
|
|
break;
|
|
case "Run RO Editor":
|
|
RunROEditor(SelectedNode as VETreeNode);
|
|
break;
|
|
case "Update RO Values":
|
|
UpdateROValues(SelectedNode as VETreeNode);
|
|
break;
|
|
case "Create Continuous Action Summary":
|
|
OnCreateContinuousActionSummary(this, new vlnTreeEventArgs(SelectedNode as VETreeNode, null,0));
|
|
break;
|
|
//case "Check Out Procedure Set":
|
|
// CheckOutDocVersion(SelectedNode as VETreeNode);
|
|
// break;
|
|
//case "Check In Procedure Set":
|
|
// CheckInDocVersion(SelectedNode as VETreeNode);
|
|
// break;
|
|
default:
|
|
MessageBox.Show(string.Format("Unrecognized Menu Item '{0}'", mi.Text));
|
|
break;
|
|
}
|
|
}
|
|
private void CollapseProcedures()
|
|
{
|
|
CollapseProcedures(SelectedNode as VETreeNode);
|
|
}
|
|
private void CollapseProcedures(VETreeNode tn)
|
|
{
|
|
if (tn == null) return;
|
|
if (!tn.IsExpanded) return;
|
|
if (tn.VEObject.GetType() == typeof(ProcedureInfo))
|
|
{
|
|
tn.Collapse();
|
|
return;
|
|
}
|
|
foreach (VETreeNode tnc in tn.Nodes)
|
|
CollapseProcedures(tnc);
|
|
}
|
|
private void tv_RemoveChgIds()
|
|
{
|
|
//Console.WriteLine("HERE"); // add code/query to clear change ids in config.
|
|
}
|
|
private Dictionary<int, int> MyCheckedOutDocVersions;
|
|
private void CheckInDocVersion(VETreeNode tn)
|
|
{
|
|
DocVersionInfo MyDVI = tn.VEObject as DocVersionInfo;
|
|
MySessionInfo.CheckInItem(MyCheckedOutDocVersions[MyDVI.VersionID]);
|
|
MyCheckedOutDocVersions.Remove(MyDVI.VersionID);
|
|
}
|
|
|
|
private void UpdateROValues(VETreeNode tn)
|
|
{
|
|
InitialProgressBarMessage = "Updating ROs";
|
|
DocVersionInfo MyDVI = tn.VEObject as DocVersionInfo;
|
|
// use rodb directory path of the first rofst for the this document version. Later, will need
|
|
// to modify code to get which one (when there is more than one)
|
|
if (MyDVI.DocVersionAssociations.Count < 1)
|
|
{
|
|
MessageBox.Show("Error Updating ro.fst. No associated ro.fst");
|
|
FinalProgressBarMessage = "No ROs associated";
|
|
return;
|
|
}
|
|
ROFstInfo roFstInfo = MyDVI.DocVersionAssociations[0].MyROFst;
|
|
string rofstPath = roFstInfo.MyRODb.FolderPath + @"\ro.fst";
|
|
if (!File.Exists(rofstPath))
|
|
{
|
|
MessageBox.Show("No existing ro.fst in path " + roFstInfo.MyRODb.FolderPath + ". Check for invalid path");
|
|
FinalProgressBarMessage = "No existing RO.FST";
|
|
return;
|
|
}
|
|
FileInfo fiRofst = new FileInfo(rofstPath);
|
|
if (roFstInfo.DTS == fiRofst.LastWriteTimeUtc)
|
|
{
|
|
MessageBox.Show("ro.fst files are same for path " + roFstInfo.MyRODb.FolderPath + ", import of that ro.fst will not be done");
|
|
FinalProgressBarMessage = "RO.FST up to date";
|
|
return;
|
|
}
|
|
if (roFstInfo.DTS > fiRofst.LastWriteTimeUtc)
|
|
{
|
|
MessageBox.Show("Cannot copy older ro.fst from " + roFstInfo.MyRODb.FolderPath + ", import of that ro.fst will not be done");
|
|
FinalProgressBarMessage = "Older RO.FST";
|
|
return;
|
|
}
|
|
Cursor = Cursors.WaitCursor;
|
|
string message = string.Empty;
|
|
if (!MySessionInfo.CanCheckOutItem(MyDVI.VersionID, CheckOutType.DocVersion, ref message))
|
|
{
|
|
MessageBox.Show(this, message, "Working Draft Has Items Already Checked Out", MessageBoxButtons.OK, MessageBoxIcon.Warning);
|
|
FinalProgressBarMessage = "Cannot check-out Working Draft";
|
|
return;
|
|
}
|
|
int ownerid = MySessionInfo.CheckOutItem(MyDVI.VersionID, CheckOutType.DocVersion);
|
|
using (DocVersion dv = DocVersion.Get(MyDVI.VersionID))
|
|
{
|
|
roFstInfo.ROTableUpdate += new ROFstInfoROTableUpdateEvent(roFstInfo_ROTableUpdate);
|
|
ROFst newrofst = ROFstInfo.UpdateRoFst(roFstInfo.MyRODb, dv.DocVersionAssociations[0], dv, roFstInfo, DoProgressBarRefresh);
|
|
roFstInfo.ROTableUpdate -= new ROFstInfoROTableUpdateEvent(roFstInfo_ROTableUpdate);
|
|
StepPanelTabDisplayEventArgs args = new StepPanelTabDisplayEventArgs("DisplayROUpdateROFST");
|
|
}
|
|
MySessionInfo.CheckInItem(ownerid);
|
|
Cursor = Cursors.Default;
|
|
FinalProgressBarMessage = "ROs values updated";
|
|
}
|
|
private ProgressBarItem _ProgressBar=null;
|
|
public ProgressBarItem ProgressBar
|
|
{
|
|
get { return _ProgressBar; }
|
|
set { _ProgressBar = value; }
|
|
}
|
|
private void DoProgressBarRefresh(int value, int max, string text)
|
|
{
|
|
if (ProgressBar == null) return;
|
|
ProgressBar.Value = value;
|
|
ProgressBar.Maximum = max;
|
|
ProgressBar.Text = text;
|
|
Application.DoEvents();
|
|
}
|
|
private string InitialProgressBarMessage
|
|
{
|
|
set
|
|
{
|
|
if (ProgressBar == null) return;
|
|
ProgressBar.Value = 0;
|
|
ProgressBar.Maximum = 100;
|
|
ProgressBar.Text = value;
|
|
Application.DoEvents();
|
|
}
|
|
}
|
|
private string FinalProgressBarMessage
|
|
{
|
|
set
|
|
{
|
|
if (ProgressBar == null) return;
|
|
ProgressBar.Value = 100;
|
|
ProgressBar.Maximum = 100;
|
|
ProgressBar.Text = value;
|
|
Application.DoEvents();
|
|
}
|
|
}
|
|
public List<string> roFstInfo_ROTableUpdate(object sender, ROFstInfoROTableUpdateEventArgs args)
|
|
{
|
|
return VlnFlexGrid.ROTableUpdate(sender, args);
|
|
//string xml = null;
|
|
//string srchtxt = null;
|
|
//using (VlnFlexGrid myGrid = new VlnFlexGrid())
|
|
//{
|
|
// using (StringReader sr = new StringReader(args.OldGridXml))
|
|
// {
|
|
// myGrid.ReadXml(sr);
|
|
// myGrid.KeyActionTab = C1.Win.C1FlexGrid.KeyActionEnum.MoveAcross;
|
|
// sr.Close();
|
|
// }
|
|
// string roid = myGrid.ROID;
|
|
// int rodbid = myGrid.RODbId;
|
|
// Font GridFont = myGrid.Font;
|
|
// myGrid.Clear();
|
|
// myGrid.ParseTableFromText(args.ROText, GridLinePattern.Single);
|
|
// myGrid.AutoSizeCols();
|
|
// myGrid.AutoSizeRows();
|
|
// myGrid.MakeRTFcells();
|
|
// myGrid.RODbId = rodbid;
|
|
// myGrid.ROID = roid;
|
|
// myGrid.IsRoTable = true;
|
|
// using (StringWriter sw = new StringWriter())
|
|
// {
|
|
// myGrid.WriteXml(sw);
|
|
// xml = sw.GetStringBuilder().ToString();
|
|
// sw.Close();
|
|
// }
|
|
// srchtxt = myGrid.GetSearchableText();
|
|
//}
|
|
//List<string> retlist = new List<string>();
|
|
//retlist.Add(srchtxt);
|
|
//retlist.Add(xml);
|
|
//return retlist;
|
|
}
|
|
|
|
private void RunROEditor(VETreeNode tn)
|
|
{
|
|
DocVersionInfo MyDVI = tn.VEObject as DocVersionInfo;
|
|
if (VlnSettings.ReleaseMode.Equals("DEMO"))
|
|
{
|
|
MessageBox.Show("Referenced Object Editor not available in the Demo version.", "PROMS Demo Version");
|
|
return;
|
|
}
|
|
//string roapp = Environment.GetEnvironmentVariable("roapp");
|
|
string roapp = Volian.Base.Library.ExeInfo.GetROEditorPath(); // get the path to the RO Editor Executable
|
|
if (roapp == null || roapp == string.Empty)
|
|
{
|
|
MessageBox.Show("The 'roapp' environment variable needs to be set to the path of the RO Editor\n\n Ex: C:\\VE-PROMS.NET\\Bin\\roeditor.exe", "Environment Variable Error");
|
|
return;
|
|
}
|
|
if (!File.Exists(roapp))
|
|
{
|
|
string errtxt = string.Format("Could not find path to Referenced Objects Editor:\n\n roapp = {0}\n\n Verify the path assigned to the 'roapp' environment variable", roapp);
|
|
MessageBox.Show(errtxt, "Environment Variable Error");
|
|
//MessageBox.Show("Could not find path to Ro Editor, check 'roapp' environment variable","Environment Variable Error");
|
|
return;
|
|
}
|
|
//if (roapp == null)
|
|
//{
|
|
// MessageBox.Show("Could not find path to Ro Editor, check 'roapp' environment variable");
|
|
// return;
|
|
//}
|
|
if (MyDVI == null || MyDVI.DocVersionAssociationCount < 1)
|
|
{
|
|
MessageBox.Show("Could not find associated path for ro data.", "No RO Data", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
return;
|
|
}
|
|
string roloc = "\"" + MyDVI.DocVersionAssociations[0].MyROFst.MyRODb.FolderPath + "\"";
|
|
if (!Directory.Exists(MyDVI.DocVersionAssociations[0].MyROFst.MyRODb.FolderPath))
|
|
{
|
|
MessageBox.Show(string.Format("RO Database directory does not exist: {0}", MyDVI.DocVersionAssociations[0].MyROFst.MyRODb.FolderPath));
|
|
return;
|
|
}
|
|
System.Diagnostics.Process.Start(roapp, roloc);
|
|
}
|
|
|
|
[Serializable]
|
|
public struct PromsClipboard
|
|
{
|
|
public int cType;
|
|
public int itemId;
|
|
}
|
|
public enum PromsClipboardType : int
|
|
{
|
|
Copy = 1, Cut = 2
|
|
}
|
|
private void tv_NodePaste(string p)
|
|
{
|
|
ItemInfo iiClipboard = OnClipboardStatus(this, null);
|
|
if (iiClipboard == null) return;
|
|
string message = string.Empty;
|
|
if (iiClipboard.MyContent.MyEntry == null)
|
|
{
|
|
if (!MySessionInfo.CanCheckOutItem(iiClipboard.MyProcedure.ItemID, CheckOutType.Procedure, ref message))
|
|
{
|
|
MessageBox.Show(this, message, "Copied Procedure Already Checked Out", MessageBoxButtons.OK, MessageBoxIcon.Warning);
|
|
return;
|
|
}
|
|
}
|
|
else
|
|
{
|
|
if (!MySessionInfo.CanCheckOutItem(iiClipboard.MyContent.MyEntry.DocID, CheckOutType.Document, ref message))
|
|
{
|
|
MessageBox.Show(this, message, "Copied Document Already Checked Out", MessageBoxButtons.OK, MessageBoxIcon.Warning);
|
|
return;
|
|
}
|
|
}
|
|
VETreeNode tn = SelectedNode as VETreeNode;
|
|
DocVersionInfo dvi = tn.VEObject as DocVersionInfo;
|
|
// Check for paste into a docversion - queries/code is different than paste related to an item (into a proc or section)
|
|
if (dvi != null)
|
|
{
|
|
// If the docversion has procedures (children), change to a 'paste-after the last procedure'.
