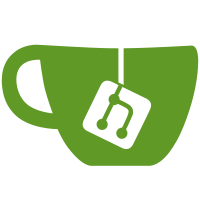
If the selected Library Document is out-of-range throw a specific error Don't fail if the user clicks on open space in the BookMark list Added property to provide a right margin (RTBMargin) between the RichTextBox and the StepItem
773 lines
32 KiB
C#
773 lines
32 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.ComponentModel;
|
|
using System.Data;
|
|
using System.Drawing;
|
|
using System.Text;
|
|
using System.Windows.Forms;
|
|
using VEPROMS.CSLA.Library;
|
|
using VEPROMS.Properties;
|
|
using DevComponents.DotNetBar;
|
|
using DevComponents.DotNetBar.Controls;
|
|
using Volian.Controls.Library;
|
|
using DescriptiveEnum;
|
|
|
|
namespace VEPROMS
|
|
{
|
|
public partial class frmSectionProperties : DevComponents.DotNetBar.Office2007Form
|
|
{
|
|
private string _DefaultFormatName = null;
|
|
private string _DefaultNumColumns = null;
|
|
private string _DefaultPaginationStyle = null;
|
|
private string _DefaultPrintSize = null;
|
|
private bool _Initializing;
|
|
private SectionConfig _SectionConfig;
|
|
private Document _DocumentToDelete = null;
|
|
private StepTabRibbon _MyStepTabRibbon;
|
|
private bool _isStepSection = true;
|
|
|
|
public frmSectionProperties(SectionConfig sectionConfig)
|
|
{
|
|
_SectionConfig = sectionConfig;
|
|
InitializeComponent();
|
|
btnGeneral.PerformClick(); // always start with General tab or button
|
|
ItemInfo ii = ItemInfo.Get(_SectionConfig.MySection.ItemID);
|
|
//if (sectionConfig.Number.Length > 0)
|
|
// this.Text = string.Format("{0} {1} Properties", sectionConfig.Number, sectionConfig.Title);
|
|
//else
|
|
// this.Text = string.Format("{0} Properties", sectionConfig.Title);
|
|
if (sectionConfig.Number.Length > 0)
|
|
this.Text = string.Format("{0} {1} Properties", ii.DisplayNumber, ii.DisplayText);
|
|
else
|
|
this.Text = string.Format("{0} Properties", ii.DisplayText);
|
|
ppSectTitleStpRTB.FieldToEdit = E_FieldToEdit.Text;
|
|
ppSectTitleStpRTB.BorderStyle = BorderStyle.Fixed3D;
|
|
ppSectTitleStpRTB.ViewRTB = false;
|
|
ppSectTitleStpRTB.MyItemInfo = ii;
|
|
|
|
ppSectNumberStpRTB.FieldToEdit = E_FieldToEdit.Number;
|
|
ppSectNumberStpRTB.BorderStyle = BorderStyle.Fixed3D;
|
|
ppSectNumberStpRTB.ViewRTB = false;
|
|
ppSectNumberStpRTB.MyItemInfo = ii;
|
|
|
|
_MyStepTabRibbon = new StepTabRibbon();
|
|
//_MyStepTabRibbon.Dock = System.Windows.Forms.DockStyle.Top;
|
|
//_MyStepTabRibbon.Location = new System.Drawing.Point(0, 0);
|
|
_MyStepTabRibbon.Name = "displayTabRibbon1";
|
|
_MyStepTabRibbon.Visible = false;
|
|
|
|
// if creating a new section, enable the StepSect and WordSect radio buttons
|
|
rbStepSect.Enabled = rbWordSect.Enabled = !(ii.HasWordContent || ii.HasStepContent);
|
|
|
|
// if this has already been set to be a step section, i.e. HasStepContent, disable the libdoc tab
|
|
// also disable if there is no word content yet - the user may want to change from step type to
|
|
// word type, as long as there is no content yet.
|
|
if (ii.HasStepContent || !ii.HasWordContent) tcpLibDoc.Enabled = false;
|
|
}
|
|
|
|
private void btnSectPropOK_Click(object sender, EventArgs e)
|
|
{
|
|
sectionConfigBindingSource.EndEdit();
|
|
|
|
// Save Default settings for User
|
|
//
|
|
// Save whether we should display the default values on this property page
|
|
Settings.Default.ShowDefaultSectionProp = ppCbShwDefSettings.Checked;
|
|
Settings.Default.Save();
|
|
DialogResult = DialogResult.OK;
|
|
// save the type based on selection.
|
|
_SectionConfig.MySection.MyContent.Type = ((DocStyle)ppCmbxStyleSectionType.SelectedItem).Index + 10000;
|
|
Section mySection = _SectionConfig.MySection.Save();
|
|
FinishSectionSave(mySection);
|
|
// if there was a document to delete, do it.
|
|
if (_DocumentToDelete != null)
|
|
{
|
|
Document.Delete(_DocumentToDelete.DocID);
|
|
_DocumentToDelete = null;
|
|
}
|
|
|
|
ppSectNumberStpRTB.SaveText();
|
|
ppSectTitleStpRTB.SaveText();
|
|
mySection.Dispose();
|
|
this.Close();
|
|
}
|
|
private static void FinishSectionSave(Section section)
|
|
{
|
|
ItemInfo sectinfo = ItemInfo.Get(section.ItemID);
|
|
|
|
// need to find out if this is a word document type section & if it is, create a new word doc.
