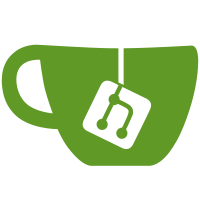
Fixed format of ToString for AnnotationAuditInfo Fixed format of ToString for ContentAuditInfo Fixed Restore if no next item Fixed ActiveParent logic after restore Added Timing logic and then Commented it Fixed Logic when there is not a NextNode Timing Debug code Update UserID when saving annotation Commented Timing Debug Created and used FixPath - StepPath Use MyItemInfo.SearchPath if Content doesn't have path Refresh Change List from Timer.Tick Added Timer for Refresh Added code to refresh ChangeBar Added and Commented timing debug Changed logic for saving ROUsages Added Closed Property that is set when the handle is destroyed Don't refresh StepRTB if it is closed
56 lines
1.3 KiB
C#
56 lines
1.3 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
|
|
namespace Volian.Base.Library
|
|
{
|
|
public class VlnTimer
|
|
{
|
|
private DateTime _LastTime = DateTime.Now;
|
|
public DateTime LastTime
|
|
{
|
|
get { return _LastTime; }
|
|
set { _LastTime = value; }
|
|
}
|
|
private string _LastProcess = "Initialize";
|
|
public string LastProcess
|
|
{
|
|
get { return _LastProcess; }
|
|
set { _LastProcess = value; }
|
|
}
|
|
public string ActiveProcess
|
|
{
|
|
get { return _LastProcess; }
|
|
set
|
|
{
|
|
DateTime tNow = DateTime.Now;
|
|
TimeSpan ts = tNow - LastTime;
|
|
LastTime = tNow;
|
|
if (!ElapsedTimes.ContainsKey(LastProcess))
|
|
ElapsedTimes.Add(LastProcess, ts);
|
|
else
|
|
ElapsedTimes[LastProcess] += ts;
|
|
LastProcess = value;
|
|
}
|
|
}
|
|
Dictionary<string, TimeSpan> _ElapsedTimes = new Dictionary<string, TimeSpan>();
|
|
public Dictionary<string, TimeSpan> ElapsedTimes
|
|
{
|
|
get { return _ElapsedTimes; }
|
|
set { _ElapsedTimes = value; }
|
|
}
|
|
public void ShowElapsedTimes()
|
|
{
|
|
ActiveProcess = "fini";
|
|
Console.WriteLine("'Process'\t'Elapsed'");
|
|
foreach (string proc in ElapsedTimes.Keys)
|
|
Console.WriteLine("'{0}'\t{1}", proc, ElapsedTimes[proc].TotalSeconds);
|
|
}
|
|
public void ShowElapsedTimes(string title)
|
|
{
|
|
Console.WriteLine(title);
|
|
ShowElapsedTimes();
|
|
}
|
|
}
|
|
}
|