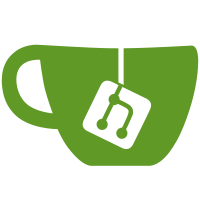
Use Font instead of SelectedFont which can be null if there are more than one font within the selected text
1058 lines
43 KiB
C#
1058 lines
43 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using System.Windows.Forms;
|
|
using System.Drawing;
|
|
using System.Runtime.InteropServices;
|
|
using System.ComponentModel;
|
|
|
|
namespace Volian.Controls.Library
|
|
{
|
|
public static class RTBAPI
|
|
{
|
|
#region SendMessage
|
|
[DllImport("user32.dll", SetLastError = true)]
|
|
private static extern int SendMessage(HandleRef hWndlock, Messages wMsg, Int32 wParam, ref Point pt);
|
|
[DllImport("user32.dll", SetLastError = true)]
|
|
private static extern int SendMessage(HandleRef hWndlock, Messages wMsg, Int32 wParam, int lParam);
|
|
[DllImport("user32.dll", CharSet = CharSet.Auto, SetLastError = true)]
|
|
public static extern int SendMessage(HandleRef hWndlock, Messages wMsg, RTBSelection wParam, ref CharFormat2 cf2);
|
|
[DllImport("user32.dll", CharSet = CharSet.Auto, SetLastError = true)]
|
|
public static extern int SendMessage(HandleRef hWndlock, Messages wMsg, int wParam, ref ParaFormat2 pf2);
|
|
#region RTB Messages
|
|
// EM_AUTOURLDETECT - An EM_AUTOURLDETECT message enables or disables automatic detection of URLs by a rich edit control.
|
|
// EM_CANPASTE - The EM_CANPASTE message determines whether a rich edit control can paste a specified clipboard format.
|
|
// EM_CANREDO - The EM_CANREDO message determines whether there are any actions in the control redo queue.
|
|
// EM_DISPLAYBAND - The EM_DISPLAYBAND message displays a portion of the contents of a rich edit control, as previously formatted for a device using the EM_FORMATRANGE message.
|
|
// EM_EXGETSEL - The EM_EXGETSEL message retrieves the starting and ending character positions of the selection in a rich edit control.
|
|
// EM_EXLIMITTEXT - The EM_EXLIMITTEXT message sets an upper limit to the amount of text the user can type or paste into a rich edit control.
|
|
// EM_EXLINEFROMCHAR - The EM_EXLINEFROMCHAR message determines which line contains the specified character in a rich edit control.
|
|
// EM_EXSETSEL - The EM_EXSETSEL message selects a range of characters or COM objects in a Rich Edit control.
|
|
// EM_FINDTEXT - The EM_FINDTEXT message finds text within a rich edit control.
|
|
// EM_FINDTEXTEX - The EM_FINDTEXTEX message finds text within a rich edit control.
|
|
// EM_FINDTEXTEXW - The EM_FINDTEXTEXW message finds Unicode text within a rich edit control.
|
|
// EM_FINDTEXTW - The EM_FINDTEXTW message finds Unicode text within a rich edit control.
|
|
// EM_FINDWORDBREAK - The EM_FINDWORDBREAK message finds the next word break before or after the specified character position or retrieves information about the character at that position.
|
|
// EM_FORMATRANGE - The EM_FORMATRANGE message formats a range of text in a rich edit control for a specific device.
|
|
// EM_GETAUTOURLDETECT - The EM_GETAUTOURLDETECT message indicates whether the auto URL detection is turned on in the rich edit control.
|
|
// EM_GETBIDIOPTIONS - The EM_GETBIDIOPTIONS message indicates the current state of the bidirectional options in the rich edit control.
|
|
// EM_GETCHARFORMAT - The EM_GETCHARFORMAT message determines the character formatting in a rich edit control.
|
|
// EM_GETCTFMODEBIAS - An application sends a EM_GETCTFMODEBIAS message to get the Text Services Framework mode bias values for a Rich Edit control.
|
|
// EM_GETCTFOPENSTATUS - An application sends an EM_GETCTFOPENSTATUS message to determine if the Text Services Framework (TSF) keyboard is open or closed.
|
|
// EM_GETEDITSTYLE - The EM_GETEDITSTYLE message retrieves the current edit style flags.
|
|
// EM_GETEVENTMASK - The EM_GETEVENTMASK message retrieves the event mask for a rich edit control. The event mask specifies which notification messages the control sends to its parent window.
|
|
// EM_GETHYPHENATEINFO - An application sends an EM_GETHYPHENATEINFO message to get information about hyphenation for a Rich Edit control.
|
|
// EM_GETIMECOLOR - The EM_GETIMECOLOR message retrieves the Input Method Editor (IME) composition color. This message is available only in Asian-language versions of the operating system.
|
|
// EM_GETIMECOMPMODE - An application sends an EM_GETIMECOMPMODE message to get the current IME mode for a rich edit control.
|
|
// EM_GETIMECOMPTEXT - An application sends an EM_GETIMECOMPTEXT message to get the IME composition text.
|
|
// EM_GETIMEMODEBIAS - An application sends an EM_GETIMEMODEBIAS message to get the IME mode bias for a Rich Edit control.
|
|
// EM_GETIMEOPTIONS - The EM_GETIMEOPTIONS message retrieves the current IME options. This message is available only in Asian-language versions of the operating system.
|
|
// EM_GETIMEPROPERTY - An application sends a EM_GETIMEPROPERTY message to get the property and capabilities of the IME associated with the current input locale.
|
|
// EM_GETLANGOPTIONS - An application sends an EM_GETLANGOPTIONS message to get a rich edit control's option settings for IME and Asian language support.
|
|
// EM_GETOLEINTERFACE - The EM_GETOLEINTERFACE message retrieves an IRichEditOle object that a client can use to access a rich edit control's COM functionality.
|
|
// EM_GETOPTIONS - The EM_GETOPTIONS message retrieves rich edit control options.
|
|
// EM_GETPAGEROTATE - Deprecated. An application sends an EM_GETPAGEROTATE message to get the text layout for a Rich Edit control.
|
|
// EM_GETPARAFORMAT - The EM_GETPARAFORMAT message retrieves the paragraph formatting of the current selection in a rich edit control.
|
|
// EM_GETPUNCTUATION - The EM_GETPUNCTUATION message gets the current punctuation characters for the rich edit control. This message is available only in Asian-language versions of the operating system.
|
|
// EM_GETREDONAME - An application sends an EM_GETREDONAME message to a rich edit control to retrieve the type of the next action, if any, in the control's redo queue.
|
|
// EM_GETSCROLLPOS - An application sends an EM_GETSCROLLPOS message to obtain the current scroll position of the edit control.
|
|
// EM_GETSELTEXT - The EM_GETSELTEXT message retrieves the currently selected text in a rich edit control.
|
|
// EM_GETTEXTEX - The EM_GETTEXTEX message allows you to get all of the text from the rich edit control in any particular code base you want.
|
|
// EM_GETTEXTLENGTHEX - The EM_GETTEXTLENGTHEX message calculates text length in various ways. It is usually called before creating a buffer to receive the text from the control.
|
|
// EM_GETTEXTMODE - An application sends an EM_GETTEXTMODE message to get the current text mode and undo level of a rich edit control.
|
|
// EM_GETTEXTRANGE - The EM_GETTEXTRANGE message retrieves a specified range of characters from a rich edit control.
|
|
// EM_GETTYPOGRAPHYOPTIONS - The EM_GETTYPOGRAPHYOPTIONS message returns the current state of the typography options of a rich edit control.
|
|
// EM_GETUNDONAME - Rich Edit 2.0 and later: An application sends an EM_GETUNDONAME message to a rich edit control to retrieve the type of the next undo action, if any.
|
|
// EM_GETWORDBREAKPROCEX - The EM_GETWORDBREAKPROCEX message retrieves the address of the currently registered extended word-break procedure.
