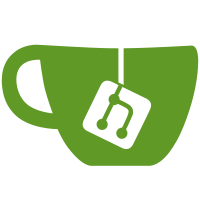
Class for processing master slave data (under development) Class for processing converting 16-bit approved data
66 lines
1.9 KiB
C#
66 lines
1.9 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using System.IO;
|
|
|
|
namespace DataLoader
|
|
{
|
|
public partial class Loader
|
|
{
|
|
public bool BuildScopeApplicability()
|
|
{
|
|
bool rv = false;
|
|
//get scopes from proc.ini
|
|
rv |= CreateScopes();
|
|
//end get scopes from proc.ini
|
|
//get apl dictionary
|
|
Dictionary<string, Dictionary<string, string>> procAPLs = new Dictionary<string, Dictionary<string, string>>();
|
|
string[] procs = Directory.GetFiles(frmMain.MySettings.ProcedureSetPath, "PROC*.APL");
|
|
foreach (string proc in procs)
|
|
{
|
|
Dictionary<string, string> procAPL = new Dictionary<string, string>();
|
|
FileInfo myFile = new FileInfo(proc);
|
|
FileStream fs = myFile.OpenRead();
|
|
BinaryReader br = new BinaryReader(fs);
|
|
byte[] myBuff = new byte[myFile.Length];
|
|
br.Read(myBuff, 0, (int)myFile.Length);
|
|
br.Close();
|
|
fs.Close();
|
|
int offset = 0;
|
|
while (offset < myBuff.Length)
|
|
{
|
|
string recnum = Encoding.Default.GetString(myBuff, offset, 8);
|
|
int applicability = BitConverter.ToInt32(myBuff, offset + 8);
|
|
string applicabilityToStr = string.Format("{0:X8}", applicability);
|
|
//4294967296
|
|
if (!procAPL.ContainsKey(recnum))
|
|
procAPL.Add(recnum, applicabilityToStr);
|
|
else
|
|
if (procAPL[recnum] != applicabilityToStr)
|
|
procAPL[recnum] = applicabilityToStr;
|
|
offset += 12;
|
|
}
|
|
procAPLs.Add(proc, procAPL);
|
|
}
|
|
//end get apl dictionary
|
|
rv = true;
|
|
return rv;
|
|
}
|
|
|
|
private bool CreateScopes()
|
|
{
|
|
string iniFile = string.Format(@"{0}\PROC.ini", frmMain.MySettings.ProcedureSetPath);
|
|
TextReader tr = File.OpenText(iniFile);
|
|
string line = string.Empty;
|
|
while (!line.StartsWith("Name="))
|
|
line = tr.ReadLine();
|
|
tr.Close();
|
|
string[] parts = line.Split('=');
|
|
string[] scopes = parts[1].Split(',');
|
|
foreach (string scope in scopes)
|
|
Console.WriteLine(scope);
|
|
return true;
|
|
}
|
|
}
|
|
}
|