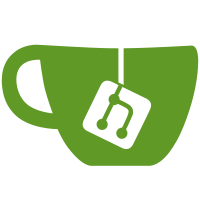
Removed GetChildren from editable object Removed HasChildren from editable object Removed ActiveParent from editable object Removed ActiveFormat from editable object Removed LocalFormat from editable object
338 lines
9.7 KiB
C#
338 lines
9.7 KiB
C#
// ========================================================================
|
|
// Copyright 2006 - Volian Enterprises, Inc. All rights reserved.
|
|
// Volian Enterprises - Proprietary Information - DO NOT COPY OR DISTRIBUTE
|
|
// ------------------------------------------------------------------------
|
|
// $Workfile: $ $Revision: $
|
|
// $Author: $ $Date: $
|
|
//
|
|
// $History: $
|
|
// ========================================================================
|
|
|
|
using System;
|
|
using System.Data;
|
|
using System.Data.SqlClient;
|
|
using Csla;
|
|
using Csla.Data;
|
|
using System.Configuration;
|
|
using System.IO;
|
|
using System.Drawing;
|
|
using System.Collections.Generic;
|
|
using System.ComponentModel;
|
|
using System.Text.RegularExpressions;
|
|
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
public partial class Folder : IVEDrillDown
|
|
{
|
|
#region Folder Config
|
|
[NonSerialized]
|
|
private FolderConfig _FolderConfig;
|
|
public FolderConfig FolderConfig
|
|
{
|
|
get
|
|
{
|
|
if (_FolderConfig == null)
|
|
{
|
|
_FolderConfig = new FolderConfig(this);
|
|
_FolderConfig.PropertyChanged += new System.ComponentModel.PropertyChangedEventHandler(_FolderConfig_PropertyChanged);
|
|
}
|
|
return _FolderConfig;
|
|
}
|
|
}
|
|
public void FolderConfigRefresh()
|
|
{
|
|
_FolderConfig = null;
|
|
}
|
|
private void _FolderConfig_PropertyChanged(object sender, System.ComponentModel.PropertyChangedEventArgs e)
|
|
{
|
|
Config = _FolderConfig.ToString();
|
|
}
|
|
#endregion
|
|
public override string ToString()
|
|
{
|
|
return _Title;
|
|
}
|
|
#region IVEReadOnlyItem
|
|
//public System.Collections.IList GetChildren()
|
|
//{
|
|
// if (FolderDocVersionCount != 0) return FolderDocVersions;
|
|
// if (ChildFolderCount != 0) return ChildFolders;
|
|
// return null;
|
|
//}
|
|
//public bool HasChildren
|
|
//{
|
|
// get { return _FolderDocVersionCount > 0 || _ChildFolderCount > 0; }
|
|
//}
|
|
//public IVEDrillDown ActiveParent
|
|
//{
|
|
// get
|
|
// {
|
|
// return MyParent;
|
|
// }
|
|
//}
|
|
//private Format _ActiveFormat = null;
|
|
//public Format ActiveFormat
|
|
//{
|
|
// get
|
|
// {
|
|
// if (_ActiveFormat == null)
|
|
// _ActiveFormat = (LocalFormat != null ? LocalFormat : ActiveParent != null ? ActiveParent.ActiveFormat : null);
|
|
// return _ActiveFormat;
|
|
// }
|
|
// set
|
|
// {
|
|
// _ActiveFormat = null; // Reset
|
|
// }
|
|
//}
|
|
//public Format LocalFormat
|
|
//{
|
|
// get { return MyFormat; }
|
|
//}
|
|
public ConfigDynamicTypeDescriptor MyConfig
|
|
{
|
|
get { return FolderConfig; }
|
|
}
|
|
#endregion
|
|
#region MakeFolders for ManualOrder
|
|
public static Folder MakeFolder(Folder myParent, Connection myConnection, string name, string title, string shortName, Format myFormat, string config, DateTime dts, string usrID)
|
|
{
|
|
return MakeFolder(myParent, myConnection, name, title, shortName, myFormat, null, config, dts, usrID);
|
|
}
|
|
public static Folder MakeFolder(Folder myParent, Connection myConnection, string name, string title, string shortName, Format myFormat, string config)
|
|
{
|
|
return MakeFolder(myParent, myConnection, name, title, shortName, myFormat, null, config);
|
|
}
|
|
#endregion
|
|
public FolderInfo MyFolderInfo
|
|
{ get { return FolderInfo.Get(FolderID); } }
|
|
}
|
|
public partial class FolderInfo:IVEDrillDownReadOnly
|
|
{
|
|
#region Folder Config (Read-Only)
|
|
[NonSerialized]
