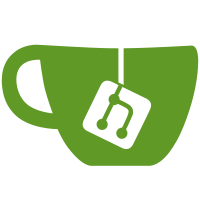
Added Ordinal to vefn_SiblingItems Added Stored Procedure vesp_SortProcedures to sort procedures within a DocVersion Added Error Handler in Range.Text Corrected logic in FindRO to return a null when an RO is not found.
1255 lines
36 KiB
C#
1255 lines
36 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using System.Reflection;
|
|
|
|
namespace LBWordLibrary
|
|
{
|
|
public abstract partial class LBComObject
|
|
{
|
|
private Object _Item;
|
|
internal Object Item
|
|
{
|
|
get { return _Item; }
|
|
set
|
|
{
|
|
_Item = value;
|
|
if (value != null) _MyType = _Item.GetType();
|
|
}
|
|
}
|
|
private Type _MyType;
|
|
public Type MyType
|
|
{
|
|
get { return _MyType; }
|
|
set { _MyType = value; }
|
|
}
|
|
protected LBComObject() { }
|
|
protected LBComObject(string ProgID)
|
|
{
|
|
Type objClassType;
|
|
objClassType = Type.GetTypeFromProgID(ProgID);
|
|
Item = Activator.CreateInstance(objClassType);
|
|
}
|
|
protected LBComObject(Object item)
|
|
{
|
|
Item = item;
|
|
}
|
|
private Object DoInvokeMember(string name, Object[] parameters, BindingFlags bf, Binder b)
|
|
{
|
|
try
|
|
{
|
|
return _MyType.InvokeMember(name, bf, b, _Item, parameters);
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new Exception(string.Format("LBComObject.DoInvokeMember {0}.{1}", _MyType.Name, name), ex);
|
|
}
|
|
return null;
|
|
}
|
|
protected void SetProperty(string propertyName, params Object[] parameters)
|
|
{
|
|
DoInvokeMember(propertyName, parameters, BindingFlags.SetProperty, null);
|
|
}
|
|
protected Object GetProperty(string propertyName)
|
|
{
|
|
return DoInvokeMember(propertyName, null, BindingFlags.GetProperty, null);
|
|
}
|
|
protected Object GetProperty(string propertyName, params Object[] parameters)
|
|
{
|
|
return DoInvokeMember(propertyName, parameters, BindingFlags.GetProperty, null);
|
|
}
|
|
protected Object InvokeMethod(string methodName, params Object[] parameters)
|
|
{
|
|
if (parameters != null)
|
|
FixParameters(parameters);
|
|
return DoInvokeMember(methodName, parameters, BindingFlags.InvokeMethod, null);
|
|
}
|
|
private void FixParameters(object[] parameters)
|
|
{
|
|
// Loop through parameters checking to make sure that none of them are LBComObjects
|
|
for (int i = 0; i < parameters.Length; i++)
|
|
if (parameters[i] is LBComObject)
|
|
parameters[i] = (parameters[i] as LBComObject).Item;
|
|
}
|
|
protected Object InvokeMethod(string methodName)
|
|
{
|
|
return InvokeMethod(methodName, null);
|
|
}
|
|
}
|
|
public class LBComObjectList<TList, TItem> : LBComObject
|
|
where TList : LBComObjectList<TList, TItem>
|
|
where TItem : LBComObject, new()
|
|
{
|
|
//public new(Object item):base(item){}
|
|
public TItem Add()
|
|
{
|
|
TItem tmp = new TItem();
|
|
tmp.Item = InvokeMethod("Add");
|
|
return tmp;
|
|
}
|
|
//public TItem this[int item]
|
|
//{
|
|
// get
|
|
// {
|
|
// TItem tmp = new TItem();
|
|
// tmp.Item = GetProperty("Item", item);
|
|
// return tmp;
|
|
// }
|
|
//}
|
|
}
|
|
public partial class LBApplicationClass : LBComObject
|
|
{
|
|
public LBApplicationClass() : base("Word.Application") { }
|
|
public LBApplicationClass(Object item) : base(item) { }
|
|
public Boolean Visible
|
|
{
|
|
get { return (GetProperty("Visible") as Boolean? ?? false); }
|
|
set { SetProperty("Visible", value); }
|
|
}
|
|
public LBDocuments Documents
|
|
{
|
|
get { return new LBDocuments(GetProperty("Documents")); }
|
|
}
|
|
public LBSelection Selection
|
|
{
|
|
get { return new LBSelection(GetProperty("Selection")); }
|
|
}
|
|
public LBDocumentClass ActiveDocument
|
|
{
|
|
get { return new LBDocumentClass(GetProperty("ActiveDocument")); }
|
|
}
|
|
public LBWindow ActiveWindow
|
|
{
|
|
get { return new LBWindow(GetProperty("ActiveWindow")); }
|
|
}
|
|
public String Version
|
|
{
|
|
get { return (GetProperty("Version").ToString()); }
|
|
}
|
|
public String ActivePrinter
|
|
{
|
|
get { return (GetProperty("ActivePrinter").ToString()); }
|
|
set { SetProperty("ActivePrinter", value); }
|
|
}
|
|
public int BackgroundPrintingStatus
|
|
{
|
|
get { return (GetProperty("BackgroundPrintingStatus") as int? ?? 0); }
|
|
}
|
|
public LBWdWindowState WindowState
|
|
{
|
|
get { return (LBWdWindowState)GetProperty("WindowState"); }
|
|
set { SetProperty("WindowState", value); }
|
|
}
|
|
public int BackgroundSavingStatus
|
|
{
|
|
get { return (GetProperty("BackgroundSavingStatus") as int? ?? 0); }
|
|
}
|
|
public void Quit()
|
|
{
|
|
InvokeMethod("Quit", Missing.Value, Missing.Value, Missing.Value);
|
|
}
|
|
public void Quit(object SaveChanges, object OriginalFormat, object RouteDocument)
|
|
{
|
|
InvokeMethod("Quit", SaveChanges, OriginalFormat, RouteDocument);
|
|
}
|
|
public void PrintOut()
|
|
{
|
|