|
|
// EM_GETWORDWRAPMODE - The EM_GETWORDWRAPMODE message gets the current word wrap and word-break options for the rich edit control. This message is available only in Asian-language versions of the operating system.
|
|
// EM_GETZOOM - The EM_GETZOOM message gets the current zoom ratio, which is always between 1/64 and 64.
|
|
// EM_HIDESELECTION - The EM_HIDESELECTION message hides or shows the selection in a rich edit control.
|
|
// EM_ISIME - An application sends a EM_ISIME message to determine if current input locale is an East Asian locale.
|
|
// EM_PASTESPECIAL - The EM_PASTESPECIAL message pastes a specific clipboard format in a rich edit control.
|
|
// EM_RECONVERSION - The EM_RECONVERSION message invokes the IME reconversion dialog box.
|
|
// EM_REDO - Send an EM_REDO message to a rich edit control to redo the next action in the control's redo queue.
|
|
// EM_REQUESTRESIZE - The EM_REQUESTRESIZE message forces a rich edit control to send an EN_REQUESTRESIZE notification message to its parent window.
|
|
// EM_SELECTIONTYPE - The EM_SELECTIONTYPE message determines the selection type for a rich edit control.
|
|
// EM_SETBIDIOPTIONS - The EM_SETBIDIOPTIONS message sets the current state of the bidirectional options in the rich edit control.
|
|
// EM_SETBKGNDCOLOR - The EM_SETBKGNDCOLOR message sets the background color for a rich edit control.
|
|
// EM_SETCHARFORMAT - The EM_SETCHARFORMAT message sets character formatting in a rich edit control.
|
|
// EM_SETCTFMODEBIAS - An application sends an EM_SETCTFMODEBIAS message to set the Text Services Framework (TSF) mode bias for a Rich Edit control.
|
|
// EM_SETCTFOPENSTATUS - An application sends an EM_SETCTFOPENSTATUS message to open or close the Text Services Framework (TSF) keyboard.
|
|
// EM_SETEDITSTYLE - The EM_SETEDITSTYLE message sets the current edit style flags.
|
|
// EM_SETEVENTMASK - The EM_SETEVENTMASK message sets the event mask for a rich edit control. The event mask specifies which notification messages the control sends to its parent window.
|
|
// EM_SETFONTSIZE - The EM_SETFONTSIZE message sets the font size for the selected text.
|
|
// EM_SETHYPHENATEINFO - An application sends an EM_SETHYPHENATEINFO message to set the way a Rich Edit control does hyphenation.
|
|
// EM_SETIMECOLOR - The EM_SETIMECOLOR message sets the IME composition color. This message is available only in Asian-language versions of the operating system.
|
|
// EM_SETIMEMODEBIAS - An application sends an EM_SETIMEMODEBIAS message to set the IME mode bias for a Rich Edit control.
|
|
// EM_SETIMEOPTIONS - The EM_SETIMEOPTIONS message sets the IME options. This message is available only in Asian-language versions of the operating system.
|
|
// EM_SETLANGOPTIONS - An application sends an EM_SETLANGOPTIONS message to set options for IME and Asian language support in a rich edit control.
|
|
// EM_SETOLECALLBACK - The EM_SETOLECALLBACK message gives a rich edit control an IRichEditOleCallback object that the control uses to get OLE-related resources and information from the client.
|
|
// EM_SETOPTIONS - The EM_SETOPTIONS message sets the options for a rich edit control.
|
|
// EM_SETPAGEROTATE - Deprecated. An application sends an EM_SETPAGEROTATE message to set the text layout for a Rich Edit control.
|
|
// EM_SETPALETTE - An application sends an EM_SETPALETTE message to change the palette that rich edit uses for its display window.
|
|
// EM_SETPARAFORMAT - The EM_SETPARAFORMAT message sets the paragraph formatting for the current selection in a rich edit control.
|
|
// EM_SETPUNCTUATION - The EM_SETPUNCTUATION message sets the punctuation characters for a rich edit control. This message is available only in Asian-language versions of the operating system.
|
|
// EM_SETSCROLLPOS - An application sends an EM_SETSCROLLPOS message to tell the rich edit control to scroll to a particular point.
|
|
// EM_SETTARGETDEVICE - The EM_SETTARGETDEVICE message sets the target device and line width used for "what you see is what you get" (WYSIWYG) formatting in a rich edit control.
|
|
// EM_SETTEXTEX - The EM_SETTEXTEX message combines the functionality of WM_SETTEXT and EM_REPLACESEL and adds the ability to set text using a code page and to use either rich text or plain text.
|
|
// EM_SETTEXTMODE - An application sends an EM_SETTEXTMODE message to set the text mode or undo level of a rich edit control. The message fails if the control contains any text.
|
|
// EM_SETTYPOGRAPHYOPTIONS - The EM_SETTYPOGRAPHYOPTIONS message sets the current state of the typography options of a rich edit control.
|
|
// EM_SETUNDOLIMIT - An application sends an EM_SETUNDOLIMIT message to a rich edit control to set the maximum number of actions that can stored in the undo queue.
|
|
// EM_SETWORDBREAKPROCEX - The EM_SETWORDBREAKPROCEX message sets the extended word-break procedure.
|
|
// EM_SETWORDWRAPMODE - The EM_SETWORDWRAPMODE message sets the word-wrapping and word-breaking options for the rich edit control. This message is available only in Asian-language versions of the operating system.
|
|
// EM_SETZOOM - The EM_SETZOOM message sets the zoom ratio anywhere between 1/64 and 64.
|
|
// EM_SHOWSCROLLBAR - The EM_SHOWSCROLLBAR message shows or hides one of the scroll bars in the Text Host window.
|
|
// EM_STOPGROUPTYPING - An application sends an EM_STOPGROUPTYPING message to a rich edit control to stop the control from collecting additional typing actions into the current undo action. The control stores the next typing action, if any, into a new action in the undo queue.
