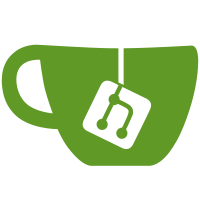
If the selected Library Document is out-of-range throw a specific error Don't fail if the user clicks on open space in the BookMark list Added property to provide a right margin (RTBMargin) between the RichTextBox and the StepItem
1293 lines
41 KiB
C#
1293 lines
41 KiB
C#
// ========================================================================
|
|
// Copyright 2007 - Volian Enterprises, Inc. All rights reserved.
|
|
// Volian Enterprises - Proprietary Information - DO NOT COPY OR DISTRIBUTE
|
|
// ------------------------------------------------------------------------
|
|
// $Workfile: $ $Revision: $
|
|
// $Author: $ $Date: $
|
|
//
|
|
// $History: $
|
|
// ========================================================================
|
|
|
|
using System;
|
|
using System.Data;
|
|
using System.Data.SqlClient;
|
|
using Csla;
|
|
using Csla.Data;
|
|
using System.Configuration;
|
|
using System.IO;
|
|
using System.ComponentModel;
|
|
using System.Collections.Generic;
|
|
using Csla.Validation;
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
/// <summary>
|
|
/// Permission Generated by MyGeneration using the CSLA Object Mapping template
|
|
/// </summary>
|
|
[Serializable()]
|
|
[TypeConverter(typeof(PermissionConverter))]
|
|
public partial class Permission : BusinessBase<Permission>, IDisposable, IVEHasBrokenRules
|
|
{
|
|
#region Log4Net
|
|
private static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
#endregion
|
|
#region Refresh
|
|
private List<Permission> _RefreshPermissions = new List<Permission>();
|
|
private void AddToRefreshList(List<Permission> refreshPermissions)
|
|
{
|
|
if (IsDirty)
|
|
refreshPermissions.Add(this);
|
|
}
|
|
private void BuildRefreshList()
|
|
{
|
|
_RefreshPermissions = new List<Permission>();
|
|
AddToRefreshList(_RefreshPermissions);
|
|
}
|
|
private void ProcessRefreshList()
|
|
{
|
|
foreach (Permission tmp in _RefreshPermissions)
|
|
{
|
|
PermissionInfo.Refresh(tmp);
|
|
if (tmp._MyRole != null) RoleInfo.Refresh(tmp._MyRole);
|
|
}
|
|
}
|
|
#endregion
|
|
#region Collection
|
|
private static List<Permission> _CacheList = new List<Permission>();
|
|
protected static void AddToCache(Permission permission)
|
|
{
|
|
if (!_CacheList.Contains(permission)) _CacheList.Add(permission); // In AddToCache
|
|
}
|
|
protected static void RemoveFromCache(Permission permission)
|
|
{
|
|
while (_CacheList.Contains(permission)) _CacheList.Remove(permission); // In RemoveFromCache
|
|
}
|
|
private static Dictionary<string, List<Permission>> _CacheByPrimaryKey = new Dictionary<string, List<Permission>>();
|
|
private static void ConvertListToDictionary()
|
|
{
|
|
while (_CacheList.Count > 0) // Move Permission(s) from temporary _CacheList to _CacheByPrimaryKey
|
|
{
|
|
Permission tmp = _CacheList[0]; // Get the first Permission
|
|
string pKey = tmp.PID.ToString();
|
|
if (!_CacheByPrimaryKey.ContainsKey(pKey))
|
|
{
|
|
_CacheByPrimaryKey[pKey] = new List<Permission>(); // Add new list for PrimaryKey
|
|
}
|
|
_CacheByPrimaryKey[pKey].Add(tmp); // Add to Primary Key list
|
|
_CacheList.RemoveAt(0); // Remove the first Permission
|
|
}
|
|
}
|
|
protected static Permission GetCachedByPrimaryKey(int pid)
|
|
{
|
|
ConvertListToDictionary();
|
|
string key = pid.ToString();
|
|
if (_CacheByPrimaryKey.ContainsKey(key)) return _CacheByPrimaryKey[key][0];
|
|
return null;
|
|
}
|
|
#endregion
|
|
#region Business Methods
|
|
private string _ErrorMessage = string.Empty;
|
|
public string ErrorMessage
|
|
{
|
|
get { return _ErrorMessage; }
|
|
}
|
|
private static int _nextPID = -1;
|
|
public static int NextPID
|
|
{
|
|
get { return _nextPID--; }
|
|
}
|
|
private int _PID;
|
|
[System.ComponentModel.DataObjectField(true, true)]
|
|
public int PID
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("PID", true);
|
|
return _PID;
|
|
}
|
|
}
|
|
private int _RID;
|
|
public int RID
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("RID", true);
|
|
if (_MyRole != null) _RID = _MyRole.RID;
|
|
return _RID;
|
|
}
|
|
}
|
|
private Role _MyRole;
|
|
public Role MyRole
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("MyRole", true);
|
|
if (_MyRole == null && _RID != 0) _MyRole = Role.Get(_RID);
|
|
return _MyRole;
|
|
}
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
set
|
|
{
|
|
CanWriteProperty("MyRole", true);
|
|
if (_MyRole != value)
|
|
{
|
|
_MyRole = value;
|
|
_RID = value.RID;// Update underlying data field
|
|
PropertyHasChanged();
|
|
}
|
|
}
|
|
}
|
|
private int _PermLevel;
|
|
/// <summary>
|
|
/// 0 - None, 1 - Security, 2 - System, 3 - RO, 4 - Procdures, 5 - Sections, 6 - Steps, 7 - Comments
|
|
/// </summary>
|
|
public int PermLevel
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("PermLevel", true);
|
|
return _PermLevel;
|
|
}
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
set
|
|
{
|
|
CanWriteProperty("PermLevel", true);
|
|
if (_PermLevel != value)
