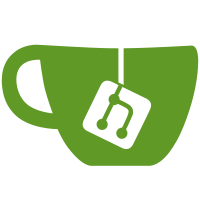
If you open two procedures and then drag one of them so that it is next to the other and then close one of the two procedures, PROMS2010 would hang.
478 lines
16 KiB
C#
478 lines
16 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.ComponentModel;
|
|
using System.Drawing;
|
|
using System.Data;
|
|
using System.Text;
|
|
using System.Windows.Forms;
|
|
using VEPROMS.CSLA.Library;
|
|
using Volian.Controls.Library;
|
|
using DevComponents.DotNetBar;
|
|
|
|
namespace Volian.Controls.Library
|
|
{
|
|
public partial class DisplayTabControl : UserControl
|
|
{
|
|
#region Private Fields
|
|
/// <summary>
|
|
/// This is a lookup table for all of the DisplayTabItems that are currently open
|
|
/// The key is:
|
|
/// "Item - " + Procedure ItemID for step pages
|
|
/// "Doc - " + DocumentID for Word Documents
|
|
/// </summary>
|
|
private Dictionary<string, DisplayTabItem> _MyDisplayTabItems;
|
|
/// <summary>
|
|
/// When a Tab is closed it is added to this list.
|
|
/// When another Tab is opened, any Tabs in this list are closed
|
|
/// </summary>
|
|
private List<DisplayTabItem> _RemovedDisplayTabItems = null;
|
|
/// <summary>
|
|
/// This stores a UniqueID for Bars as they are opened.
|
|
/// A bar is the docking location for the DisplayTabItems.
|
|
/// </summary>
|
|
private int _UniqueBarCount;
|
|
private ItemInfo _MyItemInfo = null;
|
|
private StepItem _MyStepItem = null;
|
|
#endregion
|
|
#region Events
|
|
/// <summary>
|
|
/// This event is raised when a the "Tab" of a DisplayItem is clicked with a mouse.
|
|
/// So far this has just been used for demo purposes. It could be used to select a
|
|
/// step and it's children for the purpose of copying.
|
|
/// </summary>
|
|
public event StepPanelEvent ItemClick;
|
|
/// <summary>
|
|
/// Checks to see if the ItemClick event is handled and launches it
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
internal void OnItemClick(object sender, StepPanelEventArgs args)
|
|
{
|
|
if (ItemClick != null) ItemClick(sender, args);
|
|
}
|
|
/// <summary>
|
|
/// This occurs when the user moves onto or off of a link within a RichTextBox
|
|
/// or moves between RichTextBoxes or Pages.
|
|
/// </summary>
|
|
public event StepPanelLinkEvent LinkActiveChanged;
|
|
/// <summary>
|
|
/// Checks to see if the LinkActiveChanged event is handled and launches it
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
internal void OnLinkActiveChanged(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
if (LinkActiveChanged != null) LinkActiveChanged(sender, args);
|
|
else MessageBox.Show(args.LinkInfoText, "Unhandled Link Active Changed", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
/// <summary>
|
|
/// This occurs when a Transition is inserted
|
|
/// </summary>
|
|
public event StepPanelLinkEvent LinkInsertTran;
|
|
/// <summary>
|
|
/// Checks to see if the 'LinkInsertTran' event is handled and launches it
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
internal void OnLinkInsertTran(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
if (LinkInsertTran != null) LinkInsertTran(sender, args);
|
|
else MessageBox.Show(args.LinkInfoText, "Unhandled Link Insert Tran", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
/// <summary>
|
|
/// This occurs when an RO is inserted
|
|
/// </summary>
|
|
public event StepPanelLinkEvent LinkInsertRO;
|
|
/// <summary>
|
|
/// Checks to see if the 'LinkInsertRO' event is handled and launches it
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
internal void OnLinkInsertRO(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