|
|
// EM_STREAMIN - The EM_STREAMIN message replaces the contents of a rich edit control with a stream of data provided by an application defined
|
|
// EM_STREAMOUT - The EM_STREAMOUT message causes a rich edit control to pass its contents to an application
|
|
#endregion
|
|
#endregion
|
|
#region Constants
|
|
//const int ECOOP_XOR = 0x0004;
|
|
|
|
//const int ECO_AUTOWORDSELECTION = 0x00000001;
|
|
|
|
|
|
const int WM_USER = 0x0400;
|
|
#endregion
|
|
#region Enums
|
|
public enum Messages : int
|
|
{
|
|
EM_AUTOURLDETECT = WM_USER + 91,
|
|
EM_CANPASTE = WM_USER + 50,
|
|
EM_CANREDO = WM_USER + 85,
|
|
EM_DISPLAYBAND = WM_USER + 51,
|
|
EM_EXGETSEL = WM_USER + 52,
|
|
EM_EXLIMITTEXT = WM_USER + 53,
|
|
EM_EXLINEFROMCHAR = WM_USER + 54,
|
|
EM_EXSETSEL = WM_USER + 55,
|
|
EM_FINDTEXT = WM_USER + 56,
|
|
EM_FINDTEXTEX = WM_USER + 79,
|
|
EM_FINDTEXTEXW = WM_USER + 124,
|
|
EM_FINDTEXTW = WM_USER + 123,
|
|
EM_FINDWORDBREAK = WM_USER + 76,
|
|
EM_FORMATRANGE = WM_USER + 57,
|
|
EM_GETAUTOURLDETECT = WM_USER + 92,
|
|
EM_GETBIDIOPTIONS = WM_USER + 201,
|
|
EM_GETCHARFORMAT = WM_USER + 58,
|
|
EM_GETCTFMODEBIAS = WM_USER + 237,
|
|
EM_GETCTFOPENSTATUS = WM_USER + 240,
|
|
EM_GETEDITSTYLE = WM_USER + 205,
|
|
EM_GETEVENTMASK = WM_USER + 59,
|
|
EM_GETHYPHENATEINFO = WM_USER + 230,
|
|
EM_GETIMECOLOR = WM_USER + 105,
|
|
EM_GETIMECOMPMODE = WM_USER + 122,
|
|
EM_GETIMECOMPTEXT = WM_USER + 242,
|
|
EM_GETIMEMODEBIAS = WM_USER + 127,
|
|
EM_GETIMEOPTIONS = WM_USER + 107,
|
|
EM_GETIMEPROPERTY = WM_USER + 244,
|
|
EM_GETLANGOPTIONS = WM_USER + 121,
|
|
EM_GETOLEINTERFACE = WM_USER + 60,
|
|
EM_GETOPTIONS = WM_USER + 78,
|
|
EM_GETPAGEROTATE = WM_USER + 235,
|
|
EM_GETPARAFORMAT = WM_USER + 61,
|
|
EM_GETPUNCTUATION = WM_USER + 101,
|
|
EM_GETREDONAME = WM_USER + 87,
|
|
EM_GETSCROLLPOS = WM_USER + 221,
|
|
EM_GETSELTEXT = WM_USER + 62,
|
|
EM_GETTEXTEX = WM_USER + 94,
|
|
EM_GETTEXTLENGTHEX = WM_USER + 95,
|
|
EM_GETTEXTMODE = WM_USER + 90,
|
|
EM_GETTEXTRANGE = WM_USER + 75,
|
|
EM_GETTYPOGRAPHYOPTIONS = WM_USER + 203,
|
|
EM_GETUNDONAME = WM_USER + 86,
|
|
EM_GETWORDBREAKPROCEX = WM_USER + 80,
|
|
EM_GETWORDWRAPMODE = WM_USER + 103,
|
|
EM_GETZOOM = WM_USER + 224,
|
|
EM_HIDESELECTION = WM_USER + 63,
|
|
EM_ISIME = WM_USER + 243,
|
|
EM_PASTESPECIAL = WM_USER + 64,
|
|
EM_RECONVERSION = WM_USER + 125,
|
|
EM_REDO = WM_USER + 84,
|
|
EM_REQUESTRESIZE = WM_USER + 65,
|
|
EM_SELECTIONTYPE = WM_USER + 66,
|
|
EM_SETBIDIOPTIONS = WM_USER + 200,
|
|
EM_SETBKGNDCOLOR = WM_USER + 67,
|
|
EM_SETCHARFORMAT = WM_USER + 68,
|
|
EM_SETCTFMODEBIAS = WM_USER + 238,
|
|
EM_SETCTFOPENSTATUS = WM_USER + 241,
|
|
EM_SETEDITSTYLE = WM_USER + 204,
|
|
EM_SETEVENTMASK = WM_USER + 69,
|
|
EM_SETFONTSIZE = WM_USER + 223,
|
|
EM_SETHYPHENATEINFO = WM_USER + 231,
|
|
EM_SETIMECOLOR = WM_USER + 104,
|
|
EM_SETIMEMODEBIAS = WM_USER + 126,
|
|
EM_SETIMEOPTIONS = WM_USER + 106,
|
|
EM_SETLANGOPTIONS = WM_USER + 120,
|
|
EM_SETOLECALLBACK = WM_USER + 70,
|
|
EM_SETOPTIONS = WM_USER + 77,
|
|
EM_SETPAGEROTATE = WM_USER + 236,
|
|
EM_SETPALETTE = WM_USER + 93,
|
|
EM_SETPARAFORMAT = WM_USER + 71,
|
|
EM_SETPUNCTUATION = WM_USER + 100,
|
|
EM_SETSCROLLPOS = WM_USER + 222,
|
|
EM_SETTARGETDEVICE = WM_USER + 72,
|
|
EM_SETTEXTEX = WM_USER + 97,
|
|
EM_SETTEXTMODE = WM_USER + 89,
|
|
EM_SETTYPOGRAPHYOPTIONS = WM_USER + 202,
|
|
EM_SETUNDOLIMIT = WM_USER + 82,
|
|
EM_SETWORDBREAKPROCEX = WM_USER + 81,
|
|
EM_SETWORDWRAPMODE = WM_USER + 102,
|
|
EM_SETZOOM = WM_USER + 225,
|
|
EM_SHOWSCROLLBAR = WM_USER + 96,
|
|
EM_STOPGROUPTYPING = WM_USER + 88,
|
|
EM_STREAMIN = WM_USER + 73,
|
|
EM_STREAMOUT = WM_USER + 74
|
|
};
|
|
[Flags]
|
|
public enum LanguageOptions : int // Options for EM_SETLANGOPTIONS and EM_GETLANGOPTIONS
|
|
{
|
|
IMF_AUTOKEYBOARD = 0x0001,
|
|
IMF_AUTOFONT = 0x0002,
|
|
IMF_IMECANCELCOMPLETE = 0x0004, // high completes the comp string when aborting, low cancels.
|
|
IMF_IMEALWAYSSENDNOTIFY = 0x0008
|
|
}
|
|
public enum TimeCompMode : int // Values for EM_GETIMECOMPMODE
|
|
{
|
|
ICM_NOTOPEN = 0x0000,
|
|
ICM_LEVEL3 = 0x0001,
|
|
ICM_LEVEL2 = 0x0002,
|
|
ICM_LEVEL2_5 = 0x0003,
|
|
ICM_LEVEL2_SUI = 0x0004
|
|
}
|
|
[Flags]
|
|
public enum EditControlOptions : int // Edit control options
|
|
{
|
|
ECO_AUTOWORDSELECTION = 0x00000001,
|
|
ECO_AUTOVSCROLL = 0x00000040,
|
|
ECO_AUTOHSCROLL = 0x00000080,
|
|
ECO_NOHIDESEL = 0x00000100,
|
|
ECO_READONLY = 0x00000800,
|
|
ECO_WANTRETURN = 0x00001000,
|
|
ECO_SAVESEL = 0x00008000,
|
|
ECO_SELECTIONBAR = 0x01000000,
|
|
ECO_VERTICAL = 0x00400000, // FE specific
|
|
}
|
|
public enum EcoOperations : int // ECO operations
|
|
{
|
|
ECOOP_SET = 0x0001,
|
|
ECOOP_OR = 0x0002,
|
|
ECOOP_AND = 0x0003,
|
|
ECOOP_XOR = 0x0004
|
|
}
|
|
public enum WordBreakFunctionActions : int // new word break function actions
|
|
{
|
|
WB_CLASSIFY = 3,
|
|
WB_MOVEWORDLEFT = 4,
|
|
WB_MOVEWORDRIGHT = 5,
|
|
WB_LEFTBREAK = 6,
|
|
WB_RIGHTBREAK = 7
|
|
}
|
|
//#define PC_FOLLOWING 1
|
|
//#define PC_LEADING 2
|
|
//#define PC_OVERFLOW 3
|
|
//#define PC_DELIMITER 4
|
|
//#define WBF_WORDWRAP 0x010
|
|
//#define WBF_WORDBREAK 0x020
|
|
//#define WBF_OVERFLOW 0x040
|
|
//#define WBF_LEVEL1 0x080
|
|
//#define WBF_LEVEL2 0x100
|
|
//#define WBF_CUSTOM 0x200
|
|
/* Word break flags (used with WB_CLASSIFY) */
|
|
