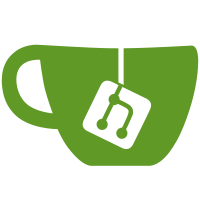
Fixed format of ToString for AnnotationAuditInfo Fixed format of ToString for ContentAuditInfo Fixed Restore if no next item Fixed ActiveParent logic after restore Added Timing logic and then Commented it Fixed Logic when there is not a NextNode Timing Debug code Update UserID when saving annotation Commented Timing Debug Created and used FixPath - StepPath Use MyItemInfo.SearchPath if Content doesn't have path Refresh Change List from Timer.Tick Added Timer for Refresh Added code to refresh ChangeBar Added and Commented timing debug Changed logic for saving ROUsages Added Closed Property that is set when the handle is destroyed Don't refresh StepRTB if it is closed
320 lines
9.2 KiB
C#
320 lines
9.2 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.ComponentModel;
|
|
using System.Drawing;
|
|
using System.Data;
|
|
using System.Text;
|
|
using System.Windows.Forms;
|
|
using VEPROMS.CSLA.Library;
|
|
using Volian.Base.Library;
|
|
|
|
namespace Volian.Controls.Library
|
|
{
|
|
public partial class AnnotationDetails : UserControl
|
|
{
|
|
#region Properties
|
|
private bool _LoadingAnnotation = false;
|
|
private bool _LoadingGrid = false;
|
|
|
|
private ItemInfo _CurrentItem = null;
|
|
private DisplaySearch _AnnotationSearch;
|
|
|
|
private AnnotationInfoList _Annotations;
|
|
public AnnotationInfoList Annotations
|
|
{
|
|
get { return _Annotations; }
|
|
set
|
|
{
|
|
_Annotations = value;
|
|
itemAnnotationsBindingSource.DataSource = _Annotations;
|
|
}
|
|
}
|
|
|
|
private AnnotationInfo _CurrentAnnotation = null;
|
|
public AnnotationInfo CurrentAnnotation
|
|
{
|
|
get { return _CurrentAnnotation; }
|
|
set
|
|
{
|
|
if (_CurrentAnnotation == null && value == null) return; // No Change
|
|
if (_CurrentAnnotation != null && value != null)
|
|
if (_CurrentAnnotation.AnnotationID == value.AnnotationID) return; // No Change
|
|
//vlnStackTrace.ShowStack("CurrentAnnotation = '{0}' Old = '{1}'", value, _CurrentAnnotation);
|
|
if (_CurrentAnnotation != null || _AddingAnnotation)
|
|
{
|
|
if (AnnotationDirty)
|
|
SaveAnnotation();
|
|
}
|
|
_CurrentAnnotation = value;
|
|
InitializeAnnotation();
|
|
}
|
|
}
|
|
|
|
private bool _AnnotationDirty = false;
|
|
private bool AnnotationDirty
|
|
{
|
|
get { return _AnnotationDirty; }
|
|
set
|
|
{
|
|
btnRemoveAnnotation.Enabled = btnAddAnnotation.Enabled = !value;
|
|
btnSaveAnnotation.Enabled = btnCancelAnnoation.Enabled = value;
|
|
_AddingAnnotation = value && (CurrentAnnotation == null);
|
|
_AnnotationDirty = value;
|
|
}
|
|
}
|
|
|
|
public string AnnotationText
|
|
{
|
|
get { return rtxbComment.Text; }
|
|
set
|
|
{
|
|
rtxbComment.Text = value;
|
|
if (rtxbComment.Text != string.Empty)
|
|
rtxbComment.SelectionStart = rtxbComment.TextLength; // position cursor to end of text
|
|
}
|
|
}
|
|
|
|
public string AnnotationRTFText
|
|
{
|
|
get { return rtxbComment.Rtf; }
|
|
set
|
|
{
|
|
rtxbComment.Rtf = value;
|
|
if (rtxbComment.Rtf != string.Empty)
|
|
rtxbComment.SelectionStart = rtxbComment.TextLength; // position cursor to end of text
|
|
}
|
|
}
|
|
|
|
#endregion
|
|
|
|
#region Constructors
|
|
public AnnotationDetails()
|
|
{
|
|
InitializeComponent();
|
|
//#if(DEBUG)
|
|
//Resize+=new EventHandler(AnnotationDetails_Resize); // Debug the resize event
