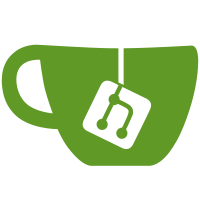
Pass the frmLoader to the ROFixer object so that status can be displayed as steps are processed Fixed MyValue so that unchanged values will not be displayed as if they had changed. Added Status outut as changed RO Values are found. Display a MessageBox when processing is complete.
127 lines
3.8 KiB
C#
127 lines
3.8 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using VEPROMS.CSLA.Library;
|
|
using System.Windows.Forms;
|
|
|
|
namespace DataLoader
|
|
{
|
|
public delegate void ROFixerEvent(object sender, ROFixerEventArgs args);
|
|
public class ROFixerEventArgs
|
|
{
|
|
private string _MyStatus;
|
|
|
|
public string MyStatus
|
|
{
|
|
get { return _MyStatus; }
|
|
set { _MyStatus = value; }
|
|
}
|
|
public ROFixerEventArgs(string myStatus)
|
|
{
|
|
_MyStatus = myStatus;
|
|
}
|
|
}
|
|
class ROFixer
|
|
{
|
|
public static readonly log4net.ILog _MyLog = log4net.LogManager.GetLogger(System.Reflection.MethodBase.GetCurrentMethod().DeclaringType);
|
|
public event ROFixerEvent StatusChanged;
|
|
private void OnStatusChanged(object sender, ROFixerEventArgs args)
|
|
{
|
|
if (StatusChanged != null)
|
|
StatusChanged(sender, args);
|
|
}
|
|
private string _Status;
|
|
|
|
public string Status
|
|
{
|
|
get { return _Status; }
|
|
set
|
|
{
|
|
_Status = value;
|
|
OnStatusChanged(this, new ROFixerEventArgs(_Status));
|
|
}
|
|
}
|
|
private int _ErrorCount = 0;
|
|
|
|
public int ErrorCount
|
|
{
|
|
get { return _ErrorCount; }
|
|
set { _ErrorCount = value; }
|
|
}
|
|
private string _LogPath;
|
|
private frmLoader frmMain;
|
|
public ROFixer(string logpath, frmLoader myfrmMain)
|
|
{
|
|
_LogPath = logpath;
|
|
frmMain = myfrmMain;
|
|
}
|
|
public TimeSpan Process()
|
|
{
|
|
DateTime tstart = DateTime.Now;
|
|
ProcessROs();
|
|
return DateTime.Now - tstart;
|
|
}
|
|
private void ProcessROs()
|
|
{
|
|
int changeCount = 0;
|
|
Status = "Getting List...";
|
|
// get list of content records
|
|
List<int> myContentList = new List<int>();
|
|
RoUsageInfoList myRoUsages = RoUsageInfoList.Get();
|
|
Dictionary<int, ROFSTLookup> roFstLookups = new Dictionary<int,ROFSTLookup>();
|
|
foreach (RoUsageInfo rou in myRoUsages)
|
|
{
|
|
if (!myContentList.Contains(rou.ContentID))
|
|
{
|
|
myContentList.Add(rou.ContentID);
|
|
}
|
|
}
|
|
int i = 0;
|
|
foreach (int cid in myContentList)
|
|
{
|
|
Status = string.Format("Processing {0} of {1} steps", ++i, myContentList.Count);
|
|
ContentInfo myContentInfo = ContentInfo.Get(cid);
|
|
DocVersionInfo dvi = myContentInfo.ContentItems[0].MyProcedure.MyDocVersion;
|
|
int versionId = dvi.VersionID;
|
|
if (!roFstLookups.ContainsKey(versionId))
|
|
{
|
|
ROFstInfo myRoFst = dvi.DocVersionAssociations[0].MyROFst;
|
|
roFstLookups.Add(versionId, myRoFst.GetROFSTLookup(dvi));
|
|
}
|
|
ROFSTLookup myLookup = roFstLookups[versionId];
|
|
using (Content ctmp = myContentInfo.Get())
|
|
{
|
|
ItemInfo ii = myContentInfo.ContentItems[0];
|
|
foreach (RoUsageInfo ru in myContentInfo.ContentRoUsages)
|
|
{
|
|
//ROFSTLookup.rochild rocc = myLookup.GetRoChild12(ru.ROID);
|
|
//if (rocc.value == null)
|
|
ROFSTLookup.rochild rocc = myLookup.GetRoChild(ru.ROID);
|
|
//string myValue = rocc.value;
|
|
|
|
int myType = rocc.type;
|
|
string myValue = myLookup.GetTranslatedRoValue(ru.ROID, ii.ActiveFormat.PlantFormat.FormatData.SectData.ConvertCaretToDelta);
|
|
myValue = myValue.Replace(@"\up2 \u8209?", @"\up2\u8209?");// Remove space between superscript command and non-breaking hyphen
|
|
myValue = myValue.Replace("\n", "");// Remove newlines in Figure data
|
|
string oldval = ctmp.FixContentText(ru, myValue, myType, null);
|
|
if (ctmp.IsDirty)
|
|
{
|
|
changeCount++;
|
|
//Console.WriteLine("'{0}', '{1}', '{2}', '{3}'", replace(oldval, @"\u8209?", "-"), replace(myValue, @"\u8209?", "-"), ru.ROID, rocc.appid);
|
|
frmMain.AddInfo("'{0}','{1}','R{2}'", (oldval??"").Replace(@"\u8209?", "-"), myValue.Replace(@"\u8209?", "-"), ru.ROID);
|
|
}
|
|
}
|
|
if (ctmp.IsDirty)
|
|
{
|
|
ctmp.DTS = DateTime.Now;
|
|
ctmp.Save();
|
|
ContentInfo.Refresh(ctmp);
|
|
}
|
|
}
|
|
}
|
|
frmMain.AddInfo("{0} RO Values Updated", changeCount);
|
|
MessageBox.Show(String.Format("{0} RO Values Updated", changeCount), "RO Value Update Complete", MessageBoxButtons.OK, MessageBoxIcon.Information);
|
|
}
|
|
}
|
|
}
|