|
|
bool isWordSect = true;
|
|
int sectype = (int)sectinfo.MyContent.Type - 10000;
|
|
PlantFormat pf = sectinfo.ActiveFormat.PlantFormat;
|
|
for (int i = 0; i < pf.DocStyles.DocStyleList.Count; i++)
|
|
{
|
|
if (pf.DocStyles.DocStyleList[i].Index == sectype)
|
|
{
|
|
isWordSect = !pf.DocStyles.DocStyleList[i].IsStepSection;
|
|
break;
|
|
}
|
|
}
|
|
if (isWordSect && !sectinfo.HasWordContent)
|
|
{
|
|
Content cont = Content.Get(sectinfo.MyContent.ContentID);
|
|
|
|
Byte[] tstbyte = System.Text.Encoding.Default.GetBytes("");
|
|
Document doc = Document.MakeDocument(null, tstbyte, null, null, null); // tstbyte, null, null, null);
|
|
Entry entry = cont.MyEntry;
|
|
entry.MyDocument = Document.Get(doc.DocID);
|
|
cont.Save().Dispose();
|
|
}
|
|
}
|
|
private void btnSectPropCancel_Click(object sender, EventArgs e)
|
|
{
|
|
_DocumentToDelete = null;
|
|
sectionConfigBindingSource.CancelEdit();
|
|
DialogResult = DialogResult.Cancel;
|
|
this.Close();
|
|
}
|
|
|
|
/// <summary>
|
|
/// Use the ParentLookup to grab the default values
|
|
/// - set the watermark property (where applicable) of the control with that value
|
|
/// - set the default setting labels with that value
|
|
/// ** the default setting labels appear when the Show Default Values checkbox is checked by the user.
|
|
/// </summary>
|
|
private void FindDefaultValues()
|
|
{
|
|
_SectionConfig.ParentLookup = true;
|
|
// Get the default format name
|
|
_DefaultFormatName = _SectionConfig.DefaultFormatSelection;
|
|
SetupDefault(_DefaultFormatName, ppLblFormatDefault, ppCmbxFormat);
|
|
//if (_DefaultFormatName != null && !(_DefaultFormatName.Equals("")))
|
|
//{
|
|
// string defName = string.Format("{0}", _DefaultFormatName);
|
|
// ppLblFormatDefault.Text = defName;
|
|
// ppCmbxFormat.WatermarkText = defName;
|
|
//}
|
|
_DefaultNumColumns = _SectionConfig.Section_ColumnMode.ToString();
|
|
SetupDefault(EnumDescConverter.GetEnumDescription(_SectionConfig.Section_ColumnMode), ppLblDefaultNumColumns, ppCmbxNumColumns);
|
|
|
|
_DefaultPaginationStyle = _SectionConfig.Section_Pagination.ToString();
|
|
SetupDefault(EnumDescConverter.GetEnumDescription(_SectionConfig.Section_Pagination), ppLblDefPaginationStyle, ppCmbxSectPagination);
|
|
|
|
//_DefaultPrintSize = _SectionConfig.Section_AttachmentPrintSize.ToString();
|
|
//SetupDefault(EnumDescConverter.GetEnumDescription(_SectionConfig.Section_AttachmentPrintSize), ppLblDefaultPrintSize, ppCmbxAccPgPrintSize);
|
|
|
|
_SectionConfig.ParentLookup = false;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Set the watermark and default label
|
|
/// </summary>
|
|
/// <param name="defaultText">The default text</param>
|
|
/// <param name="lbl">Label that displays the current default when view defaults is set</param>
|
|
/// <param name="cmboEx">Combo box with a watermark property</param>
|
|
private static void SetupDefault(string defaultText, Label lbl, ComboBoxEx cmbo)
|
|
{
|
|
if (defaultText != null && !(defaultText.Equals("")))
|
|
{
|
|
string deftext = string.Format("{0}", defaultText);
|
|
lbl.Text = deftext;
|
|
cmbo.WatermarkText = deftext;
|
|
}
|
|
}
|
|
|
|
private void frmSectionProperties_Load(object sender, EventArgs e)
|
|
{
|
|
_Initializing = true;
|
|
sectionConfigBindingSource.DataSource = _SectionConfig;
|
|
|
|
ppCmbxFormat.DataSource = null;
|
|
ppCmbxFormat.DisplayMember = "FullName";
|
|
ppCmbxFormat.ValueMember = "FullName";
|
|
ppCmbxFormat.DataSource = FormatInfoList.SortedFormatInfoList;
|
|
|
|
// Get the saved settings for this user
|
|
//
|
|
// Get setting telling us whether to display the default values on this property page
|
|
ppCbShwDefSettings.Checked = (Settings.Default["ShowDefaultSectionProp"] != null) ? Settings.Default.ShowDefaultSectionProp : false;
|
|
|
|
// Get the User's property page style "PropPageStyle" (this is a system wide user setting)
|
|
// 1 - Button Dialog (default)
|
|
// 2 - Tab Dialog
|
|
if ((int)Settings.Default["PropPageStyle"] == (int)PropPgStyle.Tab)
|
|
{
|
|
tcSectionProp.TabsVisible = true;
|
|
panSectBtns.Visible = false;
|
|
this.Width -= panSectBtns.Width;
|
|
}
|
|
|
|
// Get the default values for the property page information
|
|
FindDefaultValues();
|
|
|
|
ppCmbxSectPagination.DataSource = EnumDetail<SectionConfig.SectionPagination>.Details();
|
|
ppCmbxSectPagination.DisplayMember = "Description";
|
|
ppCmbxSectPagination.ValueMember = "EValue";
|
|
ppCmbxSectPagination.SelectedIndex = -1;
|
|
|
|
ppCmbxNumColumns.DataSource = EnumDetail<SectionConfig.SectionColumnMode>.Details();
|
|
ppCmbxNumColumns.DisplayMember = "Description";
|
|
ppCmbxNumColumns.ValueMember = "EValue";
|
|
ppCmbxNumColumns.SelectedIndex = -1;
|
|
|
|
//ppCmbxAccPgPrintSize.DataSource = EnumDetail<SectionConfig.AttPrintSize>.Details();
|
|
//ppCmbxAccPgPrintSize.DisplayMember = "Description";
|
|
//ppCmbxAccPgPrintSize.ValueMember = "Evalue";
|
|
//ppCmbxAccPgPrintSize.SelectedIndex = -1;
|
|
|
|
if (!_isStepSection)
|
|
ppCmbxLibDocFill();
|
|
|
|
// check type of section from document styles to determine if the stepsection checkbox should
|
|
// be checked.
