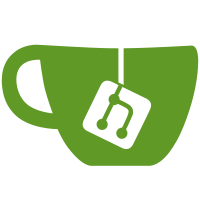
If the selected Library Document is out-of-range throw a specific error Don't fail if the user clicks on open space in the BookMark list Added property to provide a right margin (RTBMargin) between the RichTextBox and the StepItem
1235 lines
39 KiB
C#
1235 lines
39 KiB
C#
// ========================================================================
|
|
// Copyright 2007 - Volian Enterprises, Inc. All rights reserved.
|
|
// Volian Enterprises - Proprietary Information - DO NOT COPY OR DISTRIBUTE
|
|
// ------------------------------------------------------------------------
|
|
// $Workfile: $ $Revision: $
|
|
// $Author: $ $Date: $
|
|
//
|
|
// $History: $
|
|
// ========================================================================
|
|
|
|
using System;
|
|
using System.Data;
|
|
using System.Data.SqlClient;
|
|
using Csla;
|
|
using Csla.Data;
|
|
using System.Configuration;
|
|
using System.IO;
|
|
using System.ComponentModel;
|
|
using System.Collections.Generic;
|
|
using Csla.Validation;
|
|
namespace VEPROMS.CSLA.Library
|
|
{
|
|
/// <summary>
|
|
/// Assignment Generated by MyGeneration using the CSLA Object Mapping template
|
|
/// </summary>
|
|
[Serializable()]
|
|
[TypeConverter(typeof(AssignmentConverter))]
|
|
public partial class Assignment : BusinessBase<Assignment>, IDisposable, IVEHasBrokenRules
|
|
{
|
|
#region Log4Net
|
|
private static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
#endregion
|
|
#region Refresh
|
|
private List<Assignment> _RefreshAssignments = new List<Assignment>();
|
|
private void AddToRefreshList(List<Assignment> refreshAssignments)
|
|
{
|
|
if (IsDirty)
|
|
refreshAssignments.Add(this);
|
|
}
|
|
private void BuildRefreshList()
|
|
{
|
|
_RefreshAssignments = new List<Assignment>();
|
|
AddToRefreshList(_RefreshAssignments);
|
|
}
|
|
private void ProcessRefreshList()
|
|
{
|
|
foreach (Assignment tmp in _RefreshAssignments)
|
|
{
|
|
AssignmentInfo.Refresh(tmp);
|
|
if (tmp._MyFolder != null) FolderInfo.Refresh(tmp._MyFolder);
|
|
if (tmp._MyGroup != null) GroupInfo.Refresh(tmp._MyGroup);
|
|
if (tmp._MyRole != null) RoleInfo.Refresh(tmp._MyRole);
|
|
}
|
|
}
|
|
#endregion
|
|
#region Collection
|
|
private static List<Assignment> _CacheList = new List<Assignment>();
|
|
protected static void AddToCache(Assignment assignment)
|
|
{
|
|
if (!_CacheList.Contains(assignment)) _CacheList.Add(assignment); // In AddToCache
|
|
}
|
|
protected static void RemoveFromCache(Assignment assignment)
|
|
{
|
|
while (_CacheList.Contains(assignment)) _CacheList.Remove(assignment); // In RemoveFromCache
|
|
}
|
|
private static Dictionary<string, List<Assignment>> _CacheByPrimaryKey = new Dictionary<string, List<Assignment>>();
|
|
private static void ConvertListToDictionary()
|
|
{
|
|
while (_CacheList.Count > 0) // Move Assignment(s) from temporary _CacheList to _CacheByPrimaryKey
|
|
{
|
|
Assignment tmp = _CacheList[0]; // Get the first Assignment
|
|
string pKey = tmp.AID.ToString();
|
|
if (!_CacheByPrimaryKey.ContainsKey(pKey))
|
|
{
|
|
_CacheByPrimaryKey[pKey] = new List<Assignment>(); // Add new list for PrimaryKey
|
|
}
|
|
_CacheByPrimaryKey[pKey].Add(tmp); // Add to Primary Key list
|
|
_CacheList.RemoveAt(0); // Remove the first Assignment
|
|
}
|
|
}
|
|
protected static Assignment GetCachedByPrimaryKey(int aid)
|
|
{
|
|
ConvertListToDictionary();
|
|
string key = aid.ToString();
|
|
if (_CacheByPrimaryKey.ContainsKey(key)) return _CacheByPrimaryKey[key][0];
|
|
return null;
|
|
}
|
|
#endregion
|
|
#region Business Methods
|
|
private string _ErrorMessage = string.Empty;
|
|
public string ErrorMessage
|
|
{
|
|
get { return _ErrorMessage; }
|
|
}
|
|
private static int _nextAID = -1;
|
|
public static int NextAID
|
|
{
|
|
get { return _nextAID--; }
|
|
}
|
|
private int _AID;
|
|
[System.ComponentModel.DataObjectField(true, true)]
|
|
public int AID
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("AID", true);
|
|
return _AID;
|
|
}
|
|
}
|
|
private int _GID;
|
|
public int GID
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("GID", true);
|
|
if (_MyGroup != null) _GID = _MyGroup.GID;
|
|
return _GID;
|
|
}
|
|
}
|
|
private Group _MyGroup;
|
|
public Group MyGroup
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("MyGroup", true);
|
|
if (_MyGroup == null && _GID != 0) _MyGroup = Group.Get(_GID);
|
|
return _MyGroup;
|
|
}
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
set
|
|
{
|
|
CanWriteProperty("MyGroup", true);
|
|
if (_MyGroup != value)
|
|
{
|
|
_MyGroup = value;
|
|
_GID = value.GID;// Update underlying data field
|
|
PropertyHasChanged();
|
|
}
|
|
}
|
|
}
|
|
private int _RID;
|
|
public int RID
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("RID", true);
|
|