InvokeMethod("PrintOut", Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value);
|
|
}
|
|
public void PrintOut(object Background, object Append, object Range, object OutputFileName, object From, object To, object Item, object Copies, object Pages, object PageType, object PrintToFile, object Collate, object FileName, object ActivePrinterMacGX, object ManualDuplexPrint, object PrintZoomColumn, object PrintZoomRow, object PrintZoomPaperWidth, object PrintZoomPaperHeight)
|
|
{
|
|
InvokeMethod("PrintOut", Background, Append, Range, OutputFileName, From, To, Item, Copies, Pages, PageType, PrintToFile, Collate, FileName, ActivePrinterMacGX, ManualDuplexPrint, PrintZoomColumn, PrintZoomRow, PrintZoomPaperWidth, PrintZoomPaperHeight);
|
|
}
|
|
public void Activate()
|
|
{
|
|
InvokeMethod("Activate");
|
|
}
|
|
}
|
|
public partial class LBDocuments : LBComObjectList<LBDocuments, LBDocumentClass> /* Collection */
|
|
{
|
|
public LBDocuments(Object item)
|
|
{
|
|
Item = item;
|
|
}
|
|
public LBDocumentClass Open(object FileName)
|
|
{
|
|
return new LBDocumentClass(InvokeMethod("Open", FileName, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value));
|
|
}
|
|
public LBDocumentClass Open(object FileName, object ConfirmConversions, object ReadOnly, object AddToRecentFiles, object PasswordDocument, object PasswordTemplate, object Revert, object WritePasswordDocument, object WritePasswordTemplate, object Format, object Encoding, object Visible, object OpenAndRepair, object DocumentDirection, object NoEncodingDialog, object XMLTransform)
|
|
{
|
|
return new LBDocumentClass(InvokeMethod("Open", FileName, ConfirmConversions, ReadOnly, AddToRecentFiles, PasswordDocument, PasswordTemplate, Revert, WritePasswordDocument, WritePasswordTemplate, Format, Encoding, Visible, OpenAndRepair, DocumentDirection, NoEncodingDialog, XMLTransform));
|
|
}
|
|
}
|
|
public partial class LBSelection : LBComObject
|
|
{
|
|
public LBSelection() { }
|
|
public LBSelection(Object item) : base(item) { }
|
|
public LBRange Range
|
|
{
|
|
get { return new LBRange(GetProperty("Range")); }
|
|
}
|
|
public LBParagraphFormatClass ParagraphFormat
|
|
{
|
|
get { return new LBParagraphFormatClass(GetProperty("ParagraphFormat")); }
|
|
}
|
|
public String Text
|
|
{
|
|
get { return (GetProperty("Text").ToString()); }
|
|
set { SetProperty("Text", value); }
|
|
}
|
|
public LBWdSelectionType Type
|
|
{
|
|
get { return (LBWdSelectionType)GetProperty("Type"); }
|
|
}
|
|
public LBFind Find
|
|
{
|
|
get { return new LBFind(GetProperty("Find")); }
|
|
}
|
|
public LBFontClass Font
|
|
{
|
|
get { return new LBFontClass(GetProperty("Font")); }
|
|
}
|
|
public int End
|
|
{
|
|
get { return (GetProperty("End") as int? ?? 0); }
|
|
set { SetProperty("End", value); }
|
|
}
|
|
public int Start
|
|
{
|
|
get { return (GetProperty("Start") as int? ?? 0); }
|
|
set { SetProperty("Start", value); }
|
|
}
|
|
public LBInlineShapes InlineShapes
|
|
{
|
|
get { return new LBInlineShapes(GetProperty("InlineShapes")); }
|
|
}
|
|
public LBParagraphs Paragraphs
|
|
{
|
|
get { return new LBParagraphs(GetProperty("Paragraphs")); }
|
|
}
|
|
public void WholeStory()
|
|
{
|
|
InvokeMethod("WholeStory");
|
|
}
|
|
public int MoveStart()
|
|
{
|
|
return InvokeMethod("MoveStart", Missing.Value, Missing.Value) as int? ?? 0;
|
|
}
|
|
public int MoveStart(object Unit, object Count)
|
|
{
|
|
return InvokeMethod("MoveStart", Unit, Count) as int? ?? 0;
|
|
}
|
|
public int MoveEnd()
|
|
{
|
|
return InvokeMethod("MoveEnd", Missing.Value, Missing.Value) as int? ?? 0;
|
|
}
|
|
public int MoveEnd(object Unit, object Count)
|
|
{
|
|
return InvokeMethod("MoveEnd", Unit, Count) as int? ?? 0;
|
|
}
|
|
public void Copy()
|
|
{
|
|
InvokeMethod("Copy");
|
|
}
|
|
public int EndKey()
|
|
{
|
|
return InvokeMethod("EndKey", Missing.Value, Missing.Value) as int? ?? 0;
|
|
}
|
|
public int EndKey(object Unit, object Extend)
|
|
{
|
|
return InvokeMethod("EndKey", Unit, Extend) as int? ?? 0;
|
|
}
|
|
public void TypeText(string Text)
|
|
{
|
|
InvokeMethod("TypeText", Text);
|
|
}
|
|
public int EndOf()
|
|
{
|
|
return InvokeMethod("EndOf", Missing.Value, Missing.Value) as int? ?? 0;
|
|
}
|
|
public int EndOf(object Unit, object Extend)
|
|
{
|
|
return InvokeMethod("EndOf", Unit, Extend) as int? ?? 0;
|
|
}
|
|
public LBRange GoTo()
|
|
{
|
|
return new LBRange(InvokeMethod("GoTo", Missing.Value, Missing.Value, Missing.Value, Missing.Value));
|
|
}
|
|
public LBRange GoTo(object What, object Which, object Count, object Name)
|
|
{
|
|
return new LBRange(InvokeMethod("GoTo", What, Which, Count, Name));
|
|
}
|
|
}
|
|
public partial class LBDocumentClass : LBComObject
|
|
{
|
|
public LBDocumentClass() { }
|
|
public LBDocumentClass(Object item) : base(item) { }
