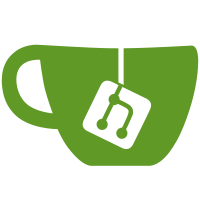
Use Command Line Parameters ass override for settings. Add logic to support partial processing of procedure sets (appending to a previously processed set.
178 lines
7.2 KiB
C#
178 lines
7.2 KiB
C#
// ========================================================================
|
|
// Copyright 2006 - Volian Enterprises, Inc. All rights reserved.
|
|
// Volian Enterprises - Proprietary Information - DO NOT COPY OR DISTRIBUTE
|
|
// ------------------------------------------------------------------------
|
|
// $Workfile: $ $Revision: $
|
|
// $Author: $ $Date: $
|
|
//
|
|
// $History: $
|
|
// ========================================================================
|
|
using System;
|
|
using System.Drawing;
|
|
using System.Collections;
|
|
using System.ComponentModel;
|
|
using System.Windows.Forms;
|
|
using System.Data;
|
|
using System.Data.OleDb;
|
|
using System.Collections.Specialized;
|
|
using System.Collections.Generic;
|
|
using System.Xml;
|
|
using System.IO;
|
|
using System.Text;
|
|
using VEPROMS.CSLA.Library;
|
|
|
|
namespace DataLoader
|
|
{
|
|
public partial class Loader
|
|
{
|
|
public TimeSpan MigrateDocVersion(DocVersion docver)
|
|
{
|
|
return MigrateDocVersion(docver, true);
|
|
}
|
|
|
|
private OutsideTransition _OutTran;
|
|
public int MultiUnitCount = 0;
|
|
public Dictionary<int, int> Old2NewApple;
|
|
public Dictionary<int, int> New2OldApple;
|
|
|
|
public TimeSpan MigrateDocVersion(DocVersion docver, bool convertProcedures)
|
|
{
|
|
long lTime = DateTime.Now.Ticks;
|
|
string pth = docver.Title;
|
|
// if the Title is empty, return because this docversion has already been migrated.
|
|
bool appending = false;
|
|
if (frmMain.Automatic && !frmMain.PurgeExistingData)
|
|
{
|
|
pth = frmMain.ProcedureSetPath;
|
|
appending = true;
|
|
}
|
|
if (pth == null || pth == "" || pth == "Title") return TimeSpan.FromTicks(0);
|
|
MultiUnitCount = docver.DocVersionConfig.Unit_Count;
|
|
if (MultiUnitCount > 0)
|
|
{
|
|
Old2NewApple = new Dictionary<int, int>();
|
|
New2OldApple = new Dictionary<int, int>();
|
|
for (int i = 1; i <= MultiUnitCount; i++)
|
|
{
|
|
docver.DocVersionConfig.SelectedSlave = i;
|
|
int oldindex = int.Parse(docver.DocVersionConfig.Old_Index);
|
|
Old2NewApple.Add(oldindex, i);
|
|
New2OldApple.Add(i, oldindex);
|
|
}
|
|
}
|
|
if (!File.Exists(pth + @"\set.dbf") || !File.Exists(pth + @"\curset.dat")) return TimeSpan.FromTicks(0);
|
|
// Open connection
|
|
OleDbConnection cn = new OleDbConnection(@"Provider=Microsoft.Jet.OLEDB.4.0;Data Source=" + pth + ";Extended Properties=dBase III;Persist Security Info=False");
|
|
if (convertProcedures)
|
|
{
|
|
// JSJ 02/10/2010 - Remove all the .INF files before proceeding to convert.
|
|
// The INF files contain a path to a corresponding NDX file - had data with bat NDX path
|
|
DeleteINFFiles(pth);
|
|
_OutTran = new OutsideTransition(cn);
|
|
frmMain.AddInfo("Before MigrateROFST{0}\r\n{1}", GC.GetTotalMemory(true), VEPROMS.CSLA.Library.CSLACache.UsageAll);
|
|
//if(!appending)
|
|
MigrateROFST(pth, docver);
|
|
GC.Collect();
|
|
frmMain.AddInfo("After MigrateROFST{0}\r\n{1}", GC.GetTotalMemory(true), VEPROMS.CSLA.Library.CSLACache.UsageAll);
|
|
// Migrate library documents
|
|
//if(!appending)
|
|
MigrateLibDocs(cn, pth, appending);
|
|
|
|
// if migrating bge, save the 'unit' number. This unit number is used in pagelist items and is
|
|
// based on the path/directory name of the procedure set.
|
|
if (true) // somehow check for BGE
|
|
{
|
|
SetDocVersionUnitConfig(pth, docver);
|
|
}
|
|
|
|
|
|
// Initialize Dictionaries
|
|
dicTrans_ItemDone = new Dictionary<string, Item>();
|
|
dicTrans_ItemIds = new Dictionary<string, Item>();
|
|
dicTrans_MigrationErrors = new Dictionary<string, List<Item>>();
|
|
|
|
// Create a 'dummy' content record. This will be used for any transitions 'to'
|
|
// that don't exist when dbf is processed. At end, use this to see if there
|
|
// are missing transitions.