|
|
{
|
|
_PermLevel = value;
|
|
PropertyHasChanged();
|
|
}
|
|
}
|
|
}
|
|
private int _VersionType;
|
|
/// <summary>
|
|
/// 0 - None, 1 - Working Draft, 2 - Approved, (3 - All)
|
|
/// </summary>
|
|
public int VersionType
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("VersionType", true);
|
|
return _VersionType;
|
|
}
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
set
|
|
{
|
|
CanWriteProperty("VersionType", true);
|
|
if (_VersionType != value)
|
|
{
|
|
_VersionType = value;
|
|
PropertyHasChanged();
|
|
}
|
|
}
|
|
}
|
|
private int _PermValue;
|
|
/// <summary>
|
|
/// 1 - Read, 2 - Write, 4 - Create, 8 - Delete (15 - All)
|
|
/// </summary>
|
|
public int PermValue
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("PermValue", true);
|
|
return _PermValue;
|
|
}
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
set
|
|
{
|
|
CanWriteProperty("PermValue", true);
|
|
if (_PermValue != value)
|
|
{
|
|
_PermValue = value;
|
|
PropertyHasChanged();
|
|
}
|
|
}
|
|
}
|
|
private int _PermAD;
|
|
/// <summary>
|
|
/// 0 - Allow, 1 - Deny
|
|
/// </summary>
|
|
public int PermAD
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("PermAD", true);
|
|
return _PermAD;
|
|
}
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
set
|
|
{
|
|
CanWriteProperty("PermAD", true);
|
|
if (_PermAD != value)
|
|
{
|
|
_PermAD = value;
|
|
PropertyHasChanged();
|
|
}
|
|
}
|
|
}
|
|
private string _StartDate = string.Empty;
|
|
public string StartDate
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("StartDate", true);
|
|
return _StartDate;
|
|
}
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
set
|
|
{
|
|
CanWriteProperty("StartDate", true);
|
|
if (value == null) value = string.Empty;
|
|
_StartDate = value;
|
|
try
|
|
{
|
|
SmartDate tmp = new SmartDate(value);
|
|
if (_StartDate != tmp.ToString())
|
|
{
|
|
_StartDate = tmp.ToString();
|
|
// CSLATODO: Any Cross Property Validation
|
|
}
|
|
}
|
|
catch
|
|
{
|
|
}
|
|
PropertyHasChanged();
|
|
}
|
|
}
|
|
private string _EndDate = string.Empty;
|
|
public string EndDate
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("EndDate", true);
|
|
return _EndDate;
|
|
}
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
set
|
|
{
|
|
CanWriteProperty("EndDate", true);
|
|
if (value == null) value = string.Empty;
|
|
_EndDate = value;
|
|
try
|
|
{
|
|
SmartDate tmp = new SmartDate(value);
|
|
if (_EndDate != tmp.ToString())
|
|
{
|
|
_EndDate = tmp.ToString();
|
|
// CSLATODO: Any Cross Property Validation
|
|
}
|
|
}
|
|
catch
|
|
{
|
|
}
|
|
PropertyHasChanged();
|
|
}
|
|
}
|
|
private string _Config = string.Empty;
|
|
public string Config
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("Config", true);
|
|
return _Config;
|
|
}
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
set
|
|
{
|
|
CanWriteProperty("Config", true);
|
|
if (value == null) value = string.Empty;
|
|
if (_Config != value)
|
|
{
|
|
_Config = value;
|
|
PropertyHasChanged();
|
|
}
|
|
}
|
|
}
|
|
private DateTime _DTS = new DateTime();
|
|
public DateTime DTS
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("DTS", true);
|
|
return _DTS;
|
|
}
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
set
|
|
{
|
|
CanWriteProperty("DTS", true);
|
|
if (_DTS != value)
|
|
{
|
|
_DTS = value;
|
|
PropertyHasChanged();
|
|
}
|
|
}
|
|
}
|
|
private string _UsrID = string.Empty;
|
|
public string UsrID
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("UsrID", true);
|
|
return _UsrID;
|
|
}
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
set
|
|
{
|
|
CanWriteProperty("UsrID", true);
|
|
if (value == null) value = string.Empty;
|
|
if (_UsrID != value)
|
|
{
|
|
_UsrID = value;
|
|
PropertyHasChanged();
|
|
}
|
|
}
|
|
}
|
|
private byte[] _LastChanged = new byte[8];//timestamp
|
|
public override bool IsDirty
|
|
{
|
|
get
|
|
{
|
|
if ( base.IsDirty )
|
|
return true;
|
|
return IsDirtyList(new List<object>());
|
|
}
|
|
}
|
|
public bool IsDirtyList(List<object> list)
|
|
{
|
|
if (base.IsDirty || list.Contains(this))
|
|
return base.IsDirty;
|
|
list.Add(this);
|
|
return base.IsDirty || (_MyRole == null ? false : _MyRole.IsDirtyList(list));
|
|
}
|
|
public override bool IsValid
|
|
{
|
|
get { return IsValidList(new List<object>()); }
|
|
}
|
|
public bool IsValidList(List<object> list)
|
|
{
|
|
if(list.Contains(this))
|
|
return (IsNew && !IsDirty) ? true : base.IsValid;
|
|
list.Add(this);
|
|
return ((IsNew && !IsDirty) ? true : base.IsValid) && (_MyRole == null ? true : _MyRole.IsValidList(list));
|
|
}
|
|
// CSLATODO: Replace base Permission.ToString function as necessary
|
|
/// <summary>
|
|
/// Overrides Base ToString
|
|
/// </summary>
|
|
/// <returns>A string representation of current Permission</returns>
|
|
//public override string ToString()
|
|
//{
|
|
// return base.ToString();
|
|
//}
|
|
// CSLATODO: Check Permission.GetIdValue to assure that the ID returned is unique