//#define WBF_CLASS ((BYTE) 0x0F)
|
|
//#define WBF_ISWHITE ((BYTE) 0x10)
|
|
//#define WBF_BREAKLINE ((BYTE) 0x20)
|
|
//#define WBF_BREAKAFTER ((BYTE) 0x40)
|
|
///* Far East specific flags */
|
|
//#define IMF_FORCENONE 0x0001
|
|
//#define IMF_FORCEENABLE 0x0002
|
|
//#define IMF_FORCEDISABLE 0x0004
|
|
//#define IMF_CLOSESTATUSWINDOW 0x0008
|
|
//#define IMF_VERTICAL 0x0020
|
|
//#define IMF_FORCEACTIVE 0x0040
|
|
//#define IMF_FORCEINACTIVE 0x0080
|
|
//#define IMF_FORCEREMEMBER 0x0100
|
|
//#define IMF_MULTIPLEEDIT 0x0400
|
|
public enum RTBSelection : int
|
|
{
|
|
SCF_DEFAULT = 0x0000,
|
|
SCF_SELECTION = 0x0001,
|
|
SCF_WORD = 0x0002,
|
|
SCF_ALL = 0x0004,
|
|
SCF_USEUIRULES = 0x0008
|
|
}
|
|
[Flags]
|
|
public enum CharFormatMasks : uint
|
|
{
|
|
CFM_NONE = 0,
|
|
CFM_BOLD = 0x00000001,
|
|
CFM_ITALIC = 0x00000002,
|
|
CFM_UNDERLINE = 0x00000004,
|
|
CFM_STRIKEOUT = 0x00000008,
|
|
CFM_PROTECTED = 0x00000010,
|
|
CFM_LINK = 0x00000020, // Exchange hyperlink extension
|
|
CFM_SIZE = 0x80000000,
|
|
CFM_COLOR = 0x40000000,
|
|
CFM_FACE = 0x20000000,
|
|
CFM_OFFSET = 0x10000000,
|
|
CFM_CHARSET = 0x08000000,
|
|
CFM_SMALLCAPS = 0x0040,
|
|
CFM_ALLCAPS = 0x0080,
|
|
CFM_HIDDEN = 0x0100,
|
|
CFM_OUTLINE = 0x0200,
|
|
CFM_SHADOW = 0x0400,
|
|
CFM_EMBOSS = 0x0800,
|
|
CFM_IMPRINT = 0x1000,
|
|
CFM_DISABLED = 0x2000,
|
|
CFM_REVISED = 0x4000,
|
|
CFM_BACKCOLOR = 0x04000000,
|
|
CFM_LCID = 0x02000000,
|
|
CFM_UNDERLINETYPE = 0x00800000,
|
|
CFM_WEIGHT = 0x00400000,
|
|
CFM_SPACING = 0x00200000,
|
|
CFM_KERNING = 0x00100000,
|
|
CFM_STYLE = 0x00080000,
|
|
CFM_ANIMATION = 0x00040000,
|
|
CFM_REVAUTHOR = 0x00008000,
|
|
CFM_SUBSCRIPT = 0x00010000,
|
|
CFM_SUPERSCRIPT = 0x00020000
|
|
// CFM_SUBorSUPERSCRIPT = 0x00030000
|
|
}
|
|
[Flags]
|
|
public enum CharFormatEffects : uint
|
|
{
|
|
CFE_NONE = 0,
|
|
CFE_BOLD = 0x0001,
|
|
CFE_ITALIC = 0x0002,
|
|
CFE_UNDERLINE = 0x0004,
|
|
CFE_STRIKEOUT = 0x0008,
|
|
CFE_PROTECTED = 0x0010,
|
|
CFE_LINK = 0x0020,
|
|
CFE_AUTOBACKCOLOR = 0x04000000, // NOTE: this corresponds to CFM_BACKCOLOR, which controls it
|
|
CFE_AUTOCOLOR = 0x40000000, // NOTE: this corresponds to CFM_COLOR, which controls it
|
|
CFE_SUBSCRIPT = 0x00010000, // Superscript and subscript are
|
|
CFE_SUPERSCRIPT = 0x00020000, // mutually exclusive
|
|
CFE_SMALLCAPS = 0x0040,
|
|
CFE_ALLCAPS = 0x0080,
|
|
CFE_HIDDEN = 0x0100,
|
|
CFE_OUTLINE = 0x0200,
|
|
CFE_SHADOW = 0x0400,
|
|
CFE_EMBOSS = 0x0800,
|
|
CFE_IMPRINT = 0x1000,
|
|
CFE_DISABLED = 0x2000,
|
|
CFE_REVISED = 0x4000
|
|
}
|
|
public enum CharUnderline : byte
|
|
{
|
|
CFU_UNDERLINENONE = 0,
|
|
CFU_UNDERLINE = 1,
|
|
CFU_UNDERLINEWORD = 2, // displayed as ordinary underline
|
|
CFU_UNDERLINEDOUBLE = 3, // displayed as ordinary underline
|
|
CFU_UNDERLINEDOTTED = 4,
|
|
CFU_UNDERLINEDASH = 5,
|
|
CFU_UNDERLINEDASHDOT = 6,
|
|
CFU_UNDERLINEDASHDOTDOT = 7,
|
|
CFU_UNDERLINEWAVE = 8,
|
|
CFU_UNDERLINETHICK = 9,
|
|
CFU_INVERT = 0xFE // For IME composition fake a selection.
|
|
}
|
|
[Flags]
|
|
public enum ParaFormatMasks : uint
|
|
{
|
|
PFM_NONE = 0,
|
|
PFM_STARTINDENT = 0x00000001,
|
|
PFM_RIGHTINDENT = 0x00000002,
|
|
PFM_OFFSET = 0x00000004,
|
|
PFM_ALIGNMENT = 0x00000008,
|
|
PFM_TABSTOPS = 0x00000010,
|
|
PFM_NUMBERING = 0x00000020,
|
|
PFM_SPACEBEFORE = 0x00000040,
|
|
PFM_SPACEAFTER = 0x00000080,
|
|
PFM_LINESPACING = 0x00000100,
|
|
PFM_RESERVED = 0x00000200,
|
|
PFM_STYLE = 0x00000400,
|
|
PFM_BORDER = 0x00000800,
|
|
PFM_SHADING = 0x00001000,
|
|
PFM_NUMBERINGSTYLE = 0x00002000,
|
|
PFM_NUMBERINGTAB = 0x00004000,
|
|
PFM_NUMBERINGSTART = 0x00008000,
|
|
// PFM_DIR = 0x00010000,
|
|
PFM_RTLPARA = 0x00010000,
|
|
PFM_KEEP = 0x00020000,
|
|
PFM_KEEPNEXT = 0x00040000,
|
|
PFM_PAGEBREAKBEFORE = 0x00080000,
|
|
PFM_NOLINENUMBER = 0x00100000,
|
|
PFM_NOWIDOWCONTROL = 0x00200000,
|
|
PFM_DONOTHYPHEN = 0x00400000,
|
|
PFM_SIDEBYSIDE = 0x00800000,
|
|
PFM_TABLEROWDELIMITER = 0x10000000,
|
|
PFM_TEXTWRAPPINGBREAK = 0x20000000,
|
|
PFM_TABLE = 0x40000000,
|
|
PFM_OFFSETINDENT = 0x80000000
|
|
}
|
|
public enum ParaFormatEffects : ushort
|
|
{
|
|
PFE_RTLPARA = 0x0001,
|
|
PFE_KEEP = 0x0002,
|
|
PFE_KEEPNEXT = 0x0004,
|
|
PFE_PAGEBREAKBEFORE = 0x0008,
|
|
PFE_NOLINENUMBER = 0x0010,
|
|
PFE_NOWIDOWCONTROL = 0x0020,
|
|
PFE_DONOTHYPHEN = 0x0040,
|
|
PFE_SIDEBYSIDE = 0x0080,
|
|
PFE_TABLEROW = 0xc000, /* These 3 options are mutually */
|
|
PFE_TABLECELLEND = 0x8000, /* exclusive and each imply */
|
|
PFE_TABLECELL = 0x4000, /* that para is part of a table*/
|
|
}
|
|
public enum ParaAlignment : ushort
|
|
{
|
|
PFA_LEFT = 1,
|
|
PFA_RIGHT = 2,
|
|
PFA_CENTER = 3,
|
|
PFA_JUSTIFY = 4,
|
|
PFA_FULL_INTERWORD = 5
|
|
}
|
|
public enum ParaNumbering : ushort
|
|
{
|
|
PFN_NONE = 0,
|
|
PFN_BULLET = 1,
|
|
PFN_ARABIC = 2,
|
|
PFN_LOWER = 3,
|
|
PFN_UPPER = 4,
|
|
PFN_LOWERROMAN = 5,
|
|
PFN_UPPERROMAN = 6,
|
|
PFN_UNI = 7
|
|
}
|
|
public enum ParaSpacing : byte
|
|
{
|
|
PFS_SINGLE = 0,
|
|
PFS_ONEANDONEHALF = 1,
|
|
PFS_DOUBLE = 2,
|
|
PFS_EXACTSINGLEMIN = 3,
|
|
PFS_EXACT = 4,
|
|
PFS_EXACT20 = 5
|
|
}
|
|
[Flags]
|
|
public enum E_FontStyle : byte
|
|
{
|
|
FS_NONE = 0,
|
|
FS_BOLD = 0x01,
|
|
FS_UNDERLINE = 0x02,
|
|
FS_ITALIC = 0x04,
|
|
FS_SUPERSCRIPT = 0x08,
|
|
FS_SUBSCRIPT = 0x10
|
|
}
|
|
#endregion
|
|
#region Structures
|
|
//struct CharRange
|