if (_MyRole != null) _RID = _MyRole.RID;
|
|
return _RID;
|
|
}
|
|
}
|
|
private Role _MyRole;
|
|
public Role MyRole
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("MyRole", true);
|
|
if (_MyRole == null && _RID != 0) _MyRole = Role.Get(_RID);
|
|
return _MyRole;
|
|
}
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
set
|
|
{
|
|
CanWriteProperty("MyRole", true);
|
|
if (_MyRole != value)
|
|
{
|
|
_MyRole = value;
|
|
_RID = value.RID;// Update underlying data field
|
|
PropertyHasChanged();
|
|
}
|
|
}
|
|
}
|
|
private int _FolderID;
|
|
public int FolderID
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("FolderID", true);
|
|
if (_MyFolder != null) _FolderID = _MyFolder.FolderID;
|
|
return _FolderID;
|
|
}
|
|
}
|
|
private Folder _MyFolder;
|
|
public Folder MyFolder
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("MyFolder", true);
|
|
if (_MyFolder == null && _FolderID != 0) _MyFolder = Folder.Get(_FolderID);
|
|
return _MyFolder;
|
|
}
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
set
|
|
{
|
|
CanWriteProperty("MyFolder", true);
|
|
if (_MyFolder != value)
|
|
{
|
|
_MyFolder = value;
|
|
_FolderID = value.FolderID;// Update underlying data field
|
|
PropertyHasChanged();
|
|
}
|
|
}
|
|
}
|
|
private string _StartDate = string.Empty;
|
|
public string StartDate
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("StartDate", true);
|
|
return _StartDate;
|
|
}
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
set
|
|
{
|
|
CanWriteProperty("StartDate", true);
|
|
if (value == null) value = string.Empty;
|
|
_StartDate = value;
|
|
try
|
|
{
|
|
SmartDate tmp = new SmartDate(value);
|
|
if (_StartDate != tmp.ToString())
|
|
{
|
|
_StartDate = tmp.ToString();
|
|
// CSLATODO: Any Cross Property Validation
|
|
}
|
|
}
|
|
catch
|
|
{
|
|
}
|
|
PropertyHasChanged();
|
|
}
|
|
}
|
|
private string _EndDate = string.Empty;
|
|
public string EndDate
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("EndDate", true);
|
|
return _EndDate;
|
|
}
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
set
|
|
{
|
|
CanWriteProperty("EndDate", true);
|
|
if (value == null) value = string.Empty;
|
|
_EndDate = value;
|
|
try
|
|
{
|
|
SmartDate tmp = new SmartDate(value);
|
|
if (_EndDate != tmp.ToString())
|
|
{
|
|
_EndDate = tmp.ToString();
|
|
// CSLATODO: Any Cross Property Validation
|
|
}
|
|
}
|
|
catch
|
|
{
|
|
}
|
|
PropertyHasChanged();
|
|
}
|
|
}
|
|
private DateTime _DTS = new DateTime();
|
|
public DateTime DTS
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("DTS", true);
|
|
return _DTS;
|
|
}
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
set
|
|
{
|
|
CanWriteProperty("DTS", true);
|
|
if (_DTS != value)
|
|
{
|
|
_DTS = value;
|
|
PropertyHasChanged();
|
|
}
|
|
}
|
|
}
|
|
private string _UsrID = string.Empty;
|
|
public string UsrID
|
|
{
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
get
|
|
{
|
|
CanReadProperty("UsrID", true);
|
|
return _UsrID;
|
|
}
|
|
[System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)]
|
|
set
|
|
{
|
|
CanWriteProperty("UsrID", true);
|
|
if (value == null) value = string.Empty;
|
|
if (_UsrID != value)
|
|
{
|
|
_UsrID = value;
|
|
PropertyHasChanged();
|
|
}
|
|
}
|
|
}
|
|
private byte[] _LastChanged = new byte[8];//timestamp
|
|
public override bool IsDirty
|
|
{
|
|
get
|
|
{
|
|
if ( base.IsDirty )
|
|
return true;
|
|
return IsDirtyList(new List<object>());
|
|
}
|
|
}
|
|
public bool IsDirtyList(List<object> list)
|
|
{
|
|
if (base.IsDirty || list.Contains(this))
|
|
return base.IsDirty;
|
|
list.Add(this);
|
|
return base.IsDirty || (_MyFolder == null ? false : _MyFolder.IsDirtyList(list)) || (_MyGroup == null ? false : _MyGroup.IsDirtyList(list)) || (_MyRole == null ? false : _MyRole.IsDirtyList(list));
|
|
}
|
|
public override bool IsValid
|
|
{
|
|
get { return IsValidList(new List<object>()); }
|
|
}
|
|
public bool IsValidList(List<object> list)
|
|
{
|
|
if(list.Contains(this))
|
|
return (IsNew && !IsDirty) ? true : base.IsValid;
|
|
list.Add(this);
|
|
return ((IsNew && !IsDirty) ? true : base.IsValid) && (_MyFolder == null ? true : _MyFolder.IsValidList(list)) && (_MyGroup == null ? true : _MyGroup.IsValidList(list)) && (_MyRole == null ? true : _MyRole.IsValidList(list));
|
|
}
|
|
// CSLATODO: Replace base Assignment.ToString function as necessary
|
|
/// <summary>
|
|
/// Overrides Base ToString
|
|
/// </summary>
|
|
/// <returns>A string representation of current Assignment</returns>
|
|
//public override string ToString()
|
|
//{
|
|
// return base.ToString();
|
|
//}
|
|
// CSLATODO: Check Assignment.GetIdValue to assure that the ID returned is unique
|
|
/// <summary>
|
|
/// Overrides Base GetIdValue - Used internally by CSLA to determine equality
|
|
/// </summary>
