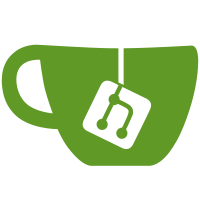
Added DocVersionInfo property to ROFSTLookup class Set DocVersionInfo to DocVersionInfo of ROFstInfo object in ROFSTLookup constructor Added Reset method to ROFSTLookup class Changed return values for ROID parameter of GetRoValue method Changes SectionConfig class to implement IItemConfig interface Added MasterSlave_Applicability property to IItemConfig Changes StepConfig class to implement IItemConfig interface Added MasterSlave_Applicability property to IItemConfig Changed GetGroup method to support multi units
797 lines
23 KiB
C#
797 lines
23 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using System.ComponentModel;
|
|
using DescriptiveEnum;
|
|
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
[Serializable]
|
|
[TypeConverter(typeof(ExpandableObjectConverter))]
|
|
public class SectionConfig : ConfigDynamicTypeDescriptor, INotifyPropertyChanged, IItemConfig
|
|
{
|
|
#region DynamicTypeDescriptor
|
|
internal override bool IsReadOnly
|
|
{
|
|
get { return false; }//_Section == null; }
|
|
}
|
|
#endregion
|
|
#region XML
|
|
private XMLProperties _Xp;
|
|
private XMLProperties Xp
|
|
{
|
|
get { return _Xp; }
|
|
}
|
|
#endregion
|
|
#region Constructors
|
|
//PROPGRID: Hide ParentLookup
|
|
[Browsable(false)]
|
|
public bool ParentLookup
|
|
{
|
|
get { return _Xp.ParentLookup; }
|
|
set { _Xp.ParentLookup = value; }
|
|
}
|
|
//PROPGRID: Had to comment out NonSerialized to hide AncestorLookup from Property Grid
|
|
//[NonSerialized]
|
|
private bool _AncestorLookup;
|
|
//PROPGRID: Hide AncestorLookup
|
|
//[Browsable(false)]
|
|
//public bool AncestorLookup
|
|
//{
|
|
// get { return _AncestorLookup; }
|
|
// set { _AncestorLookup = value; }
|
|
//}
|
|
private Section _Section;
|
|
private SectionInfo _SectionInfo;
|
|
private static int _SectionConfigUnique = 0;
|
|
private static int SectionConfigUnique
|
|
{ get { return ++_SectionConfigUnique; } }
|
|
private int _MySectionConfigUnique = SectionConfigUnique;
|
|
public int MySectionConfigUnique // Absolutely Unique ID - Info
|
|
{ get { return _MySectionConfigUnique; } }
|
|
|
|
public SectionConfig(Section section)
|
|
{
|
|
_Section = section;
|
|
string xml = section.MyContent.Config;
|
|
if (xml == string.Empty) xml = "<Config/>";
|
|
_Xp = new XMLProperties(xml);
|
|
if (section.MySectionInfo.ActiveParent != null) _Xp.LookInAncestor += new XMLPropertiesEvent(Xp_LookInAncestorFolder);
|
|
}
|
|
private string Xp_LookInAncestorFolder(object sender, XMLPropertiesArgs args)
|
|
{
|
|
if (args.AncestorLookup || ParentLookup)
|
|
{
|
|
string retval;
|
|
// There may be levels of sections, i.e. sections within a section.
|
|
SectionInfo sect = _Section != null ? _Section.MySectionInfo : _SectionInfo;
|
|
while (sect.ActiveParent.IsSection)
|
|
{
|
|
retval = sect.SectionConfig.GetValue(args.Group, args.Item);
|
|
if (retval != string.Empty) return retval;
|
|
sect = (sect.ActiveParent as SectionInfo) ?? SectionInfo.Get((sect.ActiveParent as ItemInfo).ItemID);
|
|
}
|
|
// There may be levels of procedures, i.e. procedures within a procedure.