|
//{
|
|
// public int cpMin;
|
|
// public int cpMax;
|
|
//}
|
|
[StructLayout(LayoutKind.Sequential, Pack = 4, CharSet = CharSet.Auto)]
|
|
public struct CharFormat2
|
|
{
|
|
public int cbSize;
|
|
public uint dwMask;
|
|
public uint dwEffects;
|
|
public int yHeight;
|
|
public int yOffset;
|
|
public int crTextColor;
|
|
public byte bCharSet;
|
|
public byte bPitchAndFamily;
|
|
[MarshalAs(UnmanagedType.ByValTStr, SizeConst = 32)]
|
|
public string szFaceName;
|
|
public short wWeight;
|
|
public short sSpacing;
|
|
public int crBackColor;
|
|
public int lcid;
|
|
public int dwReserved;
|
|
public short sStyle;
|
|
public short wKerning;
|
|
public byte bUnderlineType;
|
|
public byte bAnimation;
|
|
public byte bRevAuthor;
|
|
public byte bReserved1;
|
|
}
|
|
public class CharFormatTwo
|
|
{
|
|
public CharFormatTwo(CharFormat2 cf)
|
|
{
|
|
_CharFormat2 = cf;
|
|
}
|
|
private CharFormat2 _CharFormat2;
|
|
[Browsable(false)]
|
|
public CharFormat2 CharFormat2
|
|
{
|
|
get { return _CharFormat2; }
|
|
}
|
|
[Browsable(false)]
|
|
public int cbSize
|
|
{
|
|
get { return _CharFormat2.cbSize; }
|
|
set { _CharFormat2.cbSize = value; }
|
|
}
|
|
[Editor(typeof(FlagEnumUIEditor), typeof(System.Drawing.Design.UITypeEditor))]
|
|
public CharFormatMasks dwMask
|
|
{
|
|
get { return (CharFormatMasks)_CharFormat2.dwMask; }
|
|
set { _CharFormat2.dwMask = (uint)value; }
|
|
}
|
|
[Editor(typeof(FlagEnumUIEditor), typeof(System.Drawing.Design.UITypeEditor))]
|
|
public CharFormatEffects dwEffects
|
|
{
|
|
get { return (CharFormatEffects)_CharFormat2.dwEffects; }
|
|
set { _CharFormat2.dwEffects = (uint)value; }
|
|
}
|
|
public int yHeight
|
|
{
|
|
get { return _CharFormat2.yHeight; }
|
|
set { _CharFormat2.yHeight = value; }
|
|
}
|
|
public int yOffset
|
|
{
|
|
get { return _CharFormat2.yOffset; }
|
|
set { _CharFormat2.yOffset = value; }
|
|
}
|
|
private Color Int2Color(int color)
|
|
{
|
|
return Color.FromArgb(color % 256, (color / 256) % 256, color / (256 * 256));
|
|
}
|
|
private int Color2Int(Color color)
|
|
{
|
|
return color.R + (color.G * 256) + (color.B * 256 * 256);
|
|
}
|
|
public Color crTextColor
|
|
{
|
|
get { return Int2Color(_CharFormat2.crTextColor); }
|
|
set { _CharFormat2.crTextColor = Color2Int(value); }
|
|
}
|
|
public byte bCharSet
|
|
{
|
|
get { return _CharFormat2.bCharSet; }
|
|
set { _CharFormat2.bCharSet = value; }
|
|
}
|
|
public byte bPitchAndFamily
|
|
{
|
|
get { return _CharFormat2.bPitchAndFamily; }
|
|
set { _CharFormat2.bPitchAndFamily = value; }
|
|
}
|
|
public string szFaceName
|
|
{
|
|
get { return _CharFormat2.szFaceName; }
|
|
set { _CharFormat2.szFaceName = value; }
|
|
}
|
|
public short wWeight
|
|
{
|
|
get { return _CharFormat2.wWeight; }
|
|
set { _CharFormat2.wWeight = value; }
|
|
}
|
|
public short sSpacing
|
|
{
|
|
get { return _CharFormat2.sSpacing; }
|
|
set { _CharFormat2.sSpacing = value; }
|
|
}
|
|
public Color crBackColor
|
|
{
|
|
get { return Int2Color(_CharFormat2.crBackColor); }
|
|
set { _CharFormat2.crBackColor = Color2Int(value); }
|
|
}
|
|
public int lcid
|
|
{
|
|
get { return _CharFormat2.lcid; }
|
|
set { _CharFormat2.lcid = value; }
|
|
}
|
|
public int dwReserved
|
|
{
|
|
get { return _CharFormat2.dwReserved; }
|
|
set { _CharFormat2.dwReserved = value; }
|
|
}
|
|
public short sStyle
|
|
{
|
|
get { return _CharFormat2.sStyle; }
|
|
set { _CharFormat2.sStyle = value; }
|
|
}
|
|
public short wKerning
|
|
{
|
|
get { return _CharFormat2.wKerning; }
|
|
set { _CharFormat2.wKerning = value; }
|
|
}
|
|
public CharUnderline bUnderlineType
|
|
{
|
|
get { return (CharUnderline)_CharFormat2.bUnderlineType; }
|
|
set { _CharFormat2.bUnderlineType = (byte)value; }
|
|
}
|
|
public byte bAnimation
|
|
{
|
|
get { return _CharFormat2.bAnimation; }
|
|
set { _CharFormat2.bAnimation = value; }
|
|
}
|
|
public byte bRevAuthor
|
|
{
|
|
get { return _CharFormat2.bRevAuthor; }
|
|
set { _CharFormat2.bRevAuthor = value; }
|
|
}
|
|
public byte bReserved1
|
|
{
|
|
get { return _CharFormat2.bReserved1; }
|
|
set { _CharFormat2.bReserved1 = value; }
|
|
}
|
|
}
|
|
[StructLayout(LayoutKind.Sequential, Pack = 4)]
|
|
public struct ParaFormat2
|
|
{
|
|
public int cbSize;
|
|
public int dwMask;
|
|
public ushort wNumbering;
|
|
public short wEffects;
|
|
public int dxStartIndent;
|
|
public int dxRightIndent;
|
|
public int dxOffset;
|
|
public short wAlignment;
|
|
public short cTabCount;
|
|
[MarshalAs(UnmanagedType.ByValArray, SizeConst = 32)]
|
|
public int[] rgxTabs;
|
|
public int dySpaceBefore;
|
|
public int dySpaceAfter;
|
|
public int dyLineSpacing;
|
|
public short sStyle;
|
|
public byte bLineSpacingRule;
|
|
public byte bOutlineLevel;
|
|
public short wShadingWeight;
|
|
public short wShadingStyle;
|
|
public short wNumberingStart;
|
|
public short wNumberingStyle;
|
|
public short wNumberingTab;
|
|
public short wBorderSpace;
|
|
public short wBorderWidth;
|
|
public short wBorders;
|
|
}
|
|
public class ParaFormatTwo
|
|
{
|
|
private ParaFormat2 _ParaFormat2;
|
|
public ParaFormatTwo(ParaFormat2 pf)
|
|
{
|
|
_ParaFormat2 = pf;
|
|
}
|
|
public ParaFormat2 ParaFormat2
|
|
{
|
|
get { return _ParaFormat2; }
|
|
}
|
|
public int cbSize
|
|
{
|
|
get { return _ParaFormat2.cbSize; }
|
|
set { _ParaFormat2.cbSize = value; }
|
|