|
|
int secindx = (int)_SectionConfig.SectionType;
|
|
// find the index for the document style to determine whether this is a step or word section.
|
|
PlantFormat pf = _SectionConfig.MyFormat != null ? _SectionConfig.MyFormat.PlantFormat : _SectionConfig.MyDefaultFormat.PlantFormat;
|
|
for (int i = 0; i < pf.DocStyles.DocStyleList.Count; i++)
|
|
{
|
|
if (pf.DocStyles.DocStyleList[i].Index == secindx)
|
|
rbStepSect.Checked = pf.DocStyles.DocStyleList[i].IsStepSection;
|
|
}
|
|
// set up some of the format tab:
|
|
SetupPpCmbxSectionType();
|
|
ShowAvailableOptionsForSectionType();
|
|
_Initializing = false;
|
|
}
|
|
|
|
private void ppCmbxLibDocFill()
|
|
{
|
|
_Initializing = true;
|
|
bool isLib = false;
|
|
if (_SectionConfig.MySection.MyContent.MyEntry!=null && _SectionConfig.MySection.MyContent.MyEntry.MyDocument!=null && (_SectionConfig.MySection.MyContent.MyEntry.MyDocument.LibTitle != null) && (_SectionConfig.MySection.MyContent.MyEntry.MyDocument.LibTitle != "")) isLib = true;
|
|
if (isLib)
|
|
{
|
|
ppBtnCvrtToLibDoc.Text = "Convert this Section To A Non-Library Document";
|
|
lblLibraryDocument.Text = "Library Document";
|
|
superTooltip1.SetSuperTooltip(this.ppBtnCvrtToLibDoc, new DevComponents.DotNetBar.SuperTooltipInfo("Convert To Non-Library Document", "", "This button will convert the current section from a library document to a non-library document",
|
|
null, null, DevComponents.DotNetBar.eTooltipColor.Gray, true, false, new System.Drawing.Size(250, 76)));
|
|
}
|
|
else
|
|
{
|
|
if (_SectionConfig.MySection.MyContent.MyEntry.MyDocument == null)
|
|
ppBtnCvrtToLibDoc.Visible = false;
|
|
else
|
|
{
|
|
ppBtnCvrtToLibDoc.Text = "Convert this to a Library Document";
|
|
lblLibraryDocument.Text = "Select Library Document";
|
|
superTooltip1.SetSuperTooltip(this.ppBtnCvrtToLibDoc, new DevComponents.DotNetBar.SuperTooltipInfo("Convert To Library Document button", "", "This button will convert the current section to a library document, allowing it t" +
|
|
"o be shared with other procedures.", null, null, DevComponents.DotNetBar.eTooltipColor.Gray, true, false, new System.Drawing.Size(250, 76)));
|
|
}
|
|
}
|
|
|
|
DocumentInfoList LibDocList = DocumentInfoList.GetLibraries(true);
|
|
int selindx = -1;
|
|
ppCmbxLibDoc.Items.Clear();
|
|
for (int i = 0; i < LibDocList.Count; i++)
|
|
{
|
|
ppCmbxLibDoc.Items.Add(LibDocList[i].DocumentTitle);
|
|
// see if this lib doc should be the selected index?
|
|
if (isLib && (LibDocList[i].DocID == _SectionConfig.MySection.MyContent.MyEntry.MyDocument.DocID)) selindx = i;
|
|
}
|
|
if (selindx > -1) ppCmbxLibDoc.SelectedIndex = selindx;
|
|
_Initializing = false;
|
|
}
|
|
|
|
#region General tab
|
|
|
|
/// <summary>
|
|
/// This is the General button used on the button interface design
|
|
/// </summary>
|
|
/// <param name="sender">object</param>
|
|
/// <param name="e">EventArgs</param>
|
|
private void btnGeneral_Click(object sender, EventArgs e)
|
|
{
|
|
ProcessButtonClick(tiGeneral, btnGeneral);
|
|
}
|
|
|
|
#endregion
|
|
|
|
#region Format tab
|
|
|
|
/// <summary>
|
|
/// This is the Format button used on the button interface design
|
|
/// </summary>
|
|
/// <param name="sender">object</param>
|
|
/// <param name="e">EventArgs</param>
|
|
private void btnFormat_Click(object sender, EventArgs e)
|
|
{
|
|
ProcessButtonClick(tiFormat, btnFormat);
|
|
|
|
}
|
|
|
|
private void SetupPpCmbxSectionType()
|
|
{
|
|
bool myInit = _Initializing;
|
|
// pf will be the current selected format. If changing formats, then this will differ from the previously
|
|
// selected. If the format is being reset to default, selectedindex=-1. If this is a change, we'll need
|
|
// to check that there are enough docstyles to map the selected type of section.