|
|
/// <summary>
|
|
/// Overrides Base GetIdValue - Used internally by CSLA to determine equality
|
|
/// </summary>
|
|
/// <returns>A Unique ID for the current Permission</returns>
|
|
protected override object GetIdValue()
|
|
{
|
|
return MyPermissionUnique; // Absolutely Unique ID
|
|
}
|
|
#endregion
|
|
#region ValidationRules
|
|
[NonSerialized]
|
|
private bool _CheckingBrokenRules = false;
|
|
public IVEHasBrokenRules HasBrokenRules
|
|
{
|
|
get
|
|
{
|
|
if (_CheckingBrokenRules) return null;
|
|
if ((IsDirty || !IsNew) && BrokenRulesCollection.Count > 0) return this;
|
|
try
|
|
{
|
|
_CheckingBrokenRules = true;
|
|
IVEHasBrokenRules hasBrokenRules = null;
|
|
if (_MyRole != null && (hasBrokenRules = _MyRole.HasBrokenRules) != null) return hasBrokenRules;
|
|
return hasBrokenRules;
|
|
}
|
|
finally
|
|
{
|
|
_CheckingBrokenRules = false;
|
|
}
|
|
}
|
|
}
|
|
public BrokenRulesCollection BrokenRules
|
|
{
|
|
get
|
|
{
|
|
IVEHasBrokenRules hasBrokenRules = HasBrokenRules;
|
|
if (this.Equals(hasBrokenRules)) return BrokenRulesCollection;
|
|
return (hasBrokenRules != null ? hasBrokenRules.BrokenRules : null);
|
|
}
|
|
}
|
|
protected override void AddBusinessRules()
|
|
{
|
|
ValidationRules.AddRule<Permission>(MyRoleRequired, "MyRole");
|
|
ValidationRules.AddRule(
|
|
Csla.Validation.CommonRules.StringRequired, "StartDate");
|
|
ValidationRules.AddRule<Permission>(StartDateValid, "StartDate");
|
|
ValidationRules.AddRule<Permission>(EndDateValid, "EndDate");
|
|
ValidationRules.AddRule(
|
|
Csla.Validation.CommonRules.StringMaxLength,
|
|
new Csla.Validation.CommonRules.MaxLengthRuleArgs("Config", 1073741823));
|
|
ValidationRules.AddRule(
|
|
Csla.Validation.CommonRules.StringRequired, "UsrID");
|
|
ValidationRules.AddRule(
|
|
Csla.Validation.CommonRules.StringMaxLength,
|
|
new Csla.Validation.CommonRules.MaxLengthRuleArgs("UsrID", 100));
|
|
//ValidationRules.AddDependantProperty("x", "y");
|
|
_PermissionExtension.AddValidationRules(ValidationRules);
|
|
// CSLATODO: Add other validation rules
|
|
}
|
|
protected override void AddInstanceBusinessRules()
|
|
{
|
|
_PermissionExtension.AddInstanceValidationRules(ValidationRules);
|
|
// CSLATODO: Add other validation rules
|
|
}
|
|
private static bool StartDateValid(Permission target, Csla.Validation.RuleArgs e)
|
|
{
|
|
try
|
|
{
|
|
DateTime tmp = SmartDate.StringToDate(target._StartDate);
|
|
}
|
|
catch
|
|
{
|
|
e.Description = "Invalid Date";
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
private static bool EndDateValid(Permission target, Csla.Validation.RuleArgs e)
|
|
{
|
|
try
|
|
{
|
|
DateTime tmp = SmartDate.StringToDate(target._EndDate);
|
|
}
|
|
catch
|
|
{
|
|
e.Description = "Invalid Date";
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
private static bool MyRoleRequired(Permission target, Csla.Validation.RuleArgs e)
|
|
{
|
|
if (target._RID == 0 && target._MyRole == null) // Required field missing
|
|
{
|
|
e.Description = "Required";
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
// Sample data comparison validation rule
|
|
//private bool StartDateGTEndDate(object target, Csla.Validation.RuleArgs e)
|
|
//{
|
|
// if (_started > _ended)
|
|
// {
|
|
// e.Description = "Start date can't be after end date";
|
|
// return false;
|
|
// }
|
|
// else
|
|
// return true;
|
|
//}
|
|
#endregion
|
|
#region Authorization Rules
|
|
protected override void AddAuthorizationRules()
|
|
{
|
|
//CSLATODO: Who can read/write which fields
|
|
//AuthorizationRules.AllowRead(PID, "<Role(s)>");
|
|
//AuthorizationRules.AllowRead(RID, "<Role(s)>");
|
|
//AuthorizationRules.AllowRead(PermLevel, "<Role(s)>");
|
|
//AuthorizationRules.AllowRead(VersionType, "<Role(s)>");
|
|
//AuthorizationRules.AllowRead(PermValue, "<Role(s)>");
|
|
//AuthorizationRules.AllowRead(PermAD, "<Role(s)>");
|
|
//AuthorizationRules.AllowRead(StartDate, "<Role(s)>");
|
|
//AuthorizationRules.AllowRead(EndDate, "<Role(s)>");
|
|
//AuthorizationRules.AllowRead(Config, "<Role(s)>");
|
|
//AuthorizationRules.AllowRead(DTS, "<Role(s)>");
|
|
//AuthorizationRules.AllowRead(UsrID, "<Role(s)>");
|
|
//AuthorizationRules.AllowWrite(RID, "<Role(s)>");
|
|
//AuthorizationRules.AllowWrite(PermLevel, "<Role(s)>");
|
|
//AuthorizationRules.AllowWrite(VersionType, "<Role(s)>");
|
|
//AuthorizationRules.AllowWrite(PermValue, "<Role(s)>");
|
|
//AuthorizationRules.AllowWrite(PermAD, "<Role(s)>");
|
|
//AuthorizationRules.AllowWrite(StartDate, "<Role(s)>");
|
|
//AuthorizationRules.AllowWrite(EndDate, "<Role(s)>");
|
|
//AuthorizationRules.AllowWrite(Config, "<Role(s)>");
|
|
//AuthorizationRules.AllowWrite(DTS, "<Role(s)>");
|
|
//AuthorizationRules.AllowWrite(UsrID, "<Role(s)>");
|
|
_PermissionExtension.AddAuthorizationRules(AuthorizationRules);
|
|
}
|
|
protected override void AddInstanceAuthorizationRules()
|
|
{
|
|
//CSLATODO: Who can read/write which fields
|
|