|
|
ProcedureInfo proc = sect.MyProcedure;
|
|
retval = proc.ProcedureConfig.GetValue(args.Group, args.Item);
|
|
if (retval != string.Empty) return retval;
|
|
while (proc.ActiveParent.IsProcedure)
|
|
{
|
|
retval = proc.ProcedureConfig.GetValue(args.Group, args.Item);
|
|
if (retval != string.Empty) return retval;
|
|
proc = (ProcedureInfo)proc.ActiveParent;
|
|
}
|
|
DocVersionInfo docVersion = proc.ActiveParent as DocVersionInfo;
|
|
if (docVersion == null) return string.Empty;
|
|
retval = docVersion.DocVersionConfig.GetValue(args.Group, args.Item);
|
|
if (retval != string.Empty) return retval;
|
|
for (FolderInfo folder = docVersion.MyFolder; folder != null; folder = folder.MyParent)
|
|
{
|
|
retval = folder.FolderConfig.GetValue(args.Group, args.Item);
|
|
if (retval != string.Empty) return retval;
|
|
}
|
|
}
|
|
return string.Empty;
|
|
}
|
|
public SectionConfig(SectionInfo sectionInfo)
|
|
{
|
|
_SectionInfo = sectionInfo;
|
|
string xml = sectionInfo.MyContent.Config;
|
|
if (xml == string.Empty) xml = "<Config/>";
|
|
_Xp = new XMLProperties(xml);
|
|
if (_SectionInfo.ActiveParent != null) _Xp.LookInAncestor += new XMLPropertiesEvent(Xp_LookInAncestorFolder);
|
|
}
|
|
public SectionConfig(string xml)
|
|
{
|
|
if (xml == string.Empty) xml = "<Config/>";
|
|
_Xp = new XMLProperties(xml);
|
|
}
|
|
public SectionConfig()
|
|
{
|
|
_Xp = new XMLProperties();
|
|
}
|
|
internal string GetValue(string group, string item)
|
|
{
|
|
return _Xp[group, item];
|
|
}
|
|
#endregion
|
|
#region Local Properties
|
|
//[Category("Identification")]
|
|
[Category("General")]
|
|
[DisplayName("Number")]
|
|
[Description("Number")]
|
|
public string Number
|
|
{
|
|
get { return (_Section != null ? _Section.MyContent.Number : _SectionInfo.MyContent.Number); }
|
|
set { if (_Section != null) _Section.MyContent.Number = value; }
|
|
}
|
|
//[Category("Identification")]
|
|
[Category("General")]
|
|
[DisplayName("Title")]
|
|
[Description("Title")]
|
|
public string Title
|
|
{
|
|
get { return (_Section != null ? _Section.MyContent.Text : _SectionInfo.MyContent.Text); }
|
|
set { if (_Section != null) _Section.MyContent.Text = value; }
|
|
}
|
|
[Category("Identification")]
|
|
//PROPGRID: Hide Old Sequence
|
|
[Browsable(false)]
|
|
[DisplayName("Old Sequence")]
|
|
[Description("Old Sequence")]
|
|
public string OldSequence
|
|
{
|
|
get { return (_Section != null ? _Section.MyContent.MyZContent.OldStepSequence : (_SectionInfo.MyContent.MyZContent == null ? null : _SectionInfo.MyContent.MyZContent.OldStepSequence)); }
|
|
set { if (_Section != null) _Section.MyContent.MyZContent.OldStepSequence = value; }
|
|
}
|
|
[Category("Identification")]
|
|
//PROPGRID: Hide Dirty
|
|
[Browsable(false)]
|
|
[DisplayName("Dirty")]
|
|
[Description("Dirty")]
|
|
public bool Dirty
|
|
{
|
|
get { return (_Section != null ? _Section.IsDirty : false); }
|
|
}
|
|
[Category("Format")]
|
|
[DisplayName("Format")]
|
|
[Description("Format")]
|
|
[TypeConverter(typeof(FormatList))]
|
|
public string FormatSelection
|
|
{
|
|
get
|
|
{
|
|
if (_Section != null && _Section.MyContent.MyFormat != null) return _Section.MyContent.MyFormat.FullName;
