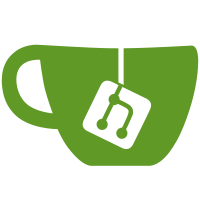
Added header information to xml for image link Replace bname with xlink:href for image references Added RNOWidthAdj flag for Braidwood
192 lines
6.9 KiB
C#
192 lines
6.9 KiB
C#
using System;
|
|
using System.Text;
|
|
using System.Xml;
|
|
using System.Drawing;
|
|
using System.Windows.Forms;
|
|
//using C1.C1Pdf;
|
|
|
|
namespace fmtxml
|
|
{
|
|
/// <summary>
|
|
/// Summary description for XmlToPdf.
|
|
/// </summary>
|
|
public class SvgToPdf
|
|
{
|
|
private float ConvertToPoints=72f;
|
|
private XmlDocument xmlDoc;
|
|
private string FileName;
|
|
private string FilePath;
|
|
//private C1.C1Pdf.C1PdfDocument _c1pdf;
|
|
|
|
private string [] FontChoice =
|
|
{"Times New Roman",
|
|
"VESymb XXXXXX",
|
|
"VolianDraw XXXXXX",
|
|
"Prestige Elite Tall",
|
|
"Courier New",
|
|
"Arial",
|
|
"Letter Gothic",
|
|
"Times New Roman",
|
|
"Letter Gothic Tall",
|
|
"Letter Gothic Tall",
|
|
"Gothic Ultra",
|
|
"VolianScript"
|
|
};
|
|
private float LeftMargin; //todo: define in page info
|
|
private float VerticalOffset; //todo: define in page info
|
|
private bool Portrait; //todo: define in page info
|
|
|
|
public SvgToPdf(string path, string fname)
|
|
{
|
|
LeftMargin=0.5f * ConvertToPoints; // KBR: TODO
|
|
VerticalOffset=0.5f * ConvertToPoints; // KBR: TODO
|
|
Portrait = true; // KBR: TODO
|
|
FileName = fname;
|
|
FilePath = path;
|
|
xmlDoc = new XmlDocument();
|
|
XmlTextReader reader = new XmlTextReader(FilePath+"\\"+FileName);
|
|
xmlDoc.Load(reader);
|
|
reader.Close();
|
|
try
|
|
{
|
|
GeneratePDFForFile();
|
|
}
|
|
catch (Exception e)
|
|
{
|
|
MessageBox.Show("Error processing: " + fname, e.Message);
|
|
}
|
|
}
|
|
|
|
private void GeneratePDFForFile()
|
|
{
|
|
//_c1pdf = new C1.C1Pdf.C1PdfDocument();
|
|
//string rootname = FileName.Substring(0,FileName.Length-4);
|
|
//// create a pdf file with a page for each macro (i.e. macro element in xml tree),
|
|
//// put it into the pdf file.
|
|
//XmlNode topnode = xmlDoc.LastChild;
|
|
//XmlNodeList ndlist = topnode.SelectNodes("/g");
|
|
//for (int i=0;i<topnode.ChildNodes.Count;i++)
|
|
//{
|
|
// XmlNode nd = topnode.ChildNodes[i];
|
|
// if (nd.Name!="desc")
|
|
// {
|
|
// SvgConvertToPdf(nd);
|
|
// _c1pdf.NewPage();
|
|
// }
|
|
//}
|
|
//string outname = "E:\\proms.net\\genmac.xml\\testpdf\\" + rootname + ".pdf";
|
|
//_c1pdf.Save(outname);
|
|
}
|
|
|
|
private string AddRTFBUI(string origStr, string bold, string underline, string italics)
|
|
{
|
|
if (bold==""&&underline==""&&italics=="")return origStr;
|
|
StringBuilder sb = new StringBuilder();
|
|
if (bold == "bold")sb.Append("{\\b");
|
|
if (underline == "underline")sb.Append("{\\u");
|
|
if (italics == "italics") sb.Append("{\\i");
|
|
sb.Append(" ");
|
|
sb.Append(origStr);
|
|
if (bold == "bold")sb.Append("}");
|
|
if (underline == "underline")sb.Append("}");
|
|
if (italics == "italics") sb.Append("}");
|
|
return sb.ToString();
|
|
}
|
|
|
|
private void SvgConvertToPdf(XmlNode nd)
|
|
{
|
|
for (int i=0; i<nd.ChildNodes.Count; i++)
|
|
{
|
|
XmlElement cmdele = (XmlElement) nd.ChildNodes[i];
|
|
string cmdline = cmdele.InnerXml;
|
|
string cmd = cmdele.Name.ToLower();
|
|
float fstx, fsty, fex, fey = 0;
|
|
float flw = 0;
|
|
// temporary for testing of negative placement (x/y) values...