|
|
/// <returns>A Unique ID for the current Assignment</returns>
|
|
protected override object GetIdValue()
|
|
{
|
|
return MyAssignmentUnique; // Absolutely Unique ID
|
|
}
|
|
#endregion
|
|
#region ValidationRules
|
|
[NonSerialized]
|
|
private bool _CheckingBrokenRules = false;
|
|
public IVEHasBrokenRules HasBrokenRules
|
|
{
|
|
get
|
|
{
|
|
if (_CheckingBrokenRules) return null;
|
|
if ((IsDirty || !IsNew) && BrokenRulesCollection.Count > 0) return this;
|
|
try
|
|
{
|
|
_CheckingBrokenRules = true;
|
|
IVEHasBrokenRules hasBrokenRules = null;
|
|
if (_MyGroup != null && (hasBrokenRules = _MyGroup.HasBrokenRules) != null) return hasBrokenRules;
|
|
if (_MyRole != null && (hasBrokenRules = _MyRole.HasBrokenRules) != null) return hasBrokenRules;
|
|
if (_MyFolder != null && (hasBrokenRules = _MyFolder.HasBrokenRules) != null) return hasBrokenRules;
|
|
return hasBrokenRules;
|
|
}
|
|
finally
|
|
{
|
|
_CheckingBrokenRules = false;
|
|
}
|
|
}
|
|
}
|
|
public BrokenRulesCollection BrokenRules
|
|
{
|
|
get
|
|
{
|
|
IVEHasBrokenRules hasBrokenRules = HasBrokenRules;
|
|
if (this.Equals(hasBrokenRules)) return BrokenRulesCollection;
|
|
return (hasBrokenRules != null ? hasBrokenRules.BrokenRules : null);
|
|
}
|
|
}
|
|
protected override void AddBusinessRules()
|
|
{
|
|
ValidationRules.AddRule<Assignment>(MyGroupRequired, "MyGroup");
|
|
ValidationRules.AddRule<Assignment>(MyRoleRequired, "MyRole");
|
|
ValidationRules.AddRule<Assignment>(MyFolderRequired, "MyFolder");
|
|
ValidationRules.AddRule(
|
|
Csla.Validation.CommonRules.StringRequired, "StartDate");
|
|
ValidationRules.AddRule<Assignment>(StartDateValid, "StartDate");
|
|
ValidationRules.AddRule<Assignment>(EndDateValid, "EndDate");
|
|
ValidationRules.AddRule(
|
|
Csla.Validation.CommonRules.StringRequired, "UsrID");
|
|
ValidationRules.AddRule(
|
|
Csla.Validation.CommonRules.StringMaxLength,
|
|
new Csla.Validation.CommonRules.MaxLengthRuleArgs("UsrID", 100));
|
|
//ValidationRules.AddDependantProperty("x", "y");
|
|
_AssignmentExtension.AddValidationRules(ValidationRules);
|
|
// CSLATODO: Add other validation rules
|
|
}
|
|
protected override void AddInstanceBusinessRules()
|
|
{
|
|
_AssignmentExtension.AddInstanceValidationRules(ValidationRules);
|
|
// CSLATODO: Add other validation rules
|
|
}
|
|
private static bool StartDateValid(Assignment target, Csla.Validation.RuleArgs e)
|
|
{
|
|
try
|
|
{
|
|
DateTime tmp = SmartDate.StringToDate(target._StartDate);
|
|
}
|
|
catch
|
|
{
|
|
e.Description = "Invalid Date";
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
private static bool EndDateValid(Assignment target, Csla.Validation.RuleArgs e)
|
|
{
|
|
try
|
|
{
|
|
DateTime tmp = SmartDate.StringToDate(target._EndDate);
|
|
}
|
|
catch
|
|
{
|
|
e.Description = "Invalid Date";
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
private static bool MyGroupRequired(Assignment target, Csla.Validation.RuleArgs e)
|
|
{
|
|
if (target._GID == 0 && target._MyGroup == null) // Required field missing
|
|
{
|
|
e.Description = "Required";
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
private static bool MyRoleRequired(Assignment target, Csla.Validation.RuleArgs e)
|
|
{
|
|
if (target._RID == 0 && target._MyRole == null) // Required field missing
|
|
{
|
|
e.Description = "Required";
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
private static bool MyFolderRequired(Assignment target, Csla.Validation.RuleArgs e)
|
|
{
|
|
if (target._FolderID == 0 && target._MyFolder == null) // Required field missing
|
|
{
|
|
e.Description = "Required";
|
|
return false;
|
|
}
|
|
return true;
|
|
}
|
|
// Sample data comparison validation rule
|
|
//private bool StartDateGTEndDate(object target, Csla.Validation.RuleArgs e)
|
|
//{
|
|
// if (_started > _ended)
|
|
// {
|
|
// e.Description = "Start date can't be after end date";
|
|
// return false;
|
|
// }
|
|
// else
|
|
// return true;
|
|
//}
|
|
#endregion
|
|
#region Authorization Rules
|
|
protected override void AddAuthorizationRules()
|
|
{
|
|
//CSLATODO: Who can read/write which fields
|
|
//AuthorizationRules.AllowRead(AID, "<Role(s)>");
|
|
//AuthorizationRules.AllowRead(GID, "<Role(s)>");
|
|
//AuthorizationRules.AllowRead(RID, "<Role(s)>");
|
|
//AuthorizationRules.AllowRead(FolderID, "<Role(s)>");
|
|
//AuthorizationRules.AllowRead(StartDate, "<Role(s)>");
|
|
//AuthorizationRules.AllowRead(EndDate, "<Role(s)>");
|
|
//AuthorizationRules.AllowRead(DTS, "<Role(s)>");
|
|
//AuthorizationRules.AllowRead(UsrID, "<Role(s)>");
|
|
//AuthorizationRules.AllowWrite(GID, "<Role(s)>");
|
|
//AuthorizationRules.AllowWrite(RID, "<Role(s)>");
|
|
//AuthorizationRules.AllowWrite(FolderID, "<Role(s)>");
|
|
//AuthorizationRules.AllowWrite(StartDate, "<Role(s)>");
|
|
