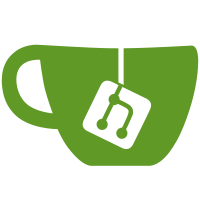
Fixed Find and Replace logic to keep it from getting into an infinite loop Used new CSLA code to hanndle deletion of procedures with external transitions New Enhanced Document properties
388 lines
12 KiB
C#
388 lines
12 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.ComponentModel;
|
|
using System.Data;
|
|
using System.Drawing;
|
|
using System.Text;
|
|
using System.Windows.Forms;
|
|
using VEPROMS.CSLA.Library;
|
|
|
|
namespace Volian.Controls.Library
|
|
{
|
|
public partial class FindReplace : DevComponents.DotNetBar.Office2007Form
|
|
{
|
|
private bool doingfind = false;
|
|
private bool findingbookmarks = false;
|
|
private bool _FoundIt = false;
|
|
public bool FoundIt
|
|
{
|
|
get { return _FoundIt; }
|
|
set { _FoundIt = value; }
|
|
}
|
|
private bool IsFound
|
|
{
|
|
get
|
|
{
|
|
if (!cbxCaseSensitive.Checked)
|
|
return MyEditItem.MyStepRTB.SelectedText.ToUpper() == cmboFindText.Text.ToUpper();
|
|
else
|
|
return MyEditItem.MyStepRTB.SelectedText == cmboFindText.Text;
|
|
}
|
|
}
|
|
//private bool _offsetStartPos = false;
|
|
private EditItem _MyEditItem;
|
|
public EditItem MyEditItem
|
|
{
|
|
get { return _MyEditItem; }
|
|
set
|
|
{
|
|
_MyEditItem = value;
|
|
if (_MyEditItem == null) return;
|
|
_MyDocVersion = _MyEditItem.MyItemInfo.MyProcedure.ActiveParent as DocVersionInfo;
|
|
SetupContextMenu();
|
|
//_offsetStartPos = false; // in a different rtf box, don't offset find position first time around
|
|
}
|
|
}
|
|
private DocVersionInfo _MyDocVersion;
|
|
public DocVersionInfo Mydocversion
|
|
{
|
|
get { return _MyDocVersion; }
|
|
set
|
|
{
|
|
_MyDocVersion = value;
|
|
//if (_MyDocVersion != null)
|
|
//{
|
|
// if (_MyDocVersion.DocVersionAssociationCount > 0)
|
|
// {
|
|
// MyROFSTLookup = _MyDocVersion.DocVersionAssociations[0].MyROFst.ROFSTLookup;
|
|
// _MyRODbID = _MyDocVersion.DocVersionAssociations[0].MyROFst.RODbID;
|
|
// }
|
|
//}
|
|
}
|
|
}
|
|
private DisplayBookMarks _myDisplayBookMarks;
|
|
|
|
public DisplayBookMarks MyDisplayBookMarks
|
|
{
|
|
get { return _myDisplayBookMarks; }
|
|
set { _myDisplayBookMarks = value; }
|
|
}
|
|
|
|
private bool _InApproved;
|
|
|
|
public bool InApproved
|
|
{
|
|
get { return _InApproved; }
|
|
set { _InApproved = value; }
|
|
}
|
|
|
|
public FindReplace()
|
|
{
|
|
InitializeComponent();
|
|
LoadFindReplaceTextListBox();
|
|
//if (dvi != null && dvi.VersionType > 127)
|
|
// InApproved = false; // in approved
|
|
cmbScope.SelectedIndex = 0;
|
|
btnBookMrkAll.Enabled = false;
|
|
btnFindNext.Enabled = false;
|
|
btnReplace.Enabled = false;
|
|
btnRplAll.Enabled = false;
|
|
//if (MyRTBItem.MyStepRTB.SelectionLength > 0)
|
|
// cmboFindText.Text = MyRTBItem.MyStepRTB.SelectedText;
|
|
cmboFindText.Focus();
|
|
//SetupContextMenu();
|
|
}
|
|
private void LoadFindReplaceTextListBox()
|
|
{
|
|
// Setup cmboFindText Combo box
|
|
cmboFindText.Items.Clear();
|
|
if (Properties.Settings.Default["FindTextList"] != null && Properties.Settings.Default.FindTextList.Count > 0)
|
|
{
|
|
foreach (string str in Properties.Settings.Default.FindTextList)
|
|
cmboFindText.Items.Add(str);
|
|
}
|
|
// Setup cmboReplaceText Combo box
|
|