|
|
public LBApplicationClass Application
|
|
{
|
|
get { return new LBApplicationClass(GetProperty("Application")); }
|
|
}
|
|
public int SaveFormat
|
|
{
|
|
get { return (GetProperty("SaveFormat") as int? ?? 0); }
|
|
}
|
|
public String FullName
|
|
{
|
|
get { return (GetProperty("FullName").ToString()); }
|
|
}
|
|
public LBShapes Shapes
|
|
{
|
|
get { return new LBShapes(GetProperty("Shapes")); }
|
|
}
|
|
public LBWindow ActiveWindow
|
|
{
|
|
get { return new LBWindow(GetProperty("ActiveWindow")); }
|
|
}
|
|
public LBPageSetup PageSetup
|
|
{
|
|
get { return new LBPageSetup(GetProperty("PageSetup")); }
|
|
}
|
|
public Boolean Saved
|
|
{
|
|
get { return (GetProperty("Saved") as Boolean? ?? false); }
|
|
set { SetProperty("Saved", value); }
|
|
}
|
|
public void SaveAs2000()
|
|
{
|
|
InvokeMethod("SaveAs2000", Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value);
|
|
}
|
|
public void SaveAs2000(object FileName, object FileFormat, object LockComments, object Password, object AddToRecentFiles, object WritePassword, object ReadOnlyRecommended, object EmbedTrueTypeFonts, object SaveNativePictureFormat, object SaveFormsData, object SaveAsAOCELetter)
|
|
{
|
|
InvokeMethod("SaveAs2000", FileName, FileFormat, LockComments, Password, AddToRecentFiles, WritePassword, ReadOnlyRecommended, EmbedTrueTypeFonts, SaveNativePictureFormat, SaveFormsData, SaveAsAOCELetter);
|
|
}
|
|
public void SaveAs()
|
|
{
|
|
InvokeMethod("SaveAs", Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value);
|
|
}
|
|
public void SaveAs(object FileName, object FileFormat, object LockComments, object Password, object AddToRecentFiles, object WritePassword, object ReadOnlyRecommended, object EmbedTrueTypeFonts, object SaveNativePictureFormat, object SaveFormsData, object SaveAsAOCELetter, object Encoding, object InsertLineBreaks, object AllowSubstitutions, object LineEnding, object AddBiDiMarks)
|
|
{
|
|
InvokeMethod("SaveAs", FileName, FileFormat, LockComments, Password, AddToRecentFiles, WritePassword, ReadOnlyRecommended, EmbedTrueTypeFonts, SaveNativePictureFormat, SaveFormsData, SaveAsAOCELetter, Encoding, InsertLineBreaks, AllowSubstitutions, LineEnding, AddBiDiMarks);
|
|
}
|
|
public void Close()
|
|
{
|
|
InvokeMethod("Close", Missing.Value, Missing.Value, Missing.Value);
|
|
}
|
|
public void Close(object SaveChanges, object OriginalFormat, object RouteDocument)
|
|
{
|
|
InvokeMethod("Close", SaveChanges, OriginalFormat, RouteDocument);
|
|
}
|
|
public void ExportAsFixedFormat(string OutputFileName, LBWdExportFormat ExportFormat)
|
|
{
|
|
InvokeMethod("ExportAsFixedFormat", OutputFileName, ExportFormat, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value);
|
|
}
|
|
public void ExportAsFixedFormat(string OutputFileName, LBWdExportFormat ExportFormat, Boolean OpenAfterExport, LBWdExportOptimizeFor OptimizeFor, LBWdExportRange Range, int From, int To, LBWdExportItem Item, Boolean IncludeDocProps, Boolean KeepIRM, LBWdExportCreateBookmarks CreateBookmarks, Boolean DocStructureTags, Boolean BitmapMissingFonts, Boolean UseISO19005_1, object FixedFormatExtClassPtr)
|
|
{
|
|
InvokeMethod("ExportAsFixedFormat", OutputFileName, ExportFormat, OpenAfterExport, OptimizeFor, Range, From, To, Item, IncludeDocProps, KeepIRM, CreateBookmarks, DocStructureTags, BitmapMissingFonts, UseISO19005_1, FixedFormatExtClassPtr);
|
|
}
|
|
public LBRange Range()
|
|
{
|
|
return new LBRange(InvokeMethod("Range", Missing.Value, Missing.Value));
|
|
}
|
|
public LBRange Range(object Start, object End)
|
|
{
|
|
return new LBRange(InvokeMethod("Range", Start, End));
|
|
}
|
|
public Boolean Undo()
|
|
{
|
|
return InvokeMethod("Undo", Missing.Value) as Boolean? ?? false;
|
|
}
|
|
public Boolean Undo(object Times)
|
|
{
|
|
return InvokeMethod("Undo", Times) as Boolean? ?? false;
|
|
}
|
|
}
|
|
public partial class LBWindow : LBComObject
|
|
{
|
|
public LBWindow() { }
|
|
public LBWindow(Object item) : base(item) { }
|
|
public LBPane ActivePane
|
|
{
|
|
get { return new LBPane(GetProperty("ActivePane")); }
|
|
}
|
|
public LBView View
|
|
{
|
|
get { return new LBView(GetProperty("View")); }
|
|
}
|
|
}
|
|
public enum LBWdWindowState
|
|
{
|
|
wdWindowStateNormal = 0,
|
|
wdWindowStateMaximize = 1,
|
|
wdWindowStateMinimize = 2
|
|
}
|
|
public partial class LBRange : LBComObject
|
|
{
|
|
public LBRange() { }
|
|
public LBRange(Object item) : base(item) { }
|
|
public LBFontClass Font
|
|
{
|
|
get { return new LBFontClass(GetProperty("Font")); }
|
|
}
|
|
public LBWdColorIndex HighlightColorIndex
|
|
{
|
|
get { return (LBWdColorIndex)GetProperty("HighlightColorIndex"); }
|
|
set { SetProperty("HighlightColorIndex", value); }
|
|
}
|
|
public LBParagraphFormatClass ParagraphFormat
|
|
{
|
|
