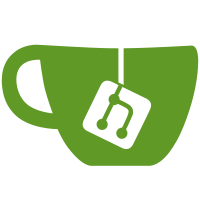
Added improvements to the code to better handle proc.ini files when they are part of a multi unit site, especially case sensitivity of elements of the proc.ini file. The changes involve all of the stored procedures, functions and table changes to support multiuser and security configuration of PROMS.
278 lines
9.5 KiB
C#
278 lines
9.5 KiB
C#
using System;
|
|
using System.Drawing;
|
|
using System.Collections;
|
|
using System.ComponentModel;
|
|
using System.Windows.Forms;
|
|
using System.Data;
|
|
using System.Data.OleDb;
|
|
using System.Collections.Specialized;
|
|
using System.Collections.Generic;
|
|
using System.Xml;
|
|
using System.IO;
|
|
using System.Text;
|
|
using Volian.MSWord;
|
|
using vlnObjectLibrary;
|
|
using vlnServerLibrary;
|
|
using VEPROMS.CSLA.Library;
|
|
using Config;
|
|
using Volian.Base.Library;
|
|
|
|
namespace DataLoader
|
|
{
|
|
public partial class Loader
|
|
{
|
|
#region ClassProperties
|
|
private int wms = 500;
|
|
private static int EDDATA = 0x01;
|
|
private static int PH = 0x02;
|
|
private static int TOC = 0x04;
|
|
private static int AUTOIND = 0x08;
|
|
private static int AUTOGEN = 0x40;
|
|
|
|
private static int STP_MULT_CHGID = 1;
|
|
private static int STP_LNK_SEQ = 2;
|
|
private static int STP_OVR_TAB = 3;
|
|
|
|
private string ProcFileName;
|
|
private string ProcNumber;
|
|
private ROFstInfo rofstinfo;
|
|
private RODb rodb;
|
|
private int EditSectId;
|
|
private Dictionary<string, int> dicLibDocRef;
|
|
|
|
// have a few variables for storing the database id record & the system record.
|
|
public Connection dbConn;
|
|
public Folder sysFolder;
|
|
public AnnotationType CommentType; // this holds the annotationtype of comment for future use
|
|
public AnnotationType MigrationErrorType; // this holds the annotationtype of Migration Error for future use
|
|
public AnnotationType VerificationRequiredType; // Using this to flag table to grid conversions
|
|
public Document MissingDocument = null; // make a document if there is a missing rtf file
|
|
// any missing will use this.
|
|
private Content TransDummyCont;
|
|
// the following two dictionaries are used to handle migration of the
|
|
// transitions... dicTrans_ItemDone gets an entry for procnumber, sequence
|
|
// number and the new item id as a step or section is created (transitions
|
|
// can go to steps or sections). When a transition is encountered, check this
|
|
// dictionary to see if the step or section was migrated & use the item
|
|
// for the step or section if it was migrated. When a transition is migrated where
|
|
// the 'to' has not been migrated yet, check if an entry exists in dicTrans_ItemIds,
|
|
// if so, use the item listed here. If no entry exists in dicTrans_ItemIds, create
|
|
// an item table record and use the id as part of the 'to', and add an entry to
|
|
// dicTrans_ItemIds to flag that the record was already created. As migrating sections
|
|
// and steps, check this dicTrans_ItemIds to see if the item record has already
|
|
// been create, if so use it and remove it from the dicTrans_ItemIds dictionary,
|
|
// otherwise, create a new item record.
|
|
private Dictionary<string, Item> dicTrans_ItemDone;
|
|
private Dictionary<string, Item> dicTrans_ItemIds;
|
|
private Dictionary<string, List<Item>> dicTrans_MigrationErrors;
|
|
private Dictionary<object, string> dicOldStepSequence;
|
|
private Dictionary<TreeNode, TreeNode> dicNeedToLoad;
|
|
private Dictionary<string, string> dicSetfileEntries;
|
|
|
|
private log4net.ILog log;
|
|
#endregion
|
|
private frmLoader frmMain;
|
|
public Loader(log4net.ILog lg, frmLoader fm)
|
|
{
|
|
dicNeedToLoad = new Dictionary<TreeNode, TreeNode>();
|
|
log = lg;
|
|
frmMain = fm;
|
|
}
|
|
public bool LoadFolders(string vepromspath)
|
|
{
|
|
try
|
|
{
|
|
//_FmtAllPath = frmMain.MySettings.FormatFolder;
|
|
//_GenmacAllPath = frmMain.MySettings.GenMacFolder;
|
|
frmMain.Status = "Make Connection";
|
|
// make the initial database connection record, annotation types & top
|
|
// system folder.