|
|
if (_SectionInfo != null && _SectionInfo.MyContent.MyFormat != null) return _SectionInfo.MyContent.MyFormat.FullName;
|
|
return null;
|
|
}
|
|
set
|
|
{
|
|
if (_Section != null)
|
|
{
|
|
_Section.MyContent.MyFormat = FormatList.ToFormat(value); // Can only be set if _DocVersion is set
|
|
//_Section.ActiveFormat = null;
|
|
DocStyleListConverter.MySection = _Section;
|
|
}
|
|
}
|
|
}
|
|
[Browsable(false)]
|
|
public FormatInfo MyFormat
|
|
{
|
|
get
|
|
{
|
|
if (_Section != null)
|
|
{
|
|
SectionInfo sectionInfo = SectionInfo.Get(_Section.ItemID);
|
|
return sectionInfo.LocalFormat;
|
|
}
|
|
if (_SectionInfo != null)return _SectionInfo.LocalFormat;
|
|
return null;
|
|
}
|
|
set
|
|
{
|
|
if (_Section != null)
|
|
_Section.MyContent.MyFormat = value == null ? null : value.GetJustFormat();
|
|
}
|
|
}
|
|
[Category("Format")]
|
|
[DisplayName("Default Format")]
|
|
[Description("Default Format")]
|
|
[TypeConverter(typeof(FormatList))]
|
|
public string DefaultFormatSelection
|
|
{
|
|
get
|
|
{
|
|
if (_Section != null && _Section.MySectionInfo.ActiveParent != null && _Section.MySectionInfo.ActiveParent.ActiveFormat != null) return _Section.MySectionInfo.ActiveParent.ActiveFormat.FullName;
|
|
if (_SectionInfo != null && _SectionInfo.MyParent != null && _SectionInfo.MyParent.ActiveFormat != null) return _SectionInfo.MyParent.ActiveFormat.FullName;
|
|
return null;
|
|
}
|
|
}
|
|
[Browsable(false)]
|
|
public FormatInfo MyDefaultFormat
|
|
{
|
|
get
|
|
{
|
|
if (_Section != null) return _Section.MySectionInfo.ActiveParent.ActiveFormat;
|
|
return _SectionInfo.ActiveParent.ActiveFormat;
|
|
}
|
|
}
|
|
//[Browsable(false)]
|
|
[Category("Format")]
|
|
[DisplayName("Section Type")]
|
|
[Description("Section Type")]
|
|
[TypeConverter(typeof(DocStyleListConverter))]
|
|
public string MySectionType
|
|
{
|
|
get
|
|
{
|
|
if (_Section != null)
|
|
{
|
|
return DocStyleListConverter.ToString(_Section.MyContent.Type);
|
|
}
|
|
return string.Empty;
|
|
}
|
|
set
|
|
{
|
|
if (_Section != null)
|
|
_Section.MyContent.Type = DocStyleListConverter.ToSectionType(value);
|
|
}
|
|
}
|
|
[Browsable(false)]
|
|
public int? SectionType
|
|
{
|
|
get
|
|
{
|
|
if (_Section != null)
|
|
{
|
|
return _Section.MyContent.Type-10000;
|
|
}
|
|
return null;
|
|
}
|
|
set
|
|
{
|
|
if (_Section != null)
|
|
_Section.MyContent.Type = value+10000;
|
|
}
|
|
}
|
|
public Section MySection
|
|
{ get { return _Section; } }
|
|
[Category("Format")]
|
|
[DisplayName("Keep Word Document Margins")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Section WordMargin")]
|
|
public string Section_WordMargin
|
|
{
|
|
get
|
|
{
|
|
string tmp = _Xp["Section", "WordMargin"];
|
|
return tmp == null || tmp == "" ? "N" : tmp;
|
|
}
|
|
set
|
|
{
|
|
_Xp["Section", "WordMargin"] = value;
|
|
OnPropertyChanged("Section_WordMargin");
|
|
}
|
|
}
|
|
#endregion
|
|
#region ToString
|
|
public override string ToString()
|
|
{
|
|
string s = _Xp.ToString();
|
|
if (s == "<Config/>" || s == "<Config></Config>") return string.Empty;
|
|
return s;
|
|
}
|
|
#endregion
|
|
#region SectionCategory // from sequence number in 16-bit database.