get { return new LBParagraphFormatClass(GetProperty("ParagraphFormat")); }
|
|
}
|
|
public LBParagraphs Paragraphs
|
|
{
|
|
get { return new LBParagraphs(GetProperty("Paragraphs")); }
|
|
}
|
|
public int End
|
|
{
|
|
get { return (GetProperty("End") as int? ?? 0); }
|
|
set { SetProperty("End", value); }
|
|
}
|
|
public int Start
|
|
{
|
|
get { return (GetProperty("Start") as int? ?? 0); }
|
|
set { SetProperty("Start", value); }
|
|
}
|
|
public String Text
|
|
{
|
|
get
|
|
{
|
|
try
|
|
{
|
|
return (GetProperty("Text").ToString());
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
return "";
|
|
}
|
|
}
|
|
set { SetProperty("Text", value); }
|
|
}
|
|
public LBRange GoTo()
|
|
{
|
|
return new LBRange(InvokeMethod("GoTo", Missing.Value, Missing.Value, Missing.Value, Missing.Value));
|
|
}
|
|
public LBRange GoTo(object What, object Which, object Count, object Name)
|
|
{
|
|
return new LBRange(InvokeMethod("GoTo", What, Which, Count, Name));
|
|
}
|
|
}
|
|
public partial class LBParagraphFormatClass : LBComObject
|
|
{
|
|
public LBParagraphFormatClass() { }
|
|
public LBParagraphFormatClass(Object item) : base(item) { }
|
|
public LBWdLineSpacing LineSpacingRule
|
|
{
|
|
get { return (LBWdLineSpacing)GetProperty("LineSpacingRule"); }
|
|
set { SetProperty("LineSpacingRule", value); }
|
|
}
|
|
public float LineSpacing
|
|
{
|
|
get { return (GetProperty("LineSpacing") as float? ?? 0); }
|
|
set { SetProperty("LineSpacing", value); }
|
|
}
|
|
public float SpaceAfter
|
|
{
|
|
get { return (GetProperty("SpaceAfter") as float? ?? 0); }
|
|
set { SetProperty("SpaceAfter", value); }
|
|
}
|
|
public int SpaceAfterAuto
|
|
{
|
|
get { return (GetProperty("SpaceAfterAuto") as int? ?? 0); }
|
|
set { SetProperty("SpaceAfterAuto", value); }
|
|
}
|
|
public float SpaceBefore
|
|
{
|
|
get { return (GetProperty("SpaceBefore") as float? ?? 0); }
|
|
set { SetProperty("SpaceBefore", value); }
|
|
}
|
|
public int SpaceBeforeAuto
|
|
{
|
|
get { return (GetProperty("SpaceBeforeAuto") as int? ?? 0); }
|
|
set { SetProperty("SpaceBeforeAuto", value); }
|
|
}
|
|
}
|
|
public enum LBWdSelectionType
|
|
{
|
|
wdNoSelection = 0,
|
|
wdSelectionIP = 1,
|
|
wdSelectionNormal = 2,
|
|
wdSelectionFrame = 3,
|
|
wdSelectionColumn = 4,
|
|
wdSelectionRow = 5,
|
|
wdSelectionBlock = 6,
|
|
wdSelectionInlineShape = 7,
|
|
wdSelectionShape = 8
|
|
}
|
|
public partial class LBFind : LBComObject
|
|
{
|
|
public LBFind() { }
|
|
public LBFind(Object item) : base(item) { }
|
|
public LBReplacement Replacement
|
|
{
|
|
get { return new LBReplacement(GetProperty("Replacement")); }
|
|
}
|
|
public Boolean Forward
|
|
{
|
|
get { return (GetProperty("Forward") as Boolean? ?? false); }
|
|
set { SetProperty("Forward", value); }
|
|
}
|
|
public LBWdFindWrap Wrap
|
|
{
|
|
get { return (LBWdFindWrap)GetProperty("Wrap"); }
|
|
set { SetProperty("Wrap", value); }
|
|
}
|
|
public Boolean Format
|
|
{
|
|
get { return (GetProperty("Format") as Boolean? ?? false); }
|
|
set { SetProperty("Format", value); }
|
|
}
|
|
public Boolean MatchWholeWord
|
|
{
|
|
get { return (GetProperty("MatchWholeWord") as Boolean? ?? false); }
|
|
set { SetProperty("MatchWholeWord", value); }
|
|
}
|
|
public Boolean MatchWildcards
|
|
{
|
|
get { return (GetProperty("MatchWildcards") as Boolean? ?? false); }
|
|
set { SetProperty("MatchWildcards", value); }
|
|
}
|
|
public Boolean MatchSoundsLike
|
|
{
|
|
get { return (GetProperty("MatchSoundsLike") as Boolean? ?? false); }
|
|
set { SetProperty("MatchSoundsLike", value); }
|
|
}
|
|
public Boolean MatchAllWordForms
|
|
{
|
|
get { return (GetProperty("MatchAllWordForms") as Boolean? ?? false); }
|
|
set { SetProperty("MatchAllWordForms", value); }
|
|
}
|
|
public String Text
|
|
{
|
|
get { return (GetProperty("Text").ToString()); }
|
|
set { SetProperty("Text", value); }
|
|
}
|
|
public Boolean MatchCase
|
|
{
|
|
get { return (GetProperty("MatchCase") as Boolean? ?? false); }
|
|
set { SetProperty("MatchCase", value); }
|
|
}
|
|
public Boolean Execute()
|
|
{
|
|
return InvokeMethod("Execute", Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value) as Boolean? ?? false;
|
|
}
|
|
public Boolean Execute(object FindText, object MatchCase, object MatchWholeWord, object MatchWildcards, object MatchSoundsLike, object MatchAllWordForms, object Forward, object Wrap, object Format, object ReplaceWith, object Replace, object MatchKashida, object MatchDiacritics, object MatchAlefHamza, object MatchControl)
|
|
{
|
|
return InvokeMethod("Execute", FindText, MatchCase, MatchWholeWord, MatchWildcards, MatchSoundsLike, MatchAllWordForms, Forward, Wrap, Format, ReplaceWith, Replace, MatchKashida, MatchDiacritics, MatchAlefHamza, MatchControl) as Boolean? ?? false;
|
|
}
|
|