_PermissionExtension.AddInstanceAuthorizationRules(AuthorizationRules);
|
|
}
|
|
public static bool CanAddObject()
|
|
{
|
|
// CSLATODO: Can Add Authorization
|
|
//return Csla.ApplicationContext.User.IsInRole("ProjectManager");
|
|
return true;
|
|
}
|
|
public static bool CanGetObject()
|
|
{
|
|
// CSLATODO: CanGet Authorization
|
|
return true;
|
|
}
|
|
public static bool CanDeleteObject()
|
|
{
|
|
// CSLATODO: CanDelete Authorization
|
|
//bool result = false;
|
|
//if (Csla.ApplicationContext.User.IsInRole("ProjectManager"))result = true;
|
|
//if (Csla.ApplicationContext.User.IsInRole("Administrator"))result = true;
|
|
//return result;
|
|
return true;
|
|
}
|
|
public static bool CanEditObject()
|
|
{
|
|
// CSLATODO: CanEdit Authorization
|
|
//return Csla.ApplicationContext.User.IsInRole("ProjectManager");
|
|
return true;
|
|
}
|
|
#endregion
|
|
#region Factory Methods
|
|
public int CurrentEditLevel
|
|
{ get { return EditLevel; } }
|
|
private static int _PermissionUnique = 0;
|
|
protected static int PermissionUnique
|
|
{ get { return ++_PermissionUnique; } }
|
|
private int _MyPermissionUnique = PermissionUnique;
|
|
public int MyPermissionUnique // Absolutely Unique ID - Editable
|
|
{ get { return _MyPermissionUnique; } }
|
|
protected Permission()
|
|
{/* require use of factory methods */
|
|
AddToCache(this);
|
|
}
|
|
public void Dispose()
|
|
{
|
|
RemoveFromDictionaries();
|
|
}
|
|
private void RemoveFromDictionaries()
|
|
{
|
|
RemoveFromCache(this);
|
|
if (_CacheByPrimaryKey.ContainsKey(PID.ToString()))
|
|
{
|
|
List<Permission> listPermission = _CacheByPrimaryKey[PID.ToString()]; // Get the list of items
|
|
while (listPermission.Contains(this)) listPermission.Remove(this); // Remove the item from the list
|
|
if (listPermission.Count == 0) //If there are no items left in the list
|
|
_CacheByPrimaryKey.Remove(PID.ToString()); // remove the list
|
|
}
|
|
}
|
|
public static Permission New()
|
|
{
|
|
if (!CanAddObject())
|
|
throw new System.Security.SecurityException("User not authorized to add a Permission");
|
|
try
|
|
{
|
|
return DataPortal.Create<Permission>();
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on Permission.New", ex);
|
|
}
|
|
}
|
|
public static Permission New(Role myRole, int permLevel, int versionType, int permValue)
|
|
{
|
|
Permission tmp = Permission.New();
|
|
tmp.MyRole = myRole;
|
|
tmp.PermLevel = permLevel;
|
|
tmp.VersionType = versionType;
|
|
tmp.PermValue = permValue;
|
|
return tmp;
|
|
}
|
|
public static Permission New(Role myRole, int permLevel, int versionType, int permValue, int permAD, string startDate, string endDate, string config, DateTime dts, string usrID)
|
|
{
|
|
Permission tmp = Permission.New();
|
|
tmp.MyRole = myRole;
|
|
tmp.PermLevel = permLevel;
|
|
tmp.VersionType = versionType;
|
|
tmp.PermValue = permValue;
|
|
tmp.PermAD = permAD;
|
|
tmp.StartDate = startDate;
|
|
tmp.EndDate = endDate;
|
|
tmp.Config = config;
|
|
tmp.DTS = dts;
|
|
tmp.UsrID = usrID;
|
|
return tmp;
|
|
}
|
|
public static Permission MakePermission(Role myRole, int permLevel, int versionType, int permValue, int permAD, string startDate, string endDate, string config, DateTime dts, string usrID)
|
|
{
|
|
Permission tmp = Permission.New(myRole, permLevel, versionType, permValue, permAD, startDate, endDate, config, dts, usrID);
|
|
if (tmp.IsSavable)
|
|
{
|
|
Permission tmp2 = tmp;
|
|
tmp = tmp2.Save();
|
|
tmp2.Dispose();
|
|
}
|
|
else
|
|
{
|
|
Csla.Validation.BrokenRulesCollection brc = tmp.ValidationRules.GetBrokenRules();
|
|
tmp._ErrorMessage = "Failed Validation:";
|
|
foreach (Csla.Validation.BrokenRule br in brc)
|
|
{
|
|
tmp._ErrorMessage += "\r\n\tFailure: " + br.RuleName;
|
|
}
|
|
}
|
|
return tmp;
|
|
}
|
|
public static Permission New(Role myRole, int permLevel, int versionType, int permValue, string endDate, string config)
|
|
{
|
|
Permission tmp = Permission.New();
|
|
tmp.MyRole = myRole;
|
|
tmp.PermLevel = permLevel;
|
|
tmp.VersionType = versionType;
|
|
tmp.PermValue = permValue;
|
|
tmp.EndDate = endDate;
|
|
tmp.Config = config;
|
|
return tmp;
|
|
}
|
|
public static Permission MakePermission(Role myRole, int permLevel, int versionType, int permValue, string endDate, string config)
|
|
{
|
|
Permission tmp = Permission.New(myRole, permLevel, versionType, permValue, endDate, config);
|
|
if (tmp.IsSavable)
|
|
{
|
|
Permission tmp2 = tmp;
|
|
tmp = tmp2.Save();
|
|
tmp2.Dispose();
|
|
}
|
|
else
|
|
{
|
|
Csla.Validation.BrokenRulesCollection brc = tmp.ValidationRules.GetBrokenRules();
|
|
tmp._ErrorMessage = "Failed Validation:";
|
|
foreach (Csla.Validation.BrokenRule br in brc)
|
|
{
|
|
tmp._ErrorMessage += "\r\n\tFailure: " + br.RuleName;
|
|
}
|
|
}
|
|
return tmp;
|
|
}
|
|
public static Permission Get(int pid)
|
|
{
|
|
if (!CanGetObject())
|
|
throw new System.Security.SecurityException("User not authorized to view a Permission");
|
|
try
|
|
{
|
|
Permission tmp = GetCachedByPrimaryKey(pid);