|
|
//#endif
|
|
}
|
|
#endregion
|
|
|
|
#region Events
|
|
private bool _AddingAnnotation = false;
|
|
private void btnAddAnnotation_Click(object sender, EventArgs e)
|
|
{
|
|
dgAnnotations.ClearSelection();
|
|
CurrentAnnotation = null;
|
|
_AddingAnnotation = true;
|
|
rtxbComment.Focus();
|
|
}
|
|
|
|
private void btnRemoveAnnotation_Click(object sender, EventArgs e)
|
|
{
|
|
//using (Annotation annotation = CurrentAnnotation.Get())
|
|
//{
|
|
// annotation.Delete();
|
|
_AnnotationSearch.LoadingList = true;
|
|
Annotation.DeleteAnnotation(CurrentAnnotation);
|
|
// annotation.Save();
|
|
_AnnotationSearch.LoadingList = false;
|
|
CurrentAnnotation = null;
|
|
UpdateAnnotationGrid();
|
|
//_AnnotationSearch.UpdateAnnotationSearchResults(); // update the search results
|
|
//}
|
|
}
|
|
|
|
private void btnSaveAnnotation_Click(object sender, EventArgs e)
|
|
{
|
|
if (cbGridAnnoType.SelectedIndex == -1)
|
|
{
|
|
MessageBox.Show("You Must Select an Annotation Type", "Annotation Type Not Selected", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
|
|
cbGridAnnoType.Focus();
|
|
return;
|
|
}
|
|
if (rtxbComment.Text == string.Empty)
|
|
{
|
|
MessageBox.Show("You Must Enter Annotation Text", "Annotation Text Is Blank", MessageBoxButtons.OK, MessageBoxIcon.Exclamation);
|
|
rtxbComment.Focus();
|
|
return;
|
|
}
|
|
SaveAnnotation();
|
|
|
|
}
|
|
|
|
private void btnCancelAnnoation_Click(object sender, EventArgs e)
|
|
{
|
|
InitializeAnnotation();
|
|
}
|
|
|
|
private void cbGridAnnoType_SelectedValueChanged(object sender, EventArgs e)
|
|
{
|
|
if (!_LoadingAnnotation)
|
|
AnnotationDirty = true;
|
|
}
|
|
|
|
private void dgAnnotations_CellClick(object sender, DataGridViewCellEventArgs e)
|
|
{
|
|
if (!_LoadingGrid) // Only set the Current Annotation when not loading the grid
|
|
{
|
|
if ((_Annotations != null) && (dgAnnotations.Rows.Count > 0))
|
|
CurrentAnnotation = _Annotations[dgAnnotations.CurrentRow.Index];
|
|
else
|
|
CurrentAnnotation = null;
|
|
}
|
|
}
|
|
|
|
private void rtxbComment_TextChanged(object sender, EventArgs e)
|
|
{
|
|
if (!_LoadingAnnotation)
|
|
AnnotationDirty = true;
|
|
}
|
|
|
|
#endregion
|
|
|
|
#region LoadControlData
|
|
|
|
public void SetupAnnotations(DisplaySearch annosrch)
|
|
{
|
|
_LoadingAnnotation = true;
|
|
_AnnotationSearch = annosrch; // reference the Annotation Search to update its lists
|
|
|
|
cbGridAnnoType.DisplayMember = "Name";
|
|
cbGridAnnoType.ValueMember = "TypeId";
|
|
cbGridAnnoType.DataSource = AnnotationTypeInfoList.Get().Clone();
|
|
cbGridAnnoType.SelectedIndex = -1; //don't pre-select an annotation type
|
|
_LoadingAnnotation = false;
|
|
}
|
|
|
|
private void InitializeAnnotation()
|
|
{
|
|
//vlnCSLAStackTrace.ShowStack("InitializeAnnotation - CurrentAnnotation = {0}", CurrentAnnotation);
|
|
_LoadingAnnotation = true;
|
|
if (CurrentAnnotation == null)
|
|
{
|
|
cbGridAnnoType.SelectedIndex = -1;
|
|
AnnotationText = "";
|
|
}
|
|
else
|
|
{
|
|
cbGridAnnoType.SelectedValue = CurrentAnnotation.TypeID;
|
|
if (CurrentAnnotation.RtfText != "")
|
|
AnnotationRTFText = CurrentAnnotation.RtfText;
|
|
else
|
|
AnnotationText = CurrentAnnotation.SearchText;
|
|
}
|
|
_LoadingAnnotation = false;
|
|
AnnotationDirty = false;
|
|
if (!_LoadingGrid)
|
|