}
|
|
[Editor(typeof(FlagEnumUIEditor), typeof(System.Drawing.Design.UITypeEditor))]
|
|
public ParaFormatMasks dwMask
|
|
{
|
|
get { return (ParaFormatMasks)_ParaFormat2.dwMask; }
|
|
set { _ParaFormat2.dwMask = (int)value; }
|
|
}
|
|
public ParaNumbering wNumbering
|
|
{
|
|
get { return (ParaNumbering)_ParaFormat2.wNumbering; }
|
|
set { _ParaFormat2.wNumbering = (ushort)value; }
|
|
}
|
|
public ParaFormatEffects wEffects
|
|
{
|
|
get { return (ParaFormatEffects)_ParaFormat2.wEffects; }
|
|
set { _ParaFormat2.wEffects = (short)value; }
|
|
}
|
|
public int dxStartIndent
|
|
{
|
|
get { return _ParaFormat2.dxStartIndent; }
|
|
set { _ParaFormat2.dxStartIndent = value; }
|
|
}
|
|
public int dxRightIndent
|
|
{
|
|
get { return _ParaFormat2.dxRightIndent; }
|
|
set { _ParaFormat2.dxRightIndent = value; }
|
|
}
|
|
public int dxOffset
|
|
{
|
|
get { return _ParaFormat2.dxOffset; }
|
|
set { _ParaFormat2.dxOffset = value; }
|
|
}
|
|
public ParaAlignment wAlignment
|
|
{
|
|
get { return (ParaAlignment)_ParaFormat2.wAlignment; }
|
|
set { _ParaFormat2.wAlignment = (short)value; }
|
|
}
|
|
public short cTabCount
|
|
{
|
|
get { return _ParaFormat2.cTabCount; }
|
|
set { _ParaFormat2.cTabCount = value; }
|
|
}
|
|
public int[] rgxTabs
|
|
{
|
|
get { return _ParaFormat2.rgxTabs; }
|
|
set { _ParaFormat2.rgxTabs = value; }
|
|
}
|
|
public int dySpaceBefore
|
|
{
|
|
get { return _ParaFormat2.dySpaceBefore; }
|
|
set { _ParaFormat2.dySpaceBefore = value; }
|
|
}
|
|
public int dySpaceAfter
|
|
{
|
|
get { return _ParaFormat2.dySpaceAfter; }
|
|
set { _ParaFormat2.dySpaceAfter = value; }
|
|
}
|
|
public int dyLineSpacing
|
|
{
|
|
get { return _ParaFormat2.dyLineSpacing; }
|
|
set { _ParaFormat2.dyLineSpacing = value; }
|
|
}
|
|
public short sStyle
|
|
{
|
|
get { return _ParaFormat2.sStyle; }
|
|
set { _ParaFormat2.sStyle = value; }
|
|
}
|
|
public ParaSpacing bLineSpacingRule
|
|
{
|
|
get { return (ParaSpacing)_ParaFormat2.bLineSpacingRule; }
|
|
set { _ParaFormat2.bLineSpacingRule = (byte)value; }
|
|
}
|
|
public byte bOutlineLevel
|
|
{
|
|
get { return _ParaFormat2.bOutlineLevel; }
|
|
set { _ParaFormat2.bOutlineLevel = value; }
|
|
}
|
|
public short wShadingWeight
|
|
{
|
|
get { return _ParaFormat2.wShadingWeight; }
|
|
set { _ParaFormat2.wShadingWeight = value; }
|
|
}
|
|
public short wShadingStyle
|
|
{
|
|
get { return _ParaFormat2.wShadingStyle; }
|
|
set { _ParaFormat2.wShadingStyle = value; }
|
|
}
|
|
public short wNumberingStart
|
|
{
|
|
get { return _ParaFormat2.wNumberingStart; }
|
|
set { _ParaFormat2.wNumberingStart = value; }
|
|
}
|
|
public short wNumberingStyle
|
|
{
|
|
get { return _ParaFormat2.wNumberingStyle; }
|
|
set { _ParaFormat2.wNumberingStyle = value; }
|
|
}
|
|
public short wNumberingTab
|
|
{
|
|
get { return _ParaFormat2.wNumberingTab; }
|
|
set { _ParaFormat2.wNumberingTab = value; }
|
|
}
|
|
public short wBorderSpace
|
|
{
|
|
get { return _ParaFormat2.wBorderSpace; }
|
|
set { _ParaFormat2.wBorderSpace = value; }
|
|
}
|
|
public short wBorderWidth
|
|
{
|
|
get { return _ParaFormat2.wBorderWidth; }
|
|
set { _ParaFormat2.wBorderWidth = value; }
|
|
}
|
|
public short wBorders
|
|
{
|
|
get { return _ParaFormat2.wBorders; }
|
|
set { _ParaFormat2.wBorders = value; }
|
|
}
|
|
}
|
|
#endregion
|
|
#region Static Methods
|
|
public static void SetScrollLocation(RichTextBox richTextBox, Point point)
|
|
{
|
|
if (SendMessage(new HandleRef(richTextBox, richTextBox.Handle), Messages.EM_SETSCROLLPOS, 0, ref point) == 0)
|
|
throw new Win32Exception();
|
|
}
|
|
public static Point GetScrollLocation(RichTextBox richTextBox)
|
|
{
|
|
Point pt = new Point();
|
|
if (SendMessage(new HandleRef(richTextBox, richTextBox.Handle), Messages.EM_GETSCROLLPOS, 0, ref pt) == 0)
|
|
throw new Win32Exception();
|
|
return pt;
|
|
}
|
|
public static void SetBackgroundColor(RichTextBox richTextBox, Color color)
|
|
{
|
|
int clr = color.R + (color.G * 256) + (color.B * 256 * 256);
|
|
if (SendMessage(new HandleRef(richTextBox, richTextBox.Handle), Messages.EM_SETBKGNDCOLOR, 0, clr) == 0)
|
|
throw new Win32Exception();
|
|
}
|
|
public static void SetAuto(RichTextBox richTextBox)
|
|
{
|
|
if (SendMessage(new HandleRef(richTextBox, richTextBox.Handle), Messages.EM_SETOPTIONS, (int)EcoOperations.ECOOP_XOR, (int)EditControlOptions.ECO_AUTOWORDSELECTION) == 0)
|
|
throw new Win32Exception();
|
|
}
|
|
public static ParaFormatTwo GetParaFormat(RichTextBox richTextBox)
|
|
{
|
|
ParaFormat2 pf = new ParaFormat2();
|
|
pf.cbSize = Marshal.SizeOf(pf);
|
|
if (SendMessage(new HandleRef(richTextBox, richTextBox.Handle), Messages.EM_GETPARAFORMAT, 0, ref pf) == 0)
|
|
throw new Win32Exception();
|
|
return new ParaFormatTwo(pf);
|
|
}
|
|
public static void SetParaFormat(RichTextBox richTextBox, ParaFormatTwo pft)
|
|
{
|
|
ParaFormat2 pf2 = pft.ParaFormat2;
|
|
if (SendMessage(new HandleRef(richTextBox, richTextBox.Handle), Messages.EM_SETPARAFORMAT, 0, ref pf2) == 0)
|
|
{
|
|
//if(Marshal.GetLastWin32Error()!=0)
|
|
throw new Win32Exception();
|
|
}
|
|
}
|
|
public static CharFormatTwo GetCharFormat(RichTextBox richTextBox, RTBSelection selection)
|
|
{
|
|
CharFormat2 cf = new CharFormat2();
|
|
cf.cbSize = Marshal.SizeOf(cf);
|
|