|
|
TransDummyCont = Content.MakeContent(null, "DUMMY CONTENT FOR TRANSITION MIGRATION", null, null, null);
|
|
}
|
|
// Process Procedures
|
|
Item itm = null;
|
|
if (convertProcedures || docver.VersionType == (int)VEPROMS.CSLA.Library.VersionTypeEnum.WorkingDraft || docver.VersionType == (int)VEPROMS.CSLA.Library.VersionTypeEnum.Approved)
|
|
{
|
|
ResetApplesToOranges();
|
|
itm = MigrateProcedures(cn, pth, docver, convertProcedures, docver.MyDocVersionInfo.ActiveFormat);
|
|
}
|
|
// Show any Missing Transtitons (i.e. Transitions which have not been processed)
|
|
lTime = DateTime.Now.Ticks - lTime;
|
|
if (convertProcedures)
|
|
{
|
|
ShowMissingTransitions(docver);
|
|
//frmMain.Status = string.Format("{0}\r\nConversion completed in {1} seconds.", pth, TimeSpan.FromTicks(lTime).TotalSeconds);
|
|
TimeSpan ts = TimeSpan.FromTicks(lTime);
|
|
frmMain.Status = string.Format("{0}\r\nConversion completion time: {1:D2}:{2:D2}:{3:D2}.{4} ({5} Total Seconds)", pth, ts.Hours, ts.Minutes, ts.Seconds, ts.Milliseconds, ts.TotalSeconds);
|
|
log.InfoFormat("Completed Migration of {0}", pth);
|
|
|
|
//MessageBox.Show("Completed Migration of " + pth); //jsj commented out to let it run through
|
|
if (rofstinfo != null) rofstinfo.GetROFSTLookup(DocVersionInfo.Get(docver.VersionID)).Close();
|
|
dicTrans_ItemDone.Clear();
|
|
dicTrans_ItemDone = null;
|
|
}
|
|
else
|
|
frmMain.Status = string.Format("{0}\r\nDone.", pth);
|
|
cn.Close();
|
|
if (itm != null)
|
|
{
|
|
docver.MyItem = itm;
|
|
if (convertProcedures) docver.Title = ""; // clearing this tell us this docver (path) was converted?
|
|
if (!docver.IsSavable) ErrorRpt.ErrorReport(docver);
|
|
//if(frmMain.MySettings.ExecutionMode == ExecutionMode.Debug)
|
|
// docver.DocVersionConfig.Print_PDFLocation = frmMain.MySettings.PDFFolder;
|
|
docver.Save();
|
|
}
|
|
return TimeSpan.FromTicks(lTime);
|
|
}
|
|
|
|
private void SetDocVersionUnitConfig(string pth, DocVersion docver)
|
|
{
|
|
// the following 'rules' were taken from 16bit code, in pagelist.c, to define the unit number.
|
|
|
|
string unitNum = "0";
|
|
string testPath = pth.ToUpper();
|
|
|
|
// back up further if this is a tmpchg or approved directory.
|
|
int lastPart = pth.LastIndexOf(@"\");
|
|
if (lastPart != -1)
|
|
{
|
|
testPath = testPath.Substring(testPath.LastIndexOf(@"\"));
|
|
if (testPath.Contains("TMPCHG") || testPath.Contains("APPROVED"))
|
|
testPath = testPath.Substring(testPath.LastIndexOf(@"\", lastPart));
|
|
}
|
|
if (testPath.IndexOf("PROCS2") > -1 || testPath.IndexOf("2.PRC") > -1)
|
|
unitNum = "2";
|
|
else if (testPath.IndexOf("PROCS") > -1 || testPath.IndexOf("1.PRC") > -1)
|
|
unitNum = "1";
|
|
else if (testPath.IndexOf("3.PRC") > -1)
|
|
unitNum = "3";
|
|
else if (testPath.IndexOf("4.PRC") > -1)
|
|
unitNum = "4";
|
|
|
|
docver.DocVersionConfig.Print_UnitNumberForPageList = unitNum;
|
|
docver.Save();
|
|
}
|
|
private void DeleteINFFiles(string pth)
|
|
{
|
|
DirectoryInfo di = new DirectoryInfo(pth);
|
|
FileInfo [] myFiles = di.GetFiles("*.INF");
|
|
foreach (FileInfo myFile in myFiles)
|
|
myFile.Delete();
|
|
}
|
|
private VEPROMS.CSLA.Library.VersionTypeEnum DocVersionType(string s)
|
|
{
|
|
if (s.EndsWith("approved")) return VEPROMS.CSLA.Library.VersionTypeEnum.Approved;
|
|
if (s.EndsWith("chgsht")) return VEPROMS.CSLA.Library.VersionTypeEnum.Revision;
|
|
if (s.EndsWith("tmpchg")) return VEPROMS.CSLA.Library.VersionTypeEnum.Temporary;
|
|
return VEPROMS.CSLA.Library.VersionTypeEnum.WorkingDraft;
|
|
}
|
|
}
|
|
} |