|
|
PlantFormat pf = _SectionConfig.MyFormat != null ? _SectionConfig.MyFormat.PlantFormat : _SectionConfig.MyDefaultFormat.PlantFormat;
|
|
if (ppCmbxFormat.SelectedIndex >= 0)
|
|
pf = FormatInfoList.SortedFormatInfoList[ppCmbxFormat.SelectedIndex].PlantFormat;
|
|
else if (!_Initializing) // if the format was changed, it may have been set to default.
|
|
pf = _SectionConfig.MyDefaultFormat.PlantFormat;
|
|
_Initializing = true;
|
|
ppCmbxStyleSectionType.DisplayMember = "Name";
|
|
ppCmbxStyleSectionType.ValueMember = "Index";
|
|
|
|
// if this has been defined as a step section, only list step section document styles, if
|
|
// a word section, only list word section styles.
|
|
// Also, if changing the format, check to be sure that the section that is selected will map
|
|
// to something valid in the new format, i.e. a section type of word or step sections exist,
|
|
// index-wise in the new format.
|
|
PlantFormat opf = null;
|
|
int oldSelIndx = -1;
|
|
if (!myInit)
|
|
{
|
|
DocStyleList oldDocStyles = null;
|
|
opf = _SectionConfig.MyFormat != null ? _SectionConfig.MyFormat.PlantFormat : _SectionConfig.MyDefaultFormat.PlantFormat;
|
|
if (pf != opf)
|
|
{
|
|
oldDocStyles = new DocStyleList(null);
|
|
for (int i = 0; i < opf.DocStyles.DocStyleList.Count; i++)
|
|
{
|
|
if (_isStepSection && opf.DocStyles.DocStyleList[i].IsStepSection)
|
|
oldDocStyles.Add(opf.DocStyles.DocStyleList[i]); // find only step section types
|
|
else if (!_isStepSection && !opf.DocStyles.DocStyleList[i].IsStepSection)
|
|
oldDocStyles.Add(opf.DocStyles.DocStyleList[i]); // find only accessory (MS Word) section types
|
|
if (_SectionConfig.SectionType == opf.DocStyles.DocStyleList[i].Index) oldSelIndx = oldDocStyles.Count - 1;
|
|
}
|
|
}
|
|
if (oldSelIndx < 0) oldSelIndx = 0;
|
|
}
|
|
|
|
DocStyleList newDocStyles = new DocStyleList(null);
|
|
int selindx = -1;
|
|
for (int i = 0; i < pf.DocStyles.DocStyleList.Count; i++)
|
|
{
|
|
if (_isStepSection && pf.DocStyles.DocStyleList[i].IsStepSection)
|
|
newDocStyles.Add(pf.DocStyles.DocStyleList[i]); // add only step section types
|
|
else if (!_isStepSection && !pf.DocStyles.DocStyleList[i].IsStepSection)
|
|
newDocStyles.Add(pf.DocStyles.DocStyleList[i]); // add only accessory (MS Word) section types
|
|
if (_SectionConfig.SectionType == pf.DocStyles.DocStyleList[i].Index) selindx = newDocStyles.Count - 1;
|
|
}
|
|
if (selindx < 0) selindx = 0;
|
|
// if changing format, check for valid type (see comment above)
|
|
if (!myInit && pf != opf)
|
|
{
|
|
if (oldSelIndx > newDocStyles.Count)
|
|
{
|
|
string msg = string.Format("The section type that is set is not available in the new format, it was reset to {0}. Verify that it is appropriate.", newDocStyles[0].Name);
|
|
MessageBox.Show(msg, "Non-existent section");
|
|
selindx = 0;
|
|
}
|
|
}
|
|
ppCmbxStyleSectionType.DataSource = newDocStyles;
|
|
ppCmbxStyleSectionType.SelectedIndex = selindx;
|
|
ppCmbxStyleSectionType.Refresh();
|
|
|
|
if (!_isStepSection)
|
|
ppCmbxLibDocFill();
|
|
_Initializing = myInit;
|
|
if (_isStepSection)
|
|
SetupCheckoffsDropdowns();
|
|
}
|
|
|
|
private void SetupCheckoffsDropdowns()
|
|
{
|
|
if (!_isStepSection) return; // not needed for accessory pages (word attachments)
|
|
bool myInit = _Initializing;
|
|
_Initializing = true;
|
|
PlantFormat pf = _SectionConfig.MyFormat!=null?_SectionConfig.MyFormat.PlantFormat:_SectionConfig.MyDefaultFormat.PlantFormat;
|
|
CheckOffList chkoffList = pf.FormatData.ProcData.CheckOffData.CheckOffList;
|
|
CheckOffHeaderList chkoffHeaderList = pf.FormatData.ProcData.CheckOffData.CheckOffHeaderList;
|
|
if (chkoffList != null && chkoffList.Count > 0)
|
|
{
|
|
ppGpbxSignoffCheckoff.Enabled = true;
|
|
ppCmbxCheckoffType.DataSource = chkoffList;
|
|
ppCmbxCheckoffType.SelectedIndex = _SectionConfig.Section_CheckoffListSelection;
|
|
}
|
|
else
|
|
{
|
|
