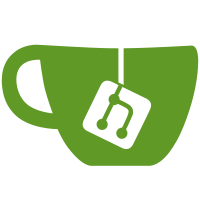
If the selected Library Document is out-of-range throw a specific error Don't fail if the user clicks on open space in the BookMark list Added property to provide a right margin (RTBMargin) between the RichTextBox and the StepItem
749 lines
22 KiB
C#
749 lines
22 KiB
C#
// ========================================================================
|
|
// Copyright 2007 - Volian Enterprises, Inc. All rights reserved.
|
|
// Volian Enterprises - Proprietary Information - DO NOT COPY OR DISTRIBUTE
|
|
// ------------------------------------------------------------------------
|
|
// $Workfile: $ $Revision: $
|
|
// $Author: $ $Date: $
|
|
//
|
|
// $History: $
|
|
// ========================================================================
|
|
|
|
using System;
|
|
using System.Data;
|
|
using System.Data.SqlClient;
|
|
using Csla;
|
|
using Csla.Data;
|
|
using System.Configuration;
|
|
using System.IO;
|
|
using System.ComponentModel;
|
|
using System.Collections.Generic;
|
|
using Csla.Validation;
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
/// <summary>
|
|
/// GroupMembership Generated by MyGeneration using the CSLA Object Mapping template
|
|
/// </summary>
|
|
[Serializable()]
|
|
[TypeConverter(typeof(GroupMembershipConverter))]
|
|
public partial class GroupMembership : BusinessBase<GroupMembership>, IVEHasBrokenRules
|
|
{
|
|
#region Log4Net
|
|
private static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
#endregion
|
|
#region Business Methods
|
|
private string _ErrorMessage = string.Empty;
|
|
public string ErrorMessage
|
|
{
|
|
get { return _ErrorMessage; }
|
|
}
|
|
private int _UGID;
|
|
[System.ComponentModel.DataObjectField(true, true)]
|
|
public int UGID
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("UGID", true);
|
|
if (_MyMembership != null) _UGID = _MyMembership.UGID;
|
|
return _UGID;
|
|
}
|
|
}
|
|
private Membership _MyMembership;
|
|
[System.ComponentModel.DataObjectField(true, true)]
|
|
public Membership MyMembership
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("MyMembership", true);
|
|
if (_MyMembership == null && _UGID != 0) _MyMembership = Membership.Get(_UGID);
|
|
return _MyMembership;
|
|
}
|
|
}
|
|
private int _UID;
|
|
public int UID
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("UID", true);
|
|
if (_MyUser != null) _UID = _MyUser.UID;
|
|
return _UID;
|
|
}
|
|
}
|
|
private User _MyUser;
|
|
public User MyUser
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("MyUser", true);
|
|
if (_MyUser == null && _UID != 0) _MyUser = User.Get(_UID);
|
|
return _MyUser;
|
|
}
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
set
|
|
{
|
|
CanWriteProperty("MyUser", true);
|
|
if (_MyUser != value)
|
|
{
|
|
_MyUser = value;
|
|
_UID = value.UID;// Update underlying data field
|
|
PropertyHasChanged();
|
|
}
|
|
}
|
|
}
|
|
private string _StartDate = string.Empty;
|
|
public string StartDate
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("StartDate", true);
|
|
return _StartDate;
|
|
}
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
set
|
|
{
|
|
CanWriteProperty("StartDate", true);
|
|
if (value == null) value = string.Empty;
|
|
_StartDate = value;
|
|
try
|
|
{
|
|
SmartDate tmp = new SmartDate(value);
|
|
if (_StartDate != tmp.ToString())
|
|
{
|
|
_StartDate = tmp.ToString();
|
|
// CSLATODO: Any Cross Property Validation
|
|
}
|
|
}
|
|
catch
|
|
{
|
|
}
|
|
PropertyHasChanged();
|
|
}
|
|
}
|
|
private string _EndDate = string.Empty;