|
|
if (tmp == null)
|
|
{
|
|
tmp = DataPortal.Fetch<Permission>(new PKCriteria(pid));
|
|
AddToCache(tmp);
|
|
}
|
|
if (tmp.ErrorMessage == "No Record Found")
|
|
{
|
|
tmp.Dispose(); // Clean-up Permission
|
|
tmp = null;
|
|
}
|
|
return tmp;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on Permission.Get", ex);
|
|
}
|
|
}
|
|
public static Permission Get(SafeDataReader dr)
|
|
{
|
|
if (dr.Read()) return new Permission(dr);
|
|
return null;
|
|
}
|
|
internal Permission(SafeDataReader dr)
|
|
{
|
|
ReadData(dr);
|
|
}
|
|
public static void Delete(int pid)
|
|
{
|
|
if (!CanDeleteObject())
|
|
throw new System.Security.SecurityException("User not authorized to remove a Permission");
|
|
try
|
|
{
|
|
DataPortal.Delete(new PKCriteria(pid));
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on Permission.Delete", ex);
|
|
}
|
|
}
|
|
public override Permission Save()
|
|
{
|
|
if (IsDeleted && !CanDeleteObject())
|
|
throw new System.Security.SecurityException("User not authorized to remove a Permission");
|
|
else if (IsNew && !CanAddObject())
|
|
throw new System.Security.SecurityException("User not authorized to add a Permission");
|
|
else if (!CanEditObject())
|
|
throw new System.Security.SecurityException("User not authorized to update a Permission");
|
|
try
|
|
{
|
|
BuildRefreshList();
|
|
Permission permission = base.Save();
|
|
RemoveFromDictionaries(); // if save is successful remove the previous Folder from the cache
|
|
AddToCache(permission);//Refresh the item in AllList
|
|
ProcessRefreshList();
|
|
return permission;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on CSLA Save", ex);
|
|
}
|
|
}
|
|
#endregion
|
|
#region Data Access Portal
|
|
[Serializable()]
|
|
protected class PKCriteria
|
|
{
|
|
private int _PID;
|
|
public int PID
|
|
{ get { return _PID; } }
|
|
public PKCriteria(int pid)
|
|
{
|
|
_PID = pid;
|
|
}
|
|
}
|
|
// CSLATODO: If Create needs to access DB - It should not be marked RunLocal
|
|
[RunLocal()]
|
|
private new void DataPortal_Create()
|
|
{
|
|
_PID = NextPID;
|
|
// Database Defaults
|
|
_PermAD = _PermissionExtension.DefaultPermAD;
|
|
_StartDate = _PermissionExtension.DefaultStartDate;
|
|
_DTS = _PermissionExtension.DefaultDTS;
|
|
_UsrID = _PermissionExtension.DefaultUsrID;
|
|
// CSLATODO: Add any defaults that are necessary
|
|
ValidationRules.CheckRules();
|
|
}
|
|
private void ReadData(SafeDataReader dr)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Permission.ReadData", GetHashCode());
|
|
try
|
|
{
|
|
_PID = dr.GetInt32("PID");
|
|
_RID = dr.GetInt32("RID");
|
|
_PermLevel = dr.GetInt32("PermLevel");
|
|
_VersionType = dr.GetInt32("VersionType");
|
|
_PermValue = dr.GetInt32("PermValue");
|
|
_PermAD = dr.GetInt32("PermAD");
|
|
_StartDate = dr.GetSmartDate("StartDate").Text;
|
|
_EndDate = dr.GetSmartDate("EndDate").Text;
|
|
_Config = dr.GetString("Config");
|
|
_DTS = dr.GetDateTime("DTS");
|
|
_UsrID = dr.GetString("UsrID");
|
|
dr.GetBytes("LastChanged", 0, _LastChanged, 0, 8);
|
|
MarkOld();
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Permission.ReadData", ex);
|
|
_ErrorMessage = ex.Message;
|
|
throw new DbCslaException("Permission.ReadData", ex);
|
|
}
|
|
}
|
|
private void DataPortal_Fetch(PKCriteria criteria)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Permission.DataPortal_Fetch", GetHashCode());
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
ApplicationContext.LocalContext["cn"] = cn;
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "getPermission";
|
|
cm.Parameters.AddWithValue("@PID", criteria.PID);
|
|
using (SafeDataReader dr = new SafeDataReader(cm.ExecuteReader()))
|
|
{
|
|
if (!dr.Read())
|
|
{
|
|
_ErrorMessage = "No Record Found";
|
|
return;
|
|
}
|
|
ReadData(dr);
|
|
}
|
|
}
|
|
// removing of item only needed for local data portal
|
|
if (ApplicationContext.ExecutionLocation == ApplicationContext.ExecutionLocations.Client)
|
|
ApplicationContext.LocalContext.Remove("cn");
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Permission.DataPortal_Fetch", ex);
|
|
_ErrorMessage = ex.Message;
|
|
throw new DbCslaException("Permission.DataPortal_Fetch", ex);
|
|
}
|
|
}
|
|
[Transactional(TransactionalTypes.TransactionScope)]
|
|
protected override void DataPortal_Insert()
|
|
{
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
ApplicationContext.LocalContext["cn"] = cn;
|
|
SQLInsert();
|
|
// removing of item only needed for local data portal
|
|
if (ApplicationContext.ExecutionLocation == ApplicationContext.ExecutionLocations.Client)
|
|
ApplicationContext.LocalContext.Remove("cn");
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Permission.DataPortal_Insert", ex);
|
|
_ErrorMessage = ex.Message;
|
|
throw new DbCslaException("Permission.DataPortal_Insert", ex);
|
|
}
|
|
finally
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Permission.DataPortal_Insert", GetHashCode());
|
|
}
|
|
}
|
|
[Transactional(TransactionalTypes.TransactionScope)]