public void ClearFormatting()
|
|
{
|
|
InvokeMethod("ClearFormatting");
|
|
}
|
|
}
|
|
public partial class LBFontClass : LBComObject
|
|
{
|
|
public LBFontClass() { }
|
|
public LBFontClass(Object item) : base(item) { }
|
|
public LBWdColor Color
|
|
{
|
|
get { return (LBWdColor)GetProperty("Color"); }
|
|
set { SetProperty("Color", value); }
|
|
}
|
|
public int Position
|
|
{
|
|
get { return (GetProperty("Position") as int? ?? 0); }
|
|
set { SetProperty("Position", value); }
|
|
}
|
|
public int Subscript
|
|
{
|
|
get { return (GetProperty("Subscript") as int? ?? 0); }
|
|
set { SetProperty("Subscript", value); }
|
|
}
|
|
public int Superscript
|
|
{
|
|
get { return (GetProperty("Superscript") as int? ?? 0); }
|
|
set { SetProperty("Superscript", value); }
|
|
}
|
|
public float Size
|
|
{
|
|
get { return (GetProperty("Size") as float? ?? 0); }
|
|
set { SetProperty("Size", value); }
|
|
}
|
|
public String Name
|
|
{
|
|
get { return (GetProperty("Name").ToString()); }
|
|
set { SetProperty("Name", value); }
|
|
}
|
|
}
|
|
public enum LBWdInformation
|
|
{
|
|
wdActiveEndAdjustedPageNumber = 1,
|
|
wdActiveEndSectionNumber = 2,
|
|
wdActiveEndPageNumber = 3,
|
|
wdNumberOfPagesInDocument = 4,
|
|
wdHorizontalPositionRelativeToPage = 5,
|
|
wdVerticalPositionRelativeToPage = 6,
|
|
wdHorizontalPositionRelativeToTextBoundary = 7,
|
|
wdVerticalPositionRelativeToTextBoundary = 8,
|
|
wdFirstCharacterColumnNumber = 9,
|
|
wdFirstCharacterLineNumber = 10,
|
|
wdFrameIsSelected = 11,
|
|
wdWithInTable = 12,
|
|
wdStartOfRangeRowNumber = 13,
|
|
wdEndOfRangeRowNumber = 14,
|
|
wdMaximumNumberOfRows = 15,
|
|
wdStartOfRangeColumnNumber = 16,
|
|
wdEndOfRangeColumnNumber = 17,
|
|
wdMaximumNumberOfColumns = 18,
|
|
wdZoomPercentage = 19,
|
|
wdSelectionMode = 20,
|
|
wdCapsLock = 21,
|
|
wdNumLock = 22,
|
|
wdOverType = 23,
|
|
wdRevisionMarking = 24,
|
|
wdInFootnoteEndnotePane = 25,
|
|
wdInCommentPane = 26,
|
|
wdInHeaderFooter = 28,
|
|
wdAtEndOfRowMarker = 31,
|
|
wdReferenceOfType = 32,
|
|
wdHeaderFooterType = 33,
|
|
wdInMasterDocument = 34,
|
|
wdInFootnote = 35,
|
|
wdInEndnote = 36,
|
|
wdInWordMail = 37,
|
|
wdInClipboard = 38
|
|
}
|
|
public partial class LBInlineShapes : LBComObjectList<LBInlineShapes, LBInlineShape> /* Collection */
|
|
{
|
|
public LBInlineShapes(Object item)
|
|
{
|
|
Item = item;
|
|
}
|
|
public LBInlineShape AddPicture(string FileName)
|
|
{
|
|
return new LBInlineShape(InvokeMethod("AddPicture", FileName, Missing.Value, Missing.Value, Missing.Value));
|
|
}
|
|
public LBInlineShape AddPicture(string FileName, object LinkToFile, object SaveWithDocument, object Range)
|
|
{
|
|
return new LBInlineShape(InvokeMethod("AddPicture", FileName, LinkToFile, SaveWithDocument, Range));
|
|
}
|
|
}
|
|
public partial class LBParagraphs : LBComObjectList<LBParagraphs, LBParagraph> /* Collection */
|
|
{
|
|
public LBParagraphs(Object item)
|
|
{
|
|
Item = item;
|
|
}
|
|
public int Count
|
|
{
|
|
get { return (GetProperty("Count") as int? ?? 0); }
|
|
}
|
|
public LBParagraph First
|
|
{
|
|
get { return new LBParagraph(GetProperty("First")); }
|
|
}
|
|
public LBParagraph Last
|
|
{
|
|
get { return new LBParagraph(GetProperty("Last")); }
|
|
}
|
|
public System.Object GetEnumerator()
|
|
{
|
|
return InvokeMethod("GetEnumerator");
|
|
}
|
|
}
|
|
public partial class LBShapes : LBComObjectList<LBShapes, LBShape> /* Collection */
|
|
{
|
|
public LBShapes(Object item)
|
|
{
|
|
Item = item;
|
|
}
|
|
public LBShape AddPicture(string FileName)
|
|
{
|
|
return new LBShape(InvokeMethod("AddPicture", FileName, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value, Missing.Value));
|
|
}
|
|
public LBShape AddPicture(string FileName, object LinkToFile, object SaveWithDocument, object Left, object Top, object Width, object Height, object Anchor)
|
|
{
|
|
return new LBShape(InvokeMethod("AddPicture", FileName, LinkToFile, SaveWithDocument, Left, Top, Width, Height, Anchor));
|
|
}
|
|
}
|
|
public partial class LBPageSetup : LBComObject
|
|
{
|
|
public LBPageSetup() { }
|
|
public LBPageSetup(Object item) : base(item) { }
|
|
public float BottomMargin
|
|
{
|
|
get { return (GetProperty("BottomMargin") as float? ?? 0); }
|
|
set { SetProperty("BottomMargin", value); }
|
|
}
|
|
public float LeftMargin
|
|
{
|
|
get { return (GetProperty("LeftMargin") as float? ?? 0); }
|
|
set { SetProperty("LeftMargin", value); }
|
|
}
|
|
public float RightMargin
|
|
{
|
|
get { return (GetProperty("RightMargin") as float? ?? 0); }
|
|
set { SetProperty("RightMargin", value); }
|
|
}
|
|
public float TopMargin
|
|
{
|
|
get { return (GetProperty("TopMargin") as float? ?? 0); }
|
|
set { SetProperty("TopMargin", value); }
|
|
}
|
|
public LBWdOrientation Orientation
|
|
{
|
|