|
|
[TypeConverter(typeof(EnumDescConverter))]
|
|
public enum SectionPagination : int
|
|
{
|
|
//Default = 0, Continuous, Separate
|
|
Continuous = 1, Separate = 2
|
|
}
|
|
[Category("Format")]
|
|
[DisplayName("Section Pagination")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Section Pagination")]
|
|
public SectionPagination Section_Pagination
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["Section", "Pagination"];
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("Section", "Pagination"); // get the parent value
|
|
//If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
{
|
|
if (MyFormat != null)
|
|
{
|
|
bool rval = MyFormat.MyStepSectionLayoutData.BreakOnSections;
|
|
return (rval) ? SectionPagination.Separate : SectionPagination.Continuous;// default to volian default
|
|
}
|
|
else
|
|
{
|
|
bool rval = MyDefaultFormat.MyStepSectionLayoutData.BreakOnSections;
|
|
return (rval) ? SectionPagination.Separate : SectionPagination.Continuous;// default to volian default
|
|
}
|
|
}
|
|
return (SectionPagination)int.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("Section", "Pagination"); // get the parent value
|
|
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
{
|
|
bool rval = _Section.MySectionInfo.ActiveFormat.MyStepSectionLayoutData.BreakOnSections;
|
|
parval = (rval) ? ((int)(SectionPagination.Separate)).ToString() : ((int)(SectionPagination.Continuous)).ToString();
|
|
//parval = ((int)(SectionPagination.Default)).ToString();
|
|
}
|
|
|
|
if (parval.Equals(((int)value).ToString()))
|
|
_Xp["Section", "Pagination"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["Section", "Pagination"] = ((int)value).ToString(); // save selected value
|
|
|
|
OnPropertyChanged("Section_Pagination");
|
|
}
|
|
}
|
|
[Category("View Settings")]
|
|
//PROPGRID: Hide Include in Background/Deviation
|
|
[DisplayName("Include in Background/Deviation")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Section LinkEnhanced")]
|
|
public string Section_LnkEnh
|
|
{
|
|
get
|
|
{
|
|
return _Xp["Section", "LnkEnh"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["Section", "LnkEnh"] = value;
|
|
OnPropertyChanged("Section_LnkEnh");
|
|
}
|
|
}
|
|
[Category("General")]
|
|
//PROPGRID: Hide Include On Table Of Contents
|
|
[DisplayName("Include On Table Of Contents")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Section TOC")]
|
|
public string Section_TOC
|
|
{
|
|
get
|
|
{
|
|
string tmp = _Xp["Section", "TOC"];
|
|
return tmp == null || tmp == "" ? "N" : tmp;
|
|
}
|
|
set
|
|
{
|
|
_Xp["Section", "TOC"] = value;
|
|
OnPropertyChanged("Section_TOC");
|
|
}
|
|
}
|
|
[Category("Section")]
|
|
//PROPGRID: Hide AutoGen
|
|
[DisplayName("Section AutoGen")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Section AutoGen")]
|
|
public string Section_AutoGen
|
|
{
|
|
get
|
|
{
|
|
return _Xp["Section", "AutoGen"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["Section", "AutoGen"] = value;
|
|
OnPropertyChanged("Section_AutoGen");
|
|
}
|
|
}
|
|
[Category("Section")]
|
|
//PROPGRID: Hide Subsection PH
|
|
[DisplayName("Section PrintHdr")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Section PrintHdr")]
|
|
public string Section_PrintHdr
|
|
{
|
|
get
|
|
{
|
|
// if null - return a "Y"
|
|
string tmp = _Xp["Section", "PrintHdr"];
|
|
return tmp == null || tmp == "" ? "Y" : tmp;
|
|
}
|
|
set
|
|
{
|
|
_Xp["Section", "PrintHdr"] = value;
|
|
OnPropertyChanged("Section_PrintHdr");
|
|
}
|
|
}
|
|
[Browsable(false)]
|
|
[DisplayName("Section OriginalSteps")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Section OriginalSteps")]
|
|
public string Section_OriginalSteps
|
|
{
|
|
get
|
|
{
|
|
return _Xp["Section", "OriginalSteps"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["Section", "OriginalSteps"] = value;
|
|
OnPropertyChanged("Section_OriginalSteps");
|
|
}
|
|
}
|
|
[Category("Section")]
|
|
//PROPGRID: Hide Section NumPages
|
|
[Browsable(false)]
|
|
[DisplayName("Section NumPages")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Section NumPages")]
|
|
public string Section_NumPages
|
|
{
|
|
get
|
|
{
|
|
return _Xp["Section", "NumPages"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["Section", "NumPages"] = value;
|
|
OnPropertyChanged("Section_NumPages");
|
|
}
|
|
}
|
|
[TypeConverter(typeof(EnumDescConverter))]
|
|
public enum SectionColumnMode : int
|
|
{
|
|
//[Description("Format Default")]
|
|
//Default = 0,
|
|
[Description("Single Column")]
|
|
One = 1,
|
|
[Description("Dual Column")]
|
|
Two = 2,
|
|
[Description("Triple Column")]
|
|
Three = 3,
|
|
[Description("Quad Column")]
|
|
Four = 4
|
|
}
|
|
[Category("Format")]
|
|
[DisplayName("Columns")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Section ColumnMode")]
|
|
public SectionColumnMode Section_ColumnMode
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["Section", "ColumnMode"];
|
|
|
|
//If there is no value to get, then get the parent value (a.k.a. default value).