|
|
internal void SQLInsert()
|
|
{
|
|
if (!this.IsDirty) return;
|
|
try
|
|
{
|
|
if (_MyRole != null) _MyRole.Update();
|
|
SqlConnection cn = (SqlConnection)ApplicationContext.LocalContext["cn"];
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "addPermission";
|
|
// Input All Fields - Except Calculated Columns
|
|
cm.Parameters.AddWithValue("@RID", RID);
|
|
cm.Parameters.AddWithValue("@PermLevel", _PermLevel);
|
|
cm.Parameters.AddWithValue("@VersionType", _VersionType);
|
|
cm.Parameters.AddWithValue("@PermValue", _PermValue);
|
|
cm.Parameters.AddWithValue("@PermAD", _PermAD);
|
|
cm.Parameters.AddWithValue("@StartDate", new SmartDate(_StartDate).DBValue);
|
|
cm.Parameters.AddWithValue("@EndDate", new SmartDate(_EndDate).DBValue);
|
|
cm.Parameters.AddWithValue("@Config", _Config);
|
|
if (_DTS.Year >= 1753 && _DTS.Year <= 9999) cm.Parameters.AddWithValue("@DTS", _DTS);
|
|
cm.Parameters.AddWithValue("@UsrID", _UsrID);
|
|
// Output Calculated Columns
|
|
SqlParameter param_PID = new SqlParameter("@newPID", SqlDbType.Int);
|
|
param_PID.Direction = ParameterDirection.Output;
|
|
cm.Parameters.Add(param_PID);
|
|
SqlParameter param_LastChanged = new SqlParameter("@newLastChanged", SqlDbType.Timestamp);
|
|
param_LastChanged.Direction = ParameterDirection.Output;
|
|
cm.Parameters.Add(param_LastChanged);
|
|
// CSLATODO: Define any additional output parameters
|
|
cm.ExecuteNonQuery();
|
|
// Save all values being returned from the Procedure
|
|
_PID = (int)cm.Parameters["@newPID"].Value;
|
|
_LastChanged = (byte[])cm.Parameters["@newLastChanged"].Value;
|
|
}
|
|
MarkOld();
|
|
// update child objects
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Permission.SQLInsert", GetHashCode());
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Permission.SQLInsert", ex);
|
|
_ErrorMessage = ex.Message;
|
|
throw new DbCslaException("Permission.SQLInsert", ex);
|
|
}
|
|
}
|
|
[Transactional(TransactionalTypes.TransactionScope)]
|
|
public static byte[] Add(SqlConnection cn, ref int pid, Role myRole, int permLevel, int versionType, int permValue, int permAD, SmartDate startDate, SmartDate endDate, string config, DateTime dts, string usrID)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Permission.Add", 0);
|
|
try
|
|
{
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "addPermission";
|
|
// Input All Fields - Except Calculated Columns
|
|
cm.Parameters.AddWithValue("@RID", myRole.RID);
|
|
cm.Parameters.AddWithValue("@PermLevel", permLevel);
|
|
cm.Parameters.AddWithValue("@VersionType", versionType);
|
|
cm.Parameters.AddWithValue("@PermValue", permValue);
|
|
cm.Parameters.AddWithValue("@PermAD", permAD);
|
|
cm.Parameters.AddWithValue("@StartDate", startDate.DBValue);
|
|
cm.Parameters.AddWithValue("@EndDate", endDate.DBValue);
|
|
cm.Parameters.AddWithValue("@Config", config);
|
|
if (dts.Year >= 1753 && dts.Year <= 9999) cm.Parameters.AddWithValue("@DTS", dts);
|
|
cm.Parameters.AddWithValue("@UsrID", usrID);
|
|
// Output Calculated Columns
|
|
SqlParameter param_PID = new SqlParameter("@newPID", SqlDbType.Int);
|
|
param_PID.Direction = ParameterDirection.Output;
|
|
cm.Parameters.Add(param_PID);
|
|
SqlParameter param_LastChanged = new SqlParameter("@newLastChanged", SqlDbType.Timestamp);
|
|
param_LastChanged.Direction = ParameterDirection.Output;
|
|
cm.Parameters.Add(param_LastChanged);
|
|
// CSLATODO: Define any additional output parameters
|
|
cm.ExecuteNonQuery();
|
|
// Save all values being returned from the Procedure
|
|
pid = (int)cm.Parameters["@newPID"].Value;
|
|
return (byte[])cm.Parameters["@newLastChanged"].Value;
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Permission.Add", ex);
|
|
throw new DbCslaException("Permission.Add", ex);
|
|
}
|
|
}
|
|
[Transactional(TransactionalTypes.TransactionScope)]
|
|
protected override void DataPortal_Update()
|
|
{
|
|
if (!IsDirty) return; // If not dirty - nothing to do
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Permission.DataPortal_Update", GetHashCode());
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
ApplicationContext.LocalContext["cn"] = cn;
|
|
SQLUpdate();
|
|
// removing of item only needed for local data portal
|
|
if (ApplicationContext.ExecutionLocation == ApplicationContext.ExecutionLocations.Client)
|
|
ApplicationContext.LocalContext.Remove("cn");
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Permission.DataPortal_Update", ex);
|
|
_ErrorMessage = ex.Message;
|
|
if (!ex.Message.EndsWith("has been edited by another user.")) throw ex;
|
|
}
|
|
}
|
|
[Transactional(TransactionalTypes.TransactionScope)]
|
|
internal void SQLUpdate()
|
|
{
|
|
if (!IsDirty) return; // If not dirty - nothing to do
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Permission.SQLUpdate", GetHashCode());
|
|
try
|
|
{
|
|
if (_MyRole != null) _MyRole.Update();
|
|
SqlConnection cn = (SqlConnection)ApplicationContext.LocalContext["cn"];
|