get { return (LBWdOrientation)GetProperty("Orientation"); }
|
|
set { SetProperty("Orientation", value); }
|
|
}
|
|
public float PageHeight
|
|
{
|
|
get { return (GetProperty("PageHeight") as float? ?? 0); }
|
|
set { SetProperty("PageHeight", value); }
|
|
}
|
|
public float PageWidth
|
|
{
|
|
get { return (GetProperty("PageWidth") as float? ?? 0); }
|
|
set { SetProperty("PageWidth", value); }
|
|
}
|
|
public LBWdPaperSize PaperSize
|
|
{
|
|
get { return (LBWdPaperSize)GetProperty("PaperSize"); }
|
|
set { SetProperty("PaperSize", value); }
|
|
}
|
|
public float LinesPage
|
|
{
|
|
get { return (GetProperty("LinesPage") as float? ?? 0); }
|
|
set { SetProperty("LinesPage", value); }
|
|
}
|
|
public float CharsLine
|
|
{
|
|
get { return (GetProperty("CharsLine") as float? ?? 0); }
|
|
set { SetProperty("CharsLine", value); }
|
|
}
|
|
}
|
|
public enum LBWdExportFormat
|
|
{
|
|
wdExportFormatPDF = 17,
|
|
wdExportFormatXPS = 18
|
|
}
|
|
public enum LBWdExportOptimizeFor
|
|
{
|
|
wdExportOptimizeForPrint = 0,
|
|
wdExportOptimizeForOnScreen = 1
|
|
}
|
|
public enum LBWdExportRange
|
|
{
|
|
wdExportAllDocument = 0,
|
|
wdExportSelection = 1,
|
|
wdExportCurrentPage = 2,
|
|
wdExportFromTo = 3
|
|
}
|
|
public enum LBWdExportItem
|
|
{
|
|
wdExportDocumentContent = 0,
|
|
wdExportDocumentWithMarkup = 7
|
|
}
|
|
public enum LBWdExportCreateBookmarks
|
|
{
|
|
wdExportCreateNoBookmarks = 0,
|
|
wdExportCreateHeadingBookmarks = 1,
|
|
wdExportCreateWordBookmarks = 2
|
|
}
|
|
public partial class LBPane : LBComObject
|
|
{
|
|
public LBPane() { }
|
|
public LBPane(Object item) : base(item) { }
|
|
public LBPages Pages
|
|
{
|
|
get { return new LBPages(GetProperty("Pages")); }
|
|
}
|
|
public LBView View
|
|
{
|
|
get { return new LBView(GetProperty("View")); }
|
|
}
|
|
}
|
|
public partial class LBView : LBComObject
|
|
{
|
|
public LBView() { }
|
|
public LBView(Object item) : base(item) { }
|
|
public LBWdViewType Type
|
|
{
|
|
get { return (LBWdViewType)GetProperty("Type"); }
|
|
set { SetProperty("Type", value); }
|
|
}
|
|
}
|
|
public enum LBWdColorIndex
|
|
{
|
|
wdNoHighlight = 0,
|
|
wdBlack = 1,
|
|
wdBlue = 2,
|
|
wdTurquoise = 3,
|
|
wdBrightGreen = 4,
|
|
wdPink = 5,
|
|
wdRed = 6,
|
|
wdYellow = 7,
|
|
wdWhite = 8,
|
|
wdDarkBlue = 9,
|
|
wdTeal = 10,
|
|
wdGreen = 11,
|
|
wdViolet = 12,
|
|
wdDarkRed = 13,
|
|
wdDarkYellow = 14,
|
|
wdGray50 = 15,
|
|
wdGray25 = 16,
|
|
wdByAuthor = -1
|
|
}
|
|
public enum LBWdLineSpacing
|
|
{
|
|
wdLineSpaceSingle = 0,
|
|
wdLineSpace1pt5 = 1,
|
|
wdLineSpaceDouble = 2,
|
|
wdLineSpaceAtLeast = 3,
|
|
wdLineSpaceExactly = 4,
|
|
wdLineSpaceMultiple = 5
|
|
}
|
|
public partial class LBReplacement : LBComObject
|
|
{
|
|
public LBReplacement() { }
|
|
public LBReplacement(Object item) : base(item) { }
|
|
public String Text
|
|
{
|
|
get { return (GetProperty("Text").ToString()); }
|
|
set { SetProperty("Text", value); }
|
|
}
|
|
public void ClearFormatting()
|
|
{
|
|
InvokeMethod("ClearFormatting");
|
|
}
|
|
}
|
|
public enum LBWdFindWrap
|
|
{
|
|
wdFindStop = 0,
|
|
wdFindContinue = 1,
|
|
wdFindAsk = 2
|
|
}
|
|
public enum LBWdColor
|
|
{
|
|
wdColorBlack = 0,
|
|
wdColorDarkRed = 128,
|
|
wdColorRed = 255,
|
|
wdColorDarkGreen = 13056,
|
|
wdColorOliveGreen = 13107,
|
|
wdColorBrown = 13209,
|
|
wdColorOrange = 26367,
|
|
wdColorGreen = 32768,
|
|
wdColorDarkYellow = 32896,
|
|
wdColorLightOrange = 39423,
|
|
wdColorLime = 52377,
|
|
wdColorGold = 52479,
|
|
wdColorBrightGreen = 65280,
|
|
wdColorYellow = 65535,
|
|
wdColorGray95 = 789516,
|
|
wdColorGray90 = 1644825,
|
|
wdColorGray875 = 2105376,
|
|
wdColorGray85 = 2500134,
|
|
wdColorGray80 = 3355443,
|
|
wdColorGray75 = 4210752,
|
|
wdColorGray70 = 5000268,
|
|
wdColorGray65 = 5855577,
|
|
wdColorGray625 = 6316128,
|
|
wdColorDarkTeal = 6697728,
|
|
wdColorPlum = 6697881,
|
|
wdColorGray60 = 6710886,
|
|
wdColorSeaGreen = 6723891,
|
|
wdColorGray55 = 7566195,
|
|
wdColorDarkBlue = 8388608,
|
|
wdColorViolet = 8388736,
|
|
wdColorTeal = 8421376,
|
|
wdColorGray50 = 8421504,
|
|
wdColorGray45 = 9211020,
|
|
wdColorIndigo = 10040115,
|
|
wdColorBlueGray = 10053222,
|
|
wdColorGray40 = 10066329,
|
|
wdColorTan = 10079487,
|
|
wdColorLightYellow = 10092543,
|
|
wdColorGray375 = 10526880,
|
|
wdColorGray35 = 10921638,
|
|
wdColorGray30 = 11776947,
|
|
wdColorGray25 = 12632256,
|
|
wdColorRose = 13408767,
|
|
wdColorAqua = 13421619,
|
|
wdColorGray20 = 13421772,
|
|
wdColorLightGreen = 13434828,
|
|
wdColorGray15 = 14277081,
|
|
wdColorGray125 = 14737632,
|
|
wdColorGray10 = 15132390,
|
|
wdColorGray05 = 15987699,
|
|
wdColorBlue = 16711680,
|
|
wdColorPink = 16711935,
|
|
wdColorLightBlue = 16737843,
|
|
wdColorLavender = 16751052,
|
|
wdColorSkyBlue = 16763904,
|
|