//AuthorizationRules.AllowWrite(EndDate, "<Role(s)>");
|
|
//AuthorizationRules.AllowWrite(DTS, "<Role(s)>");
|
|
//AuthorizationRules.AllowWrite(UsrID, "<Role(s)>");
|
|
_AssignmentExtension.AddAuthorizationRules(AuthorizationRules);
|
|
}
|
|
protected override void AddInstanceAuthorizationRules()
|
|
{
|
|
//CSLATODO: Who can read/write which fields
|
|
_AssignmentExtension.AddInstanceAuthorizationRules(AuthorizationRules);
|
|
}
|
|
public static bool CanAddObject()
|
|
{
|
|
// CSLATODO: Can Add Authorization
|
|
//return Csla.ApplicationContext.User.IsInRole("ProjectManager");
|
|
return true;
|
|
}
|
|
public static bool CanGetObject()
|
|
{
|
|
// CSLATODO: CanGet Authorization
|
|
return true;
|
|
}
|
|
public static bool CanDeleteObject()
|
|
{
|
|
// CSLATODO: CanDelete Authorization
|
|
//bool result = false;
|
|
//if (Csla.ApplicationContext.User.IsInRole("ProjectManager"))result = true;
|
|
//if (Csla.ApplicationContext.User.IsInRole("Administrator"))result = true;
|
|
//return result;
|
|
return true;
|
|
}
|
|
public static bool CanEditObject()
|
|
{
|
|
// CSLATODO: CanEdit Authorization
|
|
//return Csla.ApplicationContext.User.IsInRole("ProjectManager");
|
|
return true;
|
|
}
|
|
#endregion
|
|
#region Factory Methods
|
|
public int CurrentEditLevel
|
|
{ get { return EditLevel; } }
|
|
private static int _AssignmentUnique = 0;
|
|
protected static int AssignmentUnique
|
|
{ get { return ++_AssignmentUnique; } }
|
|
private int _MyAssignmentUnique = AssignmentUnique;
|
|
public int MyAssignmentUnique // Absolutely Unique ID - Editable
|
|
{ get { return _MyAssignmentUnique; } }
|
|
protected Assignment()
|
|
{/* require use of factory methods */
|
|
AddToCache(this);
|
|
}
|
|
public void Dispose()
|
|
{
|
|
RemoveFromDictionaries();
|
|
}
|
|
private void RemoveFromDictionaries()
|
|
{
|
|
RemoveFromCache(this);
|
|
if (_CacheByPrimaryKey.ContainsKey(AID.ToString()))
|
|
{
|
|
List<Assignment> listAssignment = _CacheByPrimaryKey[AID.ToString()]; // Get the list of items
|
|
while (listAssignment.Contains(this)) listAssignment.Remove(this); // Remove the item from the list
|
|
if (listAssignment.Count == 0) //If there are no items left in the list
|
|
_CacheByPrimaryKey.Remove(AID.ToString()); // remove the list
|
|
}
|
|
}
|
|
public static Assignment New()
|
|
{
|
|
if (!CanAddObject())
|
|
throw new System.Security.SecurityException("User not authorized to add a Assignment");
|
|
try
|
|
{
|
|
return DataPortal.Create<Assignment>();
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on Assignment.New", ex);
|
|
}
|
|
}
|
|
public static Assignment New(Group myGroup, Role myRole, Folder myFolder)
|
|
{
|
|
Assignment tmp = Assignment.New();
|
|
tmp.MyGroup = myGroup;
|
|
tmp.MyRole = myRole;
|
|
tmp.MyFolder = myFolder;
|
|
return tmp;
|
|
}
|
|
public static Assignment New(Group myGroup, Role myRole, Folder myFolder, string startDate, string endDate, DateTime dts, string usrID)
|
|
{
|
|
Assignment tmp = Assignment.New();
|
|
tmp.MyGroup = myGroup;
|
|
tmp.MyRole = myRole;
|
|
tmp.MyFolder = myFolder;
|
|
tmp.StartDate = startDate;
|
|
tmp.EndDate = endDate;
|
|
tmp.DTS = dts;
|
|
tmp.UsrID = usrID;
|
|
return tmp;
|
|
}
|
|
public static Assignment MakeAssignment(Group myGroup, Role myRole, Folder myFolder, string startDate, string endDate, DateTime dts, string usrID)
|
|
{
|
|
Assignment tmp = Assignment.New(myGroup, myRole, myFolder, startDate, endDate, dts, usrID);
|
|
if (tmp.IsSavable)
|
|
{
|
|
Assignment tmp2 = tmp;
|
|
tmp = tmp2.Save();
|
|
tmp2.Dispose();
|
|
}
|
|
else
|
|
{
|
|
Csla.Validation.BrokenRulesCollection brc = tmp.ValidationRules.GetBrokenRules();
|
|
tmp._ErrorMessage = "Failed Validation:";
|
|
foreach (Csla.Validation.BrokenRule br in brc)
|
|
{
|
|
tmp._ErrorMessage += "\r\n\tFailure: " + br.RuleName;
|
|
}
|
|
}
|
|
return tmp;
|
|
}
|
|
public static Assignment New(Group myGroup, Role myRole, Folder myFolder, string endDate)
|
|
{
|
|
Assignment tmp = Assignment.New();
|
|
tmp.MyGroup = myGroup;
|
|
tmp.MyRole = myRole;
|
|
tmp.MyFolder = myFolder;
|
|
tmp.EndDate = endDate;
|
|
return tmp;
|
|
}
|
|
public static Assignment MakeAssignment(Group myGroup, Role myRole, Folder myFolder, string endDate)
|
|
{
|
|
Assignment tmp = Assignment.New(myGroup, myRole, myFolder, endDate);
|
|
if (tmp.IsSavable)
|
|
{
|
|
Assignment tmp2 = tmp;
|
|
tmp = tmp2.Save();
|
|
tmp2.Dispose();
|
|
}
|
|
else
|
|
{
|
|
Csla.Validation.BrokenRulesCollection brc = tmp.ValidationRules.GetBrokenRules();
|
|
tmp._ErrorMessage = "Failed Validation:";
|
|
foreach (Csla.Validation.BrokenRule br in brc)
|
|
{
|
|
tmp._ErrorMessage += "\r\n\tFailure: " + br.RuleName;
|
|
}
|
|
}
|
|
return tmp;
|
|
}
|
|
public static Assignment Get(int aid)
|
|
{
|
|
if (!CanGetObject())
|
|
