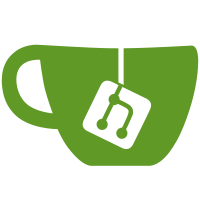
Correct dispose logic for transition destination ITEMs Correct cursor position after Transition Insert Correct logic to parse Transition Link Token Correct Save Config to dispose of ITEM Removed debug vlnStackTrace
124 lines
3.6 KiB
C#
124 lines
3.6 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.Text;
|
|
using System.Text.RegularExpressions;
|
|
using VEPROMS.CSLA.Library;
|
|
namespace Volian.Controls.Library
|
|
{
|
|
public partial class LinkText
|
|
{
|
|
public LinkText(string linkInfoText)
|
|
{
|
|
_LinkInfoText = linkInfoText;
|
|
}
|
|
public void ParseLink()
|
|
{
|
|
if (_MyParsedLinkType == ParsedLinkType.NotParsed)
|
|
{
|
|
if (_LinkInfoText == null) return;
|
|
// First parse the string
|
|
if (_LinkInfoText.Contains(@"\v"))
|
|
throw new Exception("LinkText.ParseLink found RTF token");
|
|
//_LinkInfoText = _LinkInfoText.Replace(@"\v ", ""); // this is not needed because this is selected Text does not contain RTF tokens
|
|
// for tran : "2, #, #, # and 4#Link:TransitionRange:2 10 173 166"
|
|
Match m = Regex.Match(_LinkInfoText, @"(.*)[#]Link:([A-Za-z]*):(.*)");
|
|
_MyValue = m.Groups[1].Value;
|
|
_MyLink = "#Link:" + m.Groups[2].Value + ":" + m.Groups[3].Value;
|
|
switch (m.Groups[2].Value)
|
|
{
|
|
case "ReferencedObject":
|
|
_MyParsedLinkType = ParsedLinkType.ReferencedObject;
|
|
string[] subs = m.Groups[3].Value.Split(" ".ToCharArray());
|
|
if (subs[0].IndexOf("CROUSGID")>-1)
|
|
_MyRoUsageInfo = null;
|
|
else if (subs[0].IndexOf("NewID") > -1)
|
|
_MyRoUsageInfo = null;
|
|
else
|
|
{
|
|
int roUsageid = Convert.ToInt32(subs[0]);
|
|
_MyRoUsageInfo = RoUsageInfo.Get(roUsageid);
|
|
}
|
|
break;
|
|
case "Transition":
|
|
case "TransitionRange":
|
|
_MyParsedLinkType = (ParsedLinkType)Enum.Parse(_MyParsedLinkType.GetType(), m.Groups[2].Value);
|
|
string[] subst = m.Groups[3].Value.Split(" ".ToCharArray());
|
|
if (subst[1].IndexOf("CTID") > -1)
|
|
_MyTransitionInfo = null;
|
|
else if (subst[1].IndexOf("NewID") > -1)
|
|
_MyTransitionInfo = null;
|
|
else
|
|
{
|
|
int transitionID = Convert.ToInt32(m.Groups[3].Value.Split(" ".ToCharArray())[1]);
|
|
_MyTransitionInfo = TransitionInfo.Get(transitionID);
|
|
}
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
private string _LinkInfoText;
|
|
public string LinkInfoText
|
|
{
|
|
get { return _LinkInfoText; }
|
|
}
|
|
private TransitionInfo _MyTransitionInfo = null;
|
|
public TransitionInfo MyTransitionInfo
|
|
{
|
|
get { ParseLink(); return _MyTransitionInfo; }
|
|
}
|
|
public ItemInfo MyTranToItemInfo
|
|
{
|
|
get { ParseLink(); return _MyTransitionInfo.MyItemToID; }
|
|
}
|
|
public ItemInfo MyTranRangeItemInfo
|
|
{
|
|
get { ParseLink(); return _MyTransitionInfo.MyItemRangeID; }
|
|
}
|
|
private string _MyValue = null;
|
|
//public string MyValue
|
|
//{
|
|
// get { ParseLink(); return _MyValue; }
|
|
//}
|
|
private string _MyLink = null;
|
|
//public string MyLink
|
|
//{
|
|
// get { ParseLink(); return _MyLink; }
|
|
//}
|
|
private RoUsageInfo _MyRoUsageInfo;
|
|
public RoUsageInfo MyRoUsageInfo
|
|
{
|
|
get { ParseLink(); return _MyRoUsageInfo; }
|
|
}
|
|
//private string _Roid = null; // TODO: need to return Referenced Object rather than just roid
|
|
//public string Roid
|
|
//{
|
|
// get { ParseLink(); return _Roid; }
|
|
//}
|
|
//private string _RoUsageid = null; // TODO: need to return Referenced Object rather than just roid
|
|
//public string RoUsageid
|
|
//{
|
|
// get { ParseLink(); return _RoUsageid; }
|
|
//}
|
|
//private string _RoDbid = null; // TODO: need to return Referenced Object rather than just roid
|
|
//public string RoDbid
|
|
//{
|
|
// get { ParseLink(); return _RoDbid; }
|
|
//}
|
|
private ParsedLinkType _MyParsedLinkType = ParsedLinkType.NotParsed;
|
|
//public ParsedLinkType MyParsedLinkType
|
|
//{
|
|
// get { ParseLink(); return _MyParsedLinkType; }
|
|
//}
|
|
|
|
}
|
|
#region enums
|
|
public enum ParsedLinkType : int
|
|
{
|
|
NotParsed = 0,
|
|
Transition = 1,
|
|
TransitionRange = 2,
|
|
ReferencedObject = 3
|
|
}
|
|
#endregion
|
|
}
|