|
|
if (s == string.Empty)
|
|
s = _Xp.ParentValue("Section", "ColumnMode"); // get the parent value
|
|
// If there is no parent value, then use the volian default
|
|
if (s == string.Empty)
|
|
{
|
|
int rval = 0;
|
|
if (MyFormat != null)
|
|
{
|
|
rval = (int)MyFormat.MyStepSectionLayoutData.PMode;
|
|
//int rval = (int)MyFormat.MyStepSectionLayoutData.PMode;
|
|
//return (SectionColumnMode)rval;//SectionColumnMode.Two; //SectionColumnMode.Default;// default to volian default
|
|
}
|
|
else
|
|
{
|
|
//int rval = (int)MyDefaultFormat.MyStepSectionLayoutData.PMode;
|
|
rval = (int)MyDefaultFormat.MyStepSectionLayoutData.PMode;
|
|
//return (SectionColumnMode)rval;
|
|
}
|
|
// if no pmode is defined, i.e. rval = 0, then go up to the procedure level & get
|
|
// it's format column.
|
|
if (rval == 0)
|
|
{
|
|
SectionInfo si = _SectionInfo != null? _SectionInfo: SectionInfo.Get(_Section.ItemID);
|
|
if (si != null)
|
|
{
|
|
switch (si.MyProcedure.ProcedureConfig.Format_Columns)
|
|
{
|
|
case FormatColumns.OneColumn:
|
|
return SectionColumnMode.One;
|
|
break;
|
|
case FormatColumns.TwoColumn:
|
|
return SectionColumnMode.Two;
|
|
break;
|
|
default:
|
|
return SectionColumnMode.One;
|
|
break;
|
|
}
|
|
}
|
|
else
|
|
return SectionColumnMode.One; // 16bit's default if nothing defined
|
|
}
|
|
return (SectionColumnMode)rval;
|
|
}
|
|
else
|
|
return (SectionColumnMode)int.Parse(s);
|
|
|
|
//if (s == string.Empty)
|
|
// return SectionColumnMode.Default;
|
|
|
|
//return (SectionColumnMode)int.Parse(s);
|
|
}
|
|
set
|
|
{
|
|
// if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// reset the data to use the parent value.
|
|
|
|
string parval = _Xp.ParentValue("Section", "ColumnMode"); // get the parent value
|
|
int rval = 0;
|
|
if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
{
|
|
//int rval = 0;
|
|
// Use Inherited Format (ActiveFormat for Section) if MyFormat is Null
|
|
if (MyFormat == null)
|
|
{
|
|
FormatInfo myFormat = _Section == null ? _SectionInfo.ActiveFormat : SectionInfo.Get(_Section.ItemID).ActiveFormat;
|
|
rval = (int)myFormat.MyStepSectionLayoutData.PMode;
|
|
}
|
|
else
|
|
rval =(int)MyFormat.MyStepSectionLayoutData.PMode;
|
|
//parval = ((rval > 0)?((SectionColumnMode)rval).ToString() : "2"); // if PMode is zero default to 2 column mode
|
|
}
|
|
|
|
// if still no value found in inheritance, check if the procedure has a default. if so and value matches, clear out any config item.