|
if (base.IsDirty)
|
|
{
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "updatePermission";
|
|
// All Fields including Calculated Fields
|
|
cm.Parameters.AddWithValue("@PID", _PID);
|
|
cm.Parameters.AddWithValue("@RID", RID);
|
|
cm.Parameters.AddWithValue("@PermLevel", _PermLevel);
|
|
cm.Parameters.AddWithValue("@VersionType", _VersionType);
|
|
cm.Parameters.AddWithValue("@PermValue", _PermValue);
|
|
cm.Parameters.AddWithValue("@PermAD", _PermAD);
|
|
cm.Parameters.AddWithValue("@StartDate", new SmartDate(_StartDate).DBValue);
|
|
cm.Parameters.AddWithValue("@EndDate", new SmartDate(_EndDate).DBValue);
|
|
cm.Parameters.AddWithValue("@Config", _Config);
|
|
if (_DTS.Year >= 1753 && _DTS.Year <= 9999) cm.Parameters.AddWithValue("@DTS", _DTS);
|
|
cm.Parameters.AddWithValue("@UsrID", _UsrID);
|
|
cm.Parameters.AddWithValue("@LastChanged", _LastChanged);
|
|
// Output Calculated Columns
|
|
SqlParameter param_LastChanged = new SqlParameter("@newLastChanged", SqlDbType.Timestamp);
|
|
param_LastChanged.Direction = ParameterDirection.Output;
|
|
cm.Parameters.Add(param_LastChanged);
|
|
// CSLATODO: Define any additional output parameters
|
|
cm.ExecuteNonQuery();
|
|
// Save all values being returned from the Procedure
|
|
_LastChanged = (byte[])cm.Parameters["@newLastChanged"].Value;
|
|
}
|
|
}
|
|
MarkOld();
|
|
// use the open connection to update child objects
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Permission.SQLUpdate", ex);
|
|
_ErrorMessage = ex.Message;
|
|
if (!ex.Message.EndsWith("has been edited by another user.")) throw ex;
|
|
}
|
|
}
|
|
internal void Update()
|
|
{
|
|
if (!this.IsDirty) return;
|
|
if (base.IsDirty)
|
|
{
|
|
SqlConnection cn = (SqlConnection)ApplicationContext.LocalContext["cn"];
|
|
if (IsNew)
|
|
_LastChanged = Permission.Add(cn, ref _PID, _MyRole, _PermLevel, _VersionType, _PermValue, _PermAD, new SmartDate(_StartDate), new SmartDate(_EndDate), _Config, _DTS, _UsrID);
|
|
else
|
|
_LastChanged = Permission.Update(cn, ref _PID, _RID, _PermLevel, _VersionType, _PermValue, _PermAD, new SmartDate(_StartDate), new SmartDate(_EndDate), _Config, _DTS, _UsrID, ref _LastChanged);
|
|
MarkOld();
|
|
}
|
|
}
|
|
[Transactional(TransactionalTypes.TransactionScope)]
|
|
public static byte[] Update(SqlConnection cn, ref int pid, int rid, int permLevel, int versionType, int permValue, int permAD, SmartDate startDate, SmartDate endDate, string config, DateTime dts, string usrID, ref byte[] lastChanged)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Permission.Update", 0);
|
|
try
|
|
{
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "updatePermission";
|
|
// Input All Fields - Except Calculated Columns
|
|
cm.Parameters.AddWithValue("@PID", pid);
|
|
cm.Parameters.AddWithValue("@RID", rid);
|
|
cm.Parameters.AddWithValue("@PermLevel", permLevel);
|
|
cm.Parameters.AddWithValue("@VersionType", versionType);
|
|
cm.Parameters.AddWithValue("@PermValue", permValue);
|
|
cm.Parameters.AddWithValue("@PermAD", permAD);
|
|
cm.Parameters.AddWithValue("@StartDate", startDate);
|
|
cm.Parameters.AddWithValue("@EndDate", endDate);
|
|
cm.Parameters.AddWithValue("@Config", config);
|
|
if (dts.Year >= 1753 && dts.Year <= 9999) cm.Parameters.AddWithValue("@DTS", dts);
|
|
cm.Parameters.AddWithValue("@UsrID", usrID);
|
|
cm.Parameters.AddWithValue("@LastChanged", lastChanged);
|
|
// Output Calculated Columns
|
|
SqlParameter param_LastChanged = new SqlParameter("@newLastChanged", SqlDbType.Timestamp);
|
|
param_LastChanged.Direction = ParameterDirection.Output;
|
|
cm.Parameters.Add(param_LastChanged);
|
|
// CSLATODO: Define any additional output parameters
|
|
cm.ExecuteNonQuery();
|
|
// Save all values being returned from the Procedure
|
|
return (byte[])cm.Parameters["@newLastChanged"].Value;
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Permission.Update", ex);
|
|
throw new DbCslaException("Permission.Update", ex);
|
|
}
|
|
}
|
|
[Transactional(TransactionalTypes.TransactionScope)]
|
|
protected override void DataPortal_DeleteSelf()
|
|
{
|
|
DataPortal_Delete(new PKCriteria(_PID));
|
|
}
|
|
[Transactional(TransactionalTypes.TransactionScope)]
|
|
private void DataPortal_Delete(PKCriteria criteria)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Permission.DataPortal_Delete", GetHashCode());
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "deletePermission";
|
|
cm.Parameters.AddWithValue("@PID", criteria.PID);
|
|
cm.ExecuteNonQuery();
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Permission.DataPortal_Delete", ex);
|
|
_ErrorMessage = ex.Message;
|
|
throw new DbCslaException("Permission.DataPortal_Delete", ex);
|
|
}
|
|
}
|
|
[Transactional(TransactionalTypes.TransactionScope)]
|
|
public static void Remove(SqlConnection cn, int pid)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Permission.Remove", 0);
|
|
try
|
|
{
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "deletePermission";