throw new System.Security.SecurityException("User not authorized to view a Assignment");
|
|
try
|
|
{
|
|
Assignment tmp = GetCachedByPrimaryKey(aid);
|
|
if (tmp == null)
|
|
{
|
|
tmp = DataPortal.Fetch<Assignment>(new PKCriteria(aid));
|
|
AddToCache(tmp);
|
|
}
|
|
if (tmp.ErrorMessage == "No Record Found")
|
|
{
|
|
tmp.Dispose(); // Clean-up Assignment
|
|
tmp = null;
|
|
}
|
|
return tmp;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on Assignment.Get", ex);
|
|
}
|
|
}
|
|
public static Assignment Get(SafeDataReader dr)
|
|
{
|
|
if (dr.Read()) return new Assignment(dr);
|
|
return null;
|
|
}
|
|
internal Assignment(SafeDataReader dr)
|
|
{
|
|
ReadData(dr);
|
|
}
|
|
public static void Delete(int aid)
|
|
{
|
|
if (!CanDeleteObject())
|
|
throw new System.Security.SecurityException("User not authorized to remove a Assignment");
|
|
try
|
|
{
|
|
DataPortal.Delete(new PKCriteria(aid));
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on Assignment.Delete", ex);
|
|
}
|
|
}
|
|
public override Assignment Save()
|
|
{
|
|
if (IsDeleted && !CanDeleteObject())
|
|
throw new System.Security.SecurityException("User not authorized to remove a Assignment");
|
|
else if (IsNew && !CanAddObject())
|
|
throw new System.Security.SecurityException("User not authorized to add a Assignment");
|
|
else if (!CanEditObject())
|
|
throw new System.Security.SecurityException("User not authorized to update a Assignment");
|
|
try
|
|
{
|
|
BuildRefreshList();
|
|
Assignment assignment = base.Save();
|
|
RemoveFromDictionaries(); // if save is successful remove the previous Folder from the cache
|
|
AddToCache(assignment);//Refresh the item in AllList
|
|
ProcessRefreshList();
|
|
return assignment;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on CSLA Save", ex);
|
|
}
|
|
}
|
|
#endregion
|
|
#region Data Access Portal
|
|
[Serializable()]
|
|
protected class PKCriteria
|
|
{
|
|
private int _AID;
|
|
public int AID
|
|
{ get { return _AID; } }
|
|
public PKCriteria(int aid)
|
|
{
|
|
_AID = aid;
|
|
}
|
|
}
|
|
// CSLATODO: If Create needs to access DB - It should not be marked RunLocal
|
|
[RunLocal()]
|
|
private new void DataPortal_Create()
|
|
{
|
|
_AID = NextAID;
|
|
// Database Defaults
|
|
_StartDate = _AssignmentExtension.DefaultStartDate;
|
|
_DTS = _AssignmentExtension.DefaultDTS;
|
|
_UsrID = _AssignmentExtension.DefaultUsrID;
|
|
// CSLATODO: Add any defaults that are necessary
|
|
ValidationRules.CheckRules();
|
|
}
|
|
private void ReadData(SafeDataReader dr)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Assignment.ReadData", GetHashCode());
|
|
try
|
|
{
|
|
_AID = dr.GetInt32("AID");
|
|
_GID = dr.GetInt32("GID");
|
|
_RID = dr.GetInt32("RID");
|
|
_FolderID = dr.GetInt32("FolderID");
|
|
_StartDate = dr.GetSmartDate("StartDate").Text;
|
|
_EndDate = dr.GetSmartDate("EndDate").Text;
|
|
_DTS = dr.GetDateTime("DTS");
|
|
_UsrID = dr.GetString("UsrID");
|
|
dr.GetBytes("LastChanged", 0, _LastChanged, 0, 8);
|
|
MarkOld();
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Assignment.ReadData", ex);
|
|
_ErrorMessage = ex.Message;
|
|
throw new DbCslaException("Assignment.ReadData", ex);
|
|
}
|
|
}
|
|
private void DataPortal_Fetch(PKCriteria criteria)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Assignment.DataPortal_Fetch", GetHashCode());
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
ApplicationContext.LocalContext["cn"] = cn;
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "getAssignment";
|
|
cm.Parameters.AddWithValue("@AID", criteria.AID);
|
|
using (SafeDataReader dr = new SafeDataReader(cm.ExecuteReader()))
|
|
{
|
|
if (!dr.Read())
|
|
{
|
|
_ErrorMessage = "No Record Found";
|
|
return;
|
|
}
|
|
ReadData(dr);
|
|
}
|
|
}
|
|
// removing of item only needed for local data portal
|
|
if (ApplicationContext.ExecutionLocation == ApplicationContext.ExecutionLocations.Client)
|
|
ApplicationContext.LocalContext.Remove("cn");
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Assignment.DataPortal_Fetch", ex);
|
|
_ErrorMessage = ex.Message;
|
|
throw new DbCslaException("Assignment.DataPortal_Fetch", ex);
|
|
}
|
|
}
|
|
[Transactional(TransactionalTypes.TransactionScope)]
|
|
protected override void DataPortal_Insert()
|
|
{
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
ApplicationContext.LocalContext["cn"] = cn;
|
|
SQLInsert();
|
|
// removing of item only needed for local data portal
|
|
if (ApplicationContext.ExecutionLocation == ApplicationContext.ExecutionLocations.Client)
|
|
ApplicationContext.LocalContext.Remove("cn");
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Assignment.DataPortal_Insert", ex);
|
|
_ErrorMessage = ex.Message;
|
|
throw new DbCslaException("Assignment.DataPortal_Insert", ex);
|
|
}
|
|
finally