|
|
if (tn.Nodes.Count > 0)
|
|
{
|
|
// if the node count is 1, children may not have been loaded yet - load if necessary:
|
|
if (tn.Nodes.Count == 1 && tn.Nodes[0].Text.ToUpper().Contains("DUMMY VETREENODE"))
|
|
tn.LoadChildren();
|
|
|
|
// assume that item to paste is a procedure, otherwise the menuing would not have
|
|
// included the paste options
|
|
tn = (VETreeNode) tn.Nodes[tn.Nodes.Count - 1];
|
|
p = "After";
|
|
}
|
|
else // this is an empty docversion:
|
|
{
|
|
// First check if the doc version has RO's
|
|
// Paste as a child to the doc version:
|
|
this.Cursor = Cursors.WaitCursor;
|
|
PasteAsDocVersionChild(tn, iiClipboard.ItemID);
|
|
this.Cursor = Cursors.Default;
|
|
return;
|
|
}
|
|
}
|
|
|
|
ItemInfo iiPaste = tn.VEObject as ItemInfo;
|
|
if (iiPaste == null) return;
|
|
this.Cursor = Cursors.WaitCursor;
|
|
if (p.IndexOf("Before") > -1)
|
|
PasteBeforeOrAfter(MenuSelections.StepBefore, tn, iiClipboard.ItemID);
|
|
else if (p.IndexOf("After") > -1)
|
|
PasteBeforeOrAfter(MenuSelections.StepAfter, tn, iiClipboard.ItemID);
|
|
else if (p.IndexOf("Replace") > -1)
|
|
PasteReplace(tn, iiClipboard.ItemID);
|
|
else // paste as child
|
|
PasteAsChild(tn, iiClipboard.ItemID);
|
|
this.Cursor = Cursors.Default;
|
|
}
|
|
|
|
private void PasteAsDocVersionChild(VETreeNode tn, int copyStartID)
|
|
{
|
|
// Only need to handle paste in tree since this will create a new procedure.
|
|
DocVersionInfo dvi = tn.VEObject as DocVersionInfo;
|
|
if (dvi.DocVersionAssociationCount == 0)
|
|
{
|
|
// Set docversionassociation to the copied docversion association
|
|
// so that the rofst for ro's can be found. (if there is no association set)
|
|
ROFstInfo rfi = GetAssociationRofstId(copyStartID);
|
|
Association myAs = Association.MakeAssociation(dvi.Get(), rfi.GetJustROFst(), "");
|
|
dvi.RefreshDocVersionAssociations();
|
|
}
|
|
ItemInfo newProc = dvi.PasteChild(copyStartID);
|
|
VETreeNode tn1 = new VETreeNode(newProc);
|
|
SelectedNode.Nodes.Add(tn1); // add tree node to end of list.
|
|
SelectedNode = tn1;
|
|
}
|
|
|
|
private ROFstInfo GetAssociationRofstId(int copyStartID)
|
|
{
|
|
ItemInfo ii = ItemInfo.Get(copyStartID);
|
|
return ii.MyDocVersion.DocVersionAssociations[0].MyROFst;
|
|
}
|
|
private void PasteAsChild(VETreeNode tn, int copyStartID)
|
|
{
|
|
bool pasteSectIntoEmptySect = false;
|
|
ItemInfo ii = tn.VEObject as ItemInfo;
|
|
if (ii.IsStepSection) // is pasting a subsection, need to force a reload of the treeview
|
|
{
|
|
ItemInfo tmpcopy = ItemInfo.Get(copyStartID);
|
|
if (tmpcopy.IsStepSection) pasteSectIntoEmptySect = true;
|
|
}
|
|
ItemInfo.EAddpingPart pasteOpt = ItemInfo.EAddpingPart.Child;
|
|
// If parent step is open in step editor, the 'OnPasteItemInfo' event will cause
|
|
// the item to be pasted in the step editor and the tree.
|
|
if (!OnPasteItemInfo(this, new vlnTreeItemInfoPasteEventArgs(ii, copyStartID, pasteOpt, ii.MyContent.Type)))
|
|
{
|
|
// The parent step was not open in the step editor, just paste step (in data) and add treenode.
|
|
// first, check if a changeid is required.
|
|
string chgId = OnGetChangeId(this, new vlnTreeItemInfoEventArgs(ii));
|
|
ItemInfo newItemInfo = null;
|
|
newItemInfo = ii.PasteChild(copyStartID, chgId);
|
|
}
|
|
if (pasteSectIntoEmptySect)
|
|
{
|
|
tn.ChildrenLoaded = false; // force a reload of treenode
|
|
tn.LoadChildren(true);
|
|
}
|
|
SelectedNode = tn;
|
|
}
|
|
private void PasteBeforeOrAfter(MenuSelections newtype, VETreeNode tn, int copyStartID)
|
|
{
|
|
// If paste-from step is open in the editor, use the OnPasteItemInfo to paste the step and add RTBItems
|
|
// to the step editor panel.
|
|
ItemInfo ii = tn.VEObject as ItemInfo;
|
|
ItemInfo.EAddpingPart pasteOpt = newtype == MenuSelections.StepBefore ? ItemInfo.EAddpingPart.Before : ItemInfo.EAddpingPart.After;
|
|
// If parent step is open in step editor, the 'OnPasteItemInfo' event will cause
|
|
// the item to be pasted in the step editor and the tree.
|
|
if (ii.IsProcedure || !OnPasteItemInfo(this, new vlnTreeItemInfoPasteEventArgs(ii, copyStartID, pasteOpt, ii.MyContent.Type)))
|
|
{
|
|
// The parent step was not open in the step editor, just paste step (in data) and add treenode.
|
|
// first, check if a changeid is required.
|
|
string chgId = OnGetChangeId(this, new vlnTreeItemInfoEventArgs(ii));
|
|
ItemInfo newItemInfo = null;
|
|
if (newtype == MenuSelections.StepBefore)
|
|
newItemInfo = ii.PasteSiblingBefore(copyStartID, chgId);
|
|
else
|
|
newItemInfo = ii.PasteSiblingAfter(copyStartID, chgId);
|
|
}
|
|
SelectedNode = (VETreeNode)((newtype == MenuSelections.StepAfter) ? tn.NextNode : tn.PrevNode);
|
|
}
|
|
private void PasteReplace(VETreeNode tn, int copyStartID)
|
|
{
|
|
VETreeNode prevtn = (VETreeNode) tn.PrevNode;
|
|
VETreeNode partn = (VETreeNode) tn.Parent;
|
|
ItemInfo ii = tn.VEObject as ItemInfo;
|
|
if (!OnPasteItemInfo(this, new vlnTreeItemInfoPasteEventArgs(ii, copyStartID, ItemInfo.EAddpingPart.Replace, ii.MyContent.Type)))
|
|
{
|
|
// first, check if a changeid is required.