|
|
public string EndDate
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("EndDate", true);
|
|
return _EndDate;
|
|
}
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
set
|
|
{
|
|
CanWriteProperty("EndDate", true);
|
|
if (value == null) value = string.Empty;
|
|
_EndDate = value;
|
|
try
|
|
{
|
|
SmartDate tmp = new SmartDate(value);
|
|
if (_EndDate != tmp.ToString())
|
|
{
|
|
_EndDate = tmp.ToString();
|
|
// CSLATODO: Any Cross Property Validation
|
|
}
|
|
}
|
|
catch
|
|
{
|
|
}
|
|
PropertyHasChanged();
|
|
}
|
|
}
|
|
private string _Config = string.Empty;
|
|
public string Config
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("Config", true);
|
|
return _Config;
|
|
}
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
set
|
|
{
|
|
CanWriteProperty("Config", true);
|
|
if (value == null) value = string.Empty;
|
|
if (_Config != value)
|
|
{
|
|
_Config = value;
|
|
PropertyHasChanged();
|
|
}
|
|
}
|
|
}
|
|
private DateTime _DTS = new DateTime();
|
|
public DateTime DTS
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("DTS", true);
|
|
return _DTS;
|
|
}
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
set
|
|
{
|
|
CanWriteProperty("DTS", true);
|
|
if (_DTS != value)
|
|
{
|
|
_DTS = value;
|
|
PropertyHasChanged();
|
|
}
|
|
}
|
|
}
|
|
private string _UsrID = string.Empty;
|
|
public string UsrID
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("UsrID", true);
|
|
return _UsrID;
|
|
}
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
set
|
|
{
|
|
CanWriteProperty("UsrID", true);
|
|
if (value == null) value = string.Empty;
|
|
if (_UsrID != value)
|
|
{
|
|
_UsrID = value;
|
|
PropertyHasChanged();
|
|
}
|
|
}
|
|
}
|
|
private byte[] _LastChanged = new byte[8];//timestamp
|
|
private string _User_UserID = string.Empty;
|
|
public string User_UserID
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("User_UserID", true);
|
|
return _User_UserID;
|
|
}
|
|
}
|
|
private string _User_FirstName = string.Empty;
|
|
public string User_FirstName
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("User_FirstName", true);
|
|
return _User_FirstName;
|
|
}
|
|
}
|
|
private string _User_MiddleName = string.Empty;
|
|
public string User_MiddleName
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("User_MiddleName", true);
|
|
return _User_MiddleName;
|
|
}
|
|
}
|
|
private string _User_LastName = string.Empty;
|
|
public string User_LastName
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("User_LastName", true);
|
|
return _User_LastName;
|
|
}
|
|
}
|
|
private string _User_Suffix = string.Empty;
|
|
public string User_Suffix
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("User_Suffix", true);
|
|
return _User_Suffix;
|
|
}
|
|
}
|
|
private string _User_CourtesyTitle = string.Empty;
|
|
public string User_CourtesyTitle
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("User_CourtesyTitle", true);
|
|
return _User_CourtesyTitle;
|
|
}
|
|
}
|
|
private string _User_PhoneNumber = string.Empty;
|
|
public string User_PhoneNumber
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("User_PhoneNumber", true);
|
|
return _User_PhoneNumber;
|
|
}
|
|
}
|
|
private string _User_CFGName = string.Empty;
|
|
public string User_CFGName
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("User_CFGName", true);
|
|
return _User_CFGName;
|
|
}
|
|
}
|
|
private string _User_UserLogin = string.Empty;
|
|
public string User_UserLogin
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("User_UserLogin", true);