if (SendMessage(new HandleRef(richTextBox, richTextBox.Handle), Messages.EM_GETCHARFORMAT, selection, ref cf) == 0)
|
|
throw new Win32Exception();
|
|
return new CharFormatTwo(cf);
|
|
}
|
|
public static void SetCharFormat(RichTextBox richTextBox, RTBSelection selection, CharFormatTwo cft)
|
|
{
|
|
try
|
|
{
|
|
CharFormat2 cf2 = cft.CharFormat2;
|
|
try
|
|
{
|
|
HandleRef hr = new HandleRef(richTextBox, richTextBox.Handle);
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
Console.WriteLine(ex.Message);
|
|
}
|
|
if (SendMessage(new HandleRef(richTextBox, richTextBox.Handle), Messages.EM_SETCHARFORMAT, selection, ref cf2) == 0)
|
|
throw new Win32Exception();
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
Console.WriteLine("Error on SetCharFormat {0}", ex.Message);
|
|
}
|
|
|
|
}
|
|
public static void SetHighlightColor(RichTextBox richTextBox, RTBSelection selection, Color color)
|
|
{
|
|
CharFormatTwo cft = GetCharFormat(richTextBox, selection);
|
|
cft.crBackColor = color;
|
|
cft.dwEffects = 0;
|
|
cft.dwMask = 0;
|
|
cft.dwMask |= CharFormatMasks.CFM_BACKCOLOR;
|
|
SetCharFormat(richTextBox, selection, cft);
|
|
}
|
|
public static void SetLineSpacing(RichTextBox richTextBox, ParaSpacing type)
|
|
{
|
|
ParaFormatTwo pft = GetParaFormat(richTextBox);
|
|
pft.bLineSpacingRule = type;
|
|
pft.dwMask = 0;
|
|
pft.dwMask |= ParaFormatMasks.PFM_LINESPACING;
|
|
pft.dwMask |= ParaFormatMasks.PFM_SPACEAFTER;
|
|
|
|
// get the monitor's resolution in DPI and use it to set the linespacing value for
|
|
// the richtextbox. Note that without this, the Arial Unicode font made the appearance of
|
|
// almost double linespacing. Using PFS_Exact makes it appear as regular single spacing.
|
|
Graphics g = richTextBox.CreateGraphics();
|
|
int dpi = Convert.ToInt32((g.DpiX + g.DpiY) / 2);
|
|
g.Dispose();
|
|
// dyLineSpacing is Spacing between lines. the PFS_EXACT sets line spacing as the spacing from one
|
|
//line to the next, in twips - thus the 1440.
|
|
|
|
pft.dyLineSpacing = Convert.ToInt32(.5 + richTextBox.Font.GetHeight(dpi)) * 1440 / dpi;
|
|
SetParaFormat(richTextBox, pft);
|
|
}
|
|
public static E_FontStyle GetFontStyle(RichTextBox richTextBox)
|
|
{
|
|
E_FontStyle fs = E_FontStyle.FS_NONE;
|
|
CharFormatTwo cft = GetCharFormat(richTextBox, RTBSelection.SCF_SELECTION);
|
|
if (((cft.dwMask & CharFormatMasks.CFM_BOLD) == CharFormatMasks.CFM_BOLD) &&
|
|
((cft.dwEffects & CharFormatEffects.CFE_BOLD) == CharFormatEffects.CFE_BOLD)) fs |= E_FontStyle.FS_BOLD;
|
|
if (((cft.dwMask & CharFormatMasks.CFM_UNDERLINE) == CharFormatMasks.CFM_UNDERLINE) &&
|
|
((cft.dwEffects & CharFormatEffects.CFE_UNDERLINE) == CharFormatEffects.CFE_UNDERLINE)) fs |= E_FontStyle.FS_UNDERLINE;
|
|
if (((cft.dwMask & CharFormatMasks.CFM_ITALIC) == CharFormatMasks.CFM_ITALIC) &&
|
|
((cft.dwEffects & CharFormatEffects.CFE_ITALIC) == CharFormatEffects.CFE_ITALIC)) fs |= E_FontStyle.FS_ITALIC;
|
|
if (richTextBox.SelectionCharOffset == -2) fs |= E_FontStyle.FS_SUBSCRIPT;
|
|
if (richTextBox.SelectionCharOffset == 2) fs |= E_FontStyle.FS_SUPERSCRIPT;
|
|
return fs;
|
|
}
|
|
public static void SetFontStyle(RichTextBox richTextBox, E_FontStyle fs)
|
|
{
|
|
CharFormatTwo cft = GetCharFormat(richTextBox, RTBSelection.SCF_SELECTION);
|
|
if ((fs & E_FontStyle.FS_BOLD) == E_FontStyle.FS_BOLD)
|
|
{
|
|
cft.dwEffects |= CharFormatEffects.CFE_BOLD;
|
|
cft.dwMask |= CharFormatMasks.CFM_BOLD;
|
|
}
|
|
if ((fs & E_FontStyle.FS_UNDERLINE) == E_FontStyle.FS_UNDERLINE)
|
|
{
|
|
cft.dwEffects |= CharFormatEffects.CFE_UNDERLINE;
|
|
cft.dwMask |= CharFormatMasks.CFM_UNDERLINE;
|
|
}
|
|
if ((fs & E_FontStyle.FS_ITALIC) == E_FontStyle.FS_ITALIC)
|
|
{
|
|
cft.dwEffects |= CharFormatEffects.CFE_ITALIC;
|
|
cft.dwMask |= CharFormatMasks.CFM_ITALIC;
|
|
}
|
|
if ((fs & E_FontStyle.FS_SUBSCRIPT) == E_FontStyle.FS_SUBSCRIPT)
|
|
{
|
|
richTextBox.SelectionCharOffset = -2;
|
|
}
|
|
if ((fs & E_FontStyle.FS_SUPERSCRIPT) == E_FontStyle.FS_SUPERSCRIPT)
|
|
{
|
|
richTextBox.SelectionCharOffset = 2;
|
|
}
|
|
SetCharFormat(richTextBox, RTBSelection.SCF_SELECTION, cft);
|
|
}
|
|
public static bool IsSuperScript(RichTextBox richTextBox)
|
|
{
|
|
return (richTextBox.SelectionCharOffset>0);
|
|
}
|
|
public static bool IsSubScript(RichTextBox richTextBox)
|
|
{
|
|
return (richTextBox.SelectionCharOffset < 0);
|
|
}
|
|
public static void ToggleSubscript(bool bSet, RichTextBox richTextBox, RTBSelection selection)
|
|
{
|
|
if (bSet)
|
|
richTextBox.SelectionCharOffset = -2;
|
|
else
|
|
richTextBox.SelectionCharOffset = 0;
|
|
}
|
|
public static void ToggleSuperscript(bool bSet, RichTextBox richTextBox, RTBSelection selection)
|
|
{
|
|
if (bSet)
|
|
richTextBox.SelectionCharOffset = 2;
|
|
else
|
|
richTextBox.SelectionCharOffset = 0;
|
|
}
|
|
public static bool IsBold(RichTextBox richTextBox)
|
|
{
|
|
CharFormatTwo cft = GetCharFormat(richTextBox, RTBSelection.SCF_SELECTION);
|
|
return (((cft.dwMask & CharFormatMasks.CFM_BOLD) == CharFormatMasks.CFM_BOLD) &&
|
|
((cft.dwEffects & CharFormatEffects.CFE_BOLD) == CharFormatEffects.CFE_BOLD));
|
|
}
|
|
public static void ToggleBold(bool bSet, RichTextBox richTextBox, RTBSelection selection)
|
|
{
|
|
CharFormatTwo cft = GetCharFormat(richTextBox, selection);
|
|
if (bSet)
|
|
{
|
|
cft.dwEffects = CharFormatEffects.CFE_BOLD;
|
|