|
|
string chgId = OnGetChangeId(this, new vlnTreeItemInfoEventArgs(ii));
|
|
ItemInfo replItemInfo = Item.PasteReplace(ii, copyStartID, chgId);
|
|
if(replItemInfo != null) OnOpenItem(this,new vlnTreeItemInfoEventArgs(replItemInfo));
|
|
}
|
|
SelectedNode = (VETreeNode)((prevtn != null) ? prevtn.NextNode : partn.FirstNode);
|
|
}
|
|
private void tv_NodeCopy()
|
|
{
|
|
if (SelectedNode==null)return;
|
|
VETreeNode tn = SelectedNode as VETreeNode;
|
|
OnNodeCopy(this, new vlnTreeEventArgs(tn));
|
|
}
|
|
#endregion
|
|
#region PropertyPagesInterface
|
|
private void SetupNodeProperties()
|
|
{
|
|
VETreeNode tn = SelectedNode as VETreeNode;
|
|
if (tn==null)return;
|
|
|
|
if ((tn.VEObject as FolderInfo) != null)
|
|
OpenProperties(tn.VEObject as FolderInfo);
|
|
else if ((tn.VEObject as DocVersionInfo) != null)
|
|
OpenProperties(tn.VEObject as DocVersionInfo);
|
|
else if ((tn.VEObject as ProcedureInfo) != null)
|
|
OpenProperties(tn.VEObject as ProcedureInfo);
|
|
else if ((tn.VEObject as SectionInfo) != null)
|
|
OpenProperties(tn.VEObject as SectionInfo);
|
|
else if ((tn.VEObject as StepInfo) != null)
|
|
MessageBox.Show("Open up info tab or whatever is associated with step");
|
|
tn.RefreshNode();
|
|
}
|
|
private void OpenProperties(FolderInfo folderInfo)
|
|
{
|
|
using (Folder folder = folderInfo.Get())
|
|
{
|
|
OnNodeOpenProperty(this, new vlnTreePropertyEventArgs(string.Format("{0} Properties", folder.FolderConfig.Name), folder.FolderConfig));
|
|
}
|
|
}
|
|
private void OpenProperties(DocVersionInfo dvInfo)
|
|
{
|
|
using (DocVersion dv = dvInfo.Get())
|
|
{
|
|
OnNodeOpenProperty(this, new vlnTreePropertyEventArgs(string.Format("{0} Properties", dv.DocVersionConfig.Name), dv.DocVersionConfig));
|
|
}
|
|
}
|
|
private void OpenProperties(ProcedureInfo procInfo)
|
|
{
|
|
using (Procedure proc = procInfo.Get())
|
|
{
|
|
OnNodeOpenProperty(this, new vlnTreePropertyEventArgs(string.Format("{0} {1} Properties", proc.ProcedureConfig.Number, proc.ProcedureConfig.Title), proc.ProcedureConfig));
|
|
}
|
|
}
|
|
private void OpenProperties(SectionInfo sectInfo)
|
|
{
|
|
OnSectionShouldClose(this, new vlnTreeSectionInfoEventArgs(sectInfo));
|
|
using (Section sect = sectInfo.Get())
|
|
{
|
|
string title = null;
|
|
if (sectInfo.SectionConfig.Number.Length > 0)
|
|
title = string.Format("{0} {1} Properties", sectInfo.SectionConfig.Number, sectInfo.SectionConfig.Title);
|
|
else
|
|
title = string.Format("{0} Properties", sectInfo.SectionConfig.Title);
|
|
OnNodeOpenProperty(this, new vlnTreePropertyEventArgs(title, sect.SectionConfig));
|
|
}
|
|
}
|
|
private void OpenProperties(StepInfo stpinfo)
|
|
{
|
|
using (Step stp = stpinfo.Get())
|
|
{
|
|
}
|
|
}
|
|
|
|
#endregion
|
|
#region OpenNode
|
|
private void tv_KeyPress(object sender, KeyPressEventArgs e)
|
|
{
|
|
if (e.KeyChar == '\r')
|
|
{
|
|
OpenNode();
|
|
e.Handled = true;
|
|
}
|
|
}
|
|
public void OpenNode()
|
|
{
|
|
VETreeNode tn = SelectedNode as VETreeNode;
|
|
if (tn != null)
|
|
{
|
|
if (tn.VEObject.GetType() == typeof(FolderInfo) || tn.VEObject.GetType() == typeof(DocVersionInfo) || tn.VEObject.GetType() == typeof(PartInfo))
|
|
{
|
|
if (tn.Nodes.Count > 0)
|
|
{
|
|
tn.Expand();
|
|
SelectedNode = tn.Nodes[0];
|
|
Focus();
|
|
}
|
|
}
|
|
else
|
|
OnNodeSelect(this, new vlnTreeEventArgs(SelectedNode));
|
|
}
|
|
}
|
|
#endregion
|
|
#region InsertAllLevels
|
|
public void tv_NodeNew(MenuSelections newtype)
|
|
{
|
|
VETreeNode tn = null;
|
|
if (SelectedNode == null) return;
|
|
SetLastValues((VETreeNode)SelectedNode);
|
|
|
|
#region InsertFolderOrDocVersion
|
|
if (_LastFolderInfo != null)
|
|
{
|
|
using (Folder parentfolder = _LastFolderInfo.Get())
|
|
{
|
|
if (newtype == MenuSelections.DocVersion)
|
|
{
|
|
int dvid = -1; // flag to allow user to cancel from dialog & then we remove it.
|
|
using (DocVersion docversion = DocVersion.MakeDocVersion(parentfolder, "Working Draft", "Title", null, null, null))
|
|
{
|
|
ShowBrokenRules(docversion.BrokenRulesCollection);
|
|
SetLastValues(DocVersionInfo.Get(docversion.VersionID));
|
|
if (OnNodeOpenProperty(this, new vlnTreePropertyEventArgs("Working Draft", docversion.DocVersionConfig)) == DialogResult.OK)
|
|
{
|
|
docversion.Save();
|
|
tn = new VETreeNode(_LastDocVersionInfo);
|
|
SelectedNode.Nodes.Add(tn); // add tree node to end of list.
|
|
}
|
|
else
|
|
dvid = docversion.VersionID;
|
|
}
|
|
if (dvid != -1) DocVersion.Delete(dvid);
|
|
}
|
|
else if (newtype == MenuSelections.Folder)
|
|
{
|
|
int f1 = -1; // flag to allow user to cancel from dialog & then we remove it.
|
|
string uniquename = _LastFolderInfo.UniqueChildName("New Folder");
|
|
using (Folder folder = Folder.MakeFolder(parentfolder, parentfolder.MyConnection, uniquename, string.Empty, "Short Name", null, string.Empty, DateTime.Now, VlnSettings.UserID))
|
|
{
|
|
ShowBrokenRules(folder.BrokenRulesCollection);
|
|
FolderInfo fi = FolderInfo.Get(folder.FolderID);
|
|
folder.ManualOrder = fi.ManualOrder;
|
|
SetLastValues(fi);
|
|
if (OnNodeOpenProperty(this, new vlnTreePropertyEventArgs(uniquename, folder.FolderConfig)) == DialogResult.OK)
|
|
{
|
|
folder.Save();
|
|
tn = new VETreeNode((IVEDrillDownReadOnly)_LastFolderInfo);
|
|
SelectedNode.Nodes.Add(tn); // add new tree node to end of childlist.
|
|
}
|
|
else
|
|
f1 = folder.FolderID;
|
|
}
|
|
if (f1 != -1) Folder.Delete(f1);
|
|
}
|
|
else if (newtype == MenuSelections.FolderAfter||newtype == MenuSelections.FolderBefore)
|
|
{
|
|
int f2 = -1;
|
|
string uniquename = _LastFolderInfo.MyParent.UniqueChildName("New Folder");
|
|
int myindex = SelectedNode.Index + ((newtype == MenuSelections.FolderAfter) ? 1 : 0);
|
|
FolderInfo parfolderinfo = FolderInfo.Get(parentfolder.FolderID);
|
|
double? myorder = parfolderinfo.NewManualOrder(myindex);
|
|
using (Folder folder = Folder.MakeFolder(parentfolder.MyParent, parentfolder.MyConnection, uniquename, string.Empty, "Short Name", null, myorder, string.Empty, DateTime.Now, VlnSettings.UserID))
|
|
{
|
|
ShowBrokenRules(folder.BrokenRulesCollection);
|
|
SetLastValues(FolderInfo.Get(folder.FolderID));
|
|
if (OnNodeOpenProperty(this, new vlnTreePropertyEventArgs(uniquename, folder.FolderConfig)) == DialogResult.OK)
|
|
{
|
|
folder.Save();
|
|
tn = new VETreeNode((IVEDrillDownReadOnly)_LastFolderInfo);
|
|
if (newtype == MenuSelections.FolderBefore) SelectedNode.Parent.Nodes.Insert(SelectedNode.Index, tn);
|
|
if (newtype == MenuSelections.FolderAfter) SelectedNode.Parent.Nodes.Insert(SelectedNode.Index + 1, tn);
|
|
}
|
|
else
|
|
f2 = folder.FolderID;
|
|
}
|
|
if (f2 != -1) Folder.Delete(f2);
|
|
}
|
|
}
|
|
}
|
|
#endregion
|
|
#region InsertProcedure
|
|
else if (newtype == MenuSelections.Procedure)
|
|
{
|
|
int p1 = -1;
|
|
using (Procedure procedure = Procedure.MakeProcedure(_LastDocVersionInfo, _LastDocVersionInfo.Procedures.Count!=0?_LastDocVersionInfo.Procedures[_LastDocVersionInfo.Procedures.Count-1]:null, null, "New Procedure", 0))
|
|
{
|
|
ShowBrokenRules(procedure.BrokenRulesCollection);
|
|
SetLastValues(ProcedureInfo.Get(procedure.ItemID));
|
|
if (OnNodeOpenProperty(this, new vlnTreePropertyEventArgs("New Procedure", procedure.ProcedureConfig)) == DialogResult.OK)
|
|
{
|
|
procedure.Save();
|
|
tn = new VETreeNode(_LastProcedureInfo);
|
|
SelectedNode.Nodes.Add(tn); // add tree node to end of list.
|
|
}
|
|
else
|
|
p1 = procedure.ItemID;
|
|
}
|
|
if (p1 != -1)
|
|
DeleteItemInfoAndChildren(_LastProcedureInfo);// Delete Item and reset Previous and Next
|
|
}
|
|
else if (newtype == MenuSelections.ProcedureAfter || newtype == MenuSelections.ProcedureBefore)
|
|
{
|
|
int p2 = -1;
|
|
int tvindex = SelectedNode.Index;
|
|
// if inserting before, the parent is set in case previous is null, i.e. beginning of the list.
|
|
using (Procedure procedure = Procedure.MakeProcedure((newtype == MenuSelections.ProcedureAfter) ? null : _LastProcedureInfo.ActiveParent, (newtype == MenuSelections.ProcedureAfter) ? _LastProcedureInfo : _LastProcedureInfo.MyPrevious, null, "New Procedure", 0))
|
|
{
|
|
ShowBrokenRules(procedure.BrokenRulesCollection);
|
|
SetLastValues(ProcedureInfo.Get(procedure.ItemID));
|
|
if (OnNodeOpenProperty(this, new vlnTreePropertyEventArgs("New Procedure", procedure.ProcedureConfig)) == DialogResult.OK)
|
|
{
|
|
procedure.Save();
|
|
tn = new VETreeNode(_LastProcedureInfo);
|
|
TreeNode par = SelectedNode.Parent;
|
|
par.Nodes.Insert(tvindex + ((newtype == MenuSelections.ProcedureBefore) ? 0 : 1), tn);
|
|
}
|
|
else
|
|
p2 = procedure.ItemID;
|
|
}
|
|
if (p2 != -1)
|
|
DeleteItemInfoAndChildren(_LastProcedureInfo); // Delete Item and reset Previous and Next
|
|
}
|
|
#endregion
|
|
#region InsertSection
|
|
else if (newtype == MenuSelections.Section) // Insert subsection at end of parents section list
|
|
{
|
|
string message = string.Empty;
|
|
if (_LastProcedureInfo != null)
|
|
if (!MySessionInfo.CanCheckOutItem(_LastProcedureInfo.ItemID, CheckOutType.Procedure, ref message))
|
|
{
|
|
MessageBox.Show(this, message, "Item Already Checked Out", MessageBoxButtons.OK, MessageBoxIcon.Warning);
|
|
return;
|
|
}
|
|
int s1 = -1;
|
|
if (!(_LastProcedureInfo == null) || !(_LastSectionInfo == null))
|
|
{
|
|
// May need to create 'pseudo' nodes, for the 'Steps' and 'Sections' grouping tree nodes.