if (LinkInsertRO != null) LinkInsertRO(sender, args);
|
|
else MessageBox.Show(args.LinkInfoText, "Unhandled Link Insert RO", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
/// <summary>
|
|
/// This occurs when a Transition is modified
|
|
/// </summary>
|
|
public event StepPanelLinkEvent LinkModifyTran;
|
|
/// <summary>
|
|
/// Checks to see if the 'LinkModifyTran' event is handled and launches it
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
internal void OnLinkModifyTran(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
if (LinkModifyTran != null) LinkModifyTran(sender, args);
|
|
else MessageBox.Show(args.LinkInfoText, "Unhandled Link Modify Tran", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
/// <summary>
|
|
/// This occurs when an RO is modified
|
|
/// </summary>
|
|
public event StepPanelLinkEvent LinkModifyRO;
|
|
/// <summary>
|
|
/// Checks to see if the 'LinkModifyRO' event is handled and launches it
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
internal void OnLinkModifyRO(object sender, StepPanelLinkEventArgs args)
|
|
{
|
|
if (LinkModifyRO != null) LinkModifyRO(sender, args);
|
|
else MessageBox.Show(args.LinkInfoText, "Unhandled Link Modify RO", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
/// <summary>
|
|
/// Occurs when the user selects a different item or page
|
|
/// </summary>
|
|
public event ItemSelectedChangedEvent ItemSelectedChanged;
|
|
/// <summary>
|
|
/// Checks to see if the 'ItemSelectedChanged' event is handled and launches it
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="args"></param>
|
|
internal void OnItemSelectedChanged(object sender, ItemSelectedChangedEventArgs args)
|
|
{
|
|
if (args != null)
|
|
{
|
|
_MyItemInfo = args.MyItemInfo;
|
|
_MyStepItem = args.MyStepItem;
|
|
}
|
|
else
|
|
{
|
|
_MyItemInfo = null;
|
|
_MyStepItem = null;
|
|
}
|
|
if (ItemSelectedChanged != null) ItemSelectedChanged(sender, args);
|
|
}
|
|
#endregion
|
|
#region Contructor & Setup
|
|
public DisplayTabControl()
|
|
{
|
|
InitializeComponent();
|
|
SetUp();
|
|
}
|
|
private void SetUp()
|
|
{
|
|
_RemovedDisplayTabItems = new List<DisplayTabItem>();
|
|
Dock = DockStyle.Fill;
|
|
dotNetBarManager1.Style = DevComponents.DotNetBar.eDotNetBarStyle.Office2007;
|
|
_MyDisplayTabItems = new Dictionary<string, DisplayTabItem>();
|
|
SetupBar(_MyBar);
|
|
dotNetBarManager1.BarTearOff += new EventHandler(dotNetBarManager1_BarTearOff);
|
|
this.Resize += new EventHandler(DisplayTabControl_Resize);
|
|
}
|
|
void DisplayTabControl_Resize(object sender, EventArgs e)
|
|
{
|
|
// If the currently selected Item is in a Step, then adjust the scroll as necessary to keep it visible
|
|
if (_MyStepItem != null)
|
|
_MyStepItem.ItemShow();
|
|
}
|
|
private void SetupBar(Bar myBar)
|
|
{
|
|
if (myBar.DockTabControl != null)
|
|
{
|
|
myBar.DockTabControl.CloseButtonOnTabsVisible = true;
|
|
myBar.DockTabControl.CloseButtonVisible = false;
|
|
myBar.DockTabControl.CloseButtonPosition = DevComponents.DotNetBar.eTabCloseButtonPosition.Right;
|
|
myBar.DockTabControl.Width = ClientRectangle.Width;
|
|
}
|
|
myBar.DockTabClosing += new DockTabClosingEventHandler(myBar_DockTabClosing);
|
|
if (!myBar.Visible)
|
|
myBar.Visible = true;
|
|
myBar.RecalcLayout();
|
|
}
|
|
#endregion
|
|
#region Internal Event Handlers
|
|
/// <summary>
|
|
/// This is code recommended by DotNetBar
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
void dotNetBarManager1_BarTearOff(object sender, EventArgs e)
|
|
{
|
|
Bar myBar = sender as Bar;
|
|
myBar.Enter += new EventHandler(myBar_Enter);
|
|
}
|
|
/// <summary>
|
|
/// This sets-up the bar after it is selected.