|
|
return _User_UserLogin;
|
|
}
|
|
}
|
|
private string _User_UserName = string.Empty;
|
|
public string User_UserName
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("User_UserName", true);
|
|
return _User_UserName;
|
|
}
|
|
}
|
|
private string _User_Config = string.Empty;
|
|
public string User_Config
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("User_Config", true);
|
|
return _User_Config;
|
|
}
|
|
}
|
|
private DateTime _User_DTS = new DateTime();
|
|
public DateTime User_DTS
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("User_DTS", true);
|
|
return _User_DTS;
|
|
}
|
|
}
|
|
private string _User_UsrID = string.Empty;
|
|
public string User_UsrID
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("User_UsrID", true);
|
|
return _User_UsrID;
|
|
}
|
|
}
|
|
// CSLATODO: Check GroupMembership.GetIdValue to assure that the ID returned is unique
|
|
/// <summary>
|
|
/// Overrides Base GetIdValue - Used internally by CSLA to determine equality
|
|
/// </summary>
|
|
/// <returns>A Unique ID for the current GroupMembership</returns>
|
|
protected override object GetIdValue()
|
|
{
|
|
return MyGroupMembershipUnique; // Absolutely Unique ID
|
|
}
|
|
// CSLATODO: Replace base GroupMembership.ToString function as necessary
|
|
/// <summary>
|
|
/// Overrides Base ToString
|
|
/// </summary>
|
|
/// <returns>A string representation of current GroupMembership</returns>
|
|
//public override string ToString()
|
|
//{
|
|
// return base.ToString();
|
|
//}
|
|
public override bool IsDirty
|
|
{
|
|
get
|
|
{
|
|
if ( base.IsDirty )
|
|
return true;
|
|
return IsDirtyList(new List<object>());
|
|
}
|
|
}
|
|
public bool IsDirtyList(List<object> list)
|
|
{
|
|
if (base.IsDirty || list.Contains(this))
|
|
return base.IsDirty;
|
|
list.Add(this);
|
|
return base.IsDirty || (_MyUser == null ? false : _MyUser.IsDirtyList(list));
|
|
}
|
|
public override bool IsValid
|
|
{
|
|
get { return IsValidList(new List<object>()); }
|
|
}
|
|
public bool IsValidList(List<object> list)
|
|
{
|
|
if(list.Contains(this))
|
|
return (IsNew && !IsDirty) ? true : base.IsValid;
|
|
list.Add(this);
|
|
return ((IsNew && !IsDirty) ? true : base.IsValid) && (_MyUser == null ? true : _MyUser.IsValidList(list));
|
|
}
|
|
#endregion
|
|
#region ValidationRules
|
|
[NonSerialized]
|
|
private bool _CheckingBrokenRules = false;
|
|
public IVEHasBrokenRules HasBrokenRules
|
|
{
|
|
get
|
|
{
|
|
if (_CheckingBrokenRules) return null;
|
|
if (BrokenRulesCollection.Count > 0) return this;
|
|
try
|
|
{
|
|
_CheckingBrokenRules = true;
|
|
IVEHasBrokenRules hasBrokenRules = null;
|
|
if (_MyUser != null && (hasBrokenRules = _MyUser.HasBrokenRules) != null) return hasBrokenRules;
|
|
return hasBrokenRules;
|
|
}
|
|
finally
|
|
{
|
|
_CheckingBrokenRules = false;
|
|
}
|
|
}
|
|
}
|
|
public BrokenRulesCollection BrokenRules
|
|
{
|
|
get
|
|
{
|
|
IVEHasBrokenRules hasBrokenRules = HasBrokenRules;
|
|
if (this.Equals(hasBrokenRules)) return BrokenRulesCollection;
|
|
return (hasBrokenRules != null ? hasBrokenRules.BrokenRules : null);
|
|
}
|
|
}
|
|
protected override void AddBusinessRules()
|
|
{
|
|
ValidationRules.AddRule<GroupMembership>(MyUserRequired, "MyUser");
|
|
ValidationRules.AddRule(
|
|
Csla.Validation.CommonRules.StringRequired, "StartDate");
|
|
ValidationRules.AddRule<GroupMembership>(StartDateValid, "StartDate");
|
|
ValidationRules.AddRule<GroupMembership>(EndDateValid, "EndDate");
|
|
ValidationRules.AddRule(
|
|