|
|
// If no grouping nodes exist off a section (we are adding a subsection), then
|
|
// add a 'pseudo' node.
|
|
// If step(s) exists, but not a section, then need 'pseudo' nodes, i.e. 'Steps' and 'Sections'
|
|
// tree nodes.
|
|
// Otherwise, the 'Sections' pseudo node will exist.
|
|
// Note that this check has to be done before the section is made, otherwise the item will
|
|
// have sections, i.e. the one getting added.
|
|
bool doPseudo = false;
|
|
if (_LastItemInfo.IsSection) // if adding off of a procedure, don't add pseuo.
|
|
{
|
|
if (SelectedNode.Nodes.Count == 0)
|
|
doPseudo = true;
|
|
else if (SelectedNode.Nodes.Count > 0)
|
|
{
|
|
// Check to see if sections already exist, if so, the pseudo node will too.
|
|
bool hasMetaSubs = _LastItemInfo.Sections != null && _LastItemInfo.Sections.Count > 0;
|
|
if (!hasMetaSubs) doPseudo = true;
|
|
}
|
|
}
|
|
using(Section section = CreateNewSection())
|
|
{
|
|
ShowBrokenRules(section.BrokenRulesCollection);
|
|
SetLastValues(SectionInfo.Get(section.ItemID));
|
|
if (OnNodeOpenProperty(this, new vlnTreePropertyEventArgs("New Section", section.SectionConfig)) == DialogResult.OK)
|
|
{
|
|
if (!doPseudo)
|
|
{
|
|
tn = new VETreeNode(_LastSectionInfo);
|
|
SelectedNode.Nodes.Add(tn); // add tree node to end of list.
|
|
OnNodeSelect(this, new vlnTreeEventArgs(tn));
|
|
}
|
|
else
|
|
{
|
|
// may have to add a 'steps' node if a step(s) already exist...
|
|
ItemInfo ii = (SelectedNode as VETreeNode).VEObject as ItemInfo;
|
|
int cpindx = 0;
|
|
if (SelectedNode.Nodes.Count>0)
|
|
{
|
|
VETreeNode vtn = SelectedNode.Nodes[0] as VETreeNode;
|
|
ItemInfo ichld1 = null;
|
|
if (vtn != null) ichld1 = vtn.VEObject as ItemInfo;
|
|
//ItemInfo ichld1 = (SelectedNode.Nodes[0] as VETreeNode).VEObject as ItemInfo;
|
|
if (ichld1 != null && ichld1.IsStep)
|
|
{
|
|
// find associated part node:
|
|
foreach (PartInfo pi in ii.MyContent.ContentParts)
|
|
{
|
|
if (pi.FromType == (int)E_FromType.Step)
|
|
{
|
|
// A 'Steps' pseudo node must be added and any existing steps
|
|
// must be moved below it:
|
|
VETreeNode tnStepPart = new VETreeNode(ii.MyContent.ContentParts[cpindx]);
|
|
foreach (TreeNode tnc in SelectedNode.Nodes)
|
|
{
|
|
TreeNode cloned = (TreeNode)tnc.Clone();
|
|
tnStepPart.Nodes.Add(cloned);
|
|
}
|
|
SelectedNode.Nodes.Clear();
|
|
SelectedNode.Nodes.Add(tnStepPart);
|
|
break;
|
|
}
|
|
cpindx++;
|
|
}
|
|
}
|
|
}
|
|
// At this level, there will only be 'Steps' or 'Sections' nodes. So, use
|
|
// the ContentParts[indx] that was not used to create the pseudo node.
|
|
if (cpindx == 1) cpindx = 0;
|
|
VETreeNode tnPart = new VETreeNode(ii.MyContent.ContentParts[cpindx]);
|
|
SelectedNode.Nodes.Add(tnPart);
|
|
tn = new VETreeNode(_LastSectionInfo);
|
|
tnPart.Nodes.Add(tn); // add tree node to end of list.
|
|
}
|
|
}
|
|
else
|
|
s1 = section.ItemID;
|
|
}
|
|
if (s1 != -1)
|
|
DeleteItemInfoAndChildren(_LastSectionInfo);// Delete Item and reset Previous and Next
|
|
}
|
|
}
|
|
else if (newtype == MenuSelections.SectionAfter || newtype == MenuSelections.SectionBefore)
|
|
{
|
|
string message = string.Empty;
|
|
if (!MySessionInfo.CanCheckOutItem(_LastSectionInfo.MyProcedure.ItemID, CheckOutType.Procedure, ref message))
|
|
{
|
|
MessageBox.Show(this, message, "Item Already Checked Out", MessageBoxButtons.OK, MessageBoxIcon.Warning);
|
|
return;
|
|
}
|
|
int tvindex = SelectedNode.Index;
|
|
// if inserting before, the parent is set in case previous is null, i.e. beginning of the list.
|
|
int s2 = -1;
|
|
using (Section section = Section.MakeSection((newtype == MenuSelections.SectionAfter) ? null : _LastSectionInfo.MyParent, (newtype == MenuSelections.SectionAfter) ? _LastSectionInfo : _LastSectionInfo.MyPrevious, null, "New Section", 10000))
|
|
{
|
|
ShowBrokenRules(section.BrokenRulesCollection);
|
|
SetLastValues(SectionInfo.Get(section.ItemID));
|
|
TreeNode par = SelectedNode.Parent;
|
|
if (OnNodeOpenProperty(this, new vlnTreePropertyEventArgs("New Section", section.SectionConfig)) == DialogResult.OK)
|
|
{
|
|
int indx = tvindex + ((newtype == MenuSelections.SectionBefore) ? 0 : 1);
|
|
if (indx >= par.Nodes.Count || (par.Nodes[indx] as VETreeNode).VEObject.ToString() != _LastSectionInfo.ToString())
|
|
{
|
|
tn = new VETreeNode(_LastSectionInfo);
|
|
par.Nodes.Insert(indx, tn);
|
|
}
|
|
}
|
|
else
|
|
s2 = section.ItemID;
|
|
}
|
|
if (s2 != -1)
|
|
DeleteItemInfoAndChildren(_LastSectionInfo);// Delete Item and reset Previous and Next
|
|
}
|
|
#endregion
|
|
#region InsertStep
|
|
else if (newtype == MenuSelections.Step) // insert step from section - no substeps from tree.
|
|
{
|
|
string message = string.Empty;
|
|
if (!MySessionInfo.CanCheckOutItem(_LastSectionInfo.MyProcedure.ItemID, CheckOutType.Procedure, ref message))
|
|
{
|
|
MessageBox.Show(this, message, "Item Already Checked Out", MessageBoxButtons.OK, MessageBoxIcon.Warning);
|
|
return;
|
|
}
|
|
tn = InsertChildStep((VETreeNode)SelectedNode);
|
|
}
|
|
else if (newtype == MenuSelections.StepAfter || newtype == MenuSelections.StepBefore && _LastStepInfo != null)
|
|
{
|
|
string message = string.Empty;
|
|
if (!MySessionInfo.CanCheckOutItem(_LastStepInfo.MyProcedure.ItemID, CheckOutType.Procedure, ref message))
|
|
{
|
|
MessageBox.Show(this, message, "Item Already Checked Out", MessageBoxButtons.OK, MessageBoxIcon.Warning);
|
|
return;
|
|
}
|
|
tn = InsertBeforeOrAfter(newtype, (VETreeNode)SelectedNode);
|
|
}
|
|
#endregion
|
|
|
|
if (tn != null)
|
|
{
|
|
SelectedNode = tn;
|
|
OnNodeNew(this, new vlnTreeEventArgs(SelectedNode));
|
|
Refresh();
|
|
OnNodeInsert(this, new vlnTreeEventArgs(SelectedNode));
|
|
}
|
|
}
|
|
private Section CreateNewSection()
|
|
{
|
|
if(_LastItemInfo.LastChild(E_FromType.Section) != null)
|
|
return Section.MakeSection(_LastItemInfo, _LastItemInfo.LastChild(E_FromType.Section), null, "New Section", 10000);
|
|
ItemInfo iii = _LastItemInfo.InsertChild(E_FromType.Section, 10000, "New Section", null);
|
|
return Section.Get(iii.ItemID);
|
|
}
|
|
private VETreeNode InsertChildStep(VETreeNode tn)
|
|
{
|
|
// If parent step is open in editor, use the OnInsertItemInfo to insert step & add RTBItems to step editor panel
|
|
ItemInfo ii = tn.VEObject as ItemInfo;
|
|
if (OnInsertItemInfo(this, new vlnTreeItemInfoInsertEventArgs(ii, E_InsertType.Child, "New Step", 20002, E_FromType.Step)))
|
|
{
|
|
return null; // should we try to get to the child?
|
|
}
|
|
// The parent step was not open in the step editor, just create new step and add treenode.
|
|
using (Step step = Step.MakeStep(_LastItemInfo, _LastItemInfo.LastChild(E_FromType.Step), null, "New Step", 20002, E_FromType.Step))
|
|
{
|
|
ShowBrokenRules(step.BrokenRulesCollection);
|
|
SetLastValues(StepInfo.Get(step.ItemID));
|
|
tn = new VETreeNode(_LastStepInfo);
|
|
SelectedNode.Nodes.Add(tn); // add tree node to end of list.
|
|
}
|
|
return tn;
|
|
}
|
|
|
|
private VETreeNode InsertBeforeOrAfter(MenuSelections newtype, VETreeNode tn)
|
|
{
|
|
// If parent step is open in editor, use the OnInsertItemInfo to insert step & add RTBItems to step editor panel
|
|
ItemInfo ii = tn.VEObject as ItemInfo;
|
|
if (OnInsertItemInfo(this, new vlnTreeItemInfoInsertEventArgs(ii,(newtype == MenuSelections.StepAfter) ? E_InsertType.After:E_InsertType.Before, "New Step")))
|
|
{
|
|
return (VETreeNode)((newtype == MenuSelections.StepAfter) ? tn.NextNode : tn.PrevNode);
|
|
}
|
|
// The parent step was not open in the step editor, just create new step and add treenode.
|
|
int tvindex = SelectedNode.Index;
|
|
// if inserting before, the parent is set in case previous is null, i.e. beginning of the list.