|
|
if (rval == 0)
|
|
{
|
|
SectionInfo si = _SectionInfo != null ? _SectionInfo : SectionInfo.Get(_Section.ItemID);
|
|
if (si != null)
|
|
{
|
|
switch (si.MyProcedure.ProcedureConfig.Format_Columns)
|
|
{
|
|
case FormatColumns.OneColumn:
|
|
rval = (int)SectionColumnMode.One;
|
|
break;
|
|
case FormatColumns.TwoColumn:
|
|
rval = (int)SectionColumnMode.Two;
|
|
break;
|
|
default:
|
|
rval = (int)SectionColumnMode.One;
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
parval = ((rval > 0) ? ((SectionColumnMode)rval).ToString() : "2"); // if PMode is zero default to 2 column mode
|
|
if (parval.Equals(((int)value).ToString()))
|
|
_Xp["Section", "ColumnMode"] = string.Empty; // reset to parent value
|
|
else
|
|
_Xp["Section", "ColumnMode"] = ((int)value).ToString(); // save selected value
|
|
|
|
OnPropertyChanged("Section_ColumnMode");
|
|
}
|
|
}
|
|
//char * far printtypes[] = {
|
|
// "Compressed, 8 lines per inch",
|
|
// "Elite, 6 lines per inch",
|
|
// "Pica, 6 lines per inch",
|
|
// "Default font, 4 Lines Per Inch",
|
|
// "Default font, 6 Lines Per Inch",
|
|
// "Compressed 6 LPI",
|
|
// "Default font, 7 Lines Per Inch",
|
|
// "Special Landscape, Elite, 6 lines per inch"
|
|
//};
|
|
//[TypeConverter(typeof(EnumDescConverter))]
|
|
//public enum AttPrintSize : int
|
|
//{
|
|
// [Description("Compressed, 8 lines per inch")]
|
|
// Cmp8lpi = 0,
|
|
// [Description("Elite, 6 lines per inch")]
|
|
// Elite6lpi = 1,
|
|
// [Description("Pica, 6 lines per inch")]
|
|
// Pica6lpi = 2,
|
|
// [Description("Default font, 4 Lines Per Inch")]
|
|
// Def4lpi = 3,
|
|
// [Description("Default font, 6 Lines Per Inch")]
|
|
// Def6lpi = 4,
|
|
// [Description("Compressed 6 LPI")]
|
|
// Cmp6lpi = 5,
|
|
// [Description("Default font, 7 Lines Per Inch")]
|
|
// Def7lpi = 6,
|
|
// [Description("Landscape, Elite, 6 lines per inch")]
|
|
// landElite6lpi = 7
|
|
//}
|
|
|
|
//[Category("Format")]
|
|
//[DisplayName("Attachment PrintSize")]
|
|
//[RefreshProperties(RefreshProperties.All)]
|
|
//[Description("Attachment Print Size")]
|
|
//public AttPrintSize Section_AttachmentPrintSize
|
|
//{
|
|
// get
|
|
// {
|
|
// string lpiSettings = "*pP46f7L";
|
|
// string s = _Xp["Section", "OldType"];
|
|
// int idx = -1;
|
|
|
|
// //If there is no value to get, then get the parent value (a.k.a. default value).
|
|
// if (s == string.Empty)
|
|
// s = _Xp.ParentValue("Section", "OldType"); // get the parent value
|
|
// // If there is no parent value, then use the volian default
|
|
// if (s == string.Empty)
|
|
// return AttPrintSize.Def6lpi;// default to volian default
|
|
|
|
// idx = lpiSettings.IndexOf(s[1]);
|
|
// if (idx == -1) idx = 4;
|
|
// return (AttPrintSize)idx;
|
|
// //return (AttPrintSize)int.Parse(s);
|
|
// }
|
|
// set
|
|
// {
|
|
// // if value being saved is same as the parent value, then clear the value (save blank). This will
|
|
// // reset the data to use the parent value.
|
|
|
|
// string lpiSettings = "*pP46f7L";
|
|
// string parval = _Xp.ParentValue("Section", "OldType"); // get the parent value
|
|
// StringBuilder sb = new StringBuilder();
|
|
// string curval = parval[1].ToString();
|
|
|
|
// sb.Append(parval[0]); // save first part of OldType
|
|
|
|
// if (parval.Equals(string.Empty)) // if the parent value is empty, then use the volian default
|
|
// {
|
|
// //parval = ((int)(AttPrintSize.Def6lpi)).ToString();
|
|
// //sb.Append(((int)(AttPrintSize.Def6lpi)).ToString());
|
|
// sb.Append(lpiSettings[(int)(AttPrintSize.Def6lpi)]);
|
|
// }
|
|
|
|
// if (curval.Equals(((int)value).ToString()))
|
|
// _Xp["Section", "OldType"] = string.Empty; // reset to parent value
|
|
// else
|
|
// {
|
|