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Assignment.DataPortal_Insert", GetHashCode());
|
|
}
|
|
}
|
|
[Transactional(TransactionalTypes.TransactionScope)]
|
|
internal void SQLInsert()
|
|
{
|
|
if (!this.IsDirty) return;
|
|
try
|
|
{
|
|
if (_MyFolder != null) _MyFolder.Update();
|
|
if (_MyGroup != null) _MyGroup.Update();
|
|
if (_MyRole != null) _MyRole.Update();
|
|
SqlConnection cn = (SqlConnection)ApplicationContext.LocalContext["cn"];
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "addAssignment";
|
|
// Input All Fields - Except Calculated Columns
|
|
cm.Parameters.AddWithValue("@GID", GID);
|
|
cm.Parameters.AddWithValue("@RID", RID);
|
|
cm.Parameters.AddWithValue("@FolderID", FolderID);
|
|
cm.Parameters.AddWithValue("@StartDate", new SmartDate(_StartDate).DBValue);
|
|
cm.Parameters.AddWithValue("@EndDate", new SmartDate(_EndDate).DBValue);
|
|
if (_DTS.Year >= 1753 && _DTS.Year <= 9999) cm.Parameters.AddWithValue("@DTS", _DTS);
|
|
cm.Parameters.AddWithValue("@UsrID", _UsrID);
|
|
// Output Calculated Columns
|
|
SqlParameter param_AID = new SqlParameter("@newAID", SqlDbType.Int);
|
|
param_AID.Direction = ParameterDirection.Output;
|
|
cm.Parameters.Add(param_AID);
|
|
SqlParameter param_LastChanged = new SqlParameter("@newLastChanged", SqlDbType.Timestamp);
|
|
param_LastChanged.Direction = ParameterDirection.Output;
|
|
cm.Parameters.Add(param_LastChanged);
|
|
// CSLATODO: Define any additional output parameters
|
|
cm.ExecuteNonQuery();
|
|
// Save all values being returned from the Procedure
|
|
_AID = (int)cm.Parameters["@newAID"].Value;
|
|
_LastChanged = (byte[])cm.Parameters["@newLastChanged"].Value;
|
|
}
|
|
MarkOld();
|
|
// update child objects
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Assignment.SQLInsert", GetHashCode());
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Assignment.SQLInsert", ex);
|
|
_ErrorMessage = ex.Message;
|
|
throw new DbCslaException("Assignment.SQLInsert", ex);
|
|
}
|
|
}
|
|
[Transactional(TransactionalTypes.TransactionScope)]
|
|
public static byte[] Add(SqlConnection cn, ref int aid, Group myGroup, Role myRole, Folder myFolder, SmartDate startDate, SmartDate endDate, DateTime dts, string usrID)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Assignment.Add", 0);
|
|
try
|
|
{
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "addAssignment";
|
|
// Input All Fields - Except Calculated Columns
|
|
cm.Parameters.AddWithValue("@GID", myGroup.GID);
|
|
cm.Parameters.AddWithValue("@RID", myRole.RID);
|
|
cm.Parameters.AddWithValue("@FolderID", myFolder.FolderID);
|
|
cm.Parameters.AddWithValue("@StartDate", startDate.DBValue);
|
|
cm.Parameters.AddWithValue("@EndDate", endDate.DBValue);
|
|
if (dts.Year >= 1753 && dts.Year <= 9999) cm.Parameters.AddWithValue("@DTS", dts);
|
|
cm.Parameters.AddWithValue("@UsrID", usrID);
|
|
// Output Calculated Columns
|
|
SqlParameter param_AID = new SqlParameter("@newAID", SqlDbType.Int);
|
|
param_AID.Direction = ParameterDirection.Output;
|
|
cm.Parameters.Add(param_AID);
|
|
SqlParameter param_LastChanged = new SqlParameter("@newLastChanged", SqlDbType.Timestamp);
|
|
param_LastChanged.Direction = ParameterDirection.Output;
|
|
cm.Parameters.Add(param_LastChanged);
|
|
// CSLATODO: Define any additional output parameters
|
|
cm.ExecuteNonQuery();
|
|
// Save all values being returned from the Procedure
|
|
aid = (int)cm.Parameters["@newAID"].Value;
|
|
return (byte[])cm.Parameters["@newLastChanged"].Value;
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Assignment.Add", ex);
|
|
throw new DbCslaException("Assignment.Add", ex);
|
|
}
|
|
}
|
|
[Transactional(TransactionalTypes.TransactionScope)]
|
|
protected override void DataPortal_Update()
|
|
{
|
|
if (!IsDirty) return; // If not dirty - nothing to do
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Assignment.DataPortal_Update", GetHashCode());
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
ApplicationContext.LocalContext["cn"] = cn;
|
|
SQLUpdate();
|
|
// removing of item only needed for local data portal
|
|
if (ApplicationContext.ExecutionLocation == ApplicationContext.ExecutionLocations.Client)
|
|
ApplicationContext.LocalContext.Remove("cn");
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Assignment.DataPortal_Update", ex);
|
|
_ErrorMessage = ex.Message;
|
|
if (!ex.Message.EndsWith("has been edited by another user.")) throw ex;
|
|
}
|
|
}
|
|
[Transactional(TransactionalTypes.TransactionScope)]
|
|
internal void SQLUpdate()
|
|
{
|
|
if (!IsDirty) return; // If not dirty - nothing to do
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Assignment.SQLUpdate", GetHashCode());
|
|
try
|
|
{
|
|
if (_MyFolder != null) _MyFolder.Update();
|
|
if (_MyGroup != null) _MyGroup.Update();
|
|