|
|
ItemInfo parent = (newtype == MenuSelections.StepAfter) ? null : _LastStepInfo.MyParent;
|
|
using (Step step = Step.MakeStep(parent, (newtype == MenuSelections.StepAfter) ? _LastStepInfo : _LastStepInfo.MyPrevious, null, "New Step", (int)_LastStepInfo.MyContent.Type, (E_FromType)_LastStepInfo.FirstSibling.ItemParts[0].FromType))
|
|
{
|
|
ShowBrokenRules(step.BrokenRulesCollection);
|
|
SetLastValues(StepInfo.Get(step.ItemID));
|
|
tn = new VETreeNode(_LastStepInfo);
|
|
_LastStepInfo.UpdateTransitionText();
|
|
_LastStepInfo.UpdateROText();
|
|
TreeNode par = SelectedNode.Parent;
|
|
par.Nodes.Insert(tvindex + ((newtype == MenuSelections.StepBefore) ? 0 : 1), tn);
|
|
}
|
|
return tn;
|
|
}
|
|
private void ShowBrokenRules(BrokenRulesCollection brs)
|
|
{
|
|
if (brs != null)
|
|
{
|
|
foreach (BrokenRule br in brs)
|
|
{
|
|
Console.WriteLine("broken rule {0}", br.Description);
|
|
}
|
|
}
|
|
}
|
|
#endregion
|
|
#region DeleteAllLevels
|
|
private bool tv_NodeDelete()
|
|
{
|
|
SetLastValues((VETreeNode)SelectedNode);
|
|
string message = string.Empty;
|
|
if(_LastStepInfo != null)
|
|
if (!MySessionInfo.CanCheckOutItem(_LastStepInfo.MyProcedure.ItemID, CheckOutType.Procedure, ref message))
|
|
{
|
|
MessageBox.Show(this, message, "Item Already Checked Out", MessageBoxButtons.OK, MessageBoxIcon.Warning);
|
|
return false;
|
|
}
|
|
if (_LastSectionInfo != null)
|
|
if (!MySessionInfo.CanCheckOutItem(_LastSectionInfo.MyProcedure.ItemID, CheckOutType.Procedure, ref message))
|
|
{
|
|
MessageBox.Show(this, message, "Item Already Checked Out", MessageBoxButtons.OK, MessageBoxIcon.Warning);
|
|
return false;
|
|
}
|
|
if (_LastProcedureInfo != null)
|
|
if (!MySessionInfo.CanCheckOutItem(_LastProcedureInfo.ItemID, CheckOutType.Procedure, ref message))
|
|
{
|
|
MessageBox.Show(this, message, "Item Already Checked Out", MessageBoxButtons.OK, MessageBoxIcon.Warning);
|
|
return false;
|
|
}
|
|
if (_LastDocVersionInfo != null)
|
|
{
|
|
StringBuilder sb = new StringBuilder();
|
|
foreach (ProcedureInfo pi in _LastDocVersionInfo.Procedures)
|
|
{
|
|
if (!MySessionInfo.CanCheckOutItem(pi.ItemID, CheckOutType.Procedure, ref message))
|
|
sb.AppendLine(message);
|
|
message = string.Empty;
|
|
}
|
|
if (sb.Length > 0)
|
|
{
|
|
MessageBox.Show(this, sb.ToString(), "Items Already Checked Out", MessageBoxButtons.OK, MessageBoxIcon.Warning);
|
|
return false;
|
|
}
|
|
}
|
|
if (_LastFolderInfo != null)
|
|
{
|
|
StringBuilder sb = new StringBuilder();
|
|
if (_LastFolderInfo.FolderDocVersionCount > 0)
|
|
{
|
|
foreach (DocVersionInfo dvi in _LastFolderInfo.FolderDocVersions)
|
|
{
|
|
foreach (ProcedureInfo pi in dvi.Procedures)
|
|
{
|
|
if (!MySessionInfo.CanCheckOutItem(pi.ItemID, CheckOutType.Procedure, ref message))
|
|
sb.AppendLine(message);
|
|
message = string.Empty;
|
|
}
|
|
}
|
|
}
|
|
if (sb.Length > 0)
|
|
{
|
|
MessageBox.Show(this, sb.ToString(), "Items Already Checked Out", MessageBoxButtons.OK, MessageBoxIcon.Warning);
|
|
return false;
|
|
}
|
|
}
|
|
|
|
DialogResult result = MessageBox.Show("Are you sure you want to delete " + SelectedNode.Text, "Verify Delete",
|
|
MessageBoxButtons.YesNo, MessageBoxIcon.Question);
|
|
if (result == DialogResult.Yes)
|
|
{
|
|
if (_LastFolderInfo != null)
|
|
{
|
|
Folder.Delete(_LastFolderInfo.FolderID);
|
|
_LastFolderInfo = null;
|
|
return true;
|
|
}
|
|
else if (_LastDocVersionInfo != null)
|
|
{
|
|
DocVersion.Delete(_LastDocVersionInfo.VersionID);
|
|
_LastDocVersionInfo = null;
|
|
return true;
|
|
}
|
|
else if (_LastProcedureInfo != null)
|
|
{
|
|
// always return false because an event gets fired to delete tree nodes.
|
|
if (!DeleteItemInfoAndChildren(_LastProcedureInfo)) return false;
|
|
_LastProcedureInfo = null;
|
|
return false;
|
|
}
|
|
else if (_LastSectionInfo != null)
|
|
{
|
|
OnSectionShouldClose(this, new vlnTreeSectionInfoEventArgs(_LastSectionInfo,true));
|
|
// always return false because an event gets fired to delete tree nodes.
|
|
if (!DeleteItemInfoAndChildren(_LastSectionInfo))
|
|
{
|
|
return false;
|
|
}
|
|
WordSectionEventArgs args = new WordSectionEventArgs(_LastSectionInfo);
|
|
OnWordSectionDeleted(this, args);
|
|
_LastSectionInfo = null;
|
|
return false;
|
|
}
|
|
else if (_LastStepInfo != null)
|
|
{
|
|
// always return false because an event gets fired to delete tree nodes.
|
|
if (!DeleteItemInfoAndChildren(_LastStepInfo)) return false;
|
|
_LastStepInfo = null;
|
|
return false;
|
|
}
|
|
}
|
|
return false;
|
|
}
|
|
private bool DeleteItemInfoAndChildren(ItemInfo ii)
|
|
{
|
|
DateTime dtStart = DateTime.Now;
|
|
try
|
|
{
|
|
// send an event to frmVeproms that sends an event to the stepeditor to
|
|
// do delete using RTBItem - this manages windowing from the step editor.
|
|
// If the procedure is open & you're deleting procedure, you want to close open
|
|
// window - this is done in DisplayTabControl-DeleteStepTabItem.
|
|
OnProcessing(true,"Deleting");
|
|
if (!OnDeleteItemInfo(this, new vlnTreeItemInfoEventArgs(ii)))
|
|
Item.DeleteItemAndChildren(ii);
|
|
OnProcessing(false,"Deleted");
|
|
OnProcessingComplete(dtStart,"Deleted");
|
|
return true;
|
|
}
|
|
catch (System.Data.SqlClient.SqlException ex)
|
|
{
|
|
OnProcessing(false,"Delete Failed");
|
|
OnProcessingComplete(dtStart,"Delete Failed");
|
|
ItemInfo iii = ii.HandleSqlExceptionOnDelete(ex);
|
|
if(iii != null) OnOpenItem(this, new vlnTreeItemInfoEventArgs(iii));
|
|
return false;
|
|
}
|
|
}
|
|
public event vlnTreeViewTimeEvent ProcessingComplete;
|
|
private void OnProcessingComplete(DateTime dtStart, string message)
|
|
{
|
|
if (ProcessingComplete != null)
|
|
ProcessingComplete(this, new vlnTreeTimeEventArgs(dtStart,message));
|
|
}
|
|
public event vlnTreeViewStatusEvent Processing;
|
|
private void OnProcessing(bool status, string message)
|
|
{
|
|
if (Processing != null)
|
|
Processing(this, new vlnTreeStatusEventArgs(status,message));
|
|
}
|
|
#endregion
|
|
#region SetLastValuesAndSaveIfChangedStuff
|
|
private void SetLastValues(VETreeNode node)
|
|
{
|
|
_LastTreeNode = node;
|
|
SetLastValues(node.VEObject);
|
|
}
|
|
private void SetLastValues(IVEDrillDownReadOnly veobject)
|
|
{
|
|
_LastFolderInfo = veobject as FolderInfo;
|
|
_LastDocVersionInfo = veobject as DocVersionInfo;
|
|
_LastProcedureInfo = veobject as ProcedureInfo;
|
|
_LastSectionInfo = veobject as SectionInfo;
|
|
_LastStepInfo = veobject as StepInfo;
|
|
_LastItemInfo = veobject as ItemInfo;
|
|
}
|
|
#endregion
|
|
#region Cursor
|
|
private bool SetupDragCursor(ImageList il, TreeNode tn)
|
|
{
|
|
// Reset image list used for drag image
|
|
il.Images.Clear();
|
|
int howBig = tn.Bounds.Size.Width + this.Indent;
|
|
if (howBig > 256) howBig = 256;
|
|
il.ImageSize = new Size(howBig, tn.Bounds.Height);
|
|
|
|
// Create new bitmap
|
|
// This bitmap will contain the tree node image to be dragged
|
|
Bitmap bmp = new Bitmap(tn.Bounds.Width + this.Indent, tn.Bounds.Height);
|
|
|
|
// Get graphics from bitmap
|
|
Graphics gfx = Graphics.FromImage(bmp);
|
|
|
|
// Draw node icon into the bitmap
|
|
if (this.ImageList != null) gfx.DrawImage(this.ImageList.Images[0], 0, 0);
|
|
|
|
// Draw node label into bitmap
|
|
gfx.DrawString(tn.Text, this.Font, new SolidBrush(this.ForeColor),
|
|
// new SolidBrush(Color.Blue),
|
|
(float)this.Indent, 1.0f);
|
|
|
|
// Add bitmap to imagelist
|
|