wdColorPaleBlue = 16764057,
|
|
wdColorTurquoise = 16776960,
|
|
wdColorLightTurquoise = 16777164,
|
|
wdColorWhite = 16777215,
|
|
wdColorAutomatic = -16777216
|
|
}
|
|
public partial class LBInlineShape : LBComObject
|
|
{
|
|
public LBInlineShape() { }
|
|
public LBInlineShape(Object item) : base(item) { }
|
|
public float Height
|
|
{
|
|
get { return (GetProperty("Height") as float? ?? 0); }
|
|
set { SetProperty("Height", value); }
|
|
}
|
|
public float Width
|
|
{
|
|
get { return (GetProperty("Width") as float? ?? 0); }
|
|
set { SetProperty("Width", value); }
|
|
}
|
|
}
|
|
public partial class LBParagraph : LBComObject
|
|
{
|
|
public LBParagraph() { }
|
|
public LBParagraph(Object item) : base(item) { }
|
|
public LBRange Range
|
|
{
|
|
get { return new LBRange(GetProperty("Range")); }
|
|
}
|
|
}
|
|
public partial class LBShape : LBComObject
|
|
{
|
|
public LBShape() { }
|
|
public LBShape(Object item) : base(item) { }
|
|
public float Height
|
|
{
|
|
get { return (GetProperty("Height") as float? ?? 0); }
|
|
set { SetProperty("Height", value); }
|
|
}
|
|
public float Width
|
|
{
|
|
get { return (GetProperty("Width") as float? ?? 0); }
|
|
set { SetProperty("Width", value); }
|
|
}
|
|
public LBRange Anchor
|
|
{
|
|
get { return new LBRange(GetProperty("Anchor")); }
|
|
}
|
|
public LBMsoTriState LockAspectRatio
|
|
{
|
|
get { return (LBMsoTriState)GetProperty("LockAspectRatio"); }
|
|
set { SetProperty("LockAspectRatio", value); }
|
|
}
|
|
public float Left
|
|
{
|
|
get { return (GetProperty("Left") as float? ?? 0); }
|
|
set { SetProperty("Left", value); }
|
|
}
|
|
public float LeftRelative
|
|
{
|
|
get { return (GetProperty("LeftRelative") as float? ?? 0); }
|
|
set { SetProperty("LeftRelative", value); }
|
|
}
|
|
public float Top
|
|
{
|
|
get { return (GetProperty("Top") as float? ?? 0); }
|
|
set { SetProperty("Top", value); }
|
|
}
|
|
public float TopRelative
|
|
{
|
|
get { return (GetProperty("TopRelative") as float? ?? 0); }
|
|
set { SetProperty("TopRelative", value); }
|
|
}
|
|
public LBWdRelativeHorizontalPosition RelativeHorizontalPosition
|
|
{
|
|
get { return (LBWdRelativeHorizontalPosition)GetProperty("RelativeHorizontalPosition"); }
|
|
set { SetProperty("RelativeHorizontalPosition", value); }
|
|
}
|
|
public LBWdRelativeVerticalPosition RelativeVerticalPosition
|
|
{
|
|
get { return (LBWdRelativeVerticalPosition)GetProperty("RelativeVerticalPosition"); }
|
|
set { SetProperty("RelativeVerticalPosition", value); }
|
|
}
|
|
public int LockAnchor
|
|
{
|
|
get { return (GetProperty("LockAnchor") as int? ?? 0); }
|
|
set { SetProperty("LockAnchor", value); }
|
|
}
|
|
}
|
|
public enum LBWdOrientation
|
|
{
|
|
wdOrientPortrait = 0,
|
|
wdOrientLandscape = 1
|
|
}
|
|
public enum LBWdPaperSize
|
|
{
|
|
wdPaper10x14 = 0,
|
|
wdPaper11x17 = 1,
|
|
wdPaperLetter = 2,
|
|
wdPaperLetterSmall = 3,
|
|
wdPaperLegal = 4,
|
|
wdPaperExecutive = 5,
|
|
wdPaperA3 = 6,
|
|
wdPaperA4 = 7,
|
|
wdPaperA4Small = 8,
|
|
wdPaperA5 = 9,
|
|
wdPaperB4 = 10,
|
|
wdPaperB5 = 11,
|
|
wdPaperCSheet = 12,
|
|
wdPaperDSheet = 13,
|
|
wdPaperESheet = 14,
|
|
wdPaperFanfoldLegalGerman = 15,
|
|
wdPaperFanfoldStdGerman = 16,
|
|
wdPaperFanfoldUS = 17,
|
|
wdPaperFolio = 18,
|
|
wdPaperLedger = 19,
|
|
wdPaperNote = 20,
|
|
wdPaperQuarto = 21,
|
|
wdPaperStatement = 22,
|
|
wdPaperTabloid = 23,
|
|
wdPaperEnvelope9 = 24,
|
|
wdPaperEnvelope10 = 25,
|
|
wdPaperEnvelope11 = 26,
|
|
wdPaperEnvelope12 = 27,
|
|
wdPaperEnvelope14 = 28,
|
|
wdPaperEnvelopeB4 = 29,
|
|
wdPaperEnvelopeB5 = 30,
|
|
wdPaperEnvelopeB6 = 31,
|
|
wdPaperEnvelopeC3 = 32,
|
|
wdPaperEnvelopeC4 = 33,
|
|
wdPaperEnvelopeC5 = 34,
|
|
wdPaperEnvelopeC6 = 35,
|
|
wdPaperEnvelopeC65 = 36,
|
|
wdPaperEnvelopeDL = 37,
|
|
wdPaperEnvelopeItaly = 38,
|
|
wdPaperEnvelopeMonarch = 39,
|
|
wdPaperEnvelopePersonal = 40,
|
|
wdPaperCustom = 41
|
|
}
|
|
public partial class LBPages : LBComObjectList<LBPages, LBPage> /* Collection */
|
|
{
|
|
public LBPages(Object item)
|
|
{
|
|
Item = item;
|
|
}
|
|
public int Count
|
|
{
|
|
get { return (GetProperty("Count") as int? ?? 0); }
|
|
}
|
|
}
|
|
public enum LBWdViewType
|
|
{
|
|
wdNormalView = 1,
|
|
wdOutlineView = 2,
|
|
wdPrintView = 3,
|
|
wdPrintPreview = 4,
|
|
wdMasterView = 5,
|
|
wdWebView = 6,
|
|
wdReadingView = 7
|
|
}
|
|
public enum LBWdSaveFormat
|
|
{
|
|
wdFormatDocument = 0,
|
|
wdFormatTemplate = 1,
|
|
wdFormatText = 2,
|
|
wdFormatTextLineBreaks = 3,
|
|
wdFormatDOSText = 4,
|
|
wdFormatDOSTextLineBreaks = 5,
|
|
wdFormatRTF = 6,
|
|
wdFormatUnicodeText = 7,
|
|
wdFormatHTML = 8,
|
|
wdFormatWebArchive = 9,
|
|
wdFormatFilteredHTML = 10,
|
|
wdFormatXML = 11,
|
|
wdFormatXMLDocument = 12,
|
|
wdFormatXMLDocumentMacroEnabled = 13,
|
|
wdFormatXMLTemplate = 14,