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
void myBar_Enter(object sender, EventArgs e)
|
|
{
|
|
Bar myBar = sender as Bar;
|
|
myBar.Enter -= new EventHandler(myBar_Enter);
|
|
SetupBar(sender as Bar);
|
|
}
|
|
/// <summary>
|
|
/// This handles the closing of a document page
|
|
/// </summary>
|
|
/// <param name="sender"></param>
|
|
/// <param name="e"></param>
|
|
void myBar_DockTabClosing(object sender, DockTabClosingEventArgs e)
|
|
{
|
|
e.RemoveDockTab = true;
|
|
_RemovedDisplayTabItems.Add((DisplayTabItem)e.DockContainerItem);
|
|
DisplayTabItem myTabItem = e.DockContainerItem as DisplayTabItem;
|
|
if (myTabItem != null)
|
|
{
|
|
if (myTabItem.MyDSOTabPanel != null)
|
|
{
|
|
myTabItem.MyDSOTabPanel.CloseDSO();
|
|
}
|
|
}
|
|
if (((Bar)sender).Items.Count == 1)// Remove bar if last item is closed...
|
|
{
|
|
Bar bar = sender as Bar;
|
|
if (bar != null)
|
|
{
|
|
if (dotNetBarManager1.Bars.Contains(bar.Name))
|
|
dotNetBarManager1.Bars.Remove(bar);
|
|
ActivateRemainingTab();
|
|
}
|
|
}
|
|
else
|
|
{
|
|
ActivateRemainingTab((Bar)sender);
|
|
}
|
|
}
|
|
#endregion
|
|
#region Public Methods
|
|
/// <summary>
|
|
/// Open a Step Item or a Word Item
|
|
/// </summary>
|
|
/// <param name="myItemInfo"></param>
|
|
/// <returns></returns>
|
|
public DisplayTabItem OpenItem(ItemInfo myItemInfo)
|
|
{
|
|
CleanUpClosedItems();
|
|
_MyBar = GetParentBar(myItemInfo); // Get the docking bar associated with this item.
|
|
if (myItemInfo.MyContent.MyEntry == null) // If it is a Word document open it in Word
|
|
return OpenStepTabPage(myItemInfo);
|
|
else // Otherwise open it in the step editor
|
|
return OpenDSOTabPage(myItemInfo);
|
|
}
|
|
|
|
private void CleanUpClosedItems()
|
|
{
|
|
while (_RemovedDisplayTabItems.Count > 0) // Clean-up any items that have been closed.
|
|
{
|
|
DisplayTabItem myTabItem = _RemovedDisplayTabItems[0];
|
|
_RemovedDisplayTabItems.RemoveAt(0);
|
|
RemoveItem(myTabItem);
|
|
}
|
|
}
|
|
public void CloseWordItem(ItemInfo myItemInfo)
|
|
{
|
|
string key = "Doc - " + myItemInfo.MyContent.MyEntry.MyDocument.DocID.ToString();
|
|
DisplayTabItem myTabItem = null;
|
|
if (_MyDisplayTabItems.ContainsKey(key)) // If page open, close it
|
|
{
|
|
myTabItem = _MyDisplayTabItems[key];
|
|
if (myTabItem.MyDSOTabPanel != null)
|
|
{
|
|
Bar b = myTabItem.ContainerControl as Bar;
|
|
b.Items.Remove(myTabItem);
|
|
RemoveItem(myTabItem);
|
|
}
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// Look for a tab and set it to active.