rtxbComment.Focus(); // Set the focus to the comment text
|
|
}
|
|
|
|
#endregion
|
|
|
|
#region VariousSupportMethods
|
|
|
|
/// <summary>
|
|
/// Set up the Annotation Grid for the given item
|
|
/// This is called from frmVEPROMS
|
|
/// </summary>
|
|
/// <param name="currentitem"></param>
|
|
public void UpdateAnnotationGrid(ItemInfo currentitem)
|
|
{
|
|
_CurrentItem = currentitem;
|
|
UpdateAnnotationGrid();
|
|
}
|
|
|
|
private void UpdateAnnotationGrid()
|
|
{
|
|
_LoadingGrid = true;
|
|
|
|
if (_CurrentItem == null)
|
|
_Annotations= null;
|
|
else
|
|
{
|
|
_CurrentItem.RefreshItemAnnotations();
|
|
_Annotations = _CurrentItem.ItemAnnotations;
|
|
}
|
|
itemAnnotationsBindingSource.DataSource = _Annotations;
|
|
dgAnnotations.Refresh();
|
|
if ((CurrentAnnotation == null || (_CurrentItem.ItemID != CurrentAnnotation.ItemID)))
|
|
{
|
|
if (_Annotations != null && _Annotations.Count > 0)
|
|
CurrentAnnotation = _Annotations[0];
|
|
else
|
|
CurrentAnnotation = null;
|
|
}
|
|
FindCurrentAnnotation(); // position to the grid row of the current annotation
|
|
_LoadingGrid = false;
|
|
if (_Annotations == null || _Annotations.Count == 0) btnRemoveAnnotation.Enabled = false;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Find the Current Annotation in the Annotation Grid select the corresponding row
|
|
/// Note: this is also called from AnnotationSearch.cs when a search results is selected
|
|
/// </summary>
|
|
public void FindCurrentAnnotation()
|
|
{
|
|
int row = 0;
|
|
if (CurrentAnnotation != null)
|
|
{
|
|
if (_Annotations != null)
|
|
{
|
|
foreach (AnnotationInfo ai in _Annotations)
|
|
{
|
|
if (ai.AnnotationID == CurrentAnnotation.AnnotationID)
|
|
{
|
|
row = _Annotations.IndexOf(ai);// +1;
|
|
break;
|
|
}
|
|
}
|
|
dgAnnotations.Rows[row].Selected = true;
|
|
if (!_LoadingGrid && (dgAnnotations.FirstDisplayedScrollingRowIndex != -1))
|
|
dgAnnotations.FirstDisplayedScrollingRowIndex = row;
|
|
}
|
|
}
|
|
}
|
|
|
|
private void SaveAnnotation()
|
|
{
|
|
if (cbGridAnnoType.SelectedIndex == -1) return;
|
|
if (rtxbComment.Text == string.Empty) return;
|
|
using (AnnotationType annotationType = AnnotationType.Get((int)cbGridAnnoType.SelectedValue))
|
|
{
|
|
if (_AddingAnnotation)
|
|
{
|
|
_AddingAnnotation = false;
|
|
using (Item myItem = _CurrentItem.Get())
|
|
{
|
|
using (Annotation annotation = Annotation.MakeAnnotation(myItem, annotationType, rtxbComment.Rtf, rtxbComment.Text, ""))
|
|
{
|
|
CurrentAnnotation = AnnotationInfo.Get(annotation.AnnotationID);
|
|
//annotation.DTS = DateTime.Now;
|
|
//annotation.Save();
|
|
}
|
|
}
|
|
}
|
|
else
|
|
{
|
|
using (Annotation annotation = CurrentAnnotation.Get())
|
|
{
|
|
annotation.RtfText = rtxbComment.Rtf;
|
|
annotation.SearchText = rtxbComment.Text;
|
|
annotation.MyAnnotationType = annotationType;
|
|
annotation.DTS = DateTime.Now;
|
|
annotation.UserID = Volian.Base.Library.VlnSettings.UserID;
|
|
annotation.Save();
|
|
}
|
|
}
|
|
}
|
|
AnnotationDirty = false;
|
|
UpdateAnnotationGrid();
|
|
}
|
|
#endregion
|
|
|
|
//private void AnnotationDetails_Resize(object sender, EventArgs e)
|
|
//{
|
|
// vlnStackTrace.ShowStackLocal(string.Format("Resize - Height = {0}", Height), 3, 4);
|
|
// //vlnStackTrace.ShowStack(string.Format("Resize - Height = {0}", Height));
|
|
//}
|
|
}
|
|
}
|