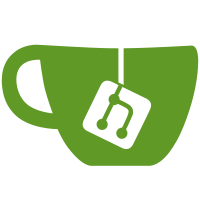
If the selected Library Document is out-of-range throw a specific error Don't fail if the user clicks on open space in the BookMark list Added property to provide a right margin (RTBMargin) between the RichTextBox and the StepItem
122 lines
3.1 KiB
C#
122 lines
3.1 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.ComponentModel;
|
|
using System.Drawing;
|
|
using System.Data;
|
|
using System.Text;
|
|
using System.Windows.Forms;
|
|
using VEPROMS.CSLA.Library;
|
|
|
|
|
|
namespace Volian.Controls.Library
|
|
{
|
|
public partial class DisplayBookMarks : UserControl
|
|
{
|
|
#region Properties
|
|
private MostRecentItemList _MyBookMarks;
|
|
private ItemInfo _CurItemInfo = null;
|
|
private bool _Initalizing = false;
|
|
private DisplayTabControl _MyDisplayTabControl;
|
|
public DisplayTabControl MyDisplayTabControl
|
|
{
|
|
get { return _MyDisplayTabControl; }
|
|
set
|
|
{
|
|
if (value == null) return;
|
|
_MyDisplayTabControl = value;
|
|
}
|
|
}
|
|
private StepRTB _MyRTB;
|
|
public StepRTB MyRTB
|
|
{
|
|
get { return _MyRTB; }
|
|
set
|
|
{
|
|
if (value == null) return;
|
|
if (_CurItemInfo != null && _CurItemInfo.ItemID == value.MyItemInfo.ItemID) return;
|
|
_MyRTB = value;
|
|
_CurItemInfo = MyRTB.MyItemInfo;
|
|
}
|
|
}
|
|
#endregion
|
|
#region Constructors
|
|
public DisplayBookMarks()
|
|
{
|
|
InitializeComponent();
|
|
SetupBookMarks();
|
|
}
|
|
private void SetupBookMarks()
|
|
{
|
|
if (_MyBookMarks == null)
|
|
{
|
|
_MyBookMarks = MostRecentItemList.GetMRILst((System.Collections.Specialized.StringCollection)(Properties.Settings.Default["BookMarks"]),0);
|
|
_MyBookMarks.AfterRemove += new ItemInfoEvent(_MyBookMarks_AfterRemove);
|
|
}
|
|
lbxBookMarks.SelectedValueChanged += new EventHandler(lbxBookMarks_SelectedValueChanged);
|
|
RefreshBookMarkData();
|
|
//btnPrevPos.Enabled = false;
|
|
//lbxBookMarks.Enabled = false;
|
|
//_PrevBookMark = null;
|
|
}
|
|
|
|
void _MyBookMarks_AfterRemove(object sender)
|
|
{
|
|
RefreshBookMarkData();
|
|
}
|
|
private void RefreshBookMarkData()
|
|
{
|
|
lbxBookMarks.DataSource = null;
|
|
lbxBookMarks.DisplayMember = "MenuTitle";
|
|
lbxBookMarks.DataSource = _MyBookMarks;
|
|
btnClrBookMrks.Enabled = (lbxBookMarks.Items.Count > 0);
|
|
btnRmvCurBookMrk.Enabled = lbxBookMarks.SelectedIndex > -1;
|
|
SaveBookMarks();
|
|
}
|
|
private void SaveBookMarks()
|
|
{
|
|
Properties.Settings.Default.BookMarks = _MyBookMarks.ToSettings();
|
|
Properties.Settings.Default.Save();
|
|
}
|
|
void lbxBookMarks_SelectedValueChanged(object sender, EventArgs e)
|
|
{
|
|
btnRmvCurBookMrk.Enabled = (lbxBookMarks.SelectedIndex >= 0);
|
|
}
|
|
public void AddBookMark(ItemInfo itm)
|
|
{
|
|
_MyBookMarks.Add(itm);
|
|
RefreshBookMarkData();
|
|
}
|
|
#endregion
|
|
#region Events
|
|
private void btnSetBookMrk_Click(object sender, EventArgs e)
|
|
{
|
|
if (_CurItemInfo == null)
|
|
{
|
|
MessageBox.Show("Cannot set a bookmark on this item.");
|
|
return;
|
|
}
|
|
_MyBookMarks.Add(_CurItemInfo);
|
|
RefreshBookMarkData();
|
|
}
|
|
|
|
private void btnRmvCurBookMrk_Click(object sender, EventArgs e)
|
|
{
|
|
_MyBookMarks.RemoveAt(lbxBookMarks.SelectedIndex);
|
|
RefreshBookMarkData();
|
|
}
|
|
|
|
private void btnClrBookMrks_Click(object sender, EventArgs e)
|
|
{
|
|
_MyBookMarks.Clear();
|
|
RefreshBookMarkData();
|
|
}
|
|
private void lbxBookMarks_Click(object sender, EventArgs e)
|
|
{
|
|
MostRecentItem itm = lbxBookMarks.SelectedValue as MostRecentItem;
|
|
if(itm != null)
|
|
MyDisplayTabControl.OpenItem(itm.MyItemInfo);
|
|
}
|
|
#endregion
|
|
}
|
|
}
|