_dragImageList.Images.Add(bmp);
|
|
|
|
// Get mouse position in client coordinates
|
|
Point p = this.PointToClient(Control.MousePosition);
|
|
|
|
// Compute delta between mouse position and node bounds
|
|
int dx = p.X + this.Indent - tn.Bounds.Left;
|
|
int dy = p.Y - tn.Bounds.Top;
|
|
|
|
// Begin dragging image
|
|
return DragHelper.ImageList_BeginDrag(_dragImageList.Handle, 0, dx, dy);
|
|
}
|
|
private void tv_ItemDrag(object sender, System.Windows.Forms.ItemDragEventArgs e)
|
|
{
|
|
// Get drag node and select it
|
|
try
|
|
{
|
|
TreeNode dragNode = (TreeNode)e.Item;
|
|
Type t = dragNode.GetType();
|
|
//if(_MyLog.IsInfoEnabled)_MyLog.InfoFormat("Item Drag {0} - {1}", t.FullName, t.BaseType.FullName);
|
|
Type t2 = Type.GetType(t.FullName);
|
|
//if(t2 != null)
|
|
// if(_MyLog.IsInfoEnabled)_MyLog.InfoFormat("Item Drag {0} - {1}", t2.FullName, t2.BaseType.FullName);
|
|
this.SelectedNode = dragNode;
|
|
ItemInfo iidrag = ((VETreeNode)dragNode).VEObject as ItemInfo;
|
|
FolderInfo fdrag = ((VETreeNode)dragNode).VEObject as FolderInfo;
|
|
DocVersionInfo ddrag = ((VETreeNode)dragNode).VEObject as DocVersionInfo;
|
|
if ((iidrag == null && fdrag == null && ddrag == null))
|
|
{
|
|
MessageBox.Show("Cannot drag/drop a grouping node.");
|
|
return;
|
|
}
|
|
// don't put up message, message kept coming up on any selection of node (to copy, properties, etc)
|
|
//if (iidrag != null && iidrag.IsStep) return;
|
|
if (SetupDragCursor(_dragImageList, dragNode))
|
|
{
|
|
this.DoDragDrop(dragNode, DragDropEffects.Move | DragDropEffects.Copy);// Begin dragging
|
|
DragHelper.ImageList_EndDrag();// End dragging image
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if(_MyLog.IsErrorEnabled)_MyLog.Error("tv_ItemDrag", ex);
|
|
}
|
|
}
|
|
#endregion
|
|
#region DragDrop
|
|
ImageList _dragImageList = new ImageList();
|
|
public enum DropPosition : int
|
|
{
|
|
Child = 0, Before = 1, After = 2
|
|
}
|
|
private class DropLocation
|
|
{
|
|
#region Business Methods
|
|
private TreeNode _dropNode;
|
|
public TreeNode DropNode
|
|
{
|
|
get { return _dropNode; }
|
|
set { _dropNode = value; }
|
|
}
|
|
private int _index;
|
|
public int Index
|
|
{
|
|
get { return _index; }
|
|
set { _index = value; }
|
|
}
|
|
private DropPosition _position;
|
|
public DropPosition Position
|
|
{
|
|
get { return _position; }
|
|
set { _position = value; }
|
|
}
|
|
DateTime _lastScroll;
|
|
public DateTime LastScroll
|
|
{
|
|
get { return _lastScroll; }
|
|
}
|
|
private string _location = string.Empty;
|
|
#endregion
|
|
#region Constructors
|
|
public DropLocation(TreeView tv, System.Windows.Forms.DragEventArgs e, DateTime lastScroll)
|
|
{
|
|
_lastScroll = lastScroll;
|
|
_dropNode = tv.GetNodeAt(tv.PointToClient(new Point(e.X, e.Y)));
|
|
if (_dropNode == null) return;
|
|
int OffsetY = tv.PointToClient(Cursor.Position).Y - _dropNode.Bounds.Top;
|
|
if (OffsetY < _dropNode.Bounds.Height / 3) // First Third - Before
|
|
{
|
|
_index = _dropNode.Index;
|
|
_dropNode = _dropNode.Parent;
|
|
_position = DropPosition.Before;
|
|
_location = string.Format("Before1 {0}[{1}] y={2}", _dropNode.Text, _index, OffsetY);
|
|
}
|
|
else if ((OffsetY / 2) < _dropNode.Bounds.Height / 3) // Second Third - Child
|
|
{
|
|
_location = string.Format("Child {0} y={1}", _dropNode.Text, OffsetY);
|
|
_position = DropPosition.Child;
|
|
_index = 0;
|
|
//if (_dropNode.Parent == null)
|
|
//{
|
|
// if(_MyLog.IsInfoEnabled)_MyLog.Info("Root Node");
|
|
//}
|
|
}
|
|
else // Last Third - After Now I need to check the X value
|
|
{
|
|
if (_dropNode.NextVisibleNode != null && _dropNode.Nodes.Count > 0 && _dropNode.IsExpanded)// Has Children & Expanded - Insert first child
|
|
{
|
|
// _dropNode = _dropNode.Nodes[0];
|
|
_index = 0;
|
|
_position = DropPosition.Before;
|
|
_location = string.Format("Before2 {0}[{1}] y={2}", _dropNode.Text, _index, OffsetY);
|
|
}
|
|
else // No Children or Children Collapsed - Insert Next at various levels depending upon horizontal location.
|
|
{
|
|
Point pt = tv.PointToClient(new Point(e.X, e.Y));
|
|
TreeNode nextParent = _dropNode.NextNode;
|
|
if (nextParent != null) nextParent = nextParent.Parent;
|
|
do
|
|
{
|
|
_index = _dropNode.Index;
|
|
_dropNode = _dropNode.Parent;
|
|
} while (pt.X < _dropNode.Bounds.X && _dropNode != nextParent);
|
|
_location = string.Format("After {0}[{1}] y={2}", _dropNode.Text, _index, OffsetY);
|
|
_position = DropPosition.After;
|
|
}
|
|
}
|
|
LimitMoves(e);
|
|
}
|
|
public void LimitMoves(DragEventArgs e)
|
|
{
|
|
if ((e.KeyState & 8) == 0)
|
|
{
|
|
//TreeNode dragNode = (TreeNode)e.Data.GetData("System.Windows.Forms.TreeNode");
|
|
//TreeNode dragNode = (TreeNode)e.Data.GetData("TreeTest.FolderTreeNode");
|
|
TreeNode dragNode = vlnTreeView.GetTreeNodeFromData(e.Data);
|
|
switch (_position)
|
|
{
|
|
case DropPosition.Before:
|
|
if (dragNode == _dropNode.Nodes[_index] || dragNode == _dropNode.Nodes[_index].PrevNode)
|
|
{
|
|
// if(_MyLog.IsInfoEnabled)_MyLog.InfoFormat("Before {0} {1} {2} {3} {4}", dragNode.Text ,_position.ToString() , _dropNode.Nodes[_index].Text
|
|
// ,_dropNode.Nodes[_index].PrevNode,_dropNode.Nodes[_index].NextNode);
|
|
_dropNode = null;
|
|
}
|
|
break;
|
|
case DropPosition.Child:
|
|
if (dragNode.Parent == _dropNode)
|
|
{
|
|
// if(_MyLog.IsInfoEnabled)_MyLog.InfoFormat("Child {0} {1} {2} {3} {4} {5}", dragNode.Text ,_position.ToString() , _dropNode.Nodes[_index].Text
|
|
// ,_dropNode.Nodes[_index].PrevNode,_dropNode.Nodes[_index].NextNode,DateTime.Now);
|
|
_dropNode = null;
|
|
}
|
|
break;
|
|
case DropPosition.After:
|
|
if (dragNode == _dropNode.Nodes[_index] || dragNode == _dropNode.Nodes[_index].NextNode)
|
|
{
|
|
// if(_MyLog.IsInfoEnabled)_MyLog.InfoFormat("After {0} {1} {2} {3} {4}", dragNode.Text ,_position.ToString() , _dropNode.Nodes[_index].Text
|
|
// ,_dropNode.Nodes[_index].PrevNode,_dropNode.Nodes[_index].NextNode);
|
|
_dropNode = null;
|
|
}
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
#endregion
|
|
public override string ToString()
|
|
{
|
|
return string.Format("{0}[{1}].{2}", _dropNode.Text, _index, _position.ToString());
|
|
}
|
|
#region Drawing
|
|
private void TreeNodeTriangle(Graphics g)
|
|
{
|
|
Rectangle r = _dropNode.Bounds;
|
|
int RightPos = r.Right + 6;
|
|
Point[] RightTriangle = new Point[]{
|
|
new Point(RightPos, r.Y ),
|
|
new Point(RightPos - (r.Height / 2), r.Y + (r.Height / 2)),
|
|
new Point(RightPos, r.Y + r.Height),
|
|
new Point(RightPos - (r.Height / 3), r.Y + (r.Height / 2))
|
|
};
|
|
g.FillPolygon(System.Drawing.Brushes.Black, RightTriangle);
|
|
}
|
|
private void InsertPointer(TreeNode tn, Graphics g)
|
|
{
|
|
TreeView tv = _dropNode.TreeView;
|
|
Rectangle r2 = _dropNode.Nodes[_index].Bounds;
|
|
Rectangle r3 = tn.Bounds;
|
|
int y = (_position == DropPosition.Before ? r2.Y : r3.Bottom);
|
|
int x = r2.Left;
|
|
|
|
if (y == 0)
|
|
{
|
|
return;
|
|
}
|
|
//if(_MyLog.IsInfoEnabled)_MyLog.InfoFormat("Line at {0} Node {1}[{2}] {3}", _location, _dropNode.Text, _index, _position.ToString());
|
|
Color lc = (_position == DropPosition.After ? Color.Red : Color.Blue);
|
|
Brush lb = (_position == DropPosition.After ? Brushes.Red : Brushes.Blue);
|
|
Point[] RightTriangle;
|
|
if (_position == DropPosition.After)
|
|
{
|
|
RightTriangle = new Point[]{
|
|
new Point(x, y ),
|
|
new Point(x+4, y+4),
|
|
new Point(x+8, y)};