if (_MyRole != null) _MyRole.Update();
|
|
SqlConnection cn = (SqlConnection)ApplicationContext.LocalContext["cn"];
|
|
if (base.IsDirty)
|
|
{
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "updateAssignment";
|
|
// All Fields including Calculated Fields
|
|
cm.Parameters.AddWithValue("@AID", _AID);
|
|
cm.Parameters.AddWithValue("@GID", GID);
|
|
cm.Parameters.AddWithValue("@RID", RID);
|
|
cm.Parameters.AddWithValue("@FolderID", FolderID);
|
|
cm.Parameters.AddWithValue("@StartDate", new SmartDate(_StartDate).DBValue);
|
|
cm.Parameters.AddWithValue("@EndDate", new SmartDate(_EndDate).DBValue);
|
|
if (_DTS.Year >= 1753 && _DTS.Year <= 9999) cm.Parameters.AddWithValue("@DTS", _DTS);
|
|
cm.Parameters.AddWithValue("@UsrID", _UsrID);
|
|
cm.Parameters.AddWithValue("@LastChanged", _LastChanged);
|
|
// Output Calculated Columns
|
|
SqlParameter param_LastChanged = new SqlParameter("@newLastChanged", SqlDbType.Timestamp);
|
|
param_LastChanged.Direction = ParameterDirection.Output;
|
|
cm.Parameters.Add(param_LastChanged);
|
|
// CSLATODO: Define any additional output parameters
|
|
cm.ExecuteNonQuery();
|
|
// Save all values being returned from the Procedure
|
|
_LastChanged = (byte[])cm.Parameters["@newLastChanged"].Value;
|
|
}
|
|
}
|
|
MarkOld();
|
|
// use the open connection to update child objects
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Assignment.SQLUpdate", ex);
|
|
_ErrorMessage = ex.Message;
|
|
if (!ex.Message.EndsWith("has been edited by another user.")) throw ex;
|
|
}
|
|
}
|
|
internal void Update()
|
|
{
|
|
if (!this.IsDirty) return;
|
|
if (base.IsDirty)
|
|
{
|
|
SqlConnection cn = (SqlConnection)ApplicationContext.LocalContext["cn"];
|
|
if (IsNew)
|
|
_LastChanged = Assignment.Add(cn, ref _AID, _MyGroup, _MyRole, _MyFolder, new SmartDate(_StartDate), new SmartDate(_EndDate), _DTS, _UsrID);
|
|
else
|
|
_LastChanged = Assignment.Update(cn, ref _AID, _GID, _RID, _FolderID, new SmartDate(_StartDate), new SmartDate(_EndDate), _DTS, _UsrID, ref _LastChanged);
|
|
MarkOld();
|
|
}
|
|
}
|
|
[Transactional(TransactionalTypes.TransactionScope)]
|
|
public static byte[] Update(SqlConnection cn, ref int aid, int gid, int rid, int folderID, SmartDate startDate, SmartDate endDate, DateTime dts, string usrID, ref byte[] lastChanged)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Assignment.Update", 0);
|
|
try
|
|
{
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "updateAssignment";
|
|
// Input All Fields - Except Calculated Columns
|
|
cm.Parameters.AddWithValue("@AID", aid);
|
|
cm.Parameters.AddWithValue("@GID", gid);
|
|
cm.Parameters.AddWithValue("@RID", rid);
|
|
cm.Parameters.AddWithValue("@FolderID", folderID);
|
|
cm.Parameters.AddWithValue("@StartDate", startDate);
|
|
cm.Parameters.AddWithValue("@EndDate", endDate);
|
|
if (dts.Year >= 1753 && dts.Year <= 9999) cm.Parameters.AddWithValue("@DTS", dts);
|
|
cm.Parameters.AddWithValue("@UsrID", usrID);
|
|
cm.Parameters.AddWithValue("@LastChanged", lastChanged);
|
|
// Output Calculated Columns
|
|
SqlParameter param_LastChanged = new SqlParameter("@newLastChanged", SqlDbType.Timestamp);
|
|
param_LastChanged.Direction = ParameterDirection.Output;
|
|
cm.Parameters.Add(param_LastChanged);
|
|
// CSLATODO: Define any additional output parameters
|
|
cm.ExecuteNonQuery();
|
|
// Save all values being returned from the Procedure
|
|
return (byte[])cm.Parameters["@newLastChanged"].Value;
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Assignment.Update", ex);
|
|
throw new DbCslaException("Assignment.Update", ex);
|
|
}
|
|
}
|
|
[Transactional(TransactionalTypes.TransactionScope)]
|
|
protected override void DataPortal_DeleteSelf()
|
|
{
|
|
DataPortal_Delete(new PKCriteria(_AID));
|
|
}
|
|
[Transactional(TransactionalTypes.TransactionScope)]
|
|
private void DataPortal_Delete(PKCriteria criteria)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Assignment.DataPortal_Delete", GetHashCode());
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "deleteAssignment";
|
|
cm.Parameters.AddWithValue("@AID", criteria.AID);
|
|
cm.ExecuteNonQuery();
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Assignment.DataPortal_Delete", ex);
|
|
_ErrorMessage = ex.Message;
|
|
throw new DbCslaException("Assignment.DataPortal_Delete", ex);
|
|
}
|
|
}
|
|
[Transactional(TransactionalTypes.TransactionScope)]
|
|
public static void Remove(SqlConnection cn, int aid)
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Assignment.Remove", 0);
|
|
try
|
|
{
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "deleteAssignment";
|
|
// Input PK Fields
|
|
cm.Parameters.AddWithValue("@AID", aid);
|
|
// CSLATODO: Define any additional output parameters
|
|
cm.ExecuteNonQuery();
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Assignment.Remove", ex);