|
|
/// </summary>
|
|
private void ActivateRemainingTab()
|
|
{
|
|
foreach (Bar myBar in dotNetBarManager1.Bars)
|
|
{
|
|
if (myBar.DockSide == eDockSide.Document && myBar.Visible)
|
|
{
|
|
ActivateRemainingTab(myBar);
|
|
return;
|
|
}
|
|
}
|
|
// No Document Tabs Remaining - need to raise OnItemSelectedChanged
|
|
OnItemSelectedChanged(this, null);
|
|
}
|
|
private DisplayTabItem FindRemainingTab(Bar myBar)
|
|
{
|
|
foreach (DisplayTabItem itm in myBar.Items)
|
|
{
|
|
if (!_RemovedDisplayTabItems.Contains(itm)) return itm;
|
|
}
|
|
return null;
|
|
}
|
|
private void ActivateRemainingTab(Bar myBar)
|
|
{
|
|
if (myBar.SelectedDockContainerItem != null)
|
|
{
|
|
if (_RemovedDisplayTabItems.Contains(myBar.SelectedDockContainerItem as DisplayTabItem))
|
|
myBar.SelectedDockContainerItem = FindRemainingTab(myBar);
|
|
myBar.SelectedDockContainerItem.Selected = true;
|
|
StepTabPanel pnl = myBar.SelectedDockContainerItem.Control as StepTabPanel;
|
|
if (pnl != null)
|
|
pnl.MyStepPanel.ItemShow();
|
|
}
|
|
}
|
|
#endregion
|
|
#region Public Properties
|
|
public DevComponents.DotNetBar.Bar MyBar
|
|
{
|
|
get { return _MyBar; }
|
|
}
|
|
#endregion
|
|
#region Private Methods
|
|
/// <summary>
|
|
/// Gets and sets the SelectedDisplayTabItem (Active Tab)
|
|
/// </summary>
|
|
public DisplayTabItem SelectedDisplayTabItem
|
|
{
|
|
get { return (DisplayTabItem)_MyBar.SelectedDockContainerItem; }
|
|
set
|
|
{
|
|
if (value != null)
|
|
{
|
|
value.Focus();
|
|
value.Selected = true;
|
|
}
|
|
}
|
|
}
|
|
/// <summary>
|
|
/// This returns the parent bar (current docking location) for an item.
|
|
/// It creates a bar if none exist
|
|
/// </summary>
|
|
/// <param name="myItemInfo"></param>
|
|
/// <returns></returns>
|
|
private Bar GetParentBar(ItemInfo myItemInfo)
|
|
{
|
|
Bar myBar = null;
|
|
foreach (Bar b in dotNetBarManager1.Bars)
|
|
{
|
|
if (b.DockSide == eDockSide.Document && b.Visible)
|
|
{
|
|
if (myBar == null) myBar = b;// Remember the first available bar if a specific bar cannot be found
|
|
foreach (object itm in b.Items)
|
|
{
|
|
DisplayTabItem myTabItem = itm as DisplayTabItem;
|
|
if (myTabItem != null && myTabItem.MyStepTabPanel != null)
|
|
if (myTabItem.MyStepTabPanel.MyProcedureItemInfo.ItemID == myItemInfo.MyProcedure.ItemID)
|
|
return b;
|
|
}
|
|
}
|
|
}
|
|
if (myBar == null)
|
|
{
|
|
// If no documents bars found, create new one
|
|
_UniqueBarCount++;
|
|
myBar = BarUtilities.CreateDocumentBar();
|
|
myBar.DockTabClosing += new DockTabClosingEventHandler(myBar_DockTabClosing);
|
|
myBar.Name = "barDocuments" + _UniqueBarCount.ToString();
|
|
fillDocDockSite.GetDocumentUIManager().Dock(myBar);
|
|
SetupBar(myBar);
|
|
}
|
|
return myBar;
|
|
}
|
|
/// <summary>
|
|
/// This opens a Step page based upon a ItemInfo.
|
|
///
|
|
/// </summary>
|
|
/// <param name="myItemInfo"></param>
|
|
/// <returns></returns>
|
|
private DisplayTabItem OpenStepTabPage(ItemInfo myItemInfo)
|
|
{
|
|
ItemInfo proc = myItemInfo.MyProcedure; // Find procedure Item
|
|
string key = "Item - " + proc.ItemID.ToString();
|
|
DisplayTabItem pg = null;
|
|
if (_MyDisplayTabItems.ContainsKey(key)) // If procedure page open use it
|
|
{
|
|
pg = _MyDisplayTabItems[key];
|
|
pg.Selected = true;
|
|
SelectedDisplayTabItem = pg;
|
|
}
|
|
else // If not already open, create a new Page
|
|
{
|
|
pg = new DisplayTabItem(this.components, this, proc, key); // Open a new Procedure Tab
|
|
_MyDisplayTabItems.Add(key, pg);
|
|
pg.Selected = true;
|
|
SelectedDisplayTabItem = pg;
|
|
pg.MyStepTabPanel.MyProcedureItemInfo = proc;
|
|
}
|
|
Application.DoEvents();
|
|
pg.SelectedItemInfo = myItemInfo; // Select the item
|
|
return pg;
|
|
}
|
|
/// <summary>
|
|
/// This opens a Word page based upon an itemInfo. Since a word document may be a library
|
|
/// document, the related DocID is used for the Word document.