cmboReplaceText.Items.Clear();
|
|
if (Properties.Settings.Default["ReplaceTextList"] != null && Properties.Settings.Default.ReplaceTextList.Count > 0)
|
|
{
|
|
foreach (string str in Properties.Settings.Default.ReplaceTextList)
|
|
cmboReplaceText.Items.Add(str);
|
|
}
|
|
}
|
|
|
|
//public FindReplace(RTBItem stpitm)
|
|
//{
|
|
// MyRTBItem = stpitm;
|
|
// DocVersionInfo dvi = MyRTBItem.MyItemInfo.MyProcedure.ActiveParent as DocVersionInfo;
|
|
// InitializeComponent();
|
|
// if (dvi != null && dvi.VersionType > 127)
|
|
// InApproved = false; // in approved
|
|
// cmbScope.SelectedIndex = 0;
|
|
// btnBookMrkAll.Enabled = false;
|
|
// btnFindNext.Enabled = false;
|
|
// btnReplace.Enabled = false;
|
|
// btnRplAll.Enabled = false;
|
|
// if (MyRTBItem.MyStepRTB.SelectionLength > 0)
|
|
// cmboFindText.Text = MyRTBItem.MyStepRTB.SelectedText;
|
|
// cmboFindText.Focus();
|
|
//}
|
|
|
|
|
|
private void btnReplace_Click(object sender, EventArgs e)
|
|
{
|
|
if (!IsFound)
|
|
btnFindNext_Click(sender, e);
|
|
else
|
|
{
|
|
MyEditItem.ReplaceText(cmboReplaceText.Text, cmboFindText.Text, cbxCaseSensitive.Checked, cbxWholeWord.Checked, cbxReverse.Checked, false, null);
|
|
}
|
|
}
|
|
|
|
private void btnRplAll_Click(object sender, EventArgs e)
|
|
{
|
|
if (!IsFound)
|
|
btnFindNext_Click(sender, e);
|
|
else
|
|
{
|
|
btnReplace_Click(sender, e);
|
|
btnFindNext_Click(sender, e);
|
|
}
|
|
}
|
|
|
|
private void btnBookMrkAll_Click(object sender, EventArgs e)
|
|
{
|
|
findingbookmarks = true;
|
|
if (!IsFound) btnFindNext_Click(sender, e);
|
|
FoundIt = true;
|
|
while (FoundIt) // found is set in btnFindNext_Click()
|
|
{
|
|
MyDisplayBookMarks.AddBookMark(MyEditItem.MyItemInfo);
|
|
btnFindNext_Click(sender, e);
|
|
}
|
|
StepPanelTabDisplayEventArgs args = new StepPanelTabDisplayEventArgs("Bookmarks");
|
|
MyEditItem.MyStepPanel.OnTabDisplay(sender, args);
|
|
findingbookmarks = false;
|
|
}
|
|
private void btnFndRplDone_Click(object sender, EventArgs e)
|
|
{
|
|
//this.Close();
|
|
doingfind = false;
|
|
this.Visible = false;
|
|
}
|
|
private void btnFindNext_Click(object sender, EventArgs e)
|
|
{
|
|
AddToComboLists();
|
|
doingfind = true;
|
|
while (!MyEditItem.FindText(cmboFindText.Text, cbxCaseSensitive.Checked, cbxWholeWord.Checked, cbxReverse.Checked))
|
|
{
|
|
ItemInfo next = (cbxReverse.Checked) ? MyEditItem.MyItemInfo.SearchPrev : MyEditItem.MyItemInfo.SearchNext;
|
|
|
|
while ((next != null) && !next.IsSection && !next.MyContent.Text.ToUpper().Contains(cmboFindText.Text.ToUpper()))
|
|
next = (cbxReverse.Checked) ? next.SearchPrev : next.SearchNext;
|
|
if ((next == null) || next.IsSection)
|
|
{
|
|
if (!findingbookmarks)
|
|
{
|
|
if (cbxReverse.Checked)
|
|
MessageBox.Show(this, "Not Found - From Here to Beginning of Section", "Find/Replace");
|
|
else
|
|
MessageBox.Show(this, "Not Found - From Here to End of Section", "Find/Replace");
|
|
}
|
|
FoundIt = false;
|
|
return;
|
|
}
|
|
|
|
MyEditItem.MyStepPanel.MyStepTabPanel.MyDisplayTabControl.OpenItem(next);
|
|
if (cbxReverse.Checked)
|
|
MyEditItem.PositionToEnd();
|
|
if (next.IsTable && !cbxReverse.Checked) MyEditItem.PositionToStart();
|
|
}
|
|