|
|
}
|
|
else
|
|
{
|
|
RightTriangle = new Point[]{
|
|
new Point(x, y),
|
|
new Point(x+4, y-4),
|
|
new Point(x+8, y)};
|
|
}
|
|
g.DrawLine(new System.Drawing.Pen(lc, 2), new Point(r2.Left, y), new Point(tv.Width - 8, y));
|
|
g.FillPolygon(lb, RightTriangle);
|
|
}
|
|
public void ShowLocation(System.Windows.Forms.DragEventArgs e, bool ScrollOnly)
|
|
{
|
|
//if (e.Effect == DragDropEffects.None) return;
|
|
if (_dropNode != null)
|
|
{
|
|
// if(_MyLog.IsInfoEnabled)_MyLog.InfoFormat("ShowLocation1 {0} {1}", e.Effect.ToString(), DateTime.Now.Millisecond);
|
|
DragHelper.ImageList_DragShowNolock(false);
|
|
TreeView tv = _dropNode.TreeView;
|
|
TreeNode tmp = tv.GetNodeAt(tv.PointToClient(new Point(e.X, e.Y)));
|
|
// if (!ScrollOnly)
|
|
// {
|
|
if (ScrollTreeView(tmp) || !ScrollOnly)
|
|
{
|
|
//if(_MyLog.IsInfoEnabled)_MyLog.InfoFormat("ShowLocation2 {0} {1}", e.Effect.ToString(), DateTime.Now.Millisecond);
|
|
tv.Refresh();
|
|
if (e.Effect != DragDropEffects.None)
|
|
{
|
|
//tv.SelectedNode = dropNode;
|
|
Graphics g = tv.CreateGraphics();
|
|
TreeNodeTriangle(g);
|
|
if (_position != DropPosition.Child)InsertPointer(tmp, g);
|
|
}
|
|
}
|
|
// }
|
|
// else ScrollTreeView(tmp);
|
|
DragHelper.ImageList_DragShowNolock(true);
|
|
}
|
|
}
|
|
#endregion
|
|
public void ShowLocation()
|
|
{
|
|
//if(_MyLog.IsInfoEnabled)_MyLog.InfoFormat("{0}[{1}] {2}", _dropNode.Text, _index, _position.ToString());
|
|
}
|
|
#region AutoScroll
|
|
private bool ScrollTreeView(TreeNode tn)
|
|
{
|
|
bool retval = false;
|
|
TimeSpan ts = new TimeSpan(DateTime.Now.Ticks - _lastScroll.Ticks);
|
|
if (ts.Milliseconds > 100)// This controls the scroll speed
|
|
{
|
|
int top = tn.Bounds.Top;
|
|
_lastScroll = DateTime.Now;
|
|
if (tn.TreeView.ClientSize.Height < tn.Bounds.Bottom) tn.EnsureVisible();// Make sure that the current node is visible
|
|
if (tn.NextVisibleNode != null && tn.TreeView.ClientSize.Height < tn.NextVisibleNode.Bounds.Bottom)
|
|
tn.NextVisibleNode.EnsureVisible();// Make sure that the next node is visible
|
|
else
|
|
if (tn.PrevVisibleNode != null && tn.PrevVisibleNode.PrevVisibleNode != null && tn.PrevVisibleNode.PrevVisibleNode.IsVisible == false)
|
|
tn.PrevVisibleNode.PrevVisibleNode.EnsureVisible();// Make sure that the previous node is visible }
|
|
else
|
|
if (tn.PrevVisibleNode != null && tn.PrevVisibleNode.IsVisible == false)
|
|
tn.PrevVisibleNode.EnsureVisible();// Make sure that the previous node is visible
|
|
retval = (top != tn.Bounds.Top);
|
|
// if (retval) if(_MyLog.IsInfoEnabled)_MyLog.Info("Scroll");
|
|
}
|
|
return retval;
|
|
}
|
|
#endregion
|
|
public bool Equals(DropLocation dl)
|
|
{
|
|
return (dl != null && _lastScroll.Equals(dl.LastScroll) && _dropNode.Equals(dl.DropNode) &&
|
|
_position.Equals(dl.Position));
|
|
}
|
|
}
|
|
private DropLocation _LastDropLocation = null;
|
|
private void tv_DragOver(object sender, System.Windows.Forms.DragEventArgs e)
|
|
{
|
|
try
|
|
{
|
|
TreeNode dragNode = GetTreeNodeFromData(e.Data);
|
|
// Compute drag position and move image
|
|
Point formP = this.FindForm().PointToClient(new Point(e.X, e.Y));
|
|
DragHelper.ImageList_DragMove(formP.X - this.Left, formP.Y - this.Top);
|
|
DropLocation dl = new DropLocation(this, e, _LastDropLocation == null ? DateTime.Now : _LastDropLocation.LastScroll);
|
|
string s = string.Empty;
|
|
if (dl.DropNode == null)
|
|
{
|
|
e.Effect = DragDropEffects.None;
|
|
}
|
|
else
|
|
{
|
|
DragDropEffects ee = e.Effect;
|
|
if (e.KeyState == 13) // Shift and Control Keys to do a move.
|
|
ee = DragDropEffects.Move;
|
|
else
|
|
ee = DragDropEffects.None; // Default - Do nothing
|
|
if (IsChild(dragNode, dl.DropNode)) // Don't copy or move to a child node
|
|
ee = DragDropEffects.None;
|
|
else if (IsDocVersion((VETreeNode)dragNode)) // Don't move docversions
|
|
ee = DragDropEffects.None;
|
|
else if (IsFolder((VETreeNode)dragNode)) // Folder move is only valid if moving to folder with NO docversions
|
|
{
|
|
FolderInfo fdropi = ((VETreeNode)dl.DropNode).VEObject as FolderInfo;
|
|
if (fdropi == null || fdropi.FolderDocVersionCount > 0) ee = DragDropEffects.None;
|
|
}
|
|
else if (IsSection((VETreeNode)dragNode))
|
|
{
|
|
// A section can be moved within a procedure or to a section within the same procedure...
|
|
// For HLP, just move within the same procedure
|
|
// TODO: allow for section move within subsections.
|
|
ProcedureInfo pdropi = ((VETreeNode)dl.DropNode).VEObject as ProcedureInfo;
|
|
if (pdropi == null || (dragNode.Parent != dl.DropNode)) ee = DragDropEffects.None;
|
|
}
|
|
else if (!IsFolder((VETreeNode)dragNode) && (dragNode.Parent != dl.DropNode))
|
|
ee = DragDropEffects.None;
|
|
if (e.Effect != ee) e.Effect = ee;
|
|
dl.ShowLocation(e, dl.Equals(_LastDropLocation));
|
|
_LastDropLocation = dl;
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if(_MyLog.IsErrorEnabled)_MyLog.Error("tv_DragOver", ex);
|
|
}
|
|
}
|
|
|
|
private bool IsSection(VETreeNode vETreeNode)
|
|
{
|
|
SectionInfo sectInfo = vETreeNode.VEObject as SectionInfo;
|
|
if (sectInfo != null) return true;
|
|
return false;
|
|
}
|
|
private bool IsProcedure(VETreeNode vETreeNode)
|
|
{
|
|
ProcedureInfo procInfo = vETreeNode.VEObject as ProcedureInfo;
|
|
if (procInfo != null) return true;
|
|
return false;
|
|
}
|
|
private bool IsDocVersion(VETreeNode dragNode)
|
|
{
|
|
DocVersionInfo dvInfo = dragNode.VEObject as DocVersionInfo;
|
|
if (dvInfo != null) return true;
|
|
return false;
|
|
}
|
|
private static TreeNode GetTreeNodeFromData(IDataObject datobj)
|
|
{
|
|
foreach (string s in datobj.GetFormats())
|
|
{
|
|
try
|
|
{
|
|
return (TreeNode)datobj.GetData(s);
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if(_MyLog.IsErrorEnabled)_MyLog.Error("GetTreeNodeFromData", ex);
|
|
}
|
|
}
|
|
return null;
|
|
}
|
|
private bool IsFolder(VETreeNode veTreeNode)
|
|
{
|
|
return (veTreeNode.VEObject.GetType() == typeof(FolderInfo));
|
|
}
|
|
private void tv_DragDrop(object sender, System.Windows.Forms.DragEventArgs e)
|
|
{
|
|
try
|
|
{
|
|
TreeNode dragNode = GetTreeNodeFromData(e.Data);
|
|
DragHelper.ImageList_DragLeave(this.Handle);
|
|
int index = _LastDropLocation.Index + (_LastDropLocation.Position == DropPosition.After ? 1 : 0);
|
|
int myIndex = index;
|
|
if (dragNode.Parent == _LastDropLocation.DropNode && dragNode.Index <= _LastDropLocation.Index) index--;
|
|
if (e.Effect == DragDropEffects.Move)// If Move Remove drag node from parent
|
|
dragNode.Remove();
|
|
else
|
|
dragNode = Clone(dragNode);
|
|
_LastDropLocation.DropNode.Nodes.Insert(index, dragNode);
|
|
this.SelectedNode = dragNode;
|
|
FolderInfo fdragi = ((VETreeNode)dragNode).VEObject as FolderInfo;
|
|
FolderInfo fdropi = ((VETreeNode)_LastDropLocation.DropNode).VEObject as FolderInfo;
|
|
if (fdragi != null && fdropi != null && fdropi.FolderDocVersionCount==0)
|
|
{
|
|
using (Folder fdrag = fdragi.Get())
|
|
{
|
|
using (Folder fdrop = fdropi.Get())
|
|
{
|
|
fdrag.ManualOrder = fdropi.NewManualOrder(myIndex);
|
|
fdrag.MyParent = fdrop;
|
|
fdrag.Save();
|
|
}
|
|
}
|
|
return;
|
|
}
|
|
// No drag/drop supported for document versions.
|
|
|
|
// Allow drag/drop of procedures within a Document Version (must have same parent). Note that drop location
|
|
// may either be a document version or a procedure depending on where the user wants to position the procedure.