|
|
/// </summary>
|
|
/// <param name="myItemInfo"></param>
|
|
/// <returns></returns>
|
|
private DisplayTabItem OpenDSOTabPage(ItemInfo myItemInfo)
|
|
{
|
|
DisplayTabItem pg = null;
|
|
EntryInfo myEntry = myItemInfo.MyContent.MyEntry;
|
|
string key = "Doc - " + myEntry.DocID;
|
|
if (_MyDisplayTabItems.ContainsKey(key)) // If document page open use it
|
|
{
|
|
pg = _MyDisplayTabItems[key];
|
|
if (pg.MyItemInfo.ItemID != myItemInfo.ItemID)
|
|
{
|
|
string msg = string.Format("{0} is already open", myItemInfo.MyContent.MyEntry.MyDocument.LibTitle);
|
|
MessageBox.Show(msg, "Library Document is Already Open", MessageBoxButtons.OK);
|
|
}
|
|
}
|
|
else
|
|
{
|
|
if (DSOTabPanel.Count > 18) // Limit the number of open document pages to 18
|
|
{
|
|
MessageBox.Show("Too many Word Documents Open. Please close one of the Documents before attempting to open another");
|
|
return null;
|
|
}
|
|
if ((myItemInfo.MyContent.MyEntry.MyDocument.LibTitle ?? "") != "")
|
|
MessageBox.Show("WARNING: All edits made to this Library Document will be applied to all uses of the Document");
|
|
pg = new DisplayTabItem(this.components, this, myItemInfo, key); // Open a new document page
|
|
_MyDisplayTabItems.Add(key, pg);
|
|
}
|
|
SelectedDisplayTabItem = pg;
|
|
pg.MyDSOTabPanel.EnterPanel();
|
|
return pg;
|
|
}
|
|
public DisplayTabItem OpenDSOTabPage(DocumentInfo myDocumentInfo)
|
|
{
|
|
CleanUpClosedItems();
|
|
DisplayTabItem pg = null;
|
|
string key = "Doc - " + myDocumentInfo.DocID;
|
|
if (_MyDisplayTabItems.ContainsKey(key)) // If document page open use it
|
|
pg = _MyDisplayTabItems[key];
|
|
else
|
|
{
|
|
if (DSOTabPanel.Count > 18) // Limit the number of open document pages to 18
|
|
{
|
|
MessageBox.Show("Too many Word Documents Open. Please close one of the Documents before attempting to open another");
|
|
return null;
|
|
}
|
|
pg = new DisplayTabItem(this.components, this, myDocumentInfo, key); // Open a new document page
|
|
_MyDisplayTabItems.Add(key, pg);
|
|
}
|
|
SelectedDisplayTabItem = pg;
|
|
pg.MyDSOTabPanel.EnterPanel();
|
|
return pg;
|
|
}
|
|
/// <summary>
|
|
/// Clean-up after a page is closed
|
|
/// </summary>
|
|
/// <param name="myDisplayTabItem"></param>
|
|
internal void RemoveItem(DisplayTabItem myDisplayTabItem)
|
|
{
|
|
_MyDisplayTabItems.Remove(myDisplayTabItem.MyKey);
|
|
// Dispose the Panel
|
|
if (myDisplayTabItem.MyStepTabPanel != null)
|
|
myDisplayTabItem.MyStepTabPanel.Dispose();
|
|
if (myDisplayTabItem.MyDSOTabPanel != null)
|
|
myDisplayTabItem.MyDSOTabPanel.CloseDSO();
|
|
components.Remove(myDisplayTabItem);
|
|
myDisplayTabItem.Dispose();
|
|
}
|
|
#endregion
|
|
}
|
|
}
|