if (MyEditItem.MyStepRTB != null && !MyEditItem.MyStepRTB.Visible)
|
|
{
|
|
MyEditItem.MyStepRTB.Visible = true;
|
|
MyEditItem.MyStepRTB.Focus();
|
|
}
|
|
FoundIt = true;
|
|
}
|
|
private bool FindNextText(ItemInfo next)
|
|
{
|
|
if (next.MyContent.MyGrid!=null) return true;
|
|
return next.MyContent.Text.ToUpper().Contains(cmboFindText.Text.ToUpper());
|
|
}
|
|
private void AddToComboLists()
|
|
{
|
|
bool hastext = this.cmboFindText.Text.Length > 0;
|
|
if (hastext && !cmboFindText.Items.Contains(cmboFindText.Text))
|
|
{
|
|
if (cmboFindText.Items.Count >= 10)
|
|
cmboFindText.Items.RemoveAt(9);
|
|
cmboFindText.Items.Insert(0, cmboFindText.Text);//.Add(cmboFindText.Text);
|
|
}
|
|
hastext = this.cmboReplaceText.Text.Length > 0;
|
|
if (hastext && btnReplace.Enabled && !cmboReplaceText.Items.Contains(cmboReplaceText.Text))
|
|
{
|
|
if (cmboReplaceText.Items.Count >= 10)
|
|
cmboReplaceText.Items.RemoveAt(9);
|
|
cmboReplaceText.Items.Insert(0, cmboReplaceText.Text);
|
|
}
|
|
}
|
|
private void cmboFindText_TextChanged(object sender, EventArgs e)
|
|
{
|
|
bool hastext = this.cmboFindText.Text.Length > 0;
|
|
btnFindNext.Enabled = hastext;
|
|
btnBookMrkAll.Enabled = hastext;
|
|
btnReplace.Enabled = btnRplAll.Enabled = hastext && !InApproved;
|
|
}
|
|
|
|
private void cmboReplaceText_TextChanged(object sender, EventArgs e)
|
|
{
|
|
btnReplace.Enabled = btnRplAll.Enabled = !InApproved && cmboFindText.Text.Length > 0;
|
|
}
|
|
|
|
public void PerformFindNext()
|
|
{
|
|
btnFindNext.PerformClick();
|
|
}
|
|
|
|
private void tabFind_Click(object sender, EventArgs e)
|
|
{
|
|
lblRplTxt.Visible = false;
|
|
cmboReplaceText.Visible = false;
|
|
btnReplace.Visible = false;
|
|
btnRplAll.Visible = false;
|
|
}
|
|
|
|
private void tabReplace_Click(object sender, EventArgs e)
|
|
{
|
|
lblRplTxt.Visible = true;
|
|
cmboReplaceText.Visible = true;
|
|
btnReplace.Visible = true;
|
|
btnRplAll.Visible = true;
|
|
}
|
|
|
|
private void FindReplace_FormClosing(object sender, FormClosingEventArgs e)
|
|
{
|
|
btnFndRplDone_Click(sender, e);
|
|
e.Cancel = true; // cancel the form close event - the Done_Click() will hide it instead
|
|
}
|
|
|
|
private void cmboFindText_Leave(object sender, EventArgs e)
|
|
{
|
|
//bool hastext = this.cmboFindText.Text.Length > 0;
|
|
//if (hastext && !cmboFindText.Items.Contains(cmboFindText.Text))
|
|
//{
|
|
// if (cmboFindText.Items.Count >= 10)
|
|
// cmboFindText.Items.RemoveAt(9);
|
|
// cmboFindText.Items.Insert(0, cmboFindText.Text);//.Add(cmboFindText.Text);
|
|
//}
|
|
}
|
|
|
|
private void btnCmCut_Click(object sender, EventArgs e)
|
|
{
|
|
Clipboard.Clear();
|
|
DataObject myDO = new DataObject(DataFormats.Text, cmboReplaceText.Focused ? cmboReplaceText.SelectedText : cmboFindText.SelectedText);
|
|
Clipboard.SetDataObject(myDO);
|
|
// Need to check which combo box activated the context menu so that we know where to take/put selected text
|
|
if (cmboFindText.Focused)
|
|
cmboFindText.SelectedText = "";
|
|
else if (cmboReplaceText.Focused)
|
|
cmboReplaceText.SelectedText = "";
|
|
}
|
|
|
|
private void btnCmCopy_Click(object sender, EventArgs e)
|
|
{
|
|
// Need to check which combo box activated the context menu so that we know where to take/put selected text