|
|
dbConn = Connection.MakeConnection("Default", "Default", frmMain.MySettings.ConnectionString.Replace("{DBName}",frmMain.MySettings.DBName), 1, null, DateTime.Now, "Migration");
|
|
ConfigFile cfg = new ConfigFile();
|
|
|
|
frmMain.Status = "Add AnnotationTypes";
|
|
CommentType = AnnotationType.MakeAnnotationType("Comment", null);
|
|
MigrationErrorType = AnnotationType.MakeAnnotationType("Migration Error", null);
|
|
|
|
AnnotationType at = AnnotationType.MakeAnnotationType("Reference", null);
|
|
at = AnnotationType.MakeAnnotationType("Action Items", null);
|
|
//at = AnnotationType.MakeAnnotationType("Verification Required", null);
|
|
VerificationRequiredType = AnnotationType.MakeAnnotationType("Verification Required", null);
|
|
at = AnnotationType.MakeAnnotationType("Volian Comment", null);
|
|
|
|
frmMain.Status = "Load veproms.ini";
|
|
XmlDocument d = cfg.LoadSystemIni(vepromspath);
|
|
|
|
frmMain.Status = "Load All Formats";
|
|
//LoadAllFormats();
|
|
//Format.UpdateFormats(_FmtAllPath, _GenmacAllPath);
|
|
|
|
Format.UpdateFormats(frmMain.MySettings.FormatFolder, frmMain.MySettings.GenMacFolder);
|
|
|
|
//Format baseFormat = Format.Get(1);
|
|
//sysFolder = Folder.MakeFolder(null, dbConn, "VEPROMS", "VEPROMS", "VEPROMS", baseFormat, d.InnerXml, DateTime.Now, "Migration");
|
|
using (Format baseFormat = Format.Get(1))
|
|
{
|
|
sysFolder = Folder.MakeFolder(null, dbConn, "VEPROMS", "VEPROMS", "VEPROMS", baseFormat, d.InnerXml, DateTime.Now, "Migration");
|
|
}
|
|
|
|
// This is to test the vln Libraries
|
|
List<Folder> lfldr = vlnDataPathFolders();
|
|
|
|
List<vlnObject> dp2 = new List<vlnObject>();
|
|
foreach (Folder fldr in lfldr)
|
|
{
|
|
TreeNode tn = frmMain.TV.Nodes.Add(fldr.Name);
|
|
tn.Tag = fldr;
|
|
vlnObject vb = new vlnObject(null, "datapath", fldr.Name, fldr.Title);
|
|
dp2.Add(vb);
|
|
vlnServer vs = new vlnServer();
|
|
frmMain.Status = "Loading " + fldr.Name;
|
|
MigrateChildren(vb, vs, dbConn, fldr, tn);
|
|
if (frmMain.ProcessFailed)
|
|
return false;
|
|
tn.Expand();
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
log.Error("Error in LoadFolders", ex);
|
|
// log.ErrorFormat("Could not load data, error = {0}", ex.Message);
|
|
//return false;
|
|
throw new Exception("Error in LoadFolders", ex);
|
|
}
|
|
return true;
|
|
}
|
|
public bool LoadFoldersIntoExisting(string s)
|
|
{
|
|
try
|
|
{
|
|
frmMain.Status = "Getting Connection";
|
|
// get the connection and annotations from the existing database.
|
|
dbConn = Connection.GetByName("Default");
|
|
//dbConn = Connection.MakeConnection("Default", "Default", frmMain.MySettings.ConnectionString.Replace("{DBName}",frmMain.MySettings.DBName), 1, null, DateTime.Now, "Migration");
|
|
|
|
frmMain.Status = "Getting AnnotationTypes";
|
|
CommentType = AnnotationType.GetByName("Comment"); // .MakeAnnotationType("Comment", null);
|
|
MigrationErrorType = AnnotationType.GetByName("Migration Error");
|
|
VerificationRequiredType = AnnotationType.GetByName("Verification Required");
|
|
|
|
List<Folder> lfldr = vlnDataPathFolders(); // get plant level list.