Csla.Validation.CommonRules.StringMaxLength,
|
|
new Csla.Validation.CommonRules.MaxLengthRuleArgs("Config", 1073741823));
|
|
ValidationRules.AddRule(
|
|
Csla.Validation.CommonRules.StringRequired, "UsrID");
|
|
ValidationRules.AddRule(
|
|
Csla.Validation.CommonRules.StringMaxLength,
|
|
new Csla.Validation.CommonRules.MaxLengthRuleArgs("UsrID", 100));
|
|
// CSLATODO: Add other validation rules
|
|
}
|
|
private static bool StartDateValid(GroupMembership target, Csla.Validation.RuleArgs e)
|
|
{
|
|
try
|
|
{
|
|
DateTime tmp = SmartDate.StringToDate(target._StartDate);
|
|
}
|
|
catch
|
|
{
|
|
e.Description = "Invalid Date";
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
private static bool EndDateValid(GroupMembership target, Csla.Validation.RuleArgs e)
|
|
{
|
|
try
|
|
{
|
|
DateTime tmp = SmartDate.StringToDate(target._EndDate);
|
|
}
|
|
catch
|
|
{
|
|
e.Description = "Invalid Date";
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
private static bool MyUserRequired(GroupMembership target, Csla.Validation.RuleArgs e)
|
|
{
|
|
if (target._UID == 0 && target._MyUser == null) // Required field missing
|
|
{
|
|
e.Description = "Required";
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
// Sample data comparison validation rule
|
|
//private bool StartDateGTEndDate(object target, Csla.Validation.RuleArgs e)
|
|
//{
|
|
// if (_started > _ended)
|
|
// {
|
|
// e.Description = "Start date can't be after end date";
|
|
// return false;
|
|
// }
|
|
// else
|
|
// return true;
|
|
//}
|
|
#endregion
|
|
#region Authorization Rules
|
|
protected override void AddAuthorizationRules()
|
|
{
|
|
//CSLATODO: Who can read/write which fields
|
|
//AuthorizationRules.AllowRead(UGID, "<Role(s)>");
|
|
//AuthorizationRules.AllowRead(UID, "<Role(s)>");
|
|
//AuthorizationRules.AllowWrite(UID, "<Role(s)>");
|
|
//AuthorizationRules.AllowRead(StartDate, "<Role(s)>");
|
|
//AuthorizationRules.AllowWrite(StartDate, "<Role(s)>");
|
|
//AuthorizationRules.AllowRead(EndDate, "<Role(s)>");
|
|
//AuthorizationRules.AllowWrite(EndDate, "<Role(s)>");
|
|
//AuthorizationRules.AllowRead(Config, "<Role(s)>");
|
|
//AuthorizationRules.AllowWrite(Config, "<Role(s)>");
|
|
//AuthorizationRules.AllowRead(DTS, "<Role(s)>");
|
|
//AuthorizationRules.AllowWrite(DTS, "<Role(s)>");
|
|
//AuthorizationRules.AllowRead(UsrID, "<Role(s)>");
|
|
//AuthorizationRules.AllowWrite(UsrID, "<Role(s)>");
|
|
}
|
|
public static bool CanAddObject()
|
|
{
|
|
// CSLATODO: Can Add Authorization
|
|
//return Csla.ApplicationContext.User.IsInRole("ProjectManager");
|
|
return true;
|
|
}
|
|
public static bool CanGetObject()
|
|
{
|
|
// CSLATODO: CanGet Authorization
|
|
return true;
|
|
}
|
|
public static bool CanDeleteObject()
|
|
{
|
|
// CSLATODO: CanDelete Authorization
|
|
//bool result = false;
|
|
//if (Csla.ApplicationContext.User.IsInRole("ProjectManager"))result = true;
|
|
//if (Csla.ApplicationContext.User.IsInRole("Administrator"))result = true;
|
|
//return result;
|
|
return true;
|
|
}
|
|
public static bool CanEditObject()
|
|
{
|
|
// CSLATODO: CanEdit Authorization
|
|
//return Csla.ApplicationContext.User.IsInRole("ProjectManager");
|
|
return true;
|
|
}
|
|
#endregion
|
|
#region Factory Methods
|
|
public int CurrentEditLevel
|
|
{ get { return EditLevel; } }
|
|
private static int _GroupMembershipUnique = 0;
|
|
private static int GroupMembershipUnique
|
|
{ get { return ++_GroupMembershipUnique; } }
|
|
private int _MyGroupMembershipUnique = GroupMembershipUnique;
|
|