|
|
private FolderConfig _FolderConfig;
|
|
public FolderConfig FolderConfig
|
|
{ get {
|
|
return (_FolderConfig != null ? _FolderConfig : _FolderConfig = new FolderConfig(this));
|
|
} }
|
|
private void FolderConfigRefresh()
|
|
{
|
|
_FolderConfig = null;
|
|
}
|
|
#endregion
|
|
#region SortingChildren
|
|
public Csla.SortedBindingList<FolderInfo> _SortedChildFolders;
|
|
public Csla.SortedBindingList<FolderInfo> SortedChildFolders
|
|
{
|
|
get
|
|
{
|
|
if (ChildFolders != null)
|
|
{
|
|
if (_SortedChildFolders == null)
|
|
{
|
|
_SortedChildFolders = new SortedBindingList<FolderInfo>(ChildFolders);
|
|
//_SortedChildFolders.ApplySort("FolderID", ListSortDirection.Ascending);
|
|
//_SortedChildFolders.ApplySort("Name", ListSortDirection.Ascending);
|
|
}
|
|
else if (_SortedChildFolders.Count != ChildFolders.Count)
|
|
{
|
|
_SortedChildFolders = new SortedBindingList<FolderInfo>(ChildFolders);
|
|
}
|
|
_SortedChildFolders.ApplySort("ManualOrder", ListSortDirection.Ascending);
|
|
}
|
|
return _SortedChildFolders;
|
|
}
|
|
}
|
|
public double? NewManualOrder(int index)
|
|
{
|
|
double? retval = 1;
|
|
if (SortedChildFolders == null || SortedChildFolders.Count == 0)
|
|
retval = 1; // First value
|
|
else if (index == 0)
|
|
{
|
|
if (retval >= SortedChildFolders[index].ManualOrder) // If one is too big, then divide first value in half
|
|
retval = SortedChildFolders[index].ManualOrder / 2;
|
|
}
|
|
else if (SortedChildFolders.Count > index)
|
|
{
|
|
retval += SortedChildFolders[index - 1].ManualOrder; // Just go to the next whole number
|
|
if (retval >= SortedChildFolders[index].ManualOrder)
|
|
retval = (SortedChildFolders[index - 1].ManualOrder + SortedChildFolders[index].ManualOrder) / 2;
|
|
}
|
|
else
|
|
retval += SortedChildFolders[index - 1].ManualOrder;
|
|
return retval;
|
|
}
|
|
/// <summary>
|
|
/// UniqueChildName will check the existing children to assure that the name is not a duplicate name.
|
|
/// </summary>
|
|
/// <param name="folderName"></param>
|
|
/// <returns></returns>
|
|
public string UniqueChildName(string folderName)
|
|
{
|
|
string retval = folderName;
|
|
int iSuffix = -1;
|
|
RefreshChildFolders();
|
|
foreach (FolderInfo fi in ChildFolders)
|
|
{
|
|
if (fi.Name.StartsWith(folderName))
|
|
{
|
|
if (fi.Name == folderName)
|
|
iSuffix = 0;
|
|
else if (Regex.IsMatch(fi.Name, folderName + "[_][0-9]+"))
|
|
{
|
|
int ii = int.Parse(fi.Name.Substring(1 + folderName.Length));
|
|
if (ii > iSuffix) iSuffix = ii;
|
|
}
|
|
}
|
|
}
|
|
if (iSuffix >= 0)
|
|
retval = string.Format("{0}_{1}", folderName, iSuffix + 1);
|
|
// Console.WriteLine("FolderName = '{0}'", retval);
|
|
return retval;
|
|
}
|
|
#endregion
|
|
#region IVEReadOnlyItem
|
|
public System.Collections.IList GetChildren()
|
|
{
|
|
if(FolderDocVersionCount != 0)return FolderDocVersions;
|
|
//if (ChildFolderCount != 0) return ChildFolders;
|
|
if (ChildFolderCount != 0) return SortedChildFolders;
|
|
return null;
|
|
}
|
|
//public bool ChildrenAreLoaded
|
|
//{
|
|
// get { return _FolderDocVersions != null || _ChildFolders != null; }
|
|
//}
|
|
public bool HasChildren
|
|
{
|
|
get { return _FolderDocVersionCount > 0 || _ChildFolderCount > 0; }
|
|
}
|
|
public IVEDrillDownReadOnly ActiveParent
|
|
{
|
|
get
|
|
{
|
|
return MyParent;
|
|
}
|
|
}
|
|
public FormatInfo ActiveFormat
|
|
{
|
|
get { return LocalFormat != null ? LocalFormat : ( ActiveParent != null ? ActiveParent.ActiveFormat : null); }
|
|
}
|
|
public FormatInfo LocalFormat
|
|
{
|
|
get { return MyFormat; }
|
|
}
|
|
public ConfigDynamicTypeDescriptor MyConfig
|
|
{
|
|
get { return Get().FolderConfig; }
|
|
}
|
|
/// <summary>
|
|
/// These settings are set on the user interface side.