// //sb.Append(((int)value).ToString());
|
|
// sb.Append(lpiSettings[(int)value]);
|
|
// _Xp["Section", "OldType"] = sb.ToString(); // save selected value
|
|
// }
|
|
|
|
// OnPropertyChanged("Section_AttachmentPrintSize");
|
|
// }
|
|
//}
|
|
/*
|
|
int chkOffType = (rid[0] & 0x007F) - '0';
|
|
if (chkOffType > 0)
|
|
ci.AddItem("Section", "CheckoffSelection", chkOffType.ToString());
|
|
|
|
if (stype.Length > 1)
|
|
{
|
|
int chkOffHeading = (stype[1] & 0x007F) - '0';
|
|
if (chkOffHeading > 0)
|
|
ci.AddItem("Section", "CheckoffHeading", chkOffHeading.ToString());
|
|
*/
|
|
[Category("Format")]
|
|
[DisplayName("Checkoff List Selection")]
|
|
[Description("Checkoff List Selection")]
|
|
public int Section_CheckoffListSelection
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["Section", "CheckoffSelection"];
|
|
if (s != null && !s.Equals(string.Empty))
|
|
return Convert.ToInt32(s);
|
|
return 0;
|
|
}
|
|
set
|
|
{
|
|
string s = value.ToString();
|
|
_Xp["Section", "CheckoffSelection"] = s;
|
|
}
|
|
}
|
|
[Category("Format")]
|
|
[DisplayName("Checkoff Header Selection")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Checkoff Header Selection")]
|
|
public int Section_CheckoffHeaderSelection
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["Section", "CheckoffHeading"];
|
|
if (s != null && !s.Equals(string.Empty))
|
|
return Convert.ToInt32(s);
|
|
return 0;
|
|
}
|
|
set
|
|
{
|
|
string s = value.ToString();
|
|
_Xp["Section", "CheckoffHeading"] = s;
|
|
}
|
|
}
|
|
#endregion
|
|
#region SubSectionCategory // from sequence number in 16-bit database.
|
|
[Category("SubSection")]
|
|
//PROPGRID: Hide SubSection Edit
|
|
[Browsable(false)]
|
|
[DisplayName("SubSection Edit")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("SubSection Edit")]
|
|
public string SubSection_Edit
|
|
{
|
|
get
|
|
{
|
|
// if null - return a "Y"
|
|
string tmp = _Xp["SubSection", "Edit"];
|
|
return tmp == null || tmp == "" ? "N" : tmp;
|
|
}
|
|
set
|
|
{
|
|
_Xp["SubSection", "Edit"] = value;
|
|
OnPropertyChanged("SubSection_Edit");
|
|
}
|
|
}
|
|
|
|
[Category("SubSection")]
|
|
//PROPGRID: Hide Subsection AutoIndent
|
|
[Browsable(false)]
|
|
[DisplayName("SubSection AutoIndent")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("SubSection AutoIndent")]
|
|
public string SubSection_AutoIndent
|
|
{
|
|
get
|
|
{
|
|
// if null - return a "Y"
|
|
string tmp = _Xp["SubSection", "AutoIndent"];
|
|
return tmp==null||tmp==""?"Y":tmp;
|
|
}
|
|
set
|
|
{
|
|
_Xp["SubSection", "AutoIndent"] = value;
|
|
OnPropertyChanged("SubSection_AutoIndent");
|
|
}
|
|
}
|
|
#endregion
|
|
#region LibDocCategory // from library document file during migration
|
|
[Category("LibraryDocument")]
|
|
//PROPGRID: Hide Libary Document Comment
|
|
[Browsable(false)]
|
|
[DisplayName("LibraryDocument Comment")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("LibraryDocument Comment")]
|
|
public string LibDoc_Comment
|
|
{
|
|
get
|
|
{
|
|
return _Xp["LibraryDocument", "Comment"];
|
|
}
|
|
set
|
|
{
|
|
_Xp["LibraryDocument", "Comment"] = value;
|
|
OnPropertyChanged("LibDoc_Comment");
|
|
}
|
|
}
|
|
|
|
#endregion
|
|
|
|
#region IItemConfig Members
|
|
|
|
[Category("Master/Slave Settings")]
|
|
[DisplayName("Applicability")]
|
|
[RefreshProperties(RefreshProperties.All)]
|
|
[Description("Scope Applicability")]
|
|
public Volian.Base.Library.BigNum MasterSlave_Applicability
|
|
{
|
|
get
|
|
{
|
|
string s = _Xp["MasterSlave", "Applicability"];
|
|
return new Volian.Base.Library.BigNum(s);
|
|
}
|
|
set
|
|
{
|
|
_Xp["MasterSlave", "Applicability"] = value.FlagList;
|
|
OnPropertyChanged("MasterSlave_Applicability");
|
|
}
|
|
}
|
|
|
|
#endregion
|
|
}
|
|
}
|