|
|
|
|
List<vlnObject> dp2 = new List<vlnObject>();
|
|
foreach (Folder fldr in lfldr)
|
|
{
|
|
TreeNode tn = frmMain.TV.Nodes.Add(fldr.Name);
|
|
tn.Tag = fldr;
|
|
vlnObject vb = new vlnObject(null, "datapath", fldr.Name, fldr.Title);
|
|
dp2.Add(vb);
|
|
vlnServer vs = new vlnServer();
|
|
frmMain.Status = "Loading " + fldr.Name;
|
|
MigrateChildren(vb, vs, dbConn, fldr, tn);
|
|
if (frmMain.ProcessFailed)
|
|
return false;
|
|
tn.Expand();
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
log.ErrorFormat("Could not load data, error = {0}", ex.Message);
|
|
//return false;
|
|
throw new Exception("Error in LoadFolders", ex);
|
|
}
|
|
return true;
|
|
}
|
|
public void ClearData()
|
|
{
|
|
int ra = PurgeDataCommand.Execute();
|
|
//Database.PurgeData();
|
|
}
|
|
public static string MakeDate(string src)
|
|
{
|
|
if (src.Trim() == "") return null;
|
|
int[] DateOffset ={ 4, 5, 47, 6, 7, 47, 0, 1, 2, 3 }; // 47 = '/'
|
|
StringBuilder datebuff = new StringBuilder(10);
|
|
for (int i = 0; i < DateOffset.Length; i++)
|
|
{
|
|
if (DateOffset[i] < 9)
|
|
datebuff.Append(src[DateOffset[i]]);
|
|
else
|
|
datebuff.Append(System.Convert.ToChar(DateOffset[i]));
|
|
}
|
|
return datebuff.ToString();
|
|
}
|
|
public DateTime GetDTS(string date, string time)
|
|
{
|
|
// Set the date/time stamp. If there is no 'date', set the date
|
|
// to 1/1/2000 (this can be changed!). If there is not 'time',
|
|
// set the time to 0:0:0 (midnight).
|
|
|
|
DateTime dts = DateTime.Now;
|
|
string month = "01";
|
|
string day = "01";
|
|
string year = "2000";
|
|
string hour = "";
|
|
string minute = "";
|
|
try
|
|
{
|
|
if (date != null && date != "")
|
|
{
|
|
int indx1 = date.IndexOf("/");
|
|
month = date.Substring(0, indx1);
|
|
int indx2 = date.IndexOf("/", indx1 + 1);
|
|
day = date.Substring(indx1 + 1, indx2 - indx1 - 1);
|
|
year = date.Substring(indx2 + 1, 4);
|
|
}
|
|
if (time == null || time == "")
|
|
{
|
|
hour = "0";
|
|
minute = "0";
|
|
}
|
|
else
|
|
{
|
|
|
|
hour = time.Substring(0, 2);
|
|
int indxc = time.IndexOfAny(":A-".ToCharArray());
|
|
if (indxc == time.Length - 1)
|
|
minute = time.Substring(2, 2);
|
|
else
|
|
minute = time.Substring(indxc + 1, time.Length - indxc - 1);
|
|
}
|
|
dts = new DateTime(System.Convert.ToInt32(year), System.Convert.ToInt32(month), System.Convert.ToInt32(day),
|
|
System.Convert.ToInt32(hour), System.Convert.ToInt32(minute), 0);
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
log.ErrorFormat("Bad Date/Time {0} {1}. Set to NOW.", date, time);
|
|
log.ErrorFormat("{0}\r\n\r\n{1}", ex.Message, ex.InnerException);
|
|
frmMain.AddError(ex, "Bad Date/Time {0} {1}", date, time);
|
|
//log.ErrorFormat(ex.StackTrace);
|
|
return dts;
|
|
}
|
|
return dts;
|
|
}
|
|
|
|
public bool LoadSecurity(string VeSamPath, string VePromsPath)
|
|
{
|
|
Security sec = new Security(VeSamPath, VePromsPath);
|
|
return sec.Migrate();
|
|
}
|
|
private static void WaitMS(int n)
|
|
{
|
|
DateTime dtw = DateTime.Now.AddMilliseconds(n);
|
|
while (DateTime.Now < dtw)
|
|
{
|
|
Application.DoEvents();
|
|
}
|
|
}
|
|
private static void Wait(int n)
|
|
{
|
|
DateTime dtw = DateTime.Now.AddSeconds(n);
|
|
while (DateTime.Now < dtw)
|
|
{
|
|
Application.DoEvents();
|
|
}
|
|
}
|
|
}
|
|
}
|