public int MyGroupMembershipUnique // Absolutely Unique ID - Editable FK
|
|
{ get { return _MyGroupMembershipUnique; } }
|
|
internal static GroupMembership New(User myUser)
|
|
{
|
|
return new GroupMembership(myUser);
|
|
}
|
|
internal static GroupMembership Get(SafeDataReader dr)
|
|
{
|
|
return new GroupMembership(dr);
|
|
}
|
|
public GroupMembership()
|
|
{
|
|
MarkAsChild();
|
|
_UGID = Membership.NextUGID;
|
|
_StartDate = _GroupMembershipExtension.DefaultStartDate;
|
|
_DTS = _GroupMembershipExtension.DefaultDTS;
|
|
_UsrID = _GroupMembershipExtension.DefaultUsrID;
|
|
ValidationRules.CheckRules();
|
|
}
|
|
private GroupMembership(User myUser)
|
|
{
|
|
MarkAsChild();
|
|
// CSLATODO: Add any initialization & defaults
|
|
_UGID = Membership.NextUGID;
|
|
_StartDate = _GroupMembershipExtension.DefaultStartDate;
|
|
_DTS = _GroupMembershipExtension.DefaultDTS;
|
|
_UsrID = _GroupMembershipExtension.DefaultUsrID;
|
|
_MyUser = myUser;
|
|
ValidationRules.CheckRules();
|
|
}
|
|
internal GroupMembership(SafeDataReader dr)
|
|
{
|
|
MarkAsChild();
|
|
Fetch(dr);
|
|
}
|
|
#endregion
|
|
#region Data Access Portal
|
|
private void Fetch(SafeDataReader dr)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] GroupMembership.FetchDR", GetHashCode());
|
|
try
|
|
{
|
|
_UGID = dr.GetInt32("UGID");
|
|
_UID = dr.GetInt32("UID");
|
|
_StartDate = dr.GetSmartDate("StartDate").Text;
|
|
_EndDate = dr.GetSmartDate("EndDate").Text;
|
|
_Config = dr.GetString("Config");
|
|
_DTS = dr.GetDateTime("DTS");
|
|
_UsrID = dr.GetString("UsrID");
|
|
dr.GetBytes("LastChanged", 0, _LastChanged, 0, 8);
|
|
_User_UserID = dr.GetString("User_UserID");
|
|
_User_FirstName = dr.GetString("User_FirstName");
|
|
_User_MiddleName = dr.GetString("User_MiddleName");
|
|
_User_LastName = dr.GetString("User_LastName");
|
|
_User_Suffix = dr.GetString("User_Suffix");
|
|
_User_CourtesyTitle = dr.GetString("User_CourtesyTitle");
|
|
_User_PhoneNumber = dr.GetString("User_PhoneNumber");
|
|
_User_CFGName = dr.GetString("User_CFGName");
|
|
_User_UserLogin = dr.GetString("User_UserLogin");
|
|
_User_UserName = dr.GetString("User_UserName");
|
|
_User_Config = dr.GetString("User_Config");
|
|
_User_DTS = dr.GetDateTime("User_DTS");
|
|
_User_UsrID = dr.GetString("User_UsrID");
|
|
}
|
|
catch (Exception ex) // FKItem Fetch
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("GroupMembership.FetchDR", ex);
|
|
throw new DbCslaException("GroupMembership.Fetch", ex);
|
|
}
|
|
MarkOld();
|
|
}
|
|
internal void Insert(Group myGroup)
|
|
{
|
|
// if we're not dirty then don't update the database
|
|
if (!this.IsDirty) return;
|
|
SqlConnection cn = (SqlConnection)ApplicationContext.LocalContext["cn"];
|
|
_LastChanged = Membership.Add(cn, ref _UGID, _MyUser, myGroup, new SmartDate(_StartDate), new SmartDate(_EndDate), _Config, _DTS, _UsrID);
|
|
MarkOld();
|
|
}
|
|
internal void Update(Group myGroup)
|
|
{
|
|
// if we're not dirty then don't update the database
|
|
if (!this.IsDirty) return;
|
|
SqlConnection cn = (SqlConnection)ApplicationContext.LocalContext["cn"];
|
|
_LastChanged = Membership.Update(cn, ref _UGID, _UID, myGroup.GID, new SmartDate(_StartDate), new SmartDate(_EndDate), _Config, _DTS, _UsrID, ref _LastChanged);
|
|
MarkOld();
|
|
}
|
|
internal void DeleteSelf(Group myGroup)
|
|
{
|
|
// if we're not dirty then don't update the database
|
|
if (!this.IsDirty) return;
|
|
// if we're new then don't update the database
|
|
if (this.IsNew) return;
|
|