|
|
wdFormatXMLTemplateMacroEnabled = 15,
|
|
wdFormatDocumentDefault = 16,
|
|
wdFormatPDF = 17,
|
|
wdFormatXPS = 18,
|
|
wdFormatFlatXML = 19,
|
|
wdFormatFlatXMLMacroEnabled = 20,
|
|
wdFormatFlatXMLTemplate = 21,
|
|
wdFormatFlatXMLTemplateMacroEnabled = 22,
|
|
wdFormatOpenDocumentText = 23
|
|
}
|
|
public enum LBWdFindMatch
|
|
{
|
|
wdMatchGraphic = 1,
|
|
wdMatchCommentMark = 5,
|
|
wdMatchTabCharacter = 9,
|
|
wdMatchCaretCharacter = 11,
|
|
wdMatchColumnBreak = 14,
|
|
wdMatchField = 19,
|
|
wdMatchNonbreakingHyphen = 30,
|
|
wdMatchOptionalHyphen = 31,
|
|
wdMatchNonbreakingSpace = 160,
|
|
wdMatchEnDash = 8211,
|
|
wdMatchEmDash = 8212,
|
|
wdMatchParagraphMark = 65551,
|
|
wdMatchFootnoteMark = 65554,
|
|
wdMatchEndnoteMark = 65555,
|
|
wdMatchManualPageBreak = 65564,
|
|
wdMatchAnyDigit = 65567,
|
|
wdMatchSectionBreak = 65580,
|
|
wdMatchAnyLetter = 65583,
|
|
wdMatchAnyCharacter = 65599,
|
|
wdMatchWhiteSpace = 65655
|
|
}
|
|
public enum LBWdReplace
|
|
{
|
|
wdReplaceNone = 0,
|
|
wdReplaceOne = 1,
|
|
wdReplaceAll = 2
|
|
}
|
|
public partial class LBPage : LBComObject
|
|
{
|
|
public LBPage() { }
|
|
public LBPage(Object item) : base(item) { }
|
|
public int Height
|
|
{
|
|
get { return (GetProperty("Height") as int? ?? 0); }
|
|
}
|
|
public int Left
|
|
{
|
|
get { return (GetProperty("Left") as int? ?? 0); }
|
|
}
|
|
public LBRectangles Rectangles
|
|
{
|
|
get { return new LBRectangles(GetProperty("Rectangles")); }
|
|
}
|
|
public int Top
|
|
{
|
|
get { return (GetProperty("Top") as int? ?? 0); }
|
|
}
|
|
public int Width
|
|
{
|
|
get { return (GetProperty("Width") as int? ?? 0); }
|
|
}
|
|
}
|
|
public partial class LBRectangles : LBComObjectList<LBRectangles, LBRectangle> /* Collection */
|
|
{
|
|
public LBRectangles(Object item)
|
|
{
|
|
Item = item;
|
|
}
|
|
public int Count
|
|
{
|
|
get { return (GetProperty("Count") as int? ?? 0); }
|
|
}
|
|
}
|
|
public partial class LBRectangle : LBComObject
|
|
{
|
|
public LBRectangle() { }
|
|
public LBRectangle(Object item) : base(item) { }
|
|
public LBLines Lines
|
|
{
|
|
get { return new LBLines(GetProperty("Lines")); }
|
|
}
|
|
}
|
|
public partial class LBLines : LBComObjectList<LBLines, LBLine> /* Collection */
|
|
{
|
|
public LBLines(Object item)
|
|
{
|
|
Item = item;
|
|
}
|
|
public int Count
|
|
{
|
|
get { return (GetProperty("Count") as int? ?? 0); }
|
|
}
|
|
}
|
|
public partial class LBLine : LBComObject
|
|
{
|
|
public LBLine() { }
|
|
public LBLine(Object item) : base(item) { }
|
|
public int Top
|
|
{
|
|
get { return (GetProperty("Top") as int? ?? 0); }
|
|
}
|
|
public int Height
|
|
{
|
|
get { return (GetProperty("Height") as int? ?? 0); }
|
|
}
|
|
}
|
|
public enum LBWdMovementType
|
|
{
|
|
wdMove = 0,
|
|
wdExtend = 1
|
|
}
|
|
public enum LBWdUnits
|
|
{
|
|
wdCharacter = 1,
|
|
wdWord = 2,
|
|
wdSentence = 3,
|
|
wdParagraph = 4,
|
|
wdLine = 5,
|
|
wdStory = 6,
|
|
wdScreen = 7,
|
|
wdSection = 8,
|
|
wdColumn = 9,
|
|
wdRow = 10,
|
|
wdWindow = 11,
|
|
wdCell = 12,
|
|
wdCharacterFormatting = 13,
|
|
wdParagraphFormatting = 14,
|
|
wdTable = 15,
|
|
wdItem = 16
|
|
}
|
|
public enum LBWdGoToDirection
|
|
{
|
|
wdGoToAbsolute = 1,
|
|
wdGoToNext = 2,
|
|
wdGoToPrevious = 3,
|
|
wdGoToLast = -1
|
|
}
|
|
public enum LBWdGoToItem
|
|
{
|
|
wdGoToSection = 0,
|
|
wdGoToPage = 1,
|
|
wdGoToTable = 2,
|
|
wdGoToLine = 3,
|
|
wdGoToFootnote = 4,
|
|
wdGoToEndnote = 5,
|
|
wdGoToComment = 6,
|
|
wdGoToField = 7,
|
|
wdGoToGraphic = 8,
|
|
wdGoToObject = 9,
|
|
wdGoToEquation = 10,
|
|
wdGoToHeading = 11,
|
|
wdGoToPercent = 12,
|
|
wdGoToSpellingError = 13,
|
|
wdGoToGrammaticalError = 14,
|
|
wdGoToProofreadingError = 15,
|
|
wdGoToBookmark = -1
|
|
}
|
|
public partial class LBPictureFormat : LBComObject
|
|
{
|
|
public LBPictureFormat() { }
|
|
public LBPictureFormat(Object item) : base(item) { }
|
|
}
|
|
public enum LBWdRelativeHorizontalPosition
|
|
{
|
|
wdRelativeHorizontalPositionMargin = 0,
|
|
wdRelativeHorizontalPositionPage = 1,
|
|
wdRelativeHorizontalPositionColumn = 2,
|
|
wdRelativeHorizontalPositionCharacter = 3,
|
|
wdRelativeHorizontalPositionLeftMarginArea = 4,
|
|
wdRelativeHorizontalPositionRightMarginArea = 5,
|
|
wdRelativeHorizontalPositionInnerMarginArea = 6,
|
|
wdRelativeHorizontalPositionOuterMarginArea = 7
|
|
}
|
|
public enum LBWdRelativeVerticalPosition
|
|
{
|
|
wdRelativeVerticalPositionMargin = 0,
|
|
wdRelativeVerticalPositionPage = 1,
|
|
wdRelativeVerticalPositionParagraph = 2,
|
|
wdRelativeVerticalPositionLine = 3,
|
|
wdRelativeVerticalPositionTopMarginArea = 4,
|
|
wdRelativeVerticalPositionBottomMarginArea = 5,
|
|
wdRelativeVerticalPositionInnerMarginArea = 6,
|
|
wdRelativeVerticalPositionOuterMarginArea = 7
|
|
}
|
|
}
|