|
|
/// This is used to control whether the Name and/or Title is displayed
|
|
/// next to the tree nodes in the user interface
|
|
/// </summary>
|
|
private bool _DisplayTreeNodeNames = true;
|
|
public bool DisplayTreeNodeNames
|
|
{
|
|
get { return _DisplayTreeNodeNames; }
|
|
set { _DisplayTreeNodeNames = value; }
|
|
}
|
|
private bool _DisplayTreeNodeTitles = false;
|
|
|
|
public bool DisplayTreeNodeTitles
|
|
{
|
|
get { return _DisplayTreeNodeTitles; }
|
|
set { _DisplayTreeNodeTitles = value; }
|
|
}
|
|
public override string ToString()
|
|
{
|
|
// assume that at least one of the two options was selected
|
|
string rtnstr = "";
|
|
if ( _DisplayTreeNodeNames) rtnstr = Name;
|
|
if (_DisplayTreeNodeTitles)
|
|
{
|
|
if (rtnstr.Length > 0) rtnstr += " - ";
|
|
rtnstr += Title;
|
|
}
|
|
return rtnstr;
|
|
//return string.Format("{0} - {1}", Name, Title);
|
|
}
|
|
//public string ToString(string str, System.IFormatProvider ifp)
|
|
//{
|
|
// return ToString();
|
|
//}
|
|
#endregion
|
|
public Color BackColor
|
|
{ get { return FolderConfig.Default_BkColor; } }
|
|
#region Extension
|
|
partial class FolderInfoExtension : extensionBase
|
|
{
|
|
public override void Refresh(FolderInfo tmp)
|
|
{
|
|
tmp.FolderConfigRefresh();
|
|
}
|
|
}
|
|
public static FolderInfo GetTop()
|
|
{
|
|
//if (!CanGetObject())
|
|
// throw new System.Security.SecurityException("User not authorized to view a Folder");
|
|
try
|
|
{
|
|
FolderInfo tmp = DataPortal.Fetch<FolderInfo>(new TopCriteria());
|
|
if (tmp.ErrorMessage == "No Record Found")
|
|
{
|
|
tmp.Dispose(); // Clean-up FolderInfo
|
|
tmp = null;
|
|
}
|
|
return tmp;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on FolderInfo.GetTop", ex);
|
|
}
|
|
}
|
|
private void DataPortal_Fetch(TopCriteria criteria)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] FolderInfo.DataPortal_Fetch", GetHashCode());
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
ApplicationContext.LocalContext["cn"] = cn;
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "getTopFolder";
|
|
using (SafeDataReader dr = new SafeDataReader(cm.ExecuteReader()))
|
|
{
|
|
if (!dr.Read())
|
|
{
|
|
_ErrorMessage = "No Record Found";
|
|
return;
|
|
}
|
|
ReadData(dr);
|
|
}
|
|
}
|
|
// removing of item only needed for local data portal
|
|
if (ApplicationContext.ExecutionLocation == ApplicationContext.ExecutionLocations.Client)
|
|
ApplicationContext.LocalContext.Remove("cn");
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("FolderInfo.DataPortal_Fetch", ex);
|
|
_ErrorMessage = ex.Message;
|
|
throw new DbCslaException("FolderInfo.DataPortal_Fetch", ex);
|
|
}
|
|
}
|
|
#endregion
|
|
[Serializable()]
|
|
protected class TopCriteria
|
|
{
|
|
public TopCriteria() { ;}
|
|
}
|
|
}
|
|
|
|
}
|