|
|
throw new DbCslaException("Assignment.Remove", ex);
|
|
}
|
|
}
|
|
#endregion
|
|
#region Exists
|
|
public static bool Exists(int aid)
|
|
{
|
|
ExistsCommand result;
|
|
try
|
|
{
|
|
result = DataPortal.Execute<ExistsCommand>(new ExistsCommand(aid));
|
|
return result.Exists;
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
throw new DbCslaException("Error on Assignment.Exists", ex);
|
|
}
|
|
}
|
|
[Serializable()]
|
|
private class ExistsCommand : CommandBase
|
|
{
|
|
private int _AID;
|
|
private bool _exists;
|
|
public bool Exists
|
|
{
|
|
get { return _exists; }
|
|
}
|
|
public ExistsCommand(int aid)
|
|
{
|
|
_AID = aid;
|
|
}
|
|
protected override void DataPortal_Execute()
|
|
{
|
|
if (_MyLog.IsDebugEnabled) _MyLog.DebugFormat("[{0}] Assignment.DataPortal_Execute", GetHashCode());
|
|
try
|
|
{
|
|
using (SqlConnection cn = Database.VEPROMS_SqlConnection)
|
|
{
|
|
cn.Open();
|
|
using (SqlCommand cm = cn.CreateCommand())
|
|
{
|
|
cm.CommandType = CommandType.StoredProcedure;
|
|
cm.CommandText = "existsAssignment";
|
|
cm.Parameters.AddWithValue("@AID", _AID);
|
|
int count = (int)cm.ExecuteScalar();
|
|
_exists = (count > 0);
|
|
}
|
|
}
|
|
}
|
|
catch (Exception ex)
|
|
{
|
|
if (_MyLog.IsErrorEnabled) _MyLog.Error("Assignment.DataPortal_Execute", ex);
|
|
throw new DbCslaException("Assignment.DataPortal_Execute", ex);
|
|
}
|
|
}
|
|
}
|
|
#endregion
|
|
// Standard Default Code
|
|
#region extension
|
|
AssignmentExtension _AssignmentExtension = new AssignmentExtension();
|
|
[Serializable()]
|
|
partial class AssignmentExtension : extensionBase
|
|
{
|
|
}
|
|
[Serializable()]
|
|
class extensionBase
|
|
{
|
|
// Default Values
|
|
public virtual string DefaultStartDate
|
|
{
|
|
get { return DateTime.Now.ToShortDateString(); }
|
|
}
|
|
public virtual DateTime DefaultDTS
|
|
{
|
|
get { return DateTime.Now; }
|
|
}
|
|
public virtual string DefaultUsrID
|
|
{
|
|
get { return Environment.UserName.ToUpper(); }
|
|
}
|
|
// Authorization Rules
|
|
public virtual void AddAuthorizationRules(Csla.Security.AuthorizationRules rules)
|
|
{
|
|
// Needs to be overriden to add new authorization rules
|
|
}
|
|
// Instance Authorization Rules
|
|
public virtual void AddInstanceAuthorizationRules(Csla.Security.AuthorizationRules rules)
|
|
{
|
|
// Needs to be overriden to add new authorization rules
|
|
}
|
|
// Validation Rules
|
|
public virtual void AddValidationRules(Csla.Validation.ValidationRules rules)
|
|
{
|
|
// Needs to be overriden to add new validation rules
|
|
}
|
|
// InstanceValidation Rules
|
|
public virtual void AddInstanceValidationRules(Csla.Validation.ValidationRules rules)
|
|
{
|
|
// Needs to be overriden to add new validation rules
|
|
}
|
|
}
|
|
#endregion
|
|
} // Class
|
|
#region Converter
|
|
internal class AssignmentConverter : ExpandableObjectConverter
|
|
{
|
|
public override object ConvertTo(ITypeDescriptorContext context, System.Globalization.CultureInfo culture, object value, Type destType)
|
|
{
|
|
if (destType == typeof(string) && value is Assignment)
|
|
{
|
|
// Return the ToString value
|
|
return ((Assignment)value).ToString();
|
|
}
|
|
return base.ConvertTo(context, culture, value, destType);
|
|
}
|
|
}
|
|
#endregion
|
|
} // Namespace
|
|
|
|
|
|
//// The following is a sample Extension File. You can use it to create AssignmentExt.cs
|
|
//using System;
|
|
//using System.Collections.Generic;
|
|
//using System.Text;
|
|
//using Csla;
|
|
|
|
//namespace VEPROMS.CSLA.Library
|
|
//{
|
|
// public partial class Assignment
|
|
// {
|
|
// partial class AssignmentExtension : extensionBase
|
|
// {
|
|
// // CSLATODO: Override automatic defaults
|
|
// public virtual SmartDate DefaultStartDate
|
|
// {
|
|
// get { return DateTime.Now.ToShortDateString(); }
|
|
// }
|
|
// public virtual DateTime DefaultDTS
|
|
// {
|
|
// get { return DateTime.Now; }
|
|
// }
|
|
// public virtual string DefaultUsrID
|
|
// {
|
|
// get { return Environment.UserName.ToUpper(); }
|
|
// }
|
|
// public new void AddAuthorizationRules(Csla.Security.AuthorizationRules rules)
|
|
// {
|
|
// //rules.AllowRead(Dbid, "<Role(s)>");
|
|
// }
|
|
// public new void AddInstanceAuthorizationRules(Csla.Security.AuthorizationRules rules)
|
|
// {
|
|
// //rules.AllowInstanceRead(Dbid, "<Role(s)>");
|
|
// }
|
|
// public new void AddValidationRules(Csla.Validation.ValidationRules rules)
|
|
// {
|
|
// rules.AddRule(
|
|
// Csla.Validation.CommonRules.StringMaxLength,
|
|
// new Csla.Validation.CommonRules.MaxLengthRuleArgs("Name", 100));
|
|
// }
|
|
// public new void AddInstanceValidationRules(Csla.Validation.ValidationRules rules)
|
|
// {
|
|
// rules.AddInstanceRule(/* Instance Validation Rule */);
|
|
// }
|
|
// }
|
|
// }
|
|
//}
|