SqlConnection cn = (SqlConnection)ApplicationContext.LocalContext["cn"];
|
|
Membership.Remove(cn, _UGID);
|
|
MarkNew();
|
|
}
|
|
#endregion
|
|
// Standard Default Code
|
|
#region extension
|
|
GroupMembershipExtension _GroupMembershipExtension = new GroupMembershipExtension();
|
|
[Serializable()]
|
|
partial class GroupMembershipExtension : extensionBase
|
|
{
|
|
}
|
|
[Serializable()]
|
|
class extensionBase
|
|
{
|
|
// Default Values
|
|
public virtual string DefaultStartDate
|
|
{
|
|
get { return DateTime.Now.ToShortDateString(); }
|
|
}
|
|
public virtual DateTime DefaultDTS
|
|
{
|
|
get { return DateTime.Now; }
|
|
}
|
|
public virtual string DefaultUsrID
|
|
{
|
|
get { return Environment.UserName.ToUpper(); }
|
|
}
|
|
// Authorization Rules
|
|
public virtual void AddAuthorizationRules(Csla.Security.AuthorizationRules rules)
|
|
{
|
|
// Needs to be overriden to add new authorization rules
|
|
}
|
|
// Instance Authorization Rules
|
|
public virtual void AddInstanceAuthorizationRules(Csla.Security.AuthorizationRules rules)
|
|
{
|
|
// Needs to be overriden to add new authorization rules
|
|
}
|
|
// Validation Rules
|
|
public virtual void AddValidationRules(Csla.Validation.ValidationRules rules)
|
|
{
|
|
// Needs to be overriden to add new validation rules
|
|
}
|
|
// InstanceValidation Rules
|
|
public virtual void AddInstanceValidationRules(Csla.Validation.ValidationRules rules)
|
|
{
|
|
// Needs to be overriden to add new validation rules
|
|
}
|
|
}
|
|
#endregion
|
|
} // Class
|
|
#region Converter
|
|
internal class GroupMembershipConverter : ExpandableObjectConverter
|
|
{
|
|
public override object ConvertTo(ITypeDescriptorContext context, System.Globalization.CultureInfo culture, object value, Type destType)
|
|
{
|
|
if (destType == typeof(string) && value is GroupMembership)
|
|
{
|
|
// Return the ToString value
|
|
return ((GroupMembership)value).ToString();
|
|
}
|
|
return base.ConvertTo(context, culture, value, destType);
|
|
}
|
|
}
|
|
#endregion
|
|
} // Namespace
|
|
|
|
|
|
//// The following is a sample Extension File. You can use it to create GroupMembershipExt.cs
|
|
//using System;
|
|
//using System.Collections.Generic;
|
|
//using System.Text;
|
|
//using Csla;
|
|
|
|
//namespace VEPROMS.CSLA.Library
|
|
//{
|
|
// public partial class GroupMembership
|
|
// {
|
|
// partial class GroupMembershipExtension : extensionBase
|
|
// {
|
|
// // CSLATODO: Override automatic defaults
|
|
// public virtual SmartDate DefaultStartDate
|
|
// {
|
|
// get { return DateTime.Now.ToShortDateString(); }
|
|
// }
|
|
// public virtual DateTime DefaultDTS
|
|
// {
|
|
// get { return DateTime.Now; }
|
|
// }
|
|
// public virtual string DefaultUsrID
|
|
// {
|
|
// get { return Environment.UserName.ToUpper(); }
|
|
// }
|
|
// public new void AddAuthorizationRules(Csla.Security.AuthorizationRules rules)
|
|
// {
|
|
// //rules.AllowRead(Dbid, "<Role(s)>");
|
|
// }
|
|
// public new void AddInstanceAuthorizationRules(Csla.Security.AuthorizationRules rules)
|
|
// {
|
|
// //rules.AllowInstanceRead(Dbid, "<Role(s)>");
|
|
// }
|
|
// public new void AddValidationRules(Csla.Validation.ValidationRules rules)
|
|
// {
|
|
// rules.AddRule(
|
|
// Csla.Validation.CommonRules.StringMaxLength,
|
|
// new Csla.Validation.CommonRules.MaxLengthRuleArgs("Name", 100));
|
|
// }
|
|
// public new void AddInstanceValidationRules(Csla.Validation.ValidationRules rules)
|
|
// {
|
|
// rules.AddInstanceRule(/* Instance Validation Rule */);
|
|
// }
|
|
// }
|
|
// }
|
|
//}
|