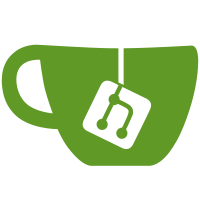
Added property settings WhatROsToConvert, SelectedROFst, AnnotateWhenConvertingToText, AnnotateWhenShowingMissingRO, and AnnotateWhenShowingDifferentRO. Added ROUpdateMode enum Changed how text is added to tbErrors textbox Added property settings WhatROsToConvert, SelectedROFst, AnnotateWhenConvertingToText, AnnotateWhenShowingMissingRO, and AnnotateWhenShowingDifferentRO Added createEmptyPROMS boolean value to allow creating an empty PROMS database Added loadTree menu item click event handler Added Load Tree menu item, Selection main menu with Fix ROs menu item under Selection menu item and Create Empty database menu item Used createEmptyPROMS value. Changed how Migration Error annotation type created.
101 lines
2.1 KiB
C#
101 lines
2.1 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.ComponentModel;
|
|
using System.Data;
|
|
using System.Drawing;
|
|
using System.Text;
|
|
using System.Windows.Forms;
|
|
using System.IO;
|
|
|
|
namespace DataLoader
|
|
{
|
|
public partial class frmErrors : Form
|
|
{
|
|
private Form _MyParent;
|
|
public frmErrors(Form myParent)
|
|
{
|
|
InitializeComponent();
|
|
_MyParent = myParent;
|
|
_MyParent.Move += new EventHandler(_MyParent_Move);
|
|
}
|
|
void _MyParent_Move(object sender, EventArgs e)
|
|
{
|
|
AdjustLocation();
|
|
}
|
|
private int _ErrorCount = 0;
|
|
public int ErrorCount
|
|
{
|
|
get { return _ErrorCount; }
|
|
set { _ErrorCount = value; }
|
|
}
|
|
private int _WarningCount = 0;
|
|
public int WarningCount
|
|
{
|
|
get { return _WarningCount; }
|
|
set { _WarningCount = value; }
|
|
}
|
|
private int _InfoCount = 0;
|
|
public int InfoCount
|
|
{
|
|
get { return _InfoCount; }
|
|
set { _InfoCount = value; }
|
|
}
|
|
string sep = "";
|
|
public void Add(string err, MessageType messageType)
|
|
{
|
|
switch (messageType)
|
|
{
|
|
case MessageType.Information:
|
|
_InfoCount++;
|
|
break;
|
|
case MessageType.Warning:
|
|
_WarningCount++;
|
|
break;
|
|
case MessageType.Error:
|
|
_ErrorCount++;
|
|
break;
|
|
default:
|
|
break;
|
|
}
|
|
//tbErrors.SelectionStart = tbErrors.TextLength;
|
|
//tbErrors.SelectedText = sep + err;
|
|
tbErrors.AppendText(sep + err);
|
|
sep = "\r\n";
|
|
Show();
|
|
Application.DoEvents();
|
|
}
|
|
public void Clear()
|
|
{
|
|
tbErrors.Text = "";
|
|
sep = "";
|
|
Hide();
|
|
}
|
|
private void frmErrors_Load(object sender, EventArgs e)
|
|
{
|
|
AdjustLocation();
|
|
}
|
|
private void AdjustLocation()
|
|
{
|
|
int maxRight = Screen.GetWorkingArea(this).Right;
|
|
if (Screen.AllScreens.Length > 1)
|
|
{
|
|
foreach (Screen sc in Screen.AllScreens)
|
|
if (sc.WorkingArea.Right > maxRight) maxRight = sc.WorkingArea.Right;
|
|
}
|
|
Top = _MyParent.Top;
|
|
Left = _MyParent.Right + Width > maxRight ? maxRight - Width : _MyParent.Right;
|
|
}
|
|
public void Save(string path)
|
|
{
|
|
StreamWriter fs = new StreamWriter(path, false);
|
|
fs.Write(tbErrors.Text);
|
|
fs.Close();
|
|
}
|
|
}
|
|
public enum MessageType
|
|
{
|
|
Information,
|
|
Warning,
|
|
Error
|
|
}
|
|
} |