ppGpbxSignoffCheckoff.Enabled = false;
|
|
}
|
|
if (chkoffHeaderList != null && chkoffHeaderList.Count > 0)
|
|
{
|
|
lblCheckoffHeading.Enabled = true;
|
|
ppCmbxCheckoffHeading.Enabled = true;
|
|
ppCmbxCheckoffHeading.DataSource = chkoffHeaderList;
|
|
ppCmbxCheckoffHeading.SelectedIndex = _SectionConfig.Section_CheckoffHeaderSelection;
|
|
}
|
|
else
|
|
{
|
|
lblCheckoffHeading.Enabled = false;
|
|
ppCmbxCheckoffHeading.Enabled = false;
|
|
}
|
|
_Initializing = myInit;
|
|
}
|
|
|
|
private void ppBtnDefaultFmt_Click(object sender, EventArgs e)
|
|
{
|
|
ppCmbxFormat.SelectedIndex = -1; //reset to the default Format setting
|
|
}
|
|
|
|
private void ppCmbxFormat_SelectedIndexChanged(object sender, EventArgs e)
|
|
{
|
|
if (_Initializing) return;
|
|
bool didDefault = false;
|
|
if ((ppCmbxFormat.SelectedIndex != -1) && _DefaultFormatName != null && _DefaultFormatName.Equals(ppCmbxFormat.SelectedValue))
|
|
{
|
|
ppBtnDefaultFmt.Focus();
|
|
ppBtnDefaultFmt.PerformClick();
|
|
didDefault = true;
|
|
}
|
|
ppBtnDefaultFmt.Visible = (ppCmbxFormat.SelectedValue != null);
|
|
ppLblFormatDefault.Visible = ppCbShwDefSettings.Checked && ppBtnDefaultFmt.Visible;
|
|
if (!didDefault)SetupPpCmbxSectionType();
|
|
}
|
|
|
|
private void ppCmbxStyleSectionType_SelectedValueChanged(object sender, EventArgs e)
|
|
{
|
|
if (_Initializing) return;
|
|
if (ppCmbxStyleSectionType.SelectedValue != null)
|
|
_SectionConfig.SectionType = (int)(ppCmbxStyleSectionType.SelectedValue);
|
|
}
|
|
|
|
#endregion
|
|
|
|
#region Library Document tab
|
|
|
|
/// <summary>
|
|
/// This is the Library Document button used on the button interface design
|
|
/// </summary>
|
|
/// <param name="sender">object</param>
|
|
/// <param name="e">EventArgs</param>
|
|
private void btnLibDocs_Click(object sender, EventArgs e)
|
|
{
|
|
ProcessButtonClick(tiLibDoc, btnLibDocs);
|
|
}
|
|
private void ppBtnCvrtToLibDoc_Click(object sender, EventArgs e)
|
|
{
|
|
// Double Check that this is not a step section, if so just return.
|
|
if (_isStepSection) return;
|
|
// If current section is library document, user selected to convert to non-library document. If
|
|
// this is the case, data from lib doc must be copied to new document and the section must point
|
|
// to id.
|
|
if (ppBtnCvrtToLibDoc.Text.IndexOf("Non") > 0) // convert to a 'non' library.
|
|
{
|
|
ItemInfo ii = ItemInfo.Get(_SectionConfig.MySection.ItemID);
|
|
DocumentInfo doclibinfo = ii.MyContent.MyEntry.MyDocument;
|
|
|
|
// if just one usage (this one), then just convert this to a non-library document. If there are more
|
|
// than one usage, make a copy so that the rest of the usages still point to the library document.
|
|
if (doclibinfo.DocumentEntryCount == 1)
|
|
_SectionConfig.MySection.MyContent.MyEntry.MyDocument.LibTitle = null;
|
|
else
|
|
{
|
|
// make new document with 'no' libtitle - use libtitle for the doc title. Then link this
|
|
// to the item...
|
|
Document doc = Document.MakeDocument(null, doclibinfo.DocContent, doclibinfo.DocAscii, doclibinfo.Config, doclibinfo.DocPdf);
|
|
_SectionConfig.MySection.MyContent.MyEntry.MyDocument = doc;
|
|
}
|
|
ppCmbxLibDoc.Items.Clear();
|
|
ppCmbxLibDoc.WatermarkEnabled = true;
|
|
ppBtnCvrtToLibDoc.Text = "Convert this to a Library Document";
|
|
superTooltip1.SetSuperTooltip(this.ppBtnCvrtToLibDoc, new DevComponents.DotNetBar.SuperTooltipInfo("Convert To Library Document button", "", "This button will convert the current section to a library document, allowing it t" +
|
|
"o be shared with other procedures.", null, null, DevComponents.DotNetBar.eTooltipColor.Gray, true, false, new System.Drawing.Size(250, 76)));
|
|
return;
|
|
}
|
|
// If current section is not a library document, it is either a new section or the user selected the
|
|
// button to convert it to a library document. Check for this, if new, just attach to document.