|
|
ProcedureInfo pdragi = ((VETreeNode)dragNode).VEObject as ProcedureInfo;
|
|
ProcedureInfo pdropi = null;
|
|
if (pdragi != null) // moving a procedure
|
|
{
|
|
pdropi = ((VETreeNode)_LastDropLocation.DropNode).VEObject as ProcedureInfo;
|
|
if (pdropi != null && pdragi.ActiveParent == pdropi.ActiveParent)
|
|
{
|
|
pdragi.MoveProcedure(pdragi.ActiveParent, myIndex);
|
|
return;
|
|
}
|
|
DocVersionInfo dvdropi = ((VETreeNode)_LastDropLocation.DropNode).VEObject as DocVersionInfo;
|
|
DocVersionInfo dvdragpar = pdragi.ActiveParent as DocVersionInfo;
|
|
if (dvdropi != null && dvdragpar.VersionID == dvdropi.VersionID)
|
|
{
|
|
pdragi.MoveProcedure(dvdropi, myIndex);
|
|
return;
|
|
}
|
|
}
|
|
|
|
// Allow drag/drop of sections within the same procedure or same section (if subsection) (must have same parent)
|
|
SectionInfo sdragi = ((VETreeNode)dragNode).VEObject as SectionInfo;
|
|
SectionInfo sdropi = null;
|
|
if (sdragi != null) // moving a section
|
|
{
|
|
sdropi = ((VETreeNode)_LastDropLocation.DropNode).VEObject as SectionInfo;
|
|
if (sdropi != null && sdragi.ActiveParent == sdropi.ActiveParent)
|
|
{
|
|
sdragi.MoveSection(sdragi, myIndex);
|
|
return;
|
|
}
|
|
pdropi = ((VETreeNode)_LastDropLocation.DropNode).VEObject as ProcedureInfo;
|
|
if (pdropi != null && ((ItemInfo)(sdragi.ActiveParent)).ItemID == pdropi.ItemID)
|
|
{
|
|
sdragi.MoveSection(pdropi, myIndex);
|
|
sdragi.Moving = true;
|
|
OnNodeSelect(dragNode, new vlnTreeEventArgs(dragNode));
|
|
return;
|
|
}
|
|
}
|
|
|
|
// Allow drag/drop of steps within the same parent only
|
|
StepInfo stdragi = ((VETreeNode)dragNode).VEObject as StepInfo;
|
|
StepInfo stdropi = null;
|
|
if (stdragi != null) // moving a step
|
|
{
|
|
stdropi = ((VETreeNode)_LastDropLocation.DropNode).VEObject as StepInfo;
|
|
if (stdropi != null && stdragi.ActiveParent == stdropi.ActiveParent)
|
|
{
|
|
stdragi.MoveStep(stdragi.ActiveParent, myIndex);
|
|
return;
|
|
}
|
|
sdropi = ((VETreeNode)_LastDropLocation.DropNode).VEObject as SectionInfo;
|
|
if (sdropi != null && stdragi.MyParent.ItemID == sdropi.ItemID)
|
|
{
|
|
stdragi.MoveStep(stdragi.ActiveParent, myIndex);
|
|
return;
|
|
}
|
|
// the following handles items under the app nodes of 'steps', 'notes', 'cautions', etc.
|
|
if (sdropi == null && dragNode.Parent == _LastDropLocation.DropNode)
|
|
{
|
|
stdragi.MoveStep(stdragi.ActiveParent, myIndex);
|
|
return;
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if(_MyLog.IsErrorEnabled)_MyLog.Error("tv_DragDrop", ex);
|
|
}
|
|
}
|
|
// private void DumpMembers(object o)
|
|
// {
|
|
// Type t = o.GetType();
|
|
// //if(_MyLog.IsInfoEnabled)_MyLog.InfoFormat("\r\n\r\nMembers for type {0}", t.ToString());
|
|
// MemberInfo[] mis = t.GetMembers();
|
|
// int i = 0;
|
|
// foreach (MemberInfo mi in mis)
|
|
// {
|
|
// i++;
|
|
// try
|
|
// {
|
|
// //if(mi.MemberType != MemberTypes.Method)
|
|
// //if(_MyLog.IsInfoEnabled)_MyLog.InfoFormat("{0} {1} {2}", i, mi.Name, mi.MemberType);
|
|
//// if (fi.Name == "TreeView")
|
|
//// fi.SetValue(o, null);
|
|
// }
|
|
// catch (Exception ex)
|
|
// {
|
|
// if(_MyLog.IsErrorEnabled)_MyLog.Error("DumpMembers", ex);
|
|
// }
|
|
// }
|
|
// }
|
|
private TreeNode Clone(TreeNode tn)
|
|
{
|
|
|
|
TreeNode tmp = (TreeNode)tn.Clone();
|
|
ExpandMatch(tmp,tn);
|
|
return tmp;
|
|
}
|
|
private void tv_DragDropOld(object sender, System.Windows.Forms.DragEventArgs e)
|
|
{
|
|
TreeNode dragNode = (TreeNode)e.Data.GetData("System.Windows.Forms.TreeNode");// Get the drag node
|
|
DragHelper.ImageList_DragLeave(this.Handle);
|
|
TreeNode cloneNode = (TreeNode)dragNode.Clone();// copy the source node
|
|
ExpandMatch(cloneNode, dragNode);
|
|
_LastDropLocation.DropNode.Nodes.Insert(_LastDropLocation.Index + (_LastDropLocation.Position == DropPosition.After ? 1 : 0), cloneNode);
|
|
if (e.Effect == DragDropEffects.Move)// If Move Remove drag node from parent
|
|
dragNode.Remove();
|
|
this.SelectedNode = cloneNode;
|
|
}
|
|
private void tv_DragEnter(object sender, System.Windows.Forms.DragEventArgs e)
|
|
{
|
|
DragHelper.ImageList_DragEnter(this.Handle, e.X - this.Left, e.Y - this.Top);
|
|
}
|
|
private void tv_DragLeave(object sender, System.EventArgs e)
|
|
{
|
|
DragHelper.ImageList_DragLeave(this.Handle);
|
|
this.Refresh();
|
|
}
|
|
private void ExpandMatch(TreeNode tn1, TreeNode tn2)
|
|
{
|
|
if (tn2.IsExpanded) tn1.Expand();
|
|
foreach (TreeNode tc in tn2.Nodes) ExpandMatch(tn1.Nodes[tc.Index], tc);
|
|
}
|
|
private bool IsChild(TreeNode parent, TreeNode child)
|
|
{
|
|
if (parent.Equals(child)) return true;// Check against self
|
|
foreach (TreeNode tc in parent.Nodes) if (IsChild(tc, child)) return true;//Check all children
|
|
return false;// Must not be a child at this level
|
|
}
|
|
#endregion
|
|
private bool _AdjustingTree = false;
|
|
public void AdjustTree(ItemInfo selectedItem)
|
|
{
|
|
// start at the top parent and walk down the nodes to find child
|
|
VETreeNode node = FindNodeAndExpand(selectedItem);
|
|
if (node != null)
|
|
{
|
|
_AdjustingTree = true;
|
|
this.SelectedNode = node;
|
|
_AdjustingTree = false;
|
|
}
|
|
}
|
|
public VETreeNode FindNodeAndExpand(IVEDrillDownReadOnly selectedItem)
|
|
{
|
|
if (selectedItem.ActiveParent == null)
|
|
{
|
|
return (VETreeNode)this.Nodes[0]; // Return the top node
|
|
}
|
|
VETreeNode parent = FindNodeAndExpand(selectedItem.ActiveParent);
|
|
if (parent == null) return null;
|
|
if (!parent.IsExpanded)
|
|
parent.Expand();
|
|
VETreeNode child = GetChildNode(selectedItem, parent);
|
|
if (child != null)
|
|
return child;
|
|
parent.ChildrenLoaded = false;
|
|
parent.RefreshNode();
|
|
child = GetChildNode(selectedItem, parent);
|
|
return child;
|
|
}
|
|
public VETreeNode FindNode(IVEDrillDownReadOnly selectedItem, TreeNodeCollection tnc)
|
|
{
|
|
foreach (TreeNode tn in tnc)
|
|
if (tn is VETreeNode)
|
|
{
|
|
if ((tn as VETreeNode).VEObject is ItemInfo && ((tn as VETreeNode).VEObject as ItemInfo).ItemID == (selectedItem as ItemInfo).ItemID)
|
|
return tn as VETreeNode;
|
|
else
|
|
{
|
|
VETreeNode cn = FindNode(selectedItem, tn.Nodes);
|
|
if (cn != null)
|
|
return cn;
|
|
}
|
|
}
|
|
return null;
|
|
}
|
|
public VETreeNode RefreshRelatedNode(IVEDrillDownReadOnly selectedItem)
|
|
{
|
|
VETreeNode child = FindNode(selectedItem, this.Nodes);
|
|
if (child == null) return null;
|
|
if (!child.IsExpanded)
|
|
child.Expand();
|
|
child.ChildrenLoaded = false;
|
|
child.RefreshNode();
|
|
return child;
|
|
}
|
|
|
|
private VETreeNode GetChildNode(IVEDrillDownReadOnly selectedItem, VETreeNode parent)
|
|
{
|
|
foreach (TreeNode childNode in parent.Nodes)
|
|
{
|
|
VETreeNode child = childNode as VETreeNode;
|
|
if (child != null && CompareVEObject(child.VEObject, selectedItem))
|
|
return child;
|
|
}
|
|
foreach (TreeNode childNode in parent.Nodes)
|
|
{
|
|
VETreeNode child = childNode as VETreeNode;
|
|
if (child.VEObject is PartInfo)
|
|
foreach (VETreeNode grandchild in child.Nodes)
|
|
if (CompareVEObject(grandchild.VEObject, selectedItem))
|
|
return grandchild;
|
|
}
|
|
return null;
|
|
}
|
|
public bool CompareVEObject(IVEDrillDownReadOnly obj1, IVEDrillDownReadOnly obj2)
|
|
{
|
|
ItemInfo myItem = obj1 as ItemInfo;
|
|
if (myItem != null)
|
|
if (myItem.ItemID == ((ItemInfo)obj2).ItemID) return true;
|
|
DocVersionInfo myDV = obj1 as DocVersionInfo;
|
|
if (myDV != null)
|
|
if (myDV.VersionID == ((DocVersionInfo)obj2).VersionID) return true;
|
|
FolderInfo myFolder = obj1 as FolderInfo;
|
|
if (myFolder != null)
|
|
if (myFolder.FolderID == ((FolderInfo)obj2).FolderID) return true;
|
|
return false;
|
|
}
|
|
}
|
|
#region DragHelper
|
|
public class DragHelper
|
|
{
|
|
[DllImport("comctl32.dll")]
|
|
public static extern bool InitCommonControls();
|
|
|
|
[DllImport("comctl32.dll", CharSet = CharSet.Auto)]
|
|
public static extern bool ImageList_BeginDrag(IntPtr himlTrack, int
|
|
iTrack, int dxHotspot, int dyHotspot);
|
|
|
|
[DllImport("comctl32.dll", CharSet = CharSet.Auto)]
|
|
public static extern bool ImageList_DragMove(int x, int y);
|
|
|
|
[DllImport("comctl32.dll", CharSet = CharSet.Auto)]
|
|
public static extern void ImageList_EndDrag();
|
|
|
|
[DllImport("comctl32.dll", CharSet = CharSet.Auto)]
|
|
public static extern bool ImageList_DragEnter(IntPtr hwndLock, int x, int y);
|
|
|
|
[DllImport("comctl32.dll", CharSet = CharSet.Auto)]
|
|
public static extern bool ImageList_DragLeave(IntPtr hwndLock);
|
|
|
|
[DllImport("comctl32.dll", CharSet = CharSet.Auto)]
|
|
public static extern bool ImageList_DragShowNolock(bool fShow);
|
|
static DragHelper()
|
|
{
|
|
InitCommonControls();
|
|
}
|
|
}
|
|
#endregion
|
|
}
|