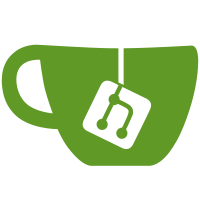
Added DocVersionInfo property to ROFSTLookup class Set DocVersionInfo to DocVersionInfo of ROFstInfo object in ROFSTLookup constructor Added Reset method to ROFSTLookup class Changed return values for ROID parameter of GetRoValue method Changes SectionConfig class to implement IItemConfig interface Added MasterSlave_Applicability property to IItemConfig Changes StepConfig class to implement IItemConfig interface Added MasterSlave_Applicability property to IItemConfig Changed GetGroup method to support multi units
282 lines
6.8 KiB
C#
282 lines
6.8 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using System.ComponentModel;
|
|
using DescriptiveEnum;
|
|
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
[Serializable]
|
|
[TypeConverter(typeof(ExpandableObjectConverter))]
|
|
public class StepConfig : ConfigDynamicTypeDescriptor, INotifyPropertyChanged, IItemConfig
|
|
{
|
|
#region DynamicTypeDescriptor
|
|
internal override bool IsReadOnly
|
|
{
|
|
get { return false; }//_Section == null; }
|
|
}
|
|
#endregion
|
|
#region XML
|
|
private XMLProperties _Xp;
|
|
private XMLProperties Xp
|
|
{
|
|
get { return _Xp; }
|
|
}
|
|
#endregion
|
|
#region Constructors
|
|
//PROPGRID: Hide ParentLookup
|
|
[Browsable(false)]
|
|
public bool ParentLookup
|
|
{
|
|
get { return _Xp.ParentLookup; }
|
|
set { _Xp.ParentLookup = value; }
|
|
}
|
|
//PROPGRID: Had to comment out NonSerialized to hide AncestorLookup from Property Grid
|
|
//[NonSerialized]
|
|
//private bool _AncestorLookup;
|
|
////PROPGRID: Hide AncestorLookup
|
|
//[Browsable(false)]
|
|
//public bool AncestorLookup
|
|
//{
|
|
// get { return _AncestorLookup; }
|
|
// set { _AncestorLookup = value; }
|
|
//}
|
|
private Step _Step;
|
|
private StepInfo _StepInfo;
|
|
public StepConfig(Step step)
|
|
{
|
|
_Step = step;
|
|
string xml = step.MyContent.Config;
|
|
if (xml == string.Empty) xml = "<Config/>";
|
|
_Xp = new XMLProperties(xml);
|
|
}
|
|
public StepConfig(StepInfo stepInfo)
|
|
{
|
|
_StepInfo = stepInfo;
|
|
string xml = stepInfo.MyContent.Config;
|
|
if (xml == string.Empty) xml = "<Config/>";
|
|
_Xp = new XMLProperties(xml);
|
|
}
|
|
public StepConfig(string xml)
|
|
{
|
|
if (xml == string.Empty) xml = "<Config/>";
|
|
_Xp = new XMLProperties(xml);
|
|
}
|
|
public StepConfig()
|
|
{
|
|
_Xp = new XMLProperties();
|
|
}
|
|
internal string GetValue(string group, string item)
|
|
{
|
|
return _Xp[group, item];
|
|
}
|
|
#endregion
|
|
#region Local Properties
|
|
|
|
#endregion
|
|
#region ToString
|
|
public override string ToString()
|
|
{
|
|
string s = _Xp.ToString();
|
|
if (s == "<Config/>" || s == "<Config></Config>") return string.Empty;
|
|
return s;
|
|
}
|
|
#endregion
|
|
#region StepAttr
|
|
//[Category("Step Attributes")]
|
|
//[DisplayName("Step Floating Foldout Association")]
|
|
//[RefreshProperties(RefreshProperties.All)]
|
|
//[Description("Step Floating Foldout Association")]
|
|
public int Step_FloatingFoldout
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["Step", "FloatingFoldout"];
|
|
if (s == string.Empty) return 0;
|
|
int tst = 0;
|
|
try
|
|
{
|
|
tst = int.Parse(s);
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
return 0;
|
|
}
|
|
return int.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
string s = _Xp["Step", "FloatingFoldout"];
|
|
if (value.ToString() == s) return;
|
|
_Xp["Step", "FloatingFoldout"] = value.ToString();
|
|
OnPropertyChanged("Step_FloatingFoldout");
|
|
}
|
|
}
|
|
//[Category("Step Attributes")]
|
|
//[DisplayName("Step Continuous Action Summary")]
|
|
//[RefreshProperties(RefreshProperties.All)]
|
|
//[Description("Step Continuous Action Summary")]
|
|
public bool Step_CAS
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["Step", "ContActSum"];
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty) return false;
|
|
if (s == "True") return true;
|
|
return false;
|
|
}
|
|
set
|
|
{
|
|
string s = _Xp["Step", "ContActSum"];
|
|
if (value.ToString() == s) return;
|
|
_Xp["Step", "ContActSum"] = value.ToString();
|
|
OnPropertyChanged("Step_CAS");
|
|
}
|
|
}
|
|
//[Category("Step Attributes")]
|
|
//[DisplayName("Step Check Off Index")]
|
|
//[RefreshProperties(RefreshProperties.All)]
|
|
//[Description("Step Check Off Index")]
|
|
public int Step_CheckOffIndex
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["Step", "CheckOffIndex"];
|
|
|
|
if (s == string.Empty) return 0;
|
|
|
|
// there was an invalid character for Wolf Creek's index. just return
|
|
// a 0 if found. The dataloader was fixed (6/8/12) to not migrate the
|
|
// bad character, but this was added, in case there are some other conditions.