|
|
else if (_SectionConfig.MySection.MyContent.MyEntry.MyDocument == null)
|
|
{
|
|
// if nothing was selected, give message & return
|
|
if (ppCmbxLibDoc.SelectedIndex == -1)
|
|
{
|
|
MessageBox.Show("Must select a library document");
|
|
return;
|
|
}
|
|
int docid = -1;
|
|
DocumentInfoList LibDocList = DocumentInfoList.GetLibraries(true);
|
|
for (int i = 0; i < LibDocList.Count; i++)
|
|
{
|
|
if (LibDocList[i].LibTitle == (string)ppCmbxLibDoc.Items[ppCmbxLibDoc.SelectedIndex])
|
|
{
|
|
docid = LibDocList[i].DocID;
|
|
break;
|
|
}
|
|
}
|
|
if (docid == -1) return;
|
|
using (Document dc = Document.Get(docid))
|
|
{
|
|
_SectionConfig.MySection.MyContent.MyEntry.MyDocument = dc;
|
|
}
|
|
}
|
|
else
|
|
// if converting to library document, just put 'text' into the 'LibTitle' property (that's what flags
|
|
// it to be a library document.
|
|
{
|
|
_SectionConfig.MySection.MyContent.MyEntry.MyDocument.LibTitle = _SectionConfig.MySection.MyContent.Text;
|
|
// Save now so that it shows up in the library document list in the ppcmbxlibdoc (combo box
|
|
// listing lib docs that is regenerated in ppCmbxLibDocFill)
|
|
_SectionConfig.MySection.MyContent.MyEntry.MyDocument.Save();
|
|
ppCmbxLibDocFill();
|
|
ppCmbxLibDoc.Enabled = false;
|
|
}
|
|
ppBtnCvrtToLibDoc.Text = "Convert this Section To A Non-Library Document";
|
|
lblLibraryDocument.Text = "Library Document";
|
|
superTooltip1.SetSuperTooltip(this.ppBtnCvrtToLibDoc, new DevComponents.DotNetBar.SuperTooltipInfo("Convert To Non-Library Document", "", "This button will convert the current section from a library document to a non-library document",
|
|
null, null, DevComponents.DotNetBar.eTooltipColor.Gray, true, false, new System.Drawing.Size(250, 76)));
|
|
}
|
|
|
|
private void ppCmbxLibDoc_SelectedIndexChanged(object sender, EventArgs e)
|
|
{
|
|
if (_Initializing) return;
|
|
|
|
// first check if this is a 'new' section - if so, then just return.
|
|
if (_SectionConfig.MySection.MyContent.MyEntry == null) return;
|
|
// see if this was NOT a library document. If it is not a library document, ask the user if
|
|
// it should be linked, thus losing the original text/data.
|
|
if (_SectionConfig.MySection.MyContent.MyEntry.MyDocument != null && !(_SectionConfig.MySection.MyContent.MyEntry.MyDocument.LibTitle != ""))
|
|
{
|
|
_SectionConfig.MySection.MyContent.MyEntry.MyDocument = Document.New();
|
|
if (ppCmbxLibDoc.SelectedIndex > -1)
|
|
{
|
|
if (MessageBox.Show("Linking to this library document will cause loss of data to this section. Do you want to continue?", "Link", MessageBoxButtons.YesNo) == DialogResult.Yes)
|
|
{
|
|
_DocumentToDelete = _SectionConfig.MySection.MyContent.MyEntry.MyDocument;
|
|
DocumentInfoList LibDocList = DocumentInfoList.GetLibraries(true);
|
|
_SectionConfig.MySection.MyContent.MyEntry.MyDocument = Document.Get(LibDocList[ppCmbxLibDoc.SelectedIndex].DocID);
|
|
ppBtnCvrtToLibDoc.Enabled = true;
|
|
}
|
|
else
|
|
{
|
|
ppCmbxLibDoc.SelectedIndex = -1;
|
|
ppBtnCvrtToLibDoc.Enabled = false;
|
|
}
|
|
}
|
|
|
|
}
|
|
//else if (_SectionConfig.MySection.MyContent.MyEntry.MyDocument == null)
|
|
//{
|
|
// // was creating a new document & selected to connect it to a libdoc
|
|
// _DocumentToDelete = _SectionConfig.MySection.MyContent.MyEntry.MyDocument;
|
|
//}
|
|
else
|
|
{
|
|
// it already is a library document, just change usages...