|
|
DataObject myDO = new DataObject(DataFormats.Text, cmboReplaceText.Focused ? cmboReplaceText.SelectedText : cmboFindText.SelectedText);
|
|
if (cmboFindText.Focused || cmboReplaceText.Focused)
|
|
{
|
|
Clipboard.Clear();
|
|
Clipboard.SetDataObject(myDO);
|
|
}
|
|
}
|
|
|
|
private void btnCmPaste_Click(object sender, EventArgs e)
|
|
{
|
|
// Need to check which combo box activated the context menu so that we know where to take/put selected text
|
|
IDataObject myDO = Clipboard.GetDataObject();
|
|
if (myDO.GetDataPresent(DataFormats.Rtf))
|
|
{
|
|
RichTextBox rtb = new RichTextBox();
|
|
rtb.SelectedRtf = ItemInfo.StripLinks(myDO.GetData(DataFormats.Rtf).ToString());
|
|
if (cmboReplaceText.Focused)
|
|
cmboReplaceText.SelectedText = rtb.Text;
|
|
else if (cmboFindText.Focused)
|
|
cmboFindText.SelectedText = rtb.Text;
|
|
}
|
|
else if (myDO.GetDataPresent(DataFormats.Text))
|
|
if (cmboReplaceText.Focused)
|
|
cmboReplaceText.SelectedText = Clipboard.GetText();
|
|
else if (cmboFindText.Focused)
|
|
cmboFindText.SelectedText = Clipboard.GetText();
|
|
}
|
|
|
|
private void btnCmHardSp_Click(object sender, EventArgs e)
|
|
{
|
|
// We use \u00A0 to represent a hard space because it show in the search text field as a blank
|
|
// It will get translated to a real hard space character prior to searching
|
|
if (cmboReplaceText.Focused)
|
|
cmboReplaceText.SelectedText = "\u00A0";
|
|
else if (cmboFindText.Focused)
|
|
cmboFindText.SelectedText = "\u00A0";
|
|
}
|
|
private void btnSym_Click(object sender, EventArgs e)
|
|
{
|
|
DevComponents.DotNetBar.ButtonItem b = (DevComponents.DotNetBar.ButtonItem)sender;
|
|
if (cmboReplaceText.Focused)
|
|
cmboReplaceText.SelectedText = (string)b.Text;
|
|
else if (cmboFindText.Focused)
|
|
cmboFindText.SelectedText = (string)b.Text;
|
|
}
|
|
private void SetupContextMenu()
|
|
{
|
|
galSymbols.SubItems.Clear();
|
|
if (_MyDocVersion != null)
|
|
{
|
|
FormatData fmtdata = _MyDocVersion.ActiveFormat.PlantFormat.FormatData;
|
|
SymbolList sl = fmtdata.SymbolList;
|
|
if (sl == null || sl.Count <= 0)
|
|
{
|
|
MessageBox.Show(this, "No symbols are available, check with administrator");
|
|
return;
|
|
}
|
|
foreach (Symbol sym in sl)
|
|
{
|
|
DevComponents.DotNetBar.ButtonItem btnCM = new DevComponents.DotNetBar.ButtonItem();
|
|
btnCM.Text = string.Format("{0}", (char)sym.Unicode);
|
|
// to name button use unicode rather than desc, desc may have spaces or odd chars
|
|
btnCM.Name = "btnCM" + sym.Unicode.ToString();
|
|
btnCM.Tooltip = sym.Desc;
|
|
btnCM.Tag = string.Format(@"{0}", sym.Unicode);
|
|
btnCM.FontBold = true;
|
|
btnCM.Click += new System.EventHandler(btnSym_Click);
|
|
galSymbols.SubItems.Add(btnCM);
|
|
}
|
|
}
|
|
}
|
|
|
|
private void FindReplace_Activated(object sender, EventArgs e)
|
|
{
|
|
if (!doingfind)
|
|
{
|
|
string find = MyEditItem.SelectedTextForFind;
|
|
if (find != null) cmboFindText.Text = find;
|
|
}
|
|
}
|
|
public void ToggleReplaceTab(E_ViewMode vm)
|
|
{
|
|
if (vm == E_ViewMode.View)
|
|
{
|
|
if (tabReplace.IsSelected)
|
|
tabFind.PerformClick();
|
|
tabReplace.Visible = false;
|
|
}
|
|
else
|
|
tabReplace.Visible = true;
|
|
}
|
|
|
|
}
|
|
} |