|
|
int tst = 0;
|
|
try
|
|
{
|
|
tst = int.Parse(s);
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
return 0;
|
|
}
|
|
return int.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
string s = _Xp["Step", "CheckOffIndex"];
|
|
if (value.ToString() == s) return;
|
|
_Xp["Step", "CheckOffIndex"] = value.ToString();
|
|
OnPropertyChanged("Step_CheckOffIndex");
|
|
}
|
|
}
|
|
//[Category("Step Attributes")]
|
|
//[DisplayName("Step Manual Pagebreak")]
|
|
//[RefreshProperties(RefreshProperties.All)]
|
|
//[Description("Step Manual Pagebreak")]
|
|
public bool Step_ManualPagebreak
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["Step", "ManualPagebreak"];
|
|
|
|
if (s == string.Empty) return false;
|
|
if (s == "True") return true;
|
|
return false;
|
|
}
|
|
set
|
|
{
|
|
string s = _Xp["Step", "ManualPagebreak"];
|
|
if (value.ToString() == s) return;
|
|
_Xp["Step", "ManualPagebreak"] = value.ToString();
|
|
OnPropertyChanged("Step_ManualPagebreak");
|
|
}
|
|
}
|
|
public bool Step_NewManualPagebreak
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["Step", "NewManualPagebreak"];
|
|
|
|
if (s == string.Empty) return false;
|
|
if (s == "True") return true;
|
|
return false;
|
|
}
|
|
set
|
|
{
|
|
string s = _Xp["Step", "NewManualPagebreak"];
|
|
if (value.ToString() == s) return;
|
|
_Xp["Step", "NewManualPagebreak"] = value.ToString();
|
|
OnPropertyChanged("Step_NewManualPagebreak");
|
|
}
|
|
}
|
|
//[Category("Step Attributes")]
|
|
//[DisplayName("Step Change Bar Override")]
|
|
//[RefreshProperties(RefreshProperties.All)]
|
|
//[Description("Step Change Bar Override")]
|
|
public string Step_CBOverride
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["Step", "CBOverride"];
|
|
|
|
if (s == string.Empty) return null;
|
|
return s;
|
|
}
|
|
set
|
|
{
|
|
string s = _Xp["Step", "CBOverride"];
|
|
|
|
if (value != null && value.ToString() == s) return;
|
|
if (value == null && s != null) _Xp["Step", "CBOverride"] = null;
|
|
else _Xp["Step", "CBOverride"] = value.ToString();
|
|
OnPropertyChanged("Step_CBOverride");
|
|
}
|
|
}
|
|
public string Step_MultipleChangeID
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["Step", "MultipleChangeID"];
|
|
|
|
if (s == string.Empty) return null;
|
|
return s;
|
|
}
|
|
set
|
|
{
|
|
string s = _Xp["Step", "MultipleChangeID"];
|
|
|
|
if (value != null && value.ToString() == s) return;
|
|
if (value == null && s != null) _Xp["Step", "MultipleChangeID"] = null;
|
|
else _Xp["Step", "MultipleChangeID"] = value.ToString();
|
|
OnPropertyChanged("Step_MultipleChangeID");
|
|
}
|
|
}
|
|
#endregion
|
|
|
|
#region IItemConfig Members
|
|
|
|
[Category("Master/Slave Settings")]
|
|
[DisplayName("Applicability")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Scope Applicability")]
|
|
public Volian.Base.Library.BigNum MasterSlave_Applicability
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["MasterSlave", "Applicability"];
|
|
return new Volian.Base.Library.BigNum(s);
|
|
}
|
|
set
|
|
{
|
|
if (value != null)
|
|
_Xp["MasterSlave", "Applicability"] = value.FlagList;
|
|
else
|
|
_Xp["MasterSlave", "Applicability"] = null;
|
|
OnPropertyChanged("MasterSlave_Applicability");
|
|
}
|
|
}
|
|
|
|
#endregion
|
|
}
|
|
}
|