|
|
DocumentInfoList LibDocList = DocumentInfoList.GetLibraries(true);
|
|
if (ppCmbxLibDoc.SelectedIndex >= 0 && ppCmbxLibDoc.SelectedIndex < LibDocList.Count)
|
|
{
|
|
_SectionConfig.MySection.MyContent.MyEntry.MyDocument = Document.Get(LibDocList[ppCmbxLibDoc.SelectedIndex].DocID);
|
|
ppBtnCvrtToLibDoc.Enabled = true;
|
|
}
|
|
else
|
|
{
|
|
throw new Exception(string.Format("Index Out Of Range Index = {0}, Count = {1}", ppCmbxLibDoc.SelectedIndex, LibDocList.Count));
|
|
}
|
|
}
|
|
}
|
|
#endregion
|
|
|
|
#region View Settings tab
|
|
|
|
/// <summary>
|
|
/// This is the View Settings button used on the button interface design
|
|
/// </summary>
|
|
/// <param name="sender">object</param>
|
|
/// <param name="e">EventArgs</param>
|
|
private void btnAutomation_Click(object sender, EventArgs e)
|
|
{
|
|
ProcessButtonClick(tiAutomation, btnAutomation);
|
|
}
|
|
|
|
#endregion
|
|
|
|
#region Generic functions used on this property page
|
|
|
|
/// <summary>
|
|
/// Determines what labels (showing default values) are visable on the property pages
|
|
/// </summary>
|
|
private void defaultSettingsVisiblity()
|
|
{
|
|
ppLblDefSettingsInfo.Visible = ppCbShwDefSettings.Checked;
|
|
ppLblFormatDefault.Visible = ppCbShwDefSettings.Checked && ppBtnDefaultFmt.Visible;
|
|
ppLblDefaultNumColumns.Visible = _isStepSection && ppCbShwDefSettings.Checked && ppBtnDefaultNumColumns.Visible;
|
|
ppLblDefPaginationStyle.Visible = _isStepSection && ppCbShwDefSettings.Checked && ppBtnDefaultPaginationStyle.Visible;
|
|
//ppLblDefaultPrintSize.Visible = !_isStepSection && ppCbShwDefSettings.Checked && ppBtnDefaultPrintSize.Visible;
|
|
|
|
}
|
|
|
|
/// <summary>
|
|
/// For the Button Interface property page style, when a button is selected (pressed),
|
|
/// it will remain in the checked state even when a different button is selected. Thus
|
|
/// we must clear the checked state of the buttons when a button is selected, then set
|
|
/// the newly selected button's state to checked.
|
|
/// </summary>
|
|
private void ClearAllCheckedButtons()
|
|
{
|
|
btnGeneral.Checked = false;
|
|
btnFormat.Checked = false;
|
|
btnLibDocs.Checked = false;
|
|
btnAutomation.Checked = false;
|
|
}
|
|
|
|
/// <summary>
|
|
/// Select the corresponding tab and set the button's state to checked
|
|
/// </summary>
|
|
/// <param name="tab">Property Page Tab</param>
|
|
/// <param name="button">Corresponding Property Page Button</param>
|
|
private void ProcessButtonClick(TabItem tab, ButtonX button)
|
|
{
|
|
ClearAllCheckedButtons();
|
|
tcSectionProp.SelectedTab = tab;
|
|
button.Checked = true;
|
|
}
|
|
|
|
/// <summary>
|
|
/// This is a generic Enter Event function for use with all of the property page tabs.
|
|
/// Found that the visiblity value of buttons is not recorded until the property page in which it resides is diplayed.
|
|
/// Thus we need to call defaultSettingVisiblity() to check and set visiblity states.
|
|
/// </summary>
|
|
/// <param name="sender"> type object</param>
|
|
/// <param name="e">type EventArgs</param>
|
|
private void tabpage_Enter(object sender, EventArgs e)
|
|
{
|
|
// Show or hide the labels containing the default values
|
|
if (!_Initializing)
|
|
defaultSettingsVisiblity();
|
|
}
|
|
/// <summary>
|
|
/// Process a change in the combo box selection
|
|
/// </summary>
|
|
/// <param name="cmbx">Combo Box Name</param>
|
|
/// <param name="defstr">string containing default text</param>
|
|
/// <param name="button">button to reset to default value</param>
|
|
/// <param name="deflabel">label containing the default</param>
|
|
private void ProcessCmbxSelectedValueChange(ComboBoxEx cmbx, string defstr, ButtonX button, Label deflabel)
|
|
{
|
|
if ((cmbx.SelectedIndex != -1) && defstr != null && defstr.Equals(cmbx.SelectedValue))
|
|
{
|
|
button.Visible = true;
|
|
button.Focus();
|
|
button.PerformClick();
|
|
}
|
|
button.Visible = (cmbx.SelectedValue != null);
|
|
deflabel.Visible = (ppCbShwDefSettings.Checked) && button.Visible;
|
|
}
|
|
/// <summary>
|
|
/// Process a change in the enum combo box selection
|
|
/// </summary>
|
|
/// <param name="cmbx">Combo Box Name</param>
|
|
/// <param name="defstr">the default enum value</param>
|
|
/// <param name="button">button to reset to default value</param>
|
|
/// <param name="deflabel">label containing the default</param>
|
|
private void ProcessCmbxSelectionEnumChanged(ComboBoxEx cmbx, object enumval, ButtonX button, Label deflabel)
|
|
{
|
|
if ((cmbx.SelectedIndex != -1) &&
|
|
cmbx.SelectedValue.Equals(enumval))
|
|
{