cft.dwMask = CharFormatMasks.CFM_BOLD;
|
|
}
|
|
else
|
|
{
|
|
cft.dwEffects = CharFormatEffects.CFE_NONE;
|
|
cft.dwMask = CharFormatMasks.CFM_BOLD;
|
|
}
|
|
SetCharFormat(richTextBox, selection, cft);
|
|
}
|
|
public static bool IsUnderline(RichTextBox richTextBox)
|
|
{
|
|
CharFormatTwo cft = GetCharFormat(richTextBox, RTBSelection.SCF_SELECTION);
|
|
return (((cft.dwMask & CharFormatMasks.CFM_UNDERLINE) == CharFormatMasks.CFM_UNDERLINE) &&
|
|
((cft.dwEffects & CharFormatEffects.CFE_UNDERLINE) == CharFormatEffects.CFE_UNDERLINE));
|
|
}
|
|
public static void ToggleUnderline(bool bSet, RichTextBox richTextBox, RTBSelection selection)
|
|
{
|
|
CharFormatTwo cft = GetCharFormat(richTextBox, selection);
|
|
if (bSet)
|
|
{
|
|
cft.dwEffects = CharFormatEffects.CFE_UNDERLINE;
|
|
cft.dwMask = CharFormatMasks.CFM_UNDERLINE;
|
|
}
|
|
else
|
|
{
|
|
cft.dwEffects = CharFormatEffects.CFE_NONE;
|
|
cft.dwMask = CharFormatMasks.CFM_UNDERLINE;
|
|
}
|
|
SetCharFormat(richTextBox, selection, cft);
|
|
}
|
|
public static bool IsItalic(RichTextBox richTextBox)
|
|
{
|
|
CharFormatTwo cft = GetCharFormat(richTextBox, RTBSelection.SCF_SELECTION);
|
|
return (((cft.dwMask & CharFormatMasks.CFM_ITALIC) == CharFormatMasks.CFM_ITALIC) &&
|
|
((cft.dwEffects & CharFormatEffects.CFE_ITALIC) == CharFormatEffects.CFE_ITALIC));
|
|
}
|
|
public static void ToggleItalic(bool bSet, RichTextBox richTextBox, RTBSelection selection)
|
|
{
|
|
CharFormatTwo cft = GetCharFormat(richTextBox, selection);
|
|
if (bSet)
|
|
{
|
|
cft.dwEffects = CharFormatEffects.CFE_ITALIC;
|
|
cft.dwMask = CharFormatMasks.CFM_ITALIC;
|
|
}
|
|
else
|
|
{
|
|
cft.dwEffects = CharFormatEffects.CFE_NONE;
|
|
cft.dwMask = CharFormatMasks.CFM_ITALIC;
|
|
}
|
|
SetCharFormat(richTextBox, selection, cft);
|
|
}
|
|
//public static bool IsLink(RichTextBox richTextBox)
|
|
//{
|
|
// CharFormatTwo cft = GetCharFormat(richTextBox, RTBSelection.SCF_SELECTION);
|
|
// return ((cft.dwEffects & CharFormatEffects.CFE_PROTECTED) == CharFormatEffects.CFE_PROTECTED);
|
|
//}
|
|
//public static void ToggleLink(bool bSet, RichTextBox richTextBox, RTBSelection selection)
|
|
//{
|
|
// CharFormatTwo cft = GetCharFormat(richTextBox, selection);
|
|
// if (bSet)
|
|
// {
|
|
// cft.dwEffects |= CharFormatEffects.CFE_LINK;
|
|
// cft.dwMask |= CharFormatMasks.CFM_LINK;
|
|
// }
|
|
// else
|
|
// {
|
|
// cft.dwEffects &= ~RTBAPI.CharFormatEffects.CFE_LINK;
|
|
// }
|
|
// SetCharFormat(richTextBox, selection, cft);
|
|
//}
|
|
//public static void UnProtect(RichTextBox richTextBox) //, string type, string text, string link)
|
|
//{
|
|
// //richTextBox.DetectUrls = false;
|
|
// CharFormatTwo cft = GetCharFormat(richTextBox, RTBAPI.RTBSelection.SCF_SELECTION); ;
|
|
// //int position = richTextBox.SelectionStart = richTextBox.TextLength;
|
|
// //richTextBox.SelectionLength = 0;
|
|
// //richTextBox.SelectedRtf = @"{\rtf1\ansi " + text + @"\v #" + link + @"\v0}";
|
|
// //richTextBox.SelectedRtf = "{" + string.Format(@"\rtf1\ansi\protect\v {0}\v0 {1}\v #{2}", type, text, link) + "}";
|
|
// //richTextBox.Select(position, type.Length + text.Length + link.Length + 1);
|
|
// cft.dwMask = RTBAPI.CharFormatMasks.CFM_PROTECTED;
|
|
// cft.dwEffects = 0;
|
|
// // The lines below can be used to allow link text to be edited
|
|
// //charFormat.dwMask = RTBAPI.CharFormatMasks.CFM_LINK;
|
|
// //charFormat.dwEffects = RTBAPI.CharFormatEffects.CFE_LINK;
|
|
// SetCharFormat(richTextBox, RTBSelection.SCF_SELECTION, cft);
|
|
// //rtbRTF.SetSelectionLink(true);
|
|
// //richTextBox.SelectionStart = richTextBox.TextLength;
|
|
// //richTextBox.SelectionLength = 0;
|
|
//}
|
|
//public static void Protect(RichTextBox richTextBox) //, string type, string text, string link)
|
|
//{
|
|
// CharFormatTwo cft = GetCharFormat(richTextBox, RTBAPI.RTBSelection.SCF_SELECTION); ;
|
|
// cft.dwMask = CharFormatMasks.CFM_PROTECTED;
|
|
// cft.dwEffects = CharFormatEffects.CFE_PROTECTED;
|
|
// SetCharFormat(richTextBox, RTBSelection.SCF_SELECTION, cft);
|
|
//}
|
|
//public static void SetLink(RichTextBox richTextBox, string type, string text, string link)
|
|
//{
|
|
// richTextBox.DetectUrls = false;
|
|
// CharFormatTwo cft = GetCharFormat(richTextBox, RTBAPI.RTBSelection.SCF_SELECTION); ;
|
|
// int position = richTextBox.SelectionStart = richTextBox.TextLength;
|
|
// richTextBox.SelectionLength = 0;
|
|
// //richTextBox.SelectedRtf = @"{\rtf1\ansi " + text + @"\v #" + link + @"\v0}";
|
|
// richTextBox.SelectedRtf = "{" + string.Format(@"\rtf1\ansi\protect\v {0}\v0 {1}\v #{2}", type, text, link) + "}";
|
|
// richTextBox.Select(position, type.Length + text.Length + link.Length + 1);
|
|
// cft.dwMask = RTBAPI.CharFormatMasks.CFM_LINK | RTBAPI.CharFormatMasks.CFM_PROTECTED;
|
|
// cft.dwEffects = RTBAPI.CharFormatEffects.CFE_LINK | RTBAPI.CharFormatEffects.CFE_PROTECTED;
|
|
// // The lines below can be used to allow link text to be edited
|
|
// //charFormat.dwMask = RTBAPI.CharFormatMasks.CFM_LINK;
|
|
// //charFormat.dwEffects = RTBAPI.CharFormatEffects.CFE_LINK;
|
|
// SetCharFormat(richTextBox, RTBSelection.SCF_SELECTION, cft);
|
|
// //rtbRTF.SetSelectionLink(true);
|
|
// richTextBox.SelectionStart = richTextBox.TextLength;
|
|
// richTextBox.SelectionLength = 0;
|
|
//}
|
|
#endregion
|
|
}
|
|
}
|