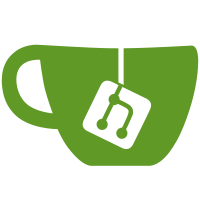
Changed the format of the Verical Ruler in the Debug PDF Layer to start at the top margin and extend to the bottom margin. Partial fix of the logic to display an XY Plot.
58 lines
1.8 KiB
C#
58 lines
1.8 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.ComponentModel;
|
|
using System.Data;
|
|
using System.Drawing;
|
|
using System.Drawing.Printing;
|
|
using System.Drawing.Imaging;
|
|
using System.Text;
|
|
using System.Windows.Forms;
|
|
using System.Diagnostics;
|
|
using System.IO;
|
|
using VG;
|
|
//using XYPlots;
|
|
|
|
namespace XYPlots
|
|
{
|
|
public partial class frmXYPlot : Form
|
|
{
|
|
private string _XYPlot;
|
|
private string _Title;
|
|
public frmXYPlot(string title,string xyPlot)
|
|
{
|
|
InitializeComponent();
|
|
int pstart = xyPlot.IndexOf("<<G"); // find the starting Plot Command
|
|
xyPlot = xyPlot.Substring(pstart); // set val to the start of the plot commands
|
|
_XYPlot =xyPlot;
|
|
_Title = title;
|
|
}
|
|
private void pnl_Paint(object sender, PaintEventArgs e)
|
|
{
|
|
e.Graphics.SmoothingMode = System.Drawing.Drawing2D.SmoothingMode.AntiAlias;
|
|
e.Graphics.TextRenderingHint = System.Drawing.Text.TextRenderingHint.AntiAlias;
|
|
XYPlots.XYPlot myPlot = new XYPlots.XYPlot(_XYPlot);
|
|
myPlot.SetMargins(.75F, .5F, .25F, .75F);
|
|
float scaleX = pnl.Width / myPlot.Width;
|
|
float scaleY = pnl.Height / myPlot.Height;
|
|
float scale = scaleX > scaleY ? scaleY : scaleX;
|
|
e.Graphics.ScaleTransform(scale, scale);
|
|
myPlot.Process(new VGOut_Graphics(e.Graphics));
|
|
}
|
|
private void pnl_Resize(object sender, EventArgs e)
|
|
{
|
|
pnl.Invalidate();
|
|
}
|
|
private void frmXYPlot_Load(object sender, EventArgs e)
|
|
{
|
|
Text = _Title;
|
|
XYPlots.XYPlot myPlot = new XYPlots.XYPlot(_XYPlot);
|
|
myPlot.SetMargins(.75F, .5F, .25F, .75F);
|
|
float scaleX = pnl.Width / myPlot.Width;
|
|
float scaleY = pnl.Height / myPlot.Height;
|
|
float scale = scaleX > scaleY ? scaleY : scaleX;
|
|
int height = (int)(Height - pnl.Height + pnl.Height * scale / scaleY);
|
|
int width = (int)(Width - pnl.Width + pnl.Width * scale / scaleX);
|
|
Size = new Size(width, height);
|
|
}
|
|
}
|
|
} |