|
|
_Initializing = true;
|
|
button.Visible = true;
|
|
button.Focus();
|
|
button.PerformClick();
|
|
cmbx.SelectedIndex = -1; // This will hide the Default button
|
|
_Initializing = false;
|
|
}
|
|
//button.Visible = ((!_FolderConfig.Name.Equals("VEPROMS")) && (cmbx.SelectedValue != null));
|
|
button.Visible = ((cmbx.SelectedValue != null) && (cmbx.SelectedIndex >= 0));
|
|
deflabel.Visible = ppCbShwDefSettings.Checked && button.Visible;
|
|
}
|
|
#endregion
|
|
|
|
private void ppSectNumberStpRTB_Enter(object sender, EventArgs e)
|
|
{
|
|
_MyStepTabRibbon.MyStepRTB = ppSectNumberStpRTB;
|
|
}
|
|
|
|
private void ppSectTitleStpRTB_Enter(object sender, EventArgs e)
|
|
{
|
|
_MyStepTabRibbon.MyStepRTB = ppSectTitleStpRTB;
|
|
}
|
|
|
|
private void ShowAvailableOptionsForSectionType()
|
|
{
|
|
lblColumns.Visible = _isStepSection;
|
|
ppCmbxNumColumns.Visible = _isStepSection;
|
|
lblPagination.Visible = _isStepSection;
|
|
ppCmbxSectPagination.Visible = _isStepSection;
|
|
//lblPrintSize.Visible = !_isStepSection;
|
|
//ppCmbxAccPgPrintSize.Visible = !_isStepSection;
|
|
ppGpbxSignoffCheckoff.Visible = _isStepSection;
|
|
}
|
|
|
|
private void rbStepSect_CheckedChanged(object sender, EventArgs e)
|
|
{
|
|
_isStepSection = rbStepSect.Checked;
|
|
rbWordSect.Checked = !rbStepSect.Checked;
|
|
if (!_Initializing)
|
|
{
|
|
SetupPpCmbxSectionType();
|
|
ShowAvailableOptionsForSectionType();
|
|
tcpLibDoc.Enabled = !_isStepSection;
|
|
}
|
|
}
|
|
|
|
private void ppCmbxSectPagination_SelectedValueChanged(object sender, EventArgs e)
|
|
{
|
|
SectionConfig.SectionPagination secpgn = (SectionConfig.SectionPagination)Enum.Parse(typeof(SectionConfig.SectionPagination), _DefaultPaginationStyle);
|
|
ProcessCmbxSelectionEnumChanged(ppCmbxSectPagination, secpgn, ppBtnDefaultPaginationStyle, ppLblDefPaginationStyle);
|
|
tcpFormat.Focus();
|
|
}
|
|
|
|
private void ppCmbxNumColumns_SelectedValueChanged(object sender, EventArgs e)
|
|
{
|
|
SectionConfig.SectionColumnMode seccols = (SectionConfig.SectionColumnMode)Enum.Parse(typeof(SectionConfig.SectionColumnMode), _DefaultNumColumns);
|
|
ProcessCmbxSelectionEnumChanged(ppCmbxNumColumns, seccols, ppBtnDefaultNumColumns, ppLblDefaultNumColumns);
|
|
tcpFormat.Focus();
|
|
}
|
|
|
|
//private void ppCmbxAccPgPrintSize_SelectedValueChanged(object sender, EventArgs e)
|
|
//{
|
|
// SectionConfig.AttPrintSize attsize = (SectionConfig.AttPrintSize)Enum.Parse(typeof(SectionConfig.AttPrintSize), _DefaultPrintSize);
|
|
// ProcessCmbxSelectionEnumChanged(ppCmbxAccPgPrintSize, attsize, ppBtnDefaultPrintSize, ppLblDefaultPrintSize);
|
|
//}
|
|
|
|
private void ppBtnDefaultPaginationStyle_Click(object sender, EventArgs e)
|
|
{
|
|
// Get the parent setting
|
|
SectionConfig.SectionPagination secpgn = (SectionConfig.SectionPagination)Enum.Parse(typeof(SectionConfig.SectionPagination), _DefaultPaginationStyle);
|
|
// Compare parent setting with current setting
|
|
if (secpgn != _SectionConfig.Section_Pagination)
|
|
_SectionConfig.Section_Pagination = secpgn; // this will force a database update (write)
|
|
ppCmbxSectPagination.SelectedIndex = -1;//reset to the default Section Pagination Style setting
|
|
tcpFormat.Focus();
|
|
}
|
|
|
|
private void ppBtnDefaultNumColumns_Click(object sender, EventArgs e)
|
|
{
|
|
// Get the parent setting
|
|
SectionConfig.SectionColumnMode seccols = (SectionConfig.SectionColumnMode)Enum.Parse(typeof(SectionConfig.SectionColumnMode), _DefaultNumColumns);
|
|
// Compare parent setting with current setting
|
|
if (seccols != _SectionConfig.Section_ColumnMode)
|
|
_SectionConfig.Section_ColumnMode = seccols; // this will force a database update (write)
|
|
ppCmbxNumColumns.SelectedIndex = -1;//reset to the default Number of Columns setting
|
|
tcpFormat.Focus();
|
|
}
|
|
|
|
private void frmSectionProperties_Shown(object sender, EventArgs e)
|
|
{
|
|
ppSectNumberStpRTB.Focus();
|
|
}
|
|
|
|
//private void ppBtnDefaultPrintSize_Click(object sender, EventArgs e)
|
|
//{
|
|
// // Get the parent setting
|
|
// SectionConfig.AttPrintSize attsize = (SectionConfig.AttPrintSize)Enum.Parse(typeof(SectionConfig.AttPrintSize), _DefaultPrintSize);
|
|
// // Compare parent setting with current setting
|
|
// if (attsize != _SectionConfig.Section_AttachmentPrintSize)
|
|
// _SectionConfig.Section_AttachmentPrintSize = attsize; // this will force a database update (write)
|
|
// ppCmbxAccPgPrintSize.SelectedIndex = -1;//reset to the default Accessory page Print Size setting
|
|
// tcpFormat.Focus();
|
|
//}
|
|
}
|
|
} |