|
|
// Input PK Fields
|
|
cm.Parameters.AddWithValue("@PID", pid);
|
|
// CSLATODO: Define any additional output parameters
|
|
cm.ExecuteNonQuery();
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Permission.Remove", ex);
|
|
throw new DbCslaException("Permission.Remove", ex);
|
|
}
|
|
}
|
|
#endregion
|
|
#region Exists
|
|
public static bool Exists(int pid)
|
|
{
|
|
ExistsCommand result;
|
|
try
|
|
{
|
|
result = DataPortal.Execute<ExistsCommand>(new ExistsCommand(pid));
|
|
return result.Exists;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on Permission.Exists", ex);
|
|
}
|
|
}
|
|
[Serializable()]
|
|
private class ExistsCommand : CommandBase
|
|
{
|
|
private int _PID;
|
|
private bool _exists;
|
|
public bool Exists
|
|
{
|
|
get { return _exists; }
|
|
}
|
|
public ExistsCommand(int pid)
|
|
{
|
|
_PID = pid;
|
|
}
|
|
protected override void DataPortal_Execute()
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Permission.DataPortal_Execute", GetHashCode());
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
cn.Open();
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "existsPermission";
|
|
cm.Parameters.AddWithValue("@PID", _PID);
|
|
int count = (int)cm.ExecuteScalar();
|
|
_exists = (count > 0);
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Permission.DataPortal_Execute", ex);
|
|
throw new DbCslaException("Permission.DataPortal_Execute", ex);
|
|
}
|
|
}
|
|
}
|
|
#endregion
|
|
// Standard Default Code
|
|
#region extension
|
|
PermissionExtension _PermissionExtension = new PermissionExtension();
|
|
[Serializable()]
|
|
partial class PermissionExtension : extensionBase
|
|
{
|
|
}
|
|
[Serializable()]
|
|
class extensionBase
|
|
{
|
|
// Default Values
|
|
public virtual int DefaultPermAD
|
|
{
|
|
get { return 0; }
|
|
}
|
|
public virtual string DefaultStartDate
|
|
{
|
|
get { return DateTime.Now.ToShortDateString(); }
|
|
}
|
|
public virtual DateTime DefaultDTS
|
|
{
|
|
get { return DateTime.Now; }
|
|
}
|
|
public virtual string DefaultUsrID
|
|
{
|
|
get { return Environment.UserName.ToUpper(); }
|
|
}
|
|
// Authorization Rules
|
|
public virtual void AddAuthorizationRules(Csla.Security.AuthorizationRules rules)
|
|
{
|
|
// Needs to be overriden to add new authorization rules
|
|
}
|
|
// Instance Authorization Rules
|
|
public virtual void AddInstanceAuthorizationRules(Csla.Security.AuthorizationRules rules)
|
|
{
|
|
// Needs to be overriden to add new authorization rules
|
|
}
|
|
// Validation Rules
|
|
public virtual void AddValidationRules(Csla.Validation.ValidationRules rules)
|
|
{
|
|
// Needs to be overriden to add new validation rules
|
|
}
|
|
// InstanceValidation Rules
|
|
public virtual void AddInstanceValidationRules(Csla.Validation.ValidationRules rules)
|
|
{
|
|
// Needs to be overriden to add new validation rules
|
|
}
|
|
}
|
|
#endregion
|
|
} // Class
|
|
#region Converter
|
|
internal class PermissionConverter : ExpandableObjectConverter
|
|
{
|
|
public override object ConvertTo(ITypeDescriptorContext context, System.Globalization.CultureInfo culture, object value, Type destType)
|
|
{
|
|
if (destType == typeof(string) && value is Permission)
|
|
{
|
|
// Return the ToString value
|
|
return ((Permission)value).ToString();
|
|
}
|
|
return base.ConvertTo(context, culture, value, destType);
|
|
}
|
|
}
|
|
#endregion
|
|
} // Namespace
|
|
|
|
|
|
//// The following is a sample Extension File. You can use it to create PermissionExt.cs
|
|
//using System;
|
|
//using System.Collections.Generic;
|
|
//using System.Text;
|
|
//using Csla;
|
|
|
|
//namespace VEPROMS.CSLA.Library
|
|
//{
|
|
// public partial class Permission
|
|
// {
|
|
// partial class PermissionExtension : extensionBase
|
|
// {
|
|
// // CSLATODO: Override automatic defaults
|
|
// public virtual int DefaultPermAD
|
|
// {
|
|
// get { return 0; }
|
|
// }
|
|
// public virtual SmartDate DefaultStartDate
|
|
// {
|
|
// get { return DateTime.Now.ToShortDateString(); }
|
|
// }
|
|
// public virtual DateTime DefaultDTS
|
|
// {
|
|
// get { return DateTime.Now; }
|
|
// }
|
|
// public virtual string DefaultUsrID
|
|
// {
|
|
// get { return Environment.UserName.ToUpper(); }
|
|
// }
|
|
// public new void AddAuthorizationRules(Csla.Security.AuthorizationRules rules)
|
|
// {
|
|
// //rules.AllowRead(Dbid, "<Role(s)>");
|
|
// }
|
|
// public new void AddInstanceAuthorizationRules(Csla.Security.AuthorizationRules rules)
|
|
// {
|
|
// //rules.AllowInstanceRead(Dbid, "<Role(s)>");
|
|
// }
|
|
// public new void AddValidationRules(Csla.Validation.ValidationRules rules)
|
|
// {
|
|
// rules.AddRule(
|
|
// Csla.Validation.CommonRules.StringMaxLength,
|
|
// new Csla.Validation.CommonRules.MaxLengthRuleArgs("Name", 100));
|
|
// }
|
|
// public new void AddInstanceValidationRules(Csla.Validation.ValidationRules rules)
|
|
// {
|
|
// rules.AddInstanceRule(/* Instance Validation Rule */);
|
|
// }
|
|
// }
|
|
// }
|
|
//}
|