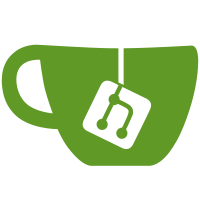
Changed background for border selection Draw Background and Borders for empty cells Set the Borders druing Grid conversion
127 lines
3.5 KiB
C#
127 lines
3.5 KiB
C#
using System;
|
|
using System.ComponentModel;
|
|
using System.Collections.Generic;
|
|
using System.Diagnostics;
|
|
using System.Text;
|
|
using System.Drawing;
|
|
using System.Windows.Forms;
|
|
|
|
namespace Volian.Controls.Library
|
|
{
|
|
public partial class BorderListBox : ListBox
|
|
{
|
|
#region Properties
|
|
public GridLinePattern SelectedLinePattern
|
|
{
|
|
get { return (Items[SelectedIndex] as GridLBItem).LinePattern; }
|
|
}
|
|
#endregion
|
|
#region ctor
|
|
public BorderListBox()
|
|
{
|
|
InitializeComponent();
|
|
SetupOptions();
|
|
}
|
|
public BorderListBox(IContainer container)
|
|
{
|
|
container.Add(this);
|
|
InitializeComponent();
|
|
SetupOptions();
|
|
}
|
|
private void SetupOptions()
|
|
{
|
|
this.DrawMode = DrawMode.OwnerDrawFixed;
|
|
//this.Font.Size;
|
|
DrawItem += new DrawItemEventHandler(BorderListBox_DrawItem);
|
|
//MeasureItem += new MeasureItemEventHandler(BorderListBox_MeasureItem);
|
|
Items.Add(new GridLBItem(GridLinePattern.None));
|
|
Items.Add(new GridLBItem(GridLinePattern.Single));
|
|
Items.Add(new GridLBItem(GridLinePattern.Double));
|
|
Items.Add(new GridLBItem(GridLinePattern.Thick));
|
|
Items.Add(new GridLBItem(GridLinePattern.Dashed));
|
|
Items.Add(new GridLBItem(GridLinePattern.Dotted));
|
|
SelectedIndex = 1;
|
|
}
|
|
public void SetupFontAndSize(int fontSize)
|
|
{
|
|
Font = new Font(Font.FontFamily, fontSize, Font.Style);
|
|
using (Graphics gr = CreateGraphics())
|
|
{
|
|
SizeF mySize = gr.MeasureString("Almg", Font);
|
|
ItemHeight = (int)Math.Ceiling(mySize.Height);
|
|
}
|
|
int newHeight = Items.Count * ItemHeight + 4;
|
|
Size = new Size(newHeight, newHeight);
|
|
}
|
|
#endregion
|
|
#region Event Handlers
|
|
//void BorderListBox_MeasureItem(object sender, MeasureItemEventArgs e)
|
|
//{
|
|
// e.ItemHeight = 22;
|
|
//}
|
|
private int _MaxLabelWidth = 0;
|
|
private int MaxLabelWidth(Graphics gr)
|
|
{
|
|
if(_MaxLabelWidth == 0)
|
|
{
|
|
for(int i=0;i<Items.Count;i++)
|
|
_MaxLabelWidth = Math.Max(_MaxLabelWidth,(int) gr.MeasureString("XX" + Items[0].ToString(), Font).Width);
|
|
}
|
|
return _MaxLabelWidth;
|
|
}
|
|
private void BorderListBox_DrawItem(object sender, DrawItemEventArgs e)
|
|
{
|
|
e.DrawBackground();
|
|
e.DrawFocusRectangle();
|
|
int y = (e.Bounds.Top + e.Bounds.Bottom) / 2;
|
|
GridLBItem myGridLBItem = Items[e.Index] as GridLBItem;
|
|
Brush myBrush = new SolidBrush(e.ForeColor);
|
|
e.Graphics.DrawString(myGridLBItem.LinePattern.ToString(), Font, myBrush, e.Bounds);
|
|
Pen pn = VlnBorders.LinePen(myGridLBItem.LinePattern, e.ForeColor);
|
|
int leftMargin = MaxLabelWidth(e.Graphics);
|
|
int rightMargin = 4;
|
|
switch (myGridLBItem.LinePattern)
|
|
{
|
|
case GridLinePattern.Double:
|
|
e.Graphics.DrawLine(pn, e.Bounds.Left + leftMargin, y + 1, e.Bounds.Right - rightMargin, y + 1);
|
|
e.Graphics.DrawLine(pn, e.Bounds.Left + leftMargin, y - 1, e.Bounds.Right - rightMargin, y - 1);
|
|
break;
|
|
case GridLinePattern.None:
|
|
break;
|
|
case GridLinePattern.Single:
|
|
case GridLinePattern.Thick:
|
|
case GridLinePattern.Dotted:
|
|
case GridLinePattern.Dashed:
|
|
case GridLinePattern.Mixed:
|
|
default:
|
|
e.Graphics.DrawLine(pn, e.Bounds.Left + leftMargin, y, e.Bounds.Right - rightMargin, y);
|
|
break;
|
|
}
|
|
}
|
|
#endregion
|
|
}
|
|
public class GridLBItem
|
|
{
|
|
#region Properties
|
|
private GridLinePattern _LinePattern;
|
|
public GridLinePattern LinePattern
|
|
{
|
|
get { return _LinePattern; }
|
|
set { _LinePattern = value; }
|
|
}
|
|
#endregion
|
|
#region ctor
|
|
public GridLBItem(GridLinePattern linePattern)
|
|
{
|
|
LinePattern = linePattern;
|
|
}
|
|
#endregion
|
|
#region Public Methods
|
|
public override string ToString()
|
|
{
|
|
return LinePattern.ToString();
|
|
}
|
|
#endregion
|
|
}
|
|
}
|