|
|
XmlElement tstele = (XmlElement) nd;
|
|
if (tstele.GetAttribute("id")=="CNUM")
|
|
{
|
|
LeftMargin = 100.0f;
|
|
VerticalOffset = 100f;
|
|
}
|
|
Pen pn = null;
|
|
switch (cmd)
|
|
{
|
|
case "rect":
|
|
fstx = (float) System.Convert.ToDouble(cmdele.GetAttribute("x"));
|
|
fsty = (float) System.Convert.ToDouble(cmdele.GetAttribute("y"));
|
|
fex = (float) System.Convert.ToDouble(cmdele.GetAttribute("width"));
|
|
fey = (float) System.Convert.ToDouble(cmdele.GetAttribute("height"));
|
|
flw = (float) System.Convert.ToDouble(cmdele.GetAttribute("stroke-width"));
|
|
pn = new Pen(Brushes.Black,flw);
|
|
//KBR: TODO - storing page stuff such as portland, vertical offset, tab, etc.
|
|
RectangleF rc = new RectangleF(LeftMargin+fstx,VerticalOffset+fsty,fex,fey);
|
|
//_c1pdf.DrawRectangle(pn,rc);
|
|
break;
|
|
case "line": // command is LINE startx, starty, endx, endy, lnwidth
|
|
fstx = (float) System.Convert.ToDouble(cmdele.GetAttribute("x1"));
|
|
fsty = (float) System.Convert.ToDouble(cmdele.GetAttribute("y1"));
|
|
fex = (float) System.Convert.ToDouble(cmdele.GetAttribute("x2"));
|
|
fey = (float) System.Convert.ToDouble(cmdele.GetAttribute("y2"));
|
|
float flw1 = (float) System.Convert.ToDouble(cmdele.GetAttribute("stroke-width"));
|
|
//KBR: TODO - storing page stuff such as portland, vertical offset, tab, etc.
|
|
// startx = portland ? (vof + cy + adjy) : (cx + tab + adjx);
|
|
// starty = portland ? (tab - (cx + adjx)) : (cy + vof + adjy);
|
|
pn = new Pen(Brushes.Black,flw1);
|
|
//KBR: TODO - move ablsolute?
|
|
// if (MoveAbsolute)
|
|
// {tab = 0;vof = 0;}
|
|
//_c1pdf.DrawLine(pn, LeftMargin+fstx, VerticalOffset+fsty, LeftMargin+fex, VerticalOffset+fey);
|
|
break;
|
|
case "text":
|
|
fstx = (float) System.Convert.ToDouble(cmdele.GetAttribute("x"));
|
|
fsty = (float) System.Convert.ToDouble(cmdele.GetAttribute("y"));
|
|
int FontSize = System.Convert.ToInt32(cmdele.GetAttribute("font-size"));
|
|
string bold = cmdele.GetAttribute("font-weight");
|
|
string underline = cmdele.GetAttribute("text-decoration");
|
|
string italics = cmdele.GetAttribute("font-style");
|
|
Font myfont = new Font(cmdele.GetAttribute("font-family"),FontSize);
|
|
rc = new Rectangle();
|
|
//rc.Size = _c1pdf.MeasureStringRtf(cmdele.InnerText,myfont,500); // kbr todo: 500?
|
|
rc.X = LeftMargin+fstx;
|
|
rc.Y = VerticalOffset+fsty-FontSize;
|
|
// add bold, underline & italics for entire object.
|
|
string rtfout = AddRTFBUI(cmdele.InnerText,bold,underline,italics);
|
|
//_c1pdf.DrawStringRtf(rtfout,myfont,Brushes.Black,rc);
|
|
break;
|
|
case "gdiadj":
|
|
break;
|
|
case "ellipse":
|
|
fstx = (float) System.Convert.ToDouble(cmdele.GetAttribute("cx"));
|
|
fsty = (float) System.Convert.ToDouble(cmdele.GetAttribute("cy"));
|
|
fex = (float) System.Convert.ToDouble(cmdele.GetAttribute("rx"));
|
|
fey = (float) System.Convert.ToDouble(cmdele.GetAttribute("ry"));
|
|
flw = (float) System.Convert.ToDouble(cmdele.GetAttribute("stroke-width"));
|
|
pn = new Pen(Brushes.Black,flw);
|
|
//_c1pdf.DrawEllipse(pn,LeftMargin+fstx, VerticalOffset+fsty, fex, fey);
|
|
break;
|
|
case "image":
|
|
fstx = (float) System.Convert.ToDouble(cmdele.GetAttribute("x"));
|
|
fsty = (float) System.Convert.ToDouble(cmdele.GetAttribute("y"));
|
|
int scale=1;
|
|
if (cmdele.HasAttribute("scale")) scale = System.Convert.ToInt32(cmdele.GetAttribute("scale"));
|
|
string fname = cmdele.GetAttribute("xlink:href");
|
|
Bitmap bm = new Bitmap(fname);
|
|
Image img = Image.FromFile(fname);
|
|
rc = new RectangleF();
|
|
rc.Height = (((img.Height*scale)*4.8f)/1440f)*72f;
|
|
rc.Width = (((img.Width*scale)*4.8f)/1440f)*72f;
|
|
rc.X = LeftMargin+fstx;
|
|
rc.Y = VerticalOffset+fsty;
|
|
//_c1pdf.DrawImage(img, rc, ContentAlignment.MiddleCenter, ImageSizeModeEnum.Scale);
|
|
break;
|
|
case "absolute":
|
|
break;
|
|
default:
|
|
break;
|
|
}
|
|
}
|
|